In C++, an identifier is a name given to entities such as variables, functions, and classes, and it must begin with a letter or underscore, followed by letters, digits, or underscores; therefore, the following example illustrates an invalid identifier.
int 2ndNumber = 10; // Invalid identifier - cannot start with a digit
What is an Identifier?
Identifiers in C++ are fundamental components of programming. They are used to name variables, functions, classes, or any other user-defined item. Identifiers are crucial for writing clear, readable, and maintainable code.
Definition of Identifiers
At its core, an identifier is a name given to an entity in your program. It could be a variable, function, or any other element that requires a name. For example, in the line `int age;`, the word `age` is an identifier representing an integer variable.
Rules for Valid Identifiers
To effectively use identifiers, it's essential to adhere to specific rules:
-
Identifiers must begin with a letter (A-Z, a-z) or an underscore (_): They cannot start with a digit.
-
Subsequent characters can include letters, digits (0-9), and underscores: This means that after the first character, you have more flexibility in adding numbers but still need to avoid starting with one.
-
Identifiers are case-sensitive: `Variable`, `variable`, and `VARIABLE` are considered three distinct identifiers.
-
Cannot be a reserved keyword: C++ has a list of keywords (like `int`, `return`, `class`, etc.) that cannot be used as identifiers since they have predefined meanings.
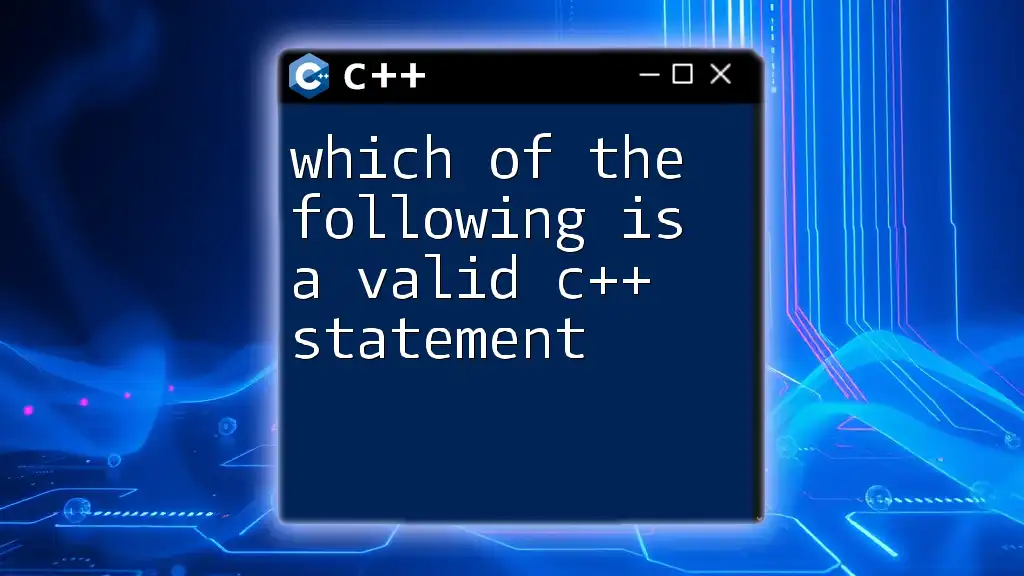
Common Mistakes to Avoid
Using Keywords as Identifiers
One of the most common pitfalls in naming identifiers is using reserved keywords. For example, trying to declare a variable like this:
int int = 5; // Invalid: 'int' is a keyword
Here, `int` is a keyword representing an integer type and cannot be used as a name for a variable. Always check the list of keywords to ensure your identifier doesn't clash with predefined terms.
Starting with Digits
Another rule is that identifiers cannot start with a digit. This restriction exists because it could create confusion with numeric literals. For instance:
int 2ndPlace = 10; // Invalid: Cannot start with a digit
In this scenario, the identifier `2ndPlace` is invalid because it starts with a digit. Always ensure that your identifiers begin with a letter or an underscore.
Special Characters Usage
C++ restricts the use of certain special characters in identifiers, which can lead to mistakes if you're not careful. Here are examples of invalid identifiers:
int my-variable = 20; // Invalid: Contains a dash (-)
int my variable = 10; // Invalid: Contains a space
In both cases, dashes and spaces are not permitted in identifiers. Stick to letters, digits, and underscores to avoid errors.
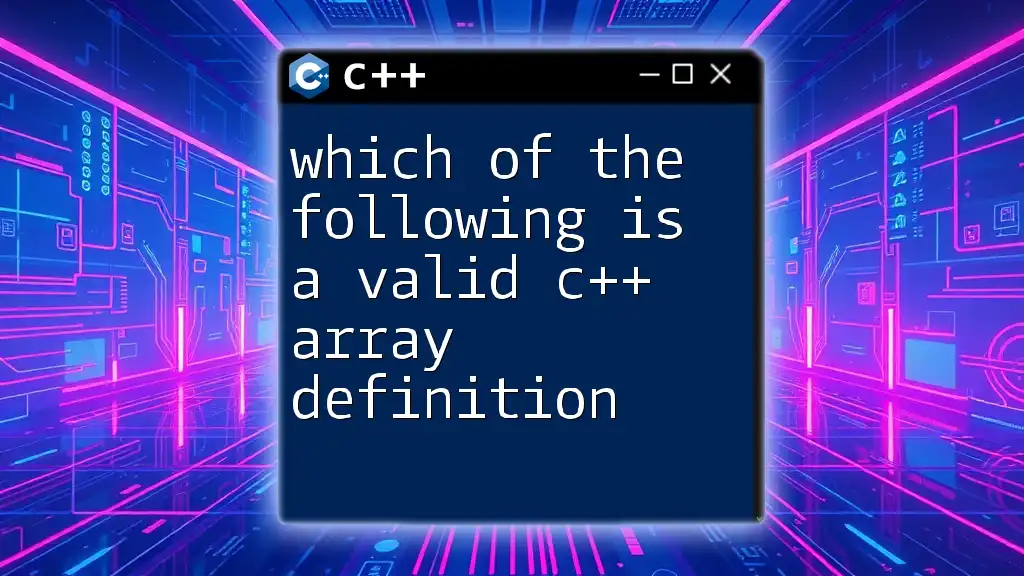
Examples of Valid Identifiers
Breakdown of Valid Identifier Examples
Valid identifiers are those that conform to the rules mentioned above. They can include meaningful names as well, which not only helps in avoiding errors but also enhances code readability. Here are a few examples:
int playerScore = 50; // Valid identifier
float _temperature = 25.5; // Valid identifier
char name1 = 'A'; // Valid identifier
In these examples, `playerScore`, `_temperature`, and `name1` all follow the identifier rules, making them valid.
Best Practices for Naming Identifiers
Choosing appropriate names for identifiers is crucial in programming. Here are some best practices:
-
Be Descriptive: Use names that convey meaning. For instance, `totalSales` is clearer than `x`.
-
Use CamelCase: For multi-word identifiers, consider using CamelCase (e.g., `averageSpeed`) to improve readability.
-
Avoid Abbreviations: While they may save time, abbreviations can lead to confusion. Be explicit instead.
By following these practices, you enhance the understanding and maintainability of your code.
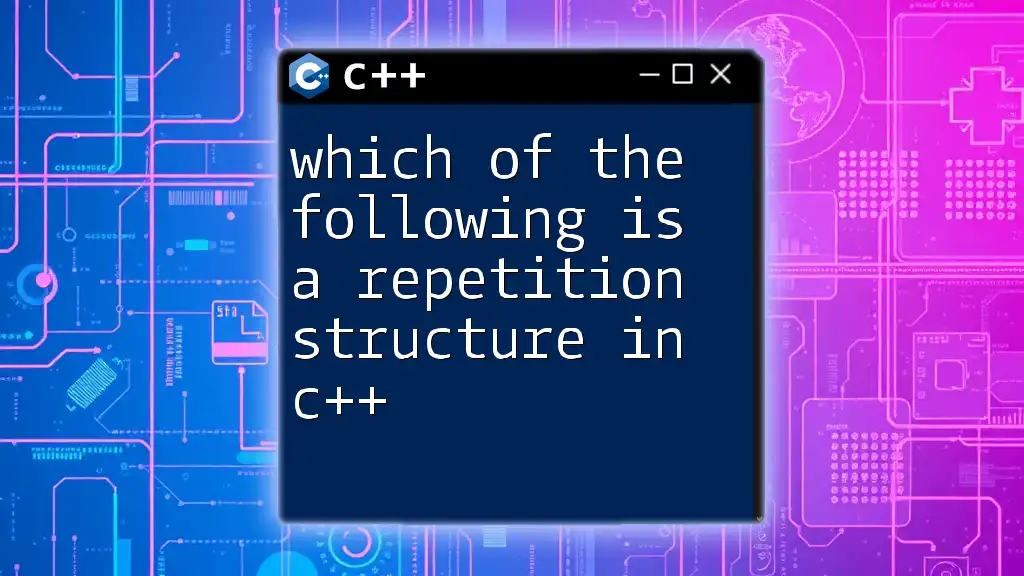
Identifying Invalid Identifiers
Common Examples of Invalid Identifiers
It’s vital to be able to recognize invalid identifiers easily. Let’s look at some that are often mistaken as valid:
double 1stNumber; // Invalid: Starts with a digit
string first-name; // Invalid: Contains a dash (-)
In the first example, `1stNumber` is invalid because it begins with a digit. The second example, `first-name`, contains a special character, which is not allowed in identifiers.
Practice Quiz: Identify the Invalid Identifier
To help solidify your understanding, consider the following identifiers and identify which one is invalid:
int validIdentifier; // Valid
int 2identifier; // Invalid
float another_validIdentifier; // Valid
In this quiz, `2identifier` is the invalid identifier because it starts with a digit.
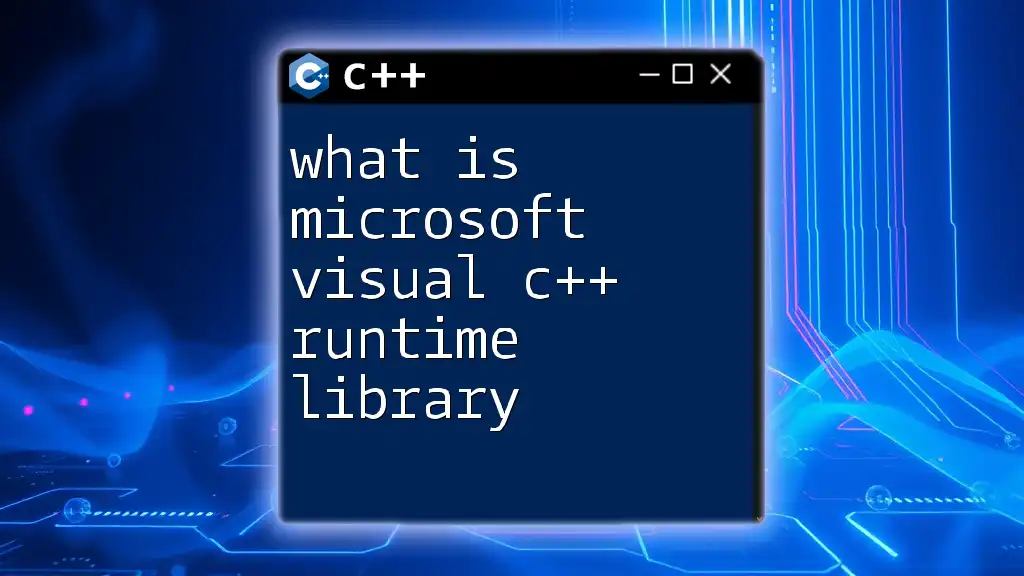
Conclusion
In summary, understanding which of the following is not a valid C++ identifier is essential for any programmer looking to write clear, maintainable code. Remember the rules governing identifiers: they should start with a letter or underscore, contain only valid characters, be case-sensitive, and not be reserved keywords. By adhering to these guidelines and avoiding common mistakes, you can enhance both the clarity and functionality of your programming efforts.
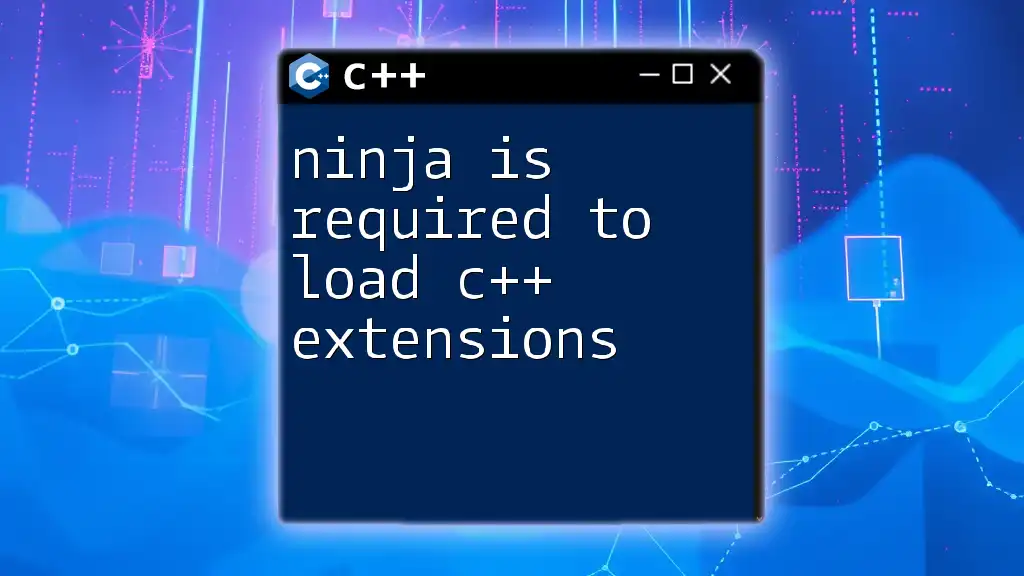
Additional Resources
Recommended Reading
To dive deeper into C++, consider exploring textbooks, online courses, and community forums dedicated to programming. These resources offer further insights into identifiers and other vital C++ concepts.
Interactive Tools
Utilizing development tools and IDEs can aid in identifying syntax errors related to identifiers. Many modern tools underline the errors, helping you learn and correct your code in real-time.