To configure GCC to use C++20, you need to specify the `-std=c++20` flag when compiling your code, as shown in the following command:
g++ -std=c++20 -o my_program my_program.cpp
Understanding GCC
What is GCC?
The GNU Compiler Collection (GCC) is a free and open-source compiler system that supports various programming languages, including C and C++. It plays a pivotal role in compiling code, converting high-level languages into machine-readable format. Developers often rely on GCC for its flexibility and powerful optimization features, making it an essential tool for C++ programmers.
Features of GCC Supporting C++20
C++20 brings a treasure trove of new features and enhancements to the language. Some notable features you can leverage with GCC include:
- Concepts: Allow you to specify requirements on template parameters, making code more readable and easier to use.
- Ranges: Enhance the functionality of standard algorithms and simplify the process of working with sequences.
- Coroutines: Introduce a new way of writing asynchronous code, making complex tasks easier to manage.
- Modules: Provide a new way to organize code, improving compile times and preventing name clashes.
Using these features can significantly enhance performance, readability, and maintainability of your C++ programs.
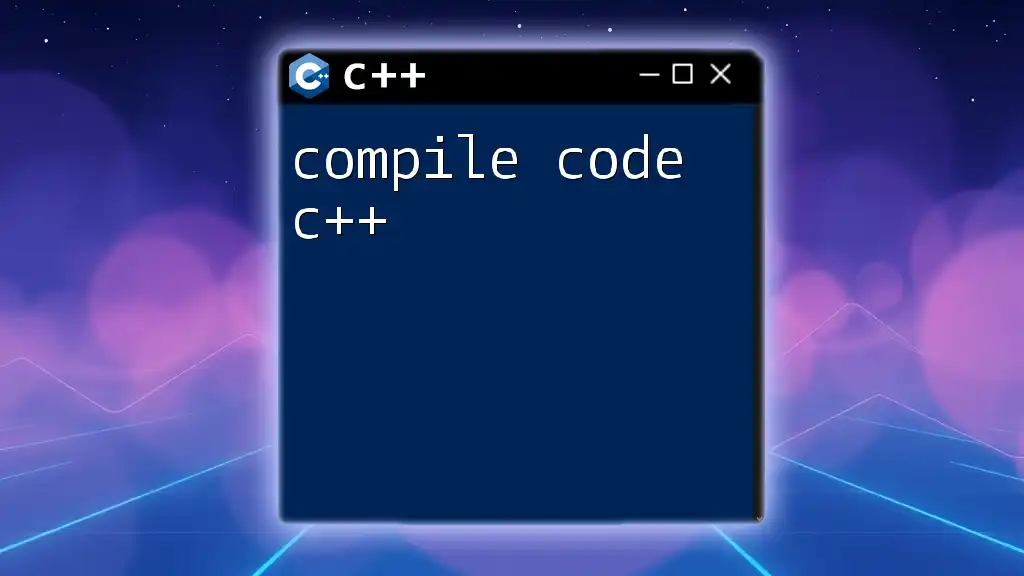
Setting Up GCC for C++20
Checking Your GCC Version
Before configuring your environment to use C++20, it's vital to check the installed version of GCC. You can do this by running the following command in your terminal:
g++ --version
For C++20 support, you need to have GCC version 10 or newer. If your version is older, you will need to upgrade.
Installing/Upgrading GCC
For Linux
If you're using a Linux distribution, upgrading or installing GCC can typically be achieved via the package manager. For example, if you're using Ubuntu or Debian, run:
sudo apt update
sudo apt install g++
For Red Hat-based distributions, you might use:
sudo yum install gcc-c++
For macOS
On macOS, using Homebrew is the most efficient way to manage software packages. You can install or upgrade GCC with the following command:
brew install gcc
If you already have GCC installed, you may use:
brew upgrade gcc
For Windows
To get GCC on Windows, one of the most common methods is to install the MinGW (Minimalist GNU for Windows) package. You can download it from the MinGW website, install it, and ensure that its bin directory is included in your system's PATH environment variable.
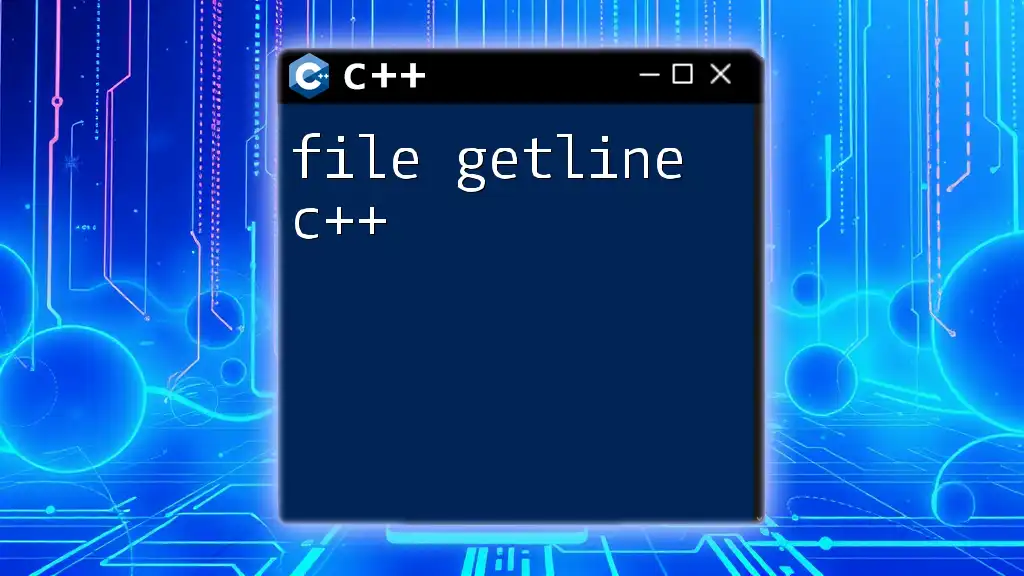
Configuring GCC for C++20
Default Compiler Flags
When you compile C++ code, GCC uses default flags, which may not include the specification for C++20. Understanding these flags will help you tailor the compilation process to your needs.
Specifying C++20 Standard
To enable C++20 support in your compilation process, you simply need to add the following flag when invoking the compiler:
g++ -std=c++20 your_program.cpp -o your_program
This command instructs GCC to compile `your_program.cpp` using the C++20 standard.
Additional Compiler Flags
While specifying the C++20 standard is crucial, you can enhance your compilation by using additional flags. Here are a couple useful examples:
- `-Wall`: This flag enables all warning messages, helping you catch potential issues early in the development process.
- `-O2`: This optimization flag optimizes the performance of the generated binary considerably.
By using these flags together, you can compile your program as follows:
g++ -std=c++20 -Wall -O2 your_program.cpp -o your_program
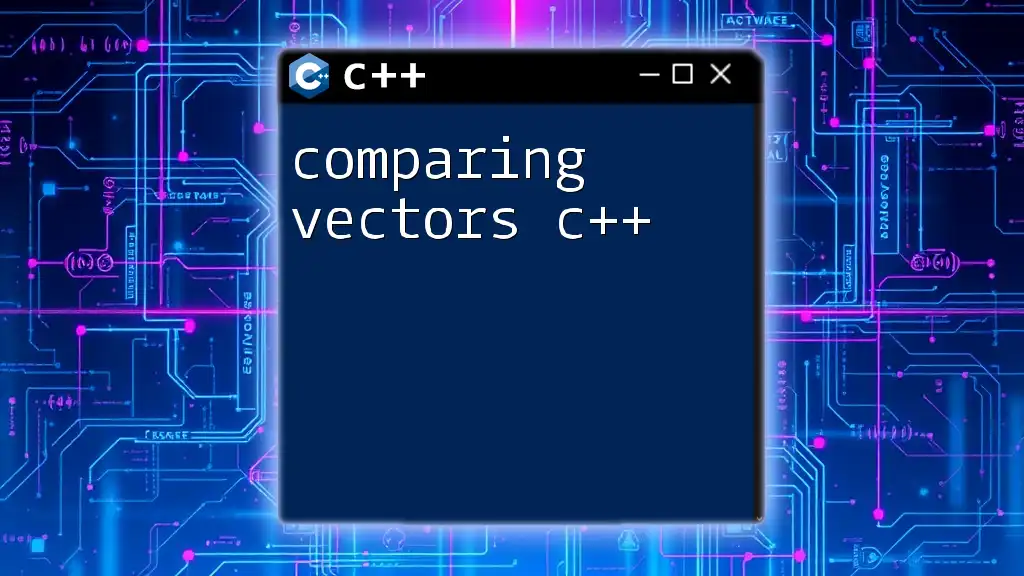
Writing a Simple C++20 Program
Sample Program: Using a C++20 Feature
Let's delve into an example that demonstrates one of C++20's exciting features—concepts. Concepts help ensure that template types meet specific criteria during compilation, enhancing code clarity.
Here’s a simple program that uses a concept to constrain a template function:
#include <iostream>
#include <concepts>
#include <vector>
// Concept to ensure the type is numerical
template<typename T>
concept IsNumber = std::is_arithmetic_v<T>;
template<IsNumber T>
T add(T a, T b) {
return a + b;
}
int main() {
std::cout << add(5, 10) << std::endl; // Output: 15
return 0;
}
In this code:
- We define a concept named `IsNumber` to specify that the type provided to the `add` function must be a numerical type.
- This approach prevents the function from being instantiated with incompatible types, leading to potential runtime errors.
Compiling and Running the Sample Program
To see your code in action, compile the sample program using the command:
g++ -std=c++20 -Wall -o sample_program sample_program.cpp
Once compiled successfully, run the program with the following command:
./sample_program
You should see the output:
15
This result confirms that our `add` function properly executed and returned the correct sum.
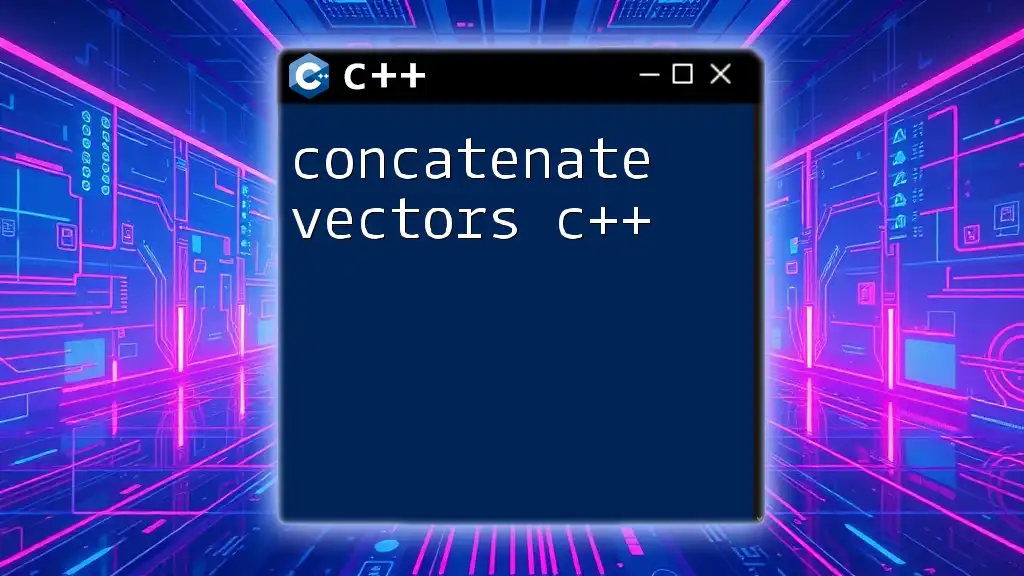
Common Issues and Troubleshooting
Compilation Errors
When working with C++20 features in GCC, you may encounter various compilation errors, particularly if your GCC version does not support C++20 or if the code uses syntax that is not recognized. Pay attention to error messages which can provide vital clues.
Tips to resolve common issues:
- Ensure that you are using the proper flags when compiling your code (`-std=c++20`).
- Check if your GCC version is compatible with the features you are trying to use.
- Consult the GCC documentation or community forums if an error message is ambiguous.
Verifying C++20 Support
To ensure that GCC is correctly configured for C++20, you can run a small test program that utilizes a known C++20 feature (e.g., concepts). If the program compiles and runs successfully, your setup is likely correct.
Additionally, refer to [cppreference.com](https://en.cppreference.com/w/cpp/20) to check relevant feature support available in your GCC version. This resource is invaluable for keeping up-to-date with C++ language developments.
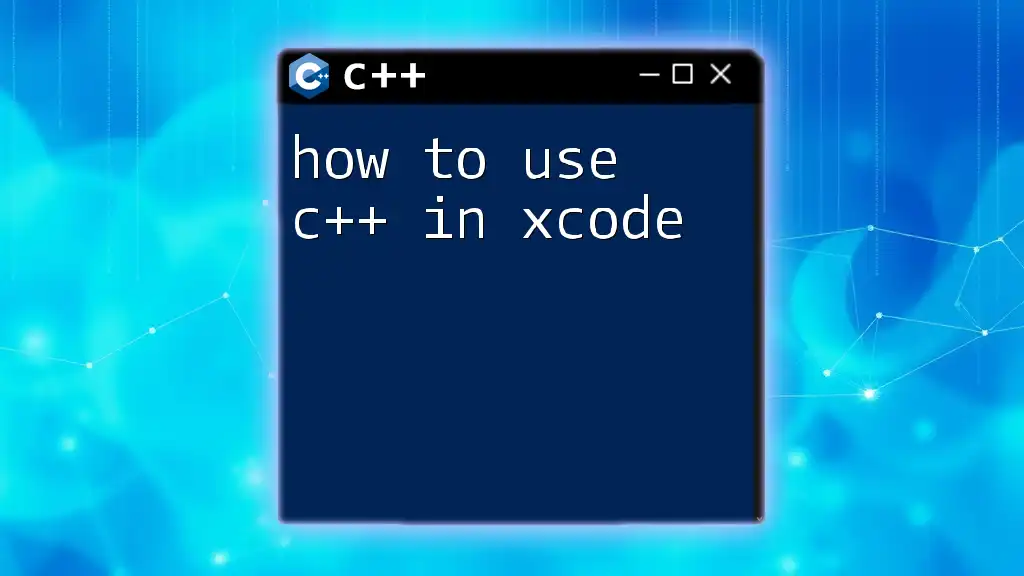
Conclusion
In this guide, you've learned how to configure GCC to use C++20 effectively. From checking your version to installing or upgrading GCC, specifying the necessary compiler flags, and even writing and running a sample program, you've equipped yourself with the knowledge to enhance your C++ programming experience.
As you dive deeper into C++20, experiment with additional features and leverage the compiler options to optimize your code. The world of C++ is vast and evolving, and staying updated with these changes is crucial for modern development practices.
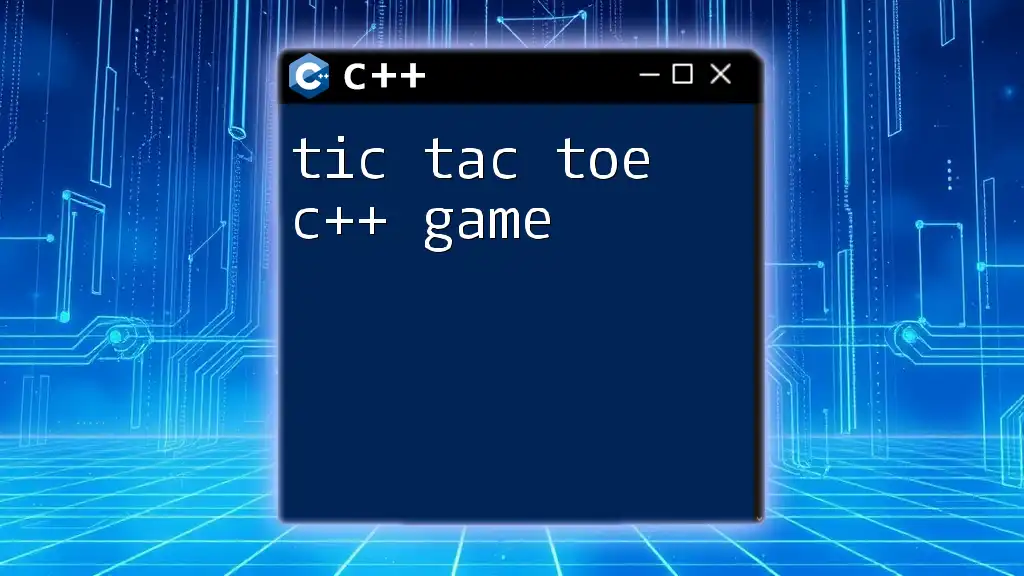
Call to Action
Subscribe for more tips and insights on C++ programming to enhance your skills further. Share your experiences with C++20 features and explore collective learning opportunities within the community. Happy coding and enjoy your programming journey!
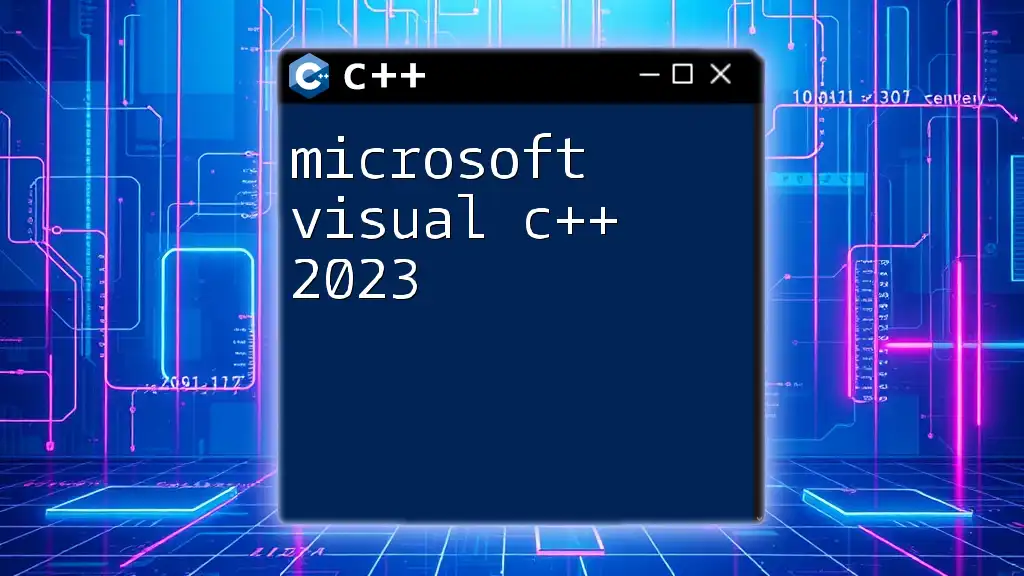
Additional Resources
For further learning, visit the following resources:
- The official GCC documentation for more in-depth explanations.
- Online forums such as Stack Overflow for troubleshooting and community support.
- C++20 feature lists to familiarize yourself with new developments.