A repetition structure in C++ allows you to execute a block of code multiple times based on a specified condition, typically using loops such as `for`, `while`, or `do...while`.
Here’s an example using a `for` loop in C++:
for (int i = 0; i < 5; i++) {
std::cout << "Iteration: " << i << std::endl;
}
Understanding Repetition Structures
What are Repetition Structures?
Repetition structures, commonly known as loops, are fundamental programming constructs that allow a set of instructions to be executed multiple times. They help to automate repetitive tasks, which contributes significantly to reducing code redundancy and enhancing program efficiency. By mastering these structures, developers can write more concise and effective code.
Types of Repetition Structures in C++
In C++, you can utilize several loop constructs to achieve repetition in your programs. The main types include:
- For Loop
- While Loop
- Do-While Loop
- Nested Loops
- Range-Based For Loop
Let’s explore each of these structures in detail.
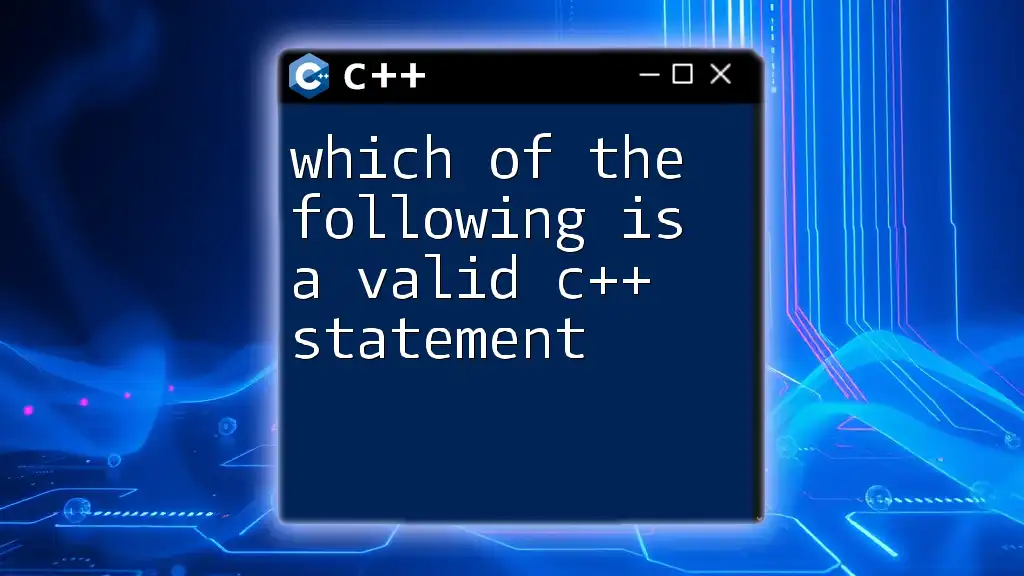
For Loop
What is a For Loop?
The for loop is particularly useful when the number of iterations is known beforehand. It comprises three main components: initialization, condition, and increment/decrement. The syntax is as follows:
for(initialization; condition; increment/decrement) {
// body of the loop
}
Code Example
for(int i = 0; i < 10; i++) {
cout << i << endl;
}
In this example, the loop initializes `i` to 0, checks if `i` is less than 10, and increments `i` by 1 after each iteration. The output will display numbers from 0 to 9.
Use Cases: The for loop is ideal for situations where you need to iterate over arrays, process elements in a collection, or perform actions a specific number of times.
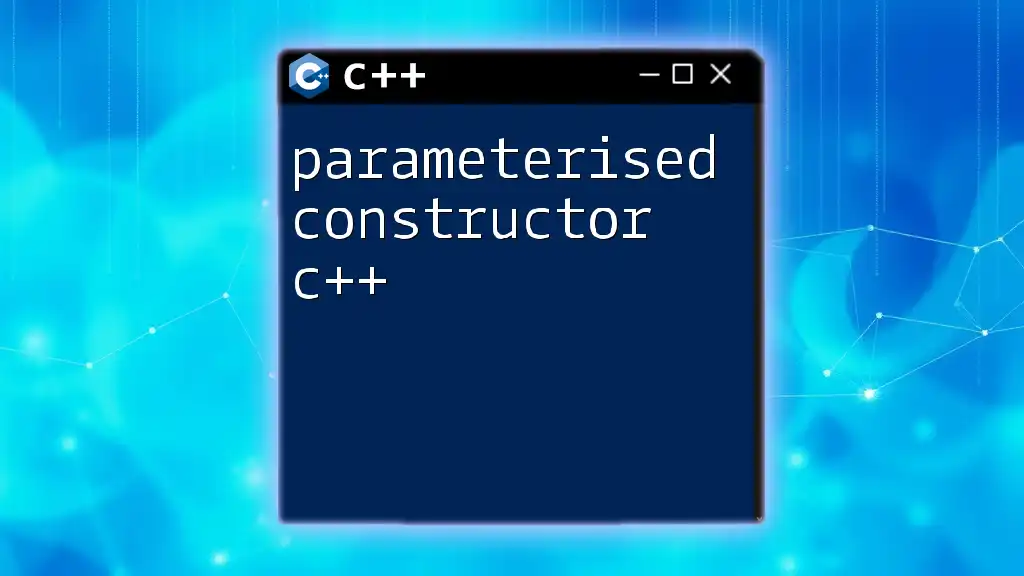
While Loop
What is a While Loop?
The while loop is suited for situations where the number of iterations isn’t predetermined. The loop continues executing as long as a given condition evaluates to true. The syntax is:
while(condition) {
// body of the loop
}
Code Example
int i = 0;
while(i < 10) {
cout << i << endl;
i++;
}
In this scenario, the loop will execute as long as `i` is less than 10, producing the same output as the previous example.
Here, it's critical to ensure that the condition eventually evaluates to false; otherwise, the loop will become an infinite loop.
Use Cases: Use while loops when the number of iterations is uncertain, such as reading data until a specific condition is met or waiting for user input.
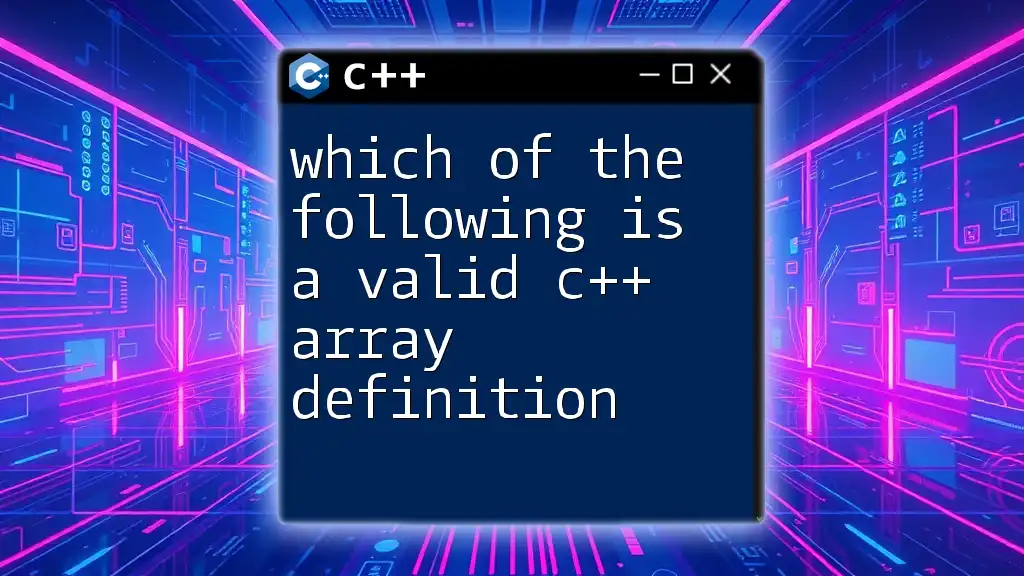
Do-While Loop
What is a Do-While Loop?
The do-while loop is unique in that it guarantees at least one execution of the loop’s body, regardless of the initial condition. Its syntax is structured as follows:
do {
// body of the loop
} while(condition);
Code Example
int i = 0;
do {
cout << i << endl;
i++;
} while(i < 10);
This code also prints numbers from 0 to 9, but it’s important to understand that the loop executes the block first before checking the condition.
When to Use: The do-while loop is particularly useful in scenarios where the loop’s body needs to execute at least once, such as input validation where you want to prompt the user initially.
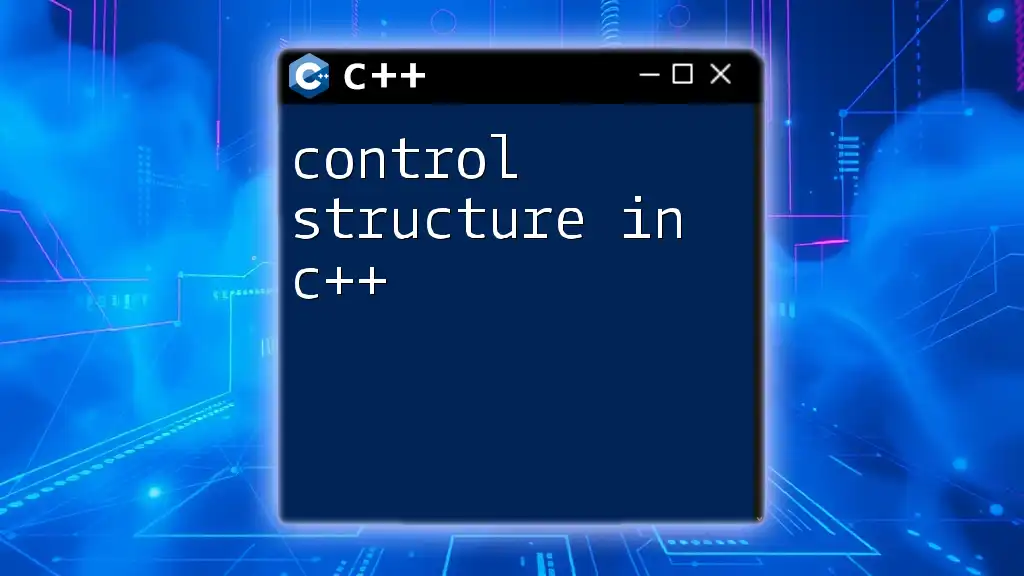
Nested Loops
What are Nested Loops?
Nested loops allow the use of one loop inside another. This can be particularly powerful for working with multi-dimensional data structures, such as matrices.
Code Example
for(int i = 0; i < 3; i++) {
for(int j = 0; j < 3; j++) {
cout << i << "," << j << endl;
}
}
In this snippet, the outer loop iterates three times, and for each iteration of the outer loop, the inner loop runs completely. The output will include pairs of `i` and `j` values representing their respective coordinates.
Use Cases: Nested loops are invaluable when handling two-dimensional arrays or managing complex data iterations, such as creating grid-like outputs or performing operations on matrices.
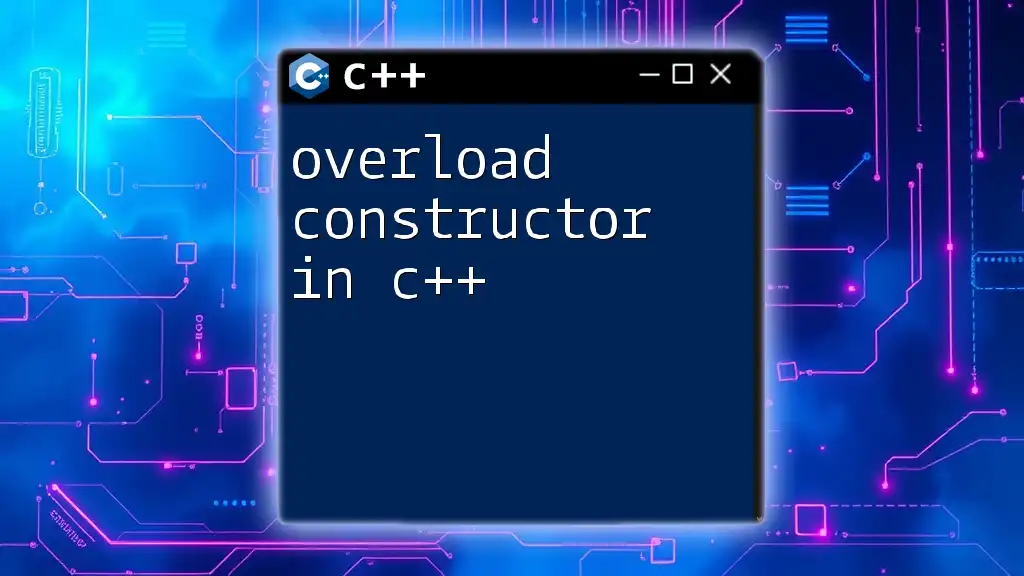
Range-Based For Loop
What is a Range-Based For Loop?
The range-based for loop was introduced in C++11 to simplify the iteration over collections like arrays and vectors. This loop eliminates the need for explicit index management, which can minimize errors and improve readability.
Code Example
vector<int> nums = {1, 2, 3, 4, 5};
for(int num : nums) {
cout << num << endl;
}
This code snippet iterates through the `nums` vector, printing each number without manually handling the indices.
Advantages: The range-based for loop enhances code clarity and is less error-prone, making it the preferred choice for straightforward iterations over collections.
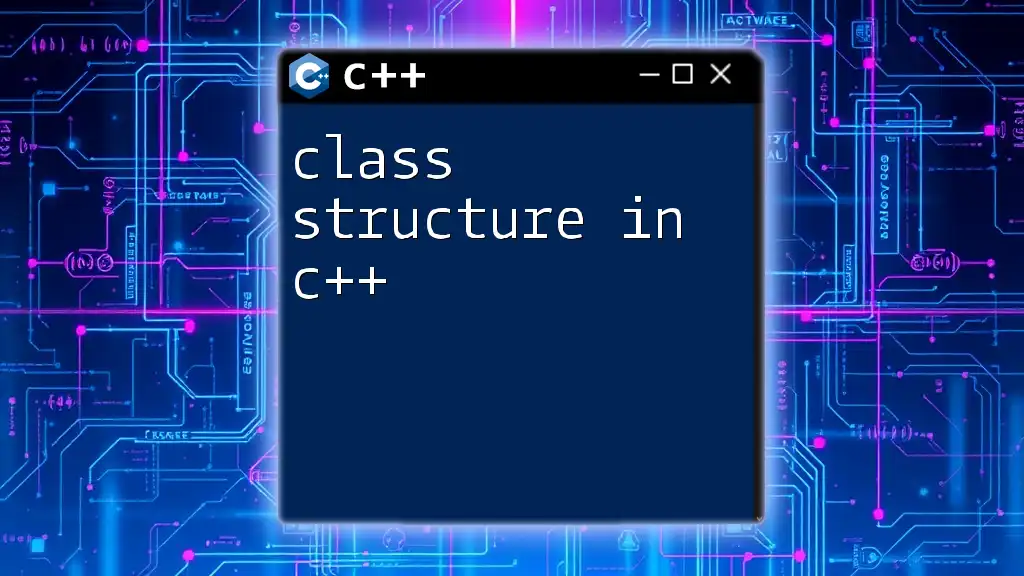
Performance Considerations
Efficiency of Different Loops
When selecting a loop structure, it’s essential to consider efficiency. For loops often have a slight advantage in terms of performance when iterating a known number of times. On the other hand, while and do-while loops can lead to increased complexity and risks of infinite loops if not implemented carefully.
Moreover, the readability of the loop is also paramount. A well-structured range-based for loop enhances clarity over traditional index-based loops, making maintenance and debugging significantly easier.
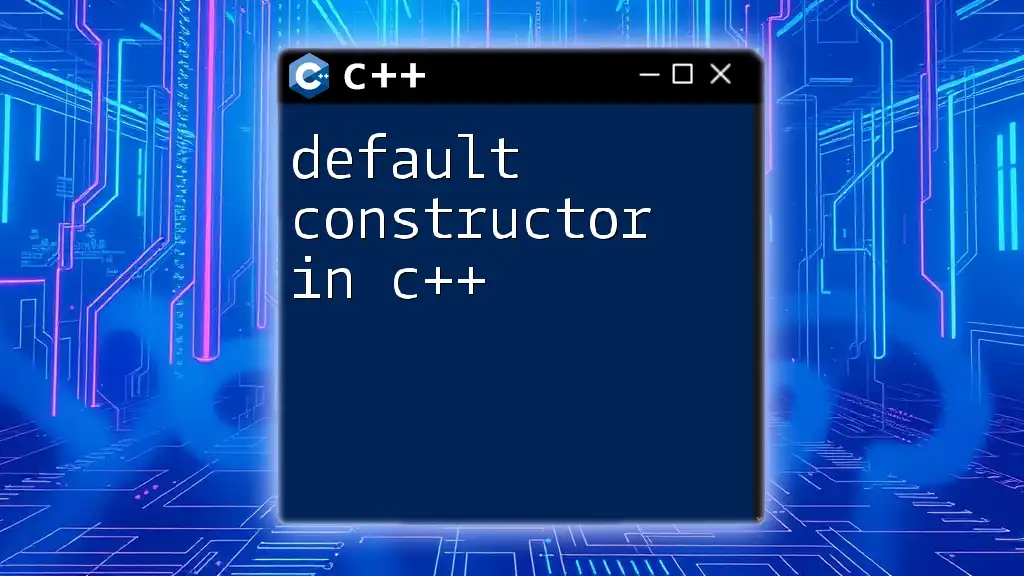
Conclusion
In C++, understanding which of the following is a repetition structure is crucial for writing efficient and effective code. By mastering the different types of loops — for, while, do-while, nested loops, and range-based for loops — you can automate repetitive tasks, optimize performance, and maintain a clear coding style.
As you continue to explore C++, invest time in practicing these structures to gain confidence in your programming skills. The ability to effectively implement repetition structures will significantly enhance your coding proficiency and overall programming experience.
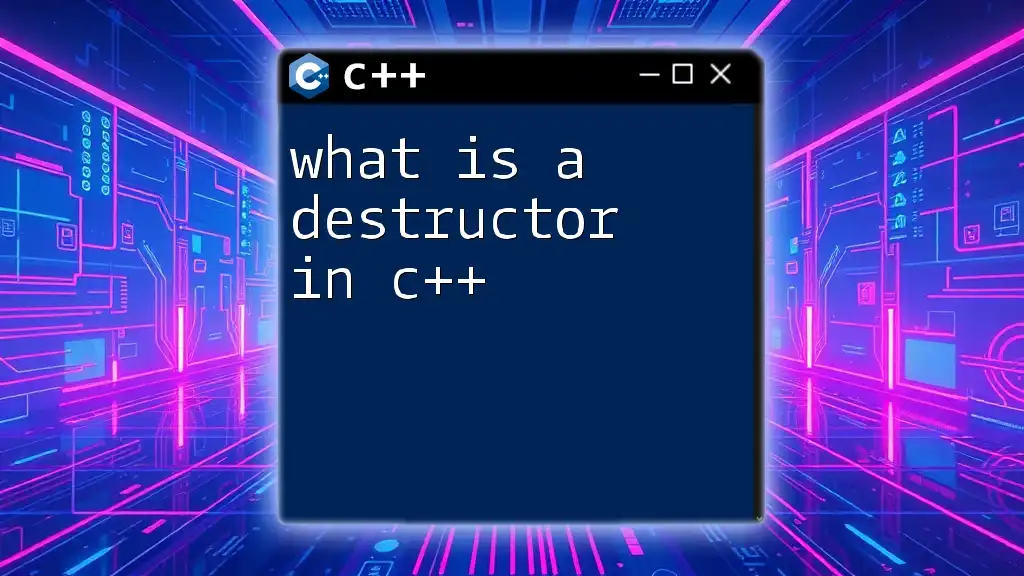
Additional Resources
For further reading and materials, consider exploring the official C++ documentation, enrolling in online C++ courses, or engaging in community forums where fellow learners share tips and experiences.
Join us in your coding journey, and let’s make learning C++ enjoyable and straightforward!