To compile and run a C++ program, use a C++ compiler like g++ to create an executable from your source code and then execute that file.
Here's a code snippet demonstrating this process:
g++ -o myProgram myProgram.cpp
./myProgram
In this example, `myProgram.cpp` is the source file and `myProgram` is the output executable.
Understanding C++ Compilation
Compilation in C++ is the process of transforming the human-readable source code into machine-readable object code. This process is crucial because the computer cannot execute high-level programming languages like C++ directly.
What happens during compilation?
The compilation process consists of three main stages:
-
Preprocessing: This is the first stage where directives such as `#include` and `#define` are handled. The preprocessor modifies the source code by including header files and replacing macros.
-
Compilation: In this stage, the actual source code is converted into object code. The compiler analyzes the code for errors and translates it into an intermediate language.
-
Linking: The linker combines all the object code files into a single executable program. It resolves references between different files and adds any necessary libraries.
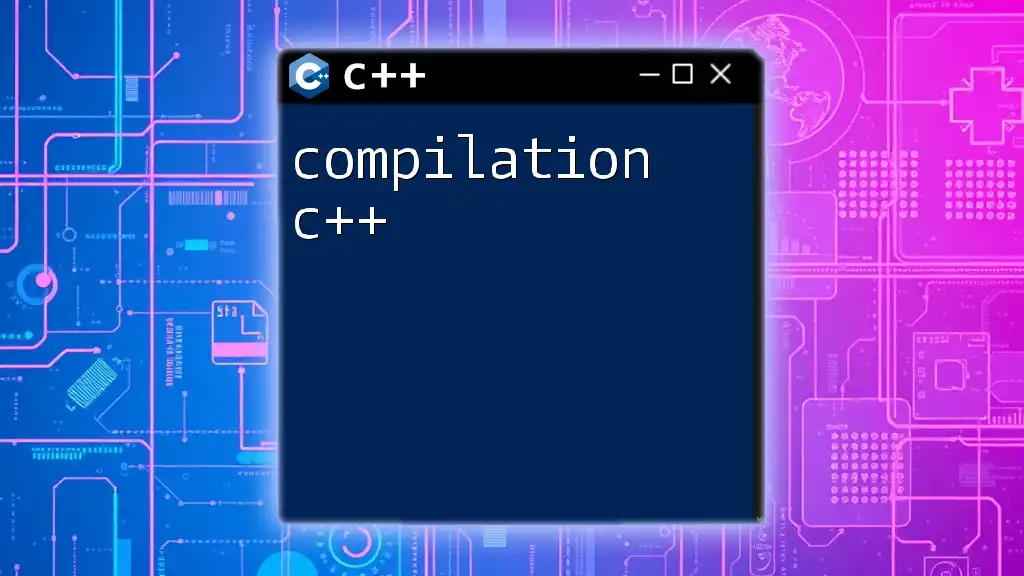
How to Compile a C++ Program
Compiling C++ using the Command Line
To compile and run C++ programs effectively, familiarity with the command line interface is essential.
Basic Compile Command
The fundamental command to compile a C++ program is as follows:
g++ -o output_name source_file.cpp
- `g++` is the GNU C++ compiler.
- `-o` specifies the name of the output executable file.
- `source_file.cpp` is the name of the C++ source file you want to compile.
Example of Compiling a C++ Program
Let’s create a simple C++ program that outputs "Hello, World!"
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
To compile this program, follow these steps:
- Save the code in a file named `hello.cpp`.
- Open your terminal and navigate to the directory where you saved the file.
- Enter the compile command:
g++ -o hello hello.cpp
If successful, this will generate an executable named `hello`.
Common Compilation Options
When compiling C++ code, you can use several options:
- `-Wall`: This flag enables all compiler warnings, helping you catch potential issues.
- `-std=c++11`: Specifies the version of the C++ standard to use. This is helpful if you want features from a particular version.
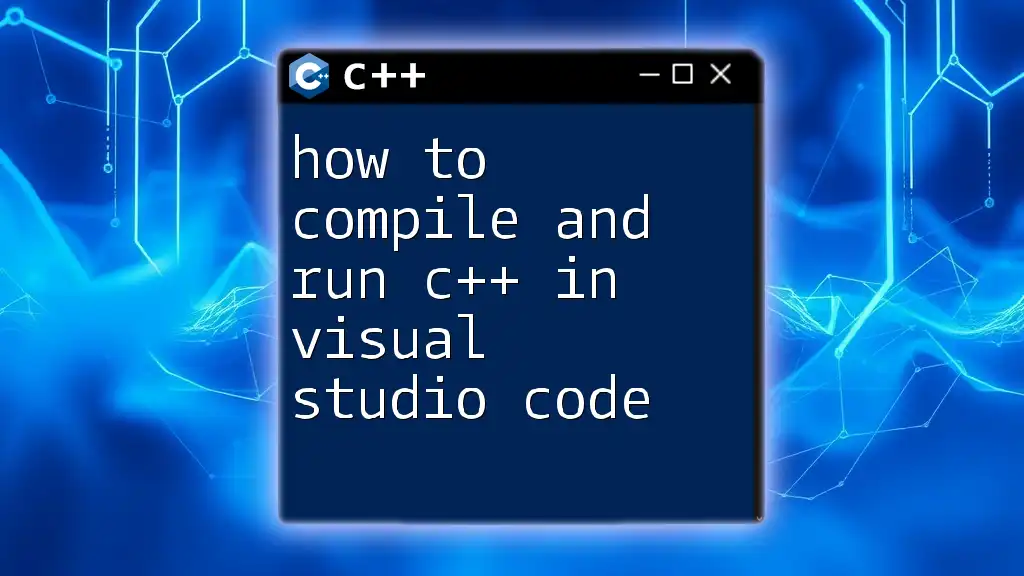
Running C++ Programs
How to Run Compiled C++ Programs
After compiling a program, the next step is to execute it. You can run the compiled executable using the following command:
./output_name
For example, to run our previously compiled program:
./hello
Example: Executing the Compiled C++ Program
After compiling `hello.cpp` and creating `hello`, execute it by entering:
./hello
Expected output:
Hello, World!
This message confirms that your program executed successfully, showcasing your ability to compile and run C++ programs.
Logging and Error Handling
If the compilation fails, the compiler will display error messages. Understanding these messages is crucial to debugging your code. Common errors include syntax errors, undeclared variables, or type mismatches.
For instance, if your code contains an error like a missing semicolon, you may see an error message guiding you to the exact line needing correction.
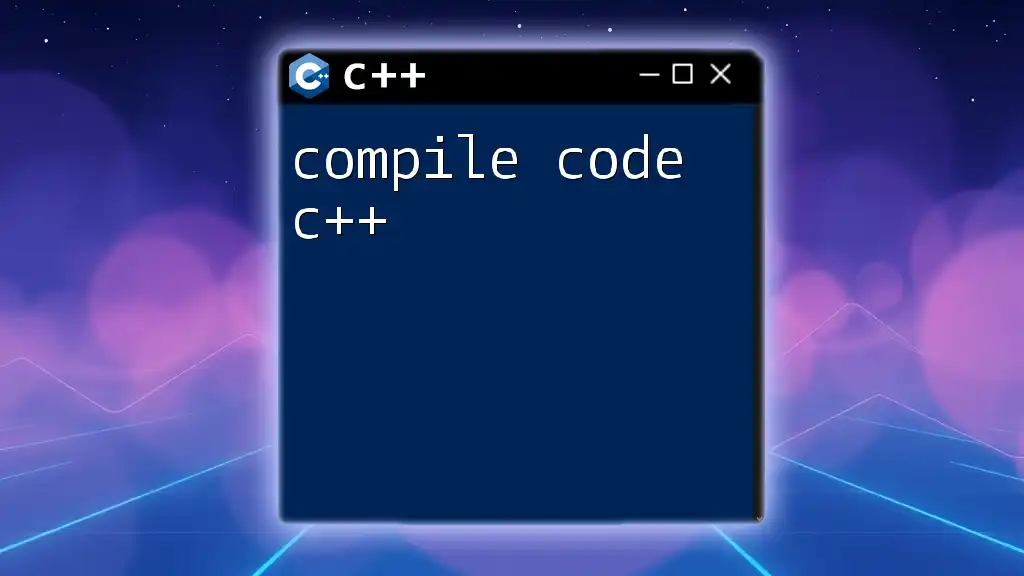
Additional Tips for Compiling and Running C++ Files
Compiling Multiple C++ Files
In larger projects, you may need to compile multiple source files together. You can do this with a command like:
g++ -o output_program file1.cpp file2.cpp
This command allows you to compile and link several C++ files into a single executable.
Compiling from an IDE
An Integrated Development Environment (IDE) can streamline the process of compiling and running your C++ programs. Popular options like Visual Studio, Code::Blocks, and Eclipse provide a user-friendly interface and built-in tools for easy compilation. Most IDEs allow you to compile your code with just a click of a button and will automatically handle linking for you.
Using Makefiles for Better Management
For projects involving multiple files, using a Makefile can significantly simplify the build process. A Makefile defines a set of tasks to be executed. Here’s a brief example of a simple Makefile:
all: main
main: main.o utils.o
g++ -o main main.o utils.o
main.o: main.cpp
g++ -c main.cpp
utils.o: utils.cpp
g++ -c utils.cpp
clean:
rm -f *.o main
With this Makefile, simply typing `make` in the terminal will compile all necessary files and link them together.
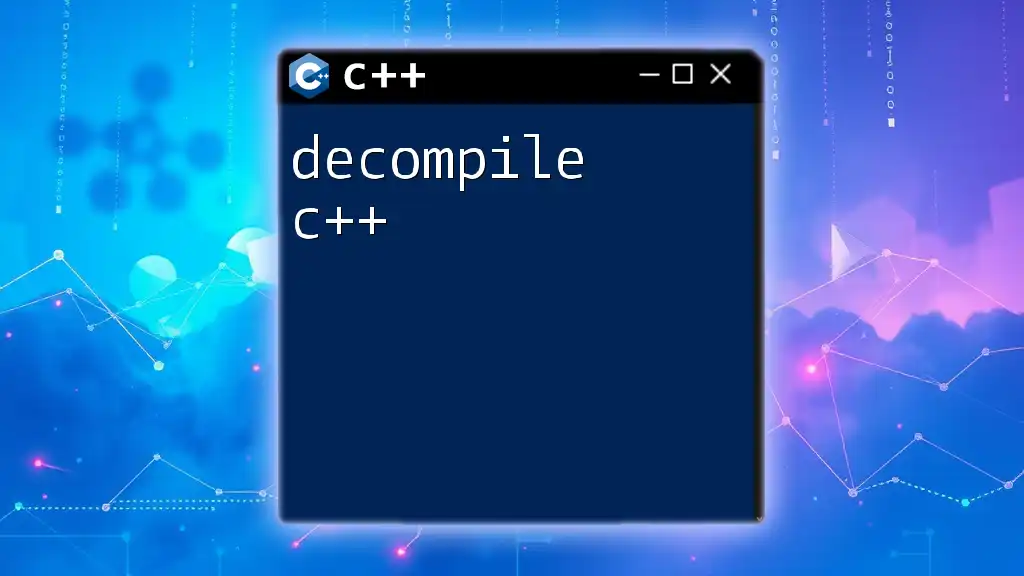
Conclusion
Compiling and running C++ programs requires understanding the compilation process and familiarity with the terminal. By following the steps outlined in this guide, you can easily compile and execute your C++ code, troubleshoot errors, and even manage larger projects with multiple files.
Now that you have the knowledge to compile and run C++ programs, it’s time to put these skills into practice by creating your own projects!