To load C++ extensions efficiently, you need the Ninja build system, which simplifies the build process with its fast and concise methodology. Here's an example of how to use Ninja to build a C++ extension:
# Create a build.ninja file with the following content
rule cxx
command = g++ -o $out $in
build my_extension: cxx my_extension.cpp
Understanding the "RuntimeError: Ninja is Required to Load C++ Extensions"
What is RuntimeError?
A RuntimeError is a type of error that occurs while a program is running, indicating something went wrong during the execution process. These errors can arise from various issues, including invalid operations, file not found exceptions, or external dependencies not being correctly set up. Understanding this concept is crucial for troubleshooting many programming scenarios.
Detailed Explanation of the Error Message
When you encounter the message "RuntimeError: ninja is required to load C++ extensions," it indicates that the Ninja build system is not available in your environment. This message can appear during the installation or execution of Python packages that rely on C or C++ extensions, often when using libraries like PyTorch, TensorFlow, or other computational frameworks.
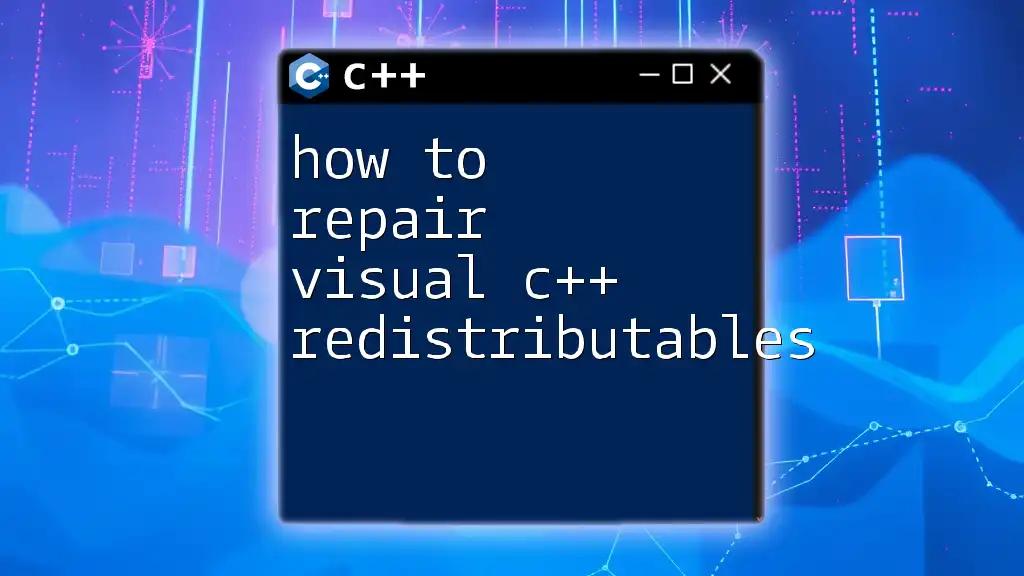
Setting Up Ninja
Installing Ninja
To use Ninja for building C++ extensions, you first need to have it installed on your system. Depending on your OS, the installation process differs slightly:
-
Installing on Windows: You can download the pre-built binaries from the [Ninja releases page](https://github.com/ninja-build/ninja/releases). Extract the binaries and add the folder to your system's PATH variable.
-
Installing on macOS: The easiest way to install Ninja is via Homebrew. Open your terminal and run:
brew install ninja
-
Installing on Linux: For most Linux distributions, you can install Ninja using the package manager. For example, on Ubuntu, you can run:
sudo apt install ninja-build
Verifying Installation
After installation, it’s vital to verify that Ninja is correctly installed. You can check by running:
ninja --version
If Ninja is installed properly, you should see the version number displayed in the terminal.
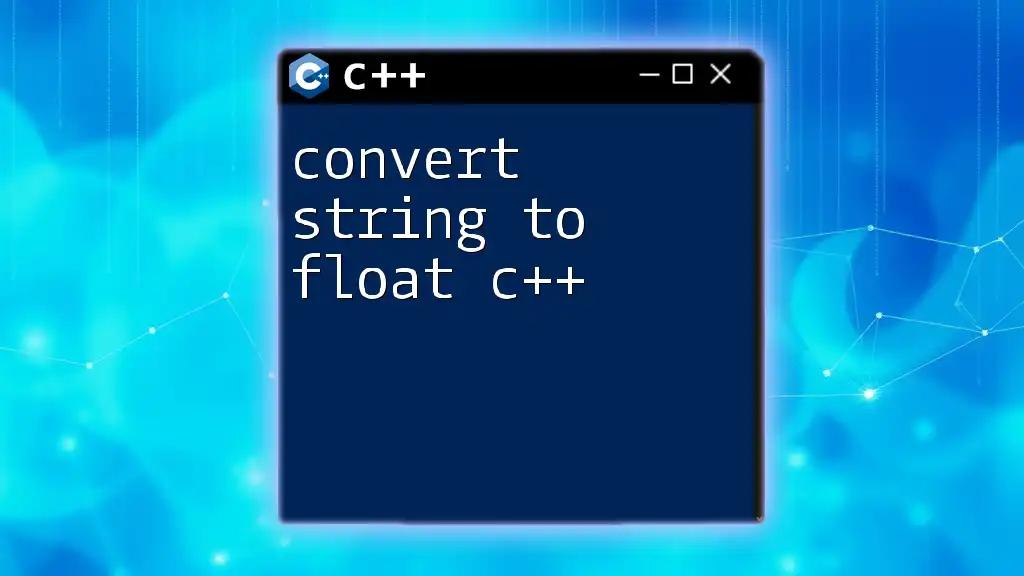
Configuring Your Environment
Setting Up Your Development Environment for C++
A well-organized development environment is essential for efficiency. Consider using a powerful Integrated Development Environment (IDE) like Visual Studio, Code::Blocks, or CLion. These tools often provide robust features for C++ development, including debugging and code maintenance.
You must also ensure that your PATH variable includes the directory where Ninja is installed. This step is crucial; otherwise, your system won’t recognize the Ninja command when you try to run it.
Using Ninja in Your Build Process
Integrating Ninja into your build process involves creating a `build.ninja` file at the root of your C++ project. This file specifies how Ninja should compile and link your extensions. Here’s a simple structure for a C++ project:
my_project/
├── src/
│ └── main.cpp
├── include/
│ └── my_header.h
└── build.ninja
A sample `build.ninja` file could look like this:
cxx = c++
cxxflags = -Iinclude
rule cxx
command = $cxx $cxxflags -o $out $in
build main: cxx src/main.cpp
This file tells Ninja how to compile `main.cpp`, including the path to the header files.
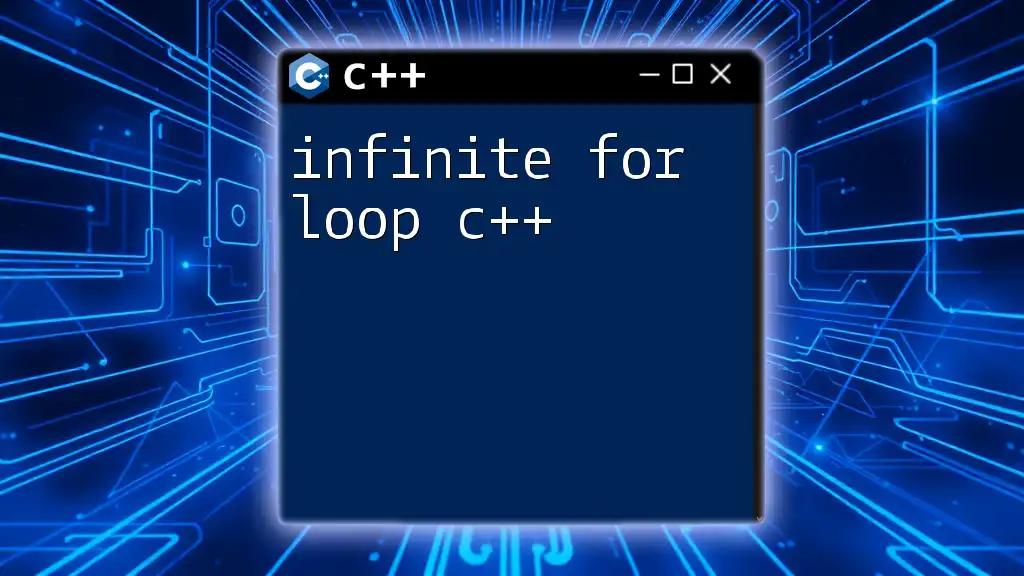
Common Issues and Troubleshooting
Steps to Resolve the "RuntimeError: Ninja is Required to Load C++ Extensions"
- Verify the Installation Path: Ensure that Ninja is installed in a directory that your OS recognizes.
- Check Environment Variables: Make sure that your PATH variable includes the directory of the Ninja executable.
- Correct Version of Ninja: Make sure you have a compatible version of Ninja for the C++ libraries you intend to use.
Other Common Errors with C++ Extensions and Ninja
While the "ninja is required to load c++ extensions" error is prevalent, you might encounter others. For instance, missing dependencies, incompatible versions, or misconfigurations in your build files can lead to additional errors. Always read the output carefully to identify issues and focus on the debug messages provided.
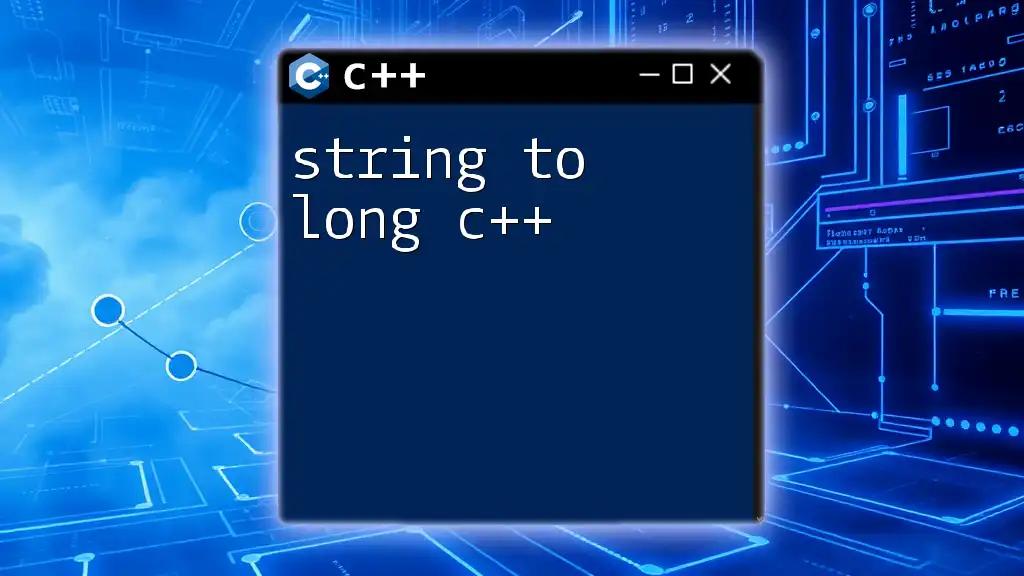
Code Snippets
Sample C++ Code Using Ninja for Extensions
Here's a simple example demonstrating a basic C++ program that uses Ninja for building:
// src/main.cpp
#include <iostream>
int main() {
std::cout << "Hello, Ninja!" << std::endl;
return 0;
}
After creating your `build.ninja` file, run Ninja in the terminal within your project directory:
ninja
If everything is set correctly, this command will compile your C++ code, generating the executable.
Making Changes to C++ Code and Rebuilding
If you modify `main.cpp`, for instance, changing the content within the `main()` function, you can rebuild your project by simply running the same `ninja` command. Ninja's intelligent build system will only recompile the files that have changed, making the process efficient.
// Updated src/main.cpp
#include <iostream>
int main() {
std::cout << "Hello, Ninja! Rebuilding..." << std::endl;
return 0;
}
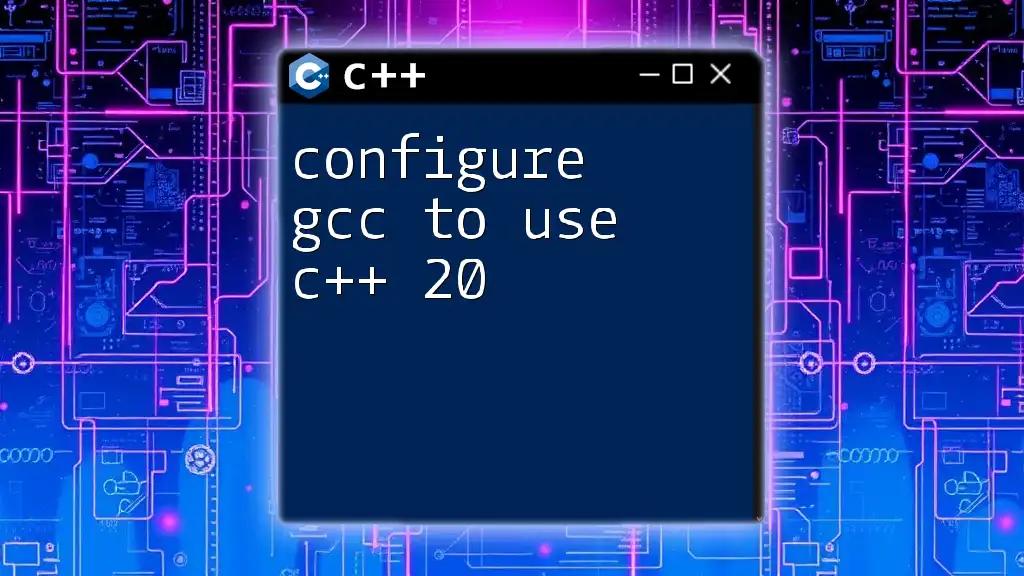
Best Practices for Working with C++ Extensions and Ninja
Organizing Your Project for C++ Extensions
A well-structured project is critical for maintaining and scaling your C++ applications. Use a clear folder structure that distinguishes between source files, headers, and build configurations. Include a README file to document your project and dependencies, which will be beneficial for future developers (or yourself) in the long run.
Maintaining Your Ninja Builds
To keep your Ninja builds efficient:
- Regularly update Ninja to utilize the latest features and bug fixes.
- Use version control (like Git) to track changes in your project files.
- Regularly clean builds by using the `ninja clean` command when you want to reset your build environment.
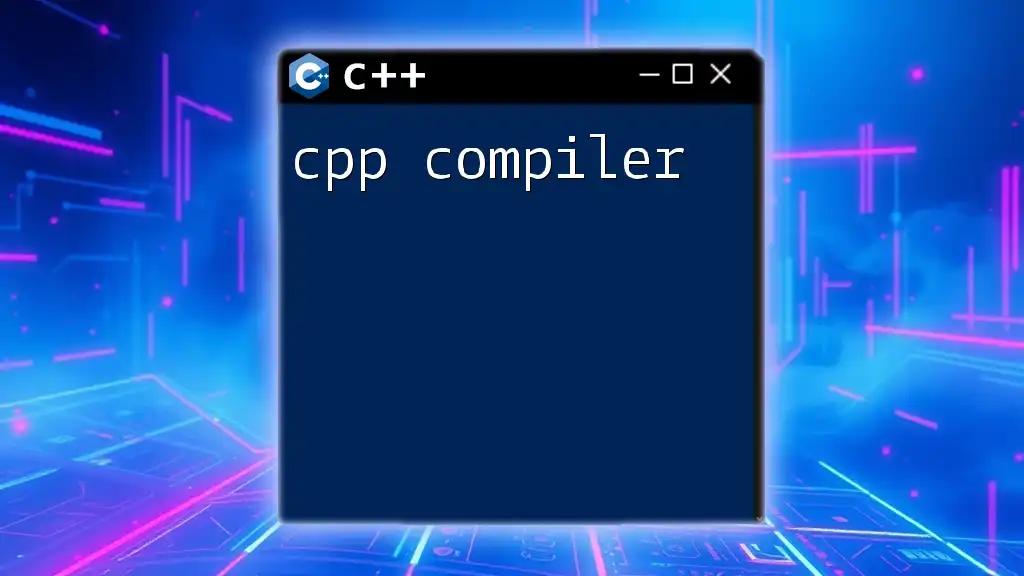
Conclusion
In summary, understanding that ninja is required to load C++ extensions is crucial for developers working with C++ in modern programming environments. Mastering Ninja not only enhances your workflow but also helps prevent common errors, like the "RuntimeError." By effectively setting up Ninja and adhering to best practices in project management and code organization, you can significantly improve your development experience and efficiency.
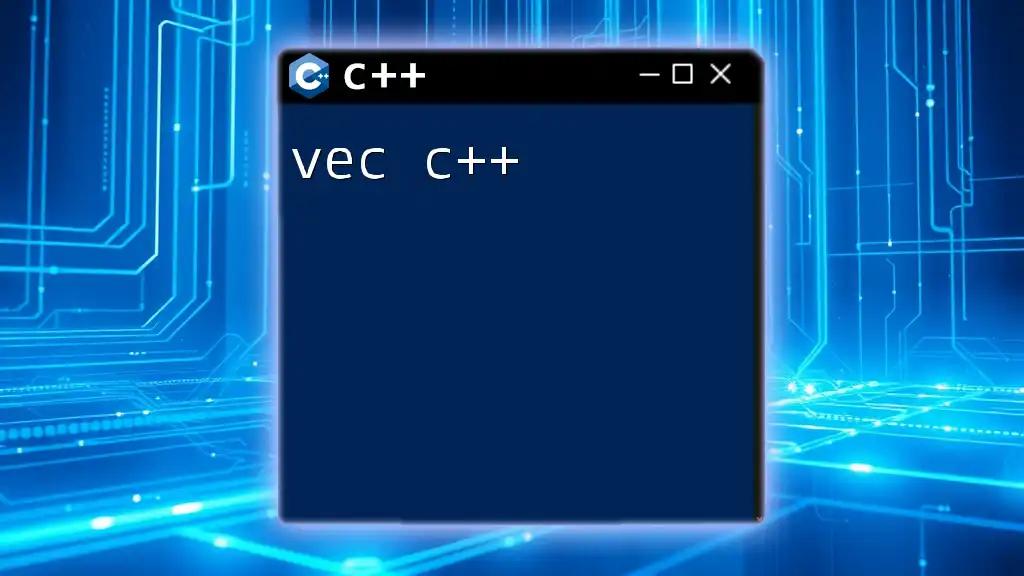
Call to Action
We encourage our readers to share their experiences and challenges faced while working with Ninja and C++ extensions in the comments below. Your insights can help shape a community of problem-solvers and learners in this evolving field. For those eager to delve deeper into C++ programming, consider exploring our courses and tutorials designed to make you a proficient C++ developer!
References
For further reading, check out the [Ninja official documentation](https://ninja-build.org/) and explore recommended resources to boost your skills in C++ programming and build systems.