Problem Solving with C++ (10th Edition) equips readers with essential programming techniques and practical examples to develop efficient solutions in C++.
Here's a simple code snippet demonstrating a basic C++ program that sums two integers:
#include <iostream>
using namespace std;
int main() {
int num1, num2, sum;
cout << "Enter two integers: ";
cin >> num1 >> num2;
sum = num1 + num2;
cout << "The sum is: " << sum << endl;
return 0;
}
Understanding Problem Solving Framework
What is Problem Solving?
Problem solving is a fundamental aspect of programming that involves identifying and addressing challenges effectively. In programming, this often entails breaking down complex tasks into manageable parts, applying algorithms, and coding a solution that meets specific criteria. The ability to solve problems is what distinguishes proficient programmers from novices.
The Importance of Problem Solving Skills
Having strong problem-solving skills enhances one's ability to think critically and logically, navigate challenges efficiently, and innovate. In the rapidly evolving field of technology, these skills are essential not merely for writing code, but also for conceptualizing and creating robust, scalable applications. Mastering problem solving in C++ can open doors to numerous career opportunities.
Steps of Problem Solving
Identifying the Problem
The first step in solving any problem is to clearly identify the issue at hand. Techniques such as asking clarifying questions or using the 5 Whys technique can help articulate the problem's nature, context, and variables involved.
Breaking Down the Problem
Once the problem is defined, decomposition is a valuable technique to simplify complexities. This method involves dividing a large problem into smaller, more manageable sub-problems. By addressing these smaller sections one at a time, you can construct a solution incrementally.
Formulating a Plan
After breaking down the problem, it's time to strategize. Effective planning can involve creating flowcharts or writing pseudocode to layout the solution before actual coding begins. This step prevents confusion and clarifies the logic to follow during implementation.
Implementing the Plan in C++
With a clear plan in place, you can begin coding your solution in C++. The following code snippet illustrates a simple implementation of a plan for adding two numbers:
#include <iostream>
int main() {
int num1, num2, sum;
// User input
std::cout << "Enter first number: ";
std::cin >> num1;
std::cout << "Enter second number: ";
std::cin >> num2;
// Calculate sum
sum = num1 + num2;
// Display result
std::cout << "The sum is: " << sum << std::endl;
return 0;
}
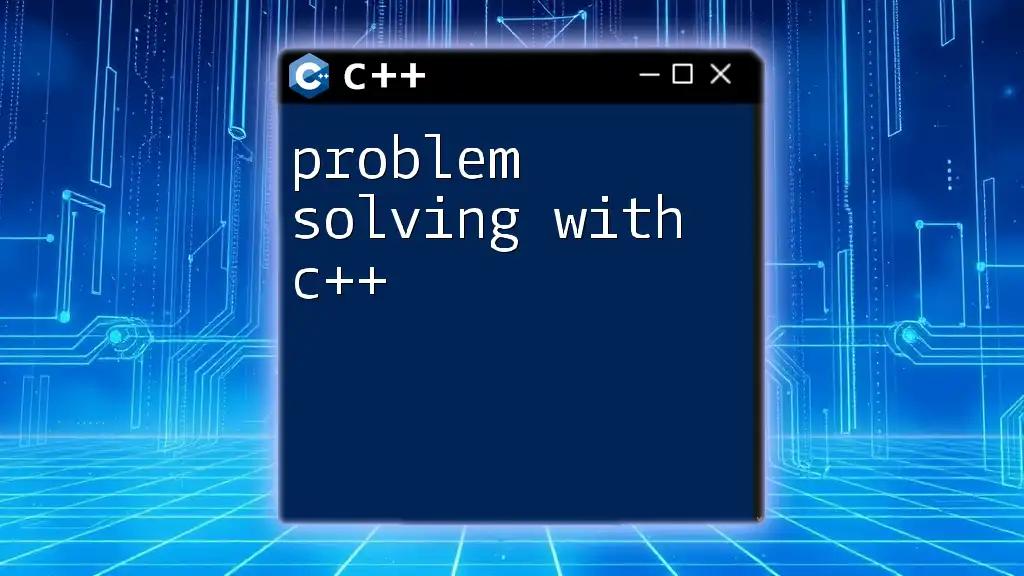
Basics of C++ Programming Language
Key Features of C++
C++ is an object-oriented programming language that allows for efficient data management and manipulation. Key characteristics include:
- Encapsulation: Bundling the data and methods that operate on the data within classes.
- Inheritance: Deriving new classes from existing ones to promote code reusability.
- Polymorphism: Allowing entities to take many forms and operate in different contexts.
Additionally, C++ provides a rich set of standard libraries like the Standard Template Library (STL), which includes pre-defined classes and functions that facilitate various programming tasks.
Data Types and Variables
Understanding data types is crucial for effective C++ programming. Common data types include:
- int for integers
- float for floating-point numbers
- char for single characters
- string for sequences of characters
Declaring and initializing these variables is straightforward. Here's an example:
#include <iostream>
#include <string>
int main() {
int age = 30; // Integer variable
float salary = 45000.50; // Float variable
char grade = 'A'; // Character variable
std::string name = "John Doe"; // String variable
std::cout << "Name: " << name << ", Age: " << age << ", Salary: $" << salary << ", Grade: " << grade << std::endl;
return 0;
}
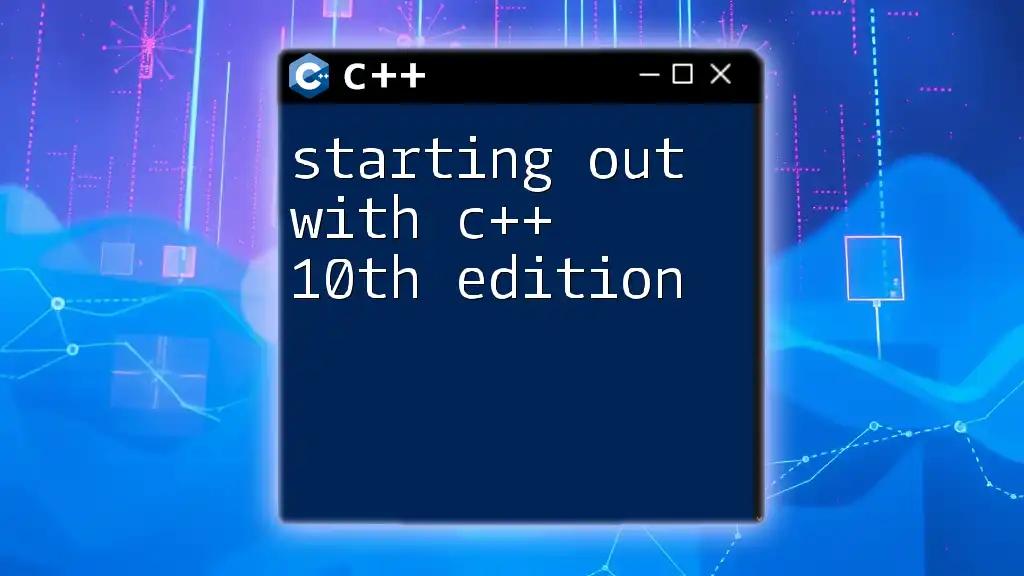
Core Problem-Solving Techniques in C++
Algorithm Design
What is an Algorithm?
An algorithm is a step-by-step procedure or formula for solving a problem. It is important to design algorithms that are both efficient and effective, leading to the desired outcomes.
Common Algorithm Paradigms
Understanding various algorithm paradigms can help you tackle problems from different angles:
- Brute Force: A straightforward method of checking all possible solutions until the correct one is found. While not always efficient, it is simple and often a starting point.
- Divide and Conquer: This technique involves breaking a problem into smaller sub-problems, solving them independently, and combining their results.
- Dynamic Programming: This method is used for optimization problems where overlapping subproblems are common. It stores the results of computed subproblems to avoid redundant calculations.
Example: Recursive Algorithms
To illustrate the concept of recursive algorithms, consider the calculation of factorial, defined as the product of all positive integers up to a given number. The recursive version can be implemented as follows:
#include <iostream>
int factorial(int n) {
if (n <= 1) return 1; // base case
return n * factorial(n - 1); // recursive case
}
int main() {
int number;
std::cout << "Enter a number to compute its factorial: ";
std::cin >> number;
std::cout << "Factorial of " << number << " is " << factorial(number) << std::endl;
return 0;
}
Data Structures
Data structures play a pivotal role in problem-solving, allowing programmers to efficiently manage and organize data. Here are some common data structures used in C++:
- Arrays and Vectors: Static and dynamic arrays for sequential data storage.
- Linked Lists: A structure of nodes that manage data in a linear fashion but allow for dynamic memory allocation.
- Stacks and Queues: Linear data structures adhering to Last In First Out (LIFO) and First In First Out (FIFO) principles, respectively.
- Trees and Graphs: More complex structures ideal for hierarchical data storage (trees) and relationships (graphs).
Example: Using Vectors
Vectors are a dynamic array implementation in C++. They can be particularly useful for operations where the size of the dataset might change over time:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {10, 20, 30, 40};
// Adding an element to the vector
numbers.push_back(50);
std::cout << "The numbers in the vector are: ";
for (int num : numbers) {
std::cout << num << " ";
}
std::cout << std::endl;
return 0;
}
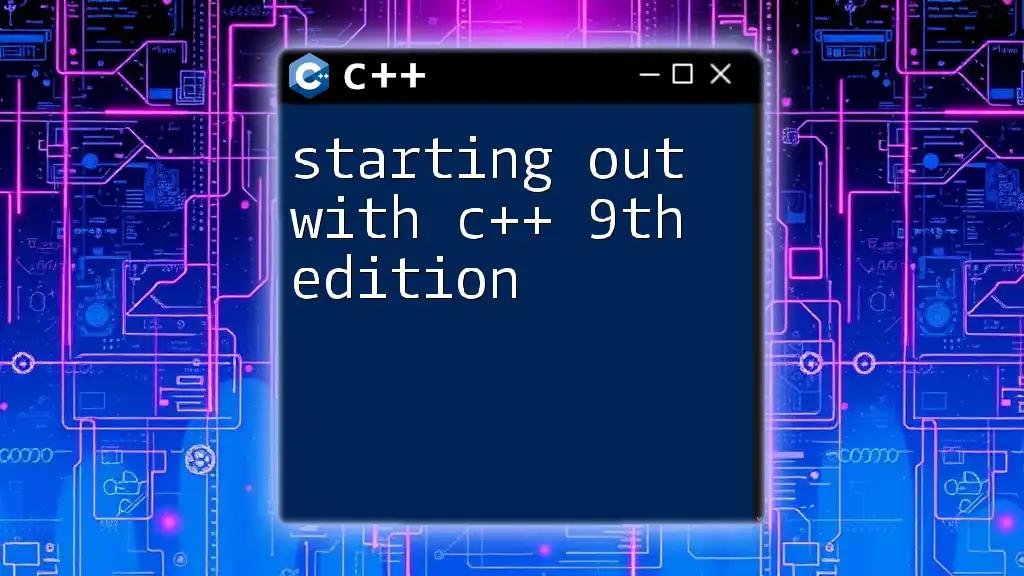
Advanced Problem Solving with C++
Handling Errors and Exceptions
Errors and exceptions are inevitable in programming. C++ provides error-handling constructs to manage unexpected behaviors.
Common Errors in C++
Understanding the distinction between syntax errors (issues with code structure) and logical errors (flaws in logic) is essential to effective debugging.
Using Try-Catch Blocks
To handle exceptions, C++ employs a mechanism called try-catch blocks. This allows programmers to write code that anticipates errors and reacts accordingly. For instance:
#include <iostream>
int main() {
int a, b;
std::cout << "Enter two numbers: ";
std::cin >> a >> b;
try {
if (b == 0) throw std::invalid_argument("Division by zero.");
std::cout << "Result: " << a / b << std::endl;
}
catch (const std::invalid_argument &e) {
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
Optimizing Solutions
Understanding Time and Space Complexity
To write efficient algorithms, one must understand time and space complexity. The Big O notation is a mathematical representation that defines the efficiency of an algorithm relative to its input size.
Optimizing Algorithms
Optimization can involve various techniques, such as reducing the number of operations or employing more efficient data structures. For example, using a hash table can dramatically reduce lookup times compared to a linear search.

Real-world Application of C++ Problem Solving
Case Study: Building a Simple Application
Let us explore the creation of a simple personal finance tracker application to illustrate real-world problem-solving in C++. The project aims to track expenses and categorize them.
Project Overview
The application will allow users to input various transactions, categorize them, summarize expenses, and offer a simple report.
Step-by-Step Implementation
- Define Requirements: Understand what features to include, such as adding expenses, viewing summaries, and categorizing entries.
- Break Down into Modules: Each feature should be implemented as a separate function or class (e.g., `addExpense`, `viewSummary`).
- Implement: Write code for each module and combine them into the main function.
Here's a snippet for adding an expense:
#include <iostream>
#include <vector>
#include <string>
struct Expense {
std::string category;
float amount;
};
std::vector<Expense> expenses;
void addExpense(const std::string& category, float amount) {
expenses.push_back({category, amount});
std::cout << "Expense added: " << category << " - $" << amount << std::endl;
}
int main() {
addExpense("Groceries", 50.75);
addExpense("Utilities", 120.00);
return 0;
}
Common Pitfalls and Best Practices
Avoiding Common Mistakes
Proficient coding requires anticipating potential pitfalls, such as:
- Undefined Behavior: Ensure variables are initialized before use.
- Memory Leaks: Manage dynamic memory carefully, utilizing delete when necessary.
Best Coding Practices
Maintaining code readability and documentation is crucial in collaborative environments. Writing clear comments, following naming conventions, and structuring code meaningfully can enhance maintainability.
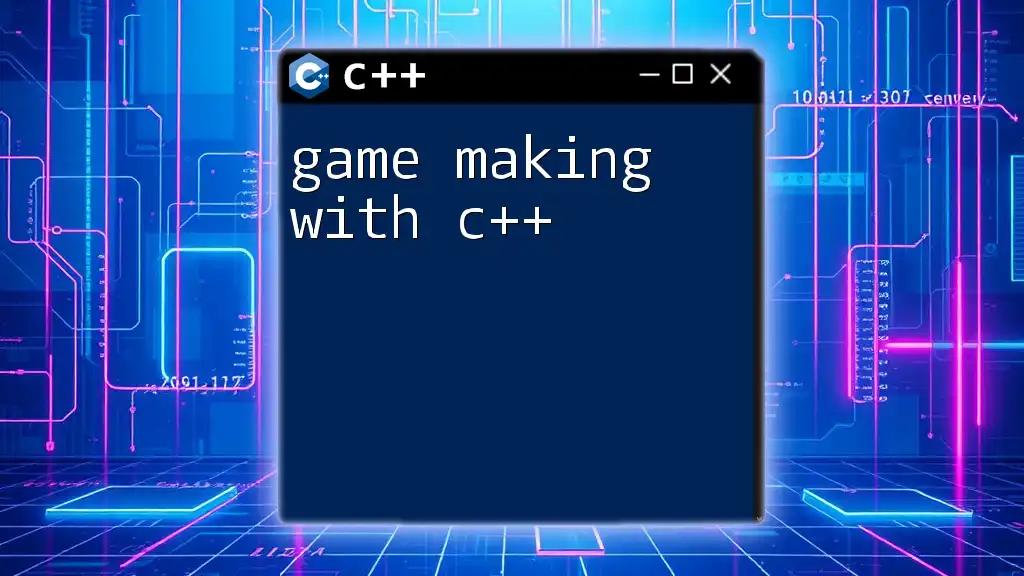
Conclusion
In summary, this comprehensive guide on problem solving with C++ (10th Edition) covers a wide array of concepts and techniques fundamental to effective programming. From identifying and defining problems, designing algorithms, leveraging data structures to real-world applications, a structured approach lays down the groundwork for success in C++ programming.
Next Steps in C++ Learning
To further cultivate your C++ skills, explore resources such as textbooks, online tutorials, and coding challenges. Engaging with the C++ community can also provide opportunities for collaborative learning and problem solving, ensuring that your skills continue to develop.

Additional Resources
For continued education, consider renowned books, tutorials, and official documentation. Furthermore, community involvement in forums or open-source projects can enhance your understanding and experience. Fostering connections within the programming community is invaluable for personal and professional growth.