The GCC (GNU Compiler Collection) is a powerful tool that allows developers to compile C++ code into executable programs using simple command-line commands.
Here’s an example of how to compile a C++ file named `example.cpp` using GCC:
g++ example.cpp -o example
What is GCC?
GCC stands for GNU Compiler Collection. It began as a compiler strictly for the C programming language but has significantly evolved over the years to support other languages, including C++. The first version was released in 1987, marking a pivotal moment in software development.
Today, the GCC compiler is not just a simple compiler; it's a comprehensive suite that provides tools for optimizing, debugging, and managing various programming tasks in C++. It is a leading choice for developers due to its power and flexibility, making it suitable for various projects, from simple applications to complex systems software.
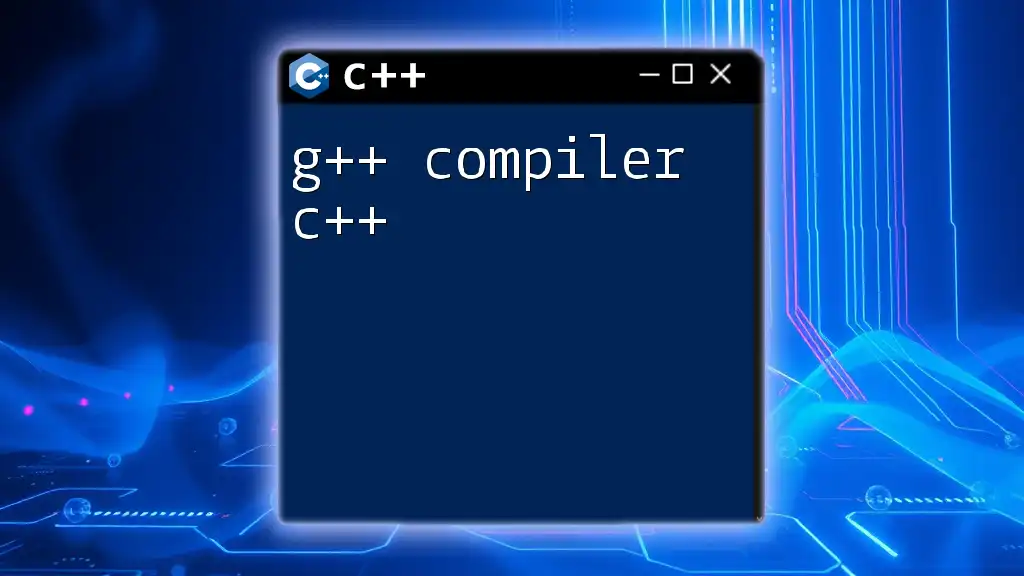
Why Use GCC for C++?
Using the GCC compiler for C++ comes with numerous advantages:
- Cross-platform compatibility: GCC runs on many systems, including Unix, Linux, Windows, and macOS, making your development experience seamless regardless of the platform.
- Open-source nature: Being open-source means that GCC can be freely used, modified, and distributed. This fosters a community-driven environment where improvements are constantly made.
- Regular updates and active community support: The GCC is continually updated with new features, bug fixes, and optimizations. A vibrant community also means more resources and user-generated content for learning and troubleshooting.
- Performance benefits: GCC’s optimization capabilities allow developers to write efficient code that performs well across various hardware architectures.
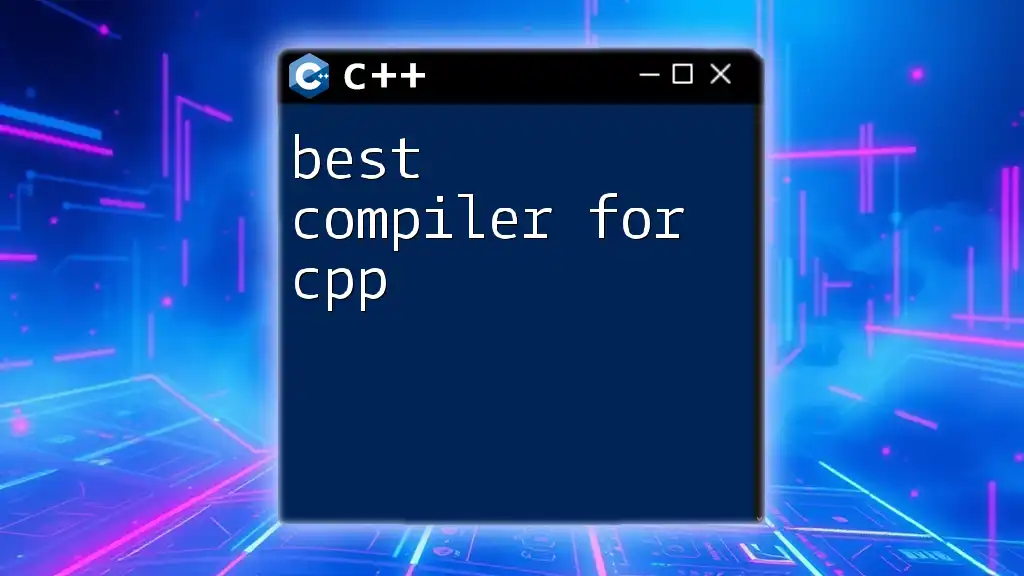
Getting Started with GCC
Installing GCC
Before diving into using the gcc compiler for C++, you need to install it on your system. Here’s a quick guide for different operating systems:
- Windows Installation via MinGW or Cygwin: These are popular environments for running GCC on Windows. MinGW can be installed by downloading the installer and following the setup instructions. Cygwin is similar; download the installer and select GCC from the package manager.
- Linux Installation using package managers: On Debian-based systems, you can install GCC with the command:
For Red Hat-based systems, use:sudo apt update sudo apt install g++
sudo yum install gcc-c++
- macOS Installation using Homebrew: First, you will need to install Homebrew if you haven’t. Then, run:
brew install gcc
After installation, verify it by typing `g++ --version` in your terminal or command prompt.
Basic Syntax of GCC Commands
Understanding the structure of a typical GCC command is essential. A basic command might look like this:
g++ -o output_file source_file.cpp
Here’s a breakdown:
- `g++`: Calls the GCC C++ compiler.
- `-o output_file`: Specifies the name of the output file.
- `source_file.cpp`: The C++ source file to compile.
Compiler flags modify the behavior of the GCC compiler. Here are a few commonly used flags:
- -Wall: Enables all compiler warnings, helping catch common issues early.
- -g: Includes debugging information useful for GDB.
- -O2: Activates optimization, improving the runtime performance of the compiled code.
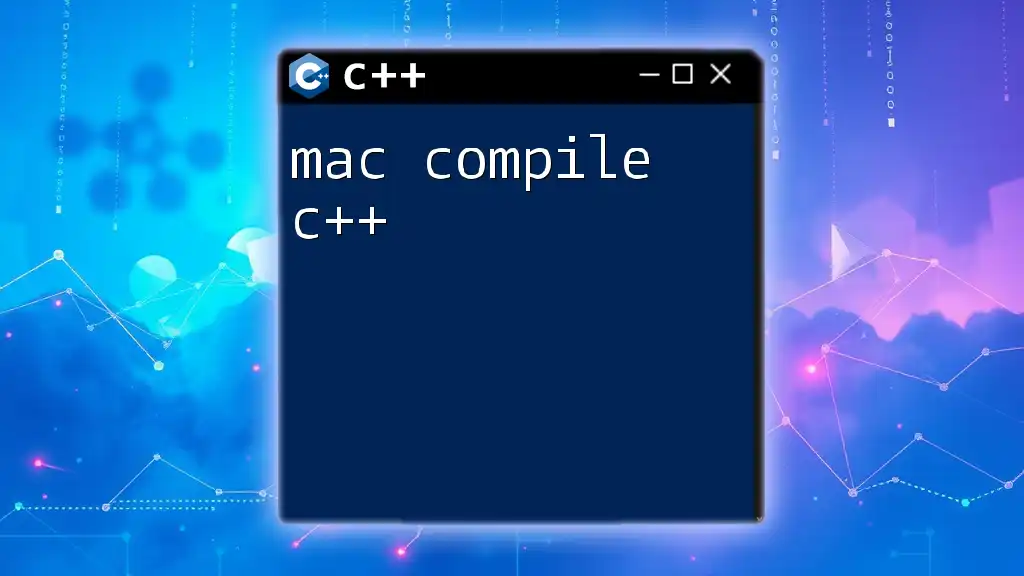
Compiling C++ Code with GCC
Compiling a Basic C++ Program
Let’s take a look at a simple C++ program to see how it works with GCC. Below is a snippet of the code:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
To compile this program using the GCC compiler for C++, you would navigate to the directory containing `hello.cpp` and run the following command:
g++ hello.cpp -o hello
This command tells GCC to compile `hello.cpp` and create an executable named `hello`. After running it, you can execute your program by typing `./hello` in your terminal.
Common GCC Command Options
As you become more familiar with the GCC compiler, you’ll find the following command options incredibly useful:
- -o: This flag allows you to specify the name of the output file. If omitted, GCC will create an output file named `a.out` by default.
- -Wall: This option enables all the compiler’s warning messages, which can help catch potential issues in your code early.
- -g: When compiling in a debugging phase, this flag includes debugging symbols in the compiled output, making it easier to debug later with GDB.
- -O2: This flag optimizes the output, which enhances performance without much increase in compilation time.
For example, to compile a program with several options, you might use:
g++ -Wall -g -O2 hello.cpp -o hello
This command will give you an executable called `hello`, while enabling warnings and debugging information, and optimizing the code.
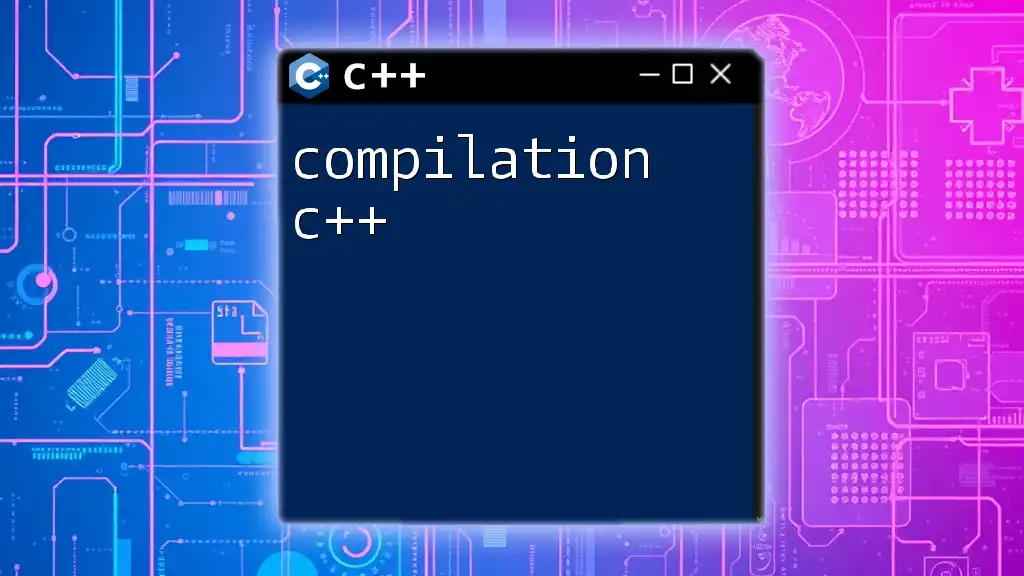
Advanced GCC Features
Multi-file Projects
For larger projects, handling multiple source files is common. Here’s an example of a basic project structure:
project/
├── main.cpp
├── utils.cpp
└── utils.h
To compile this multi-file project using GCC, you would use the following command:
g++ -o program main.cpp utils.cpp
This compiles both `main.cpp` and `utils.cpp` into a single executable called `program`. Compiling in this way helps you manage and organize your code more effectively.
Linking Libraries
When using external libraries in your projects, it’s crucial to understand the difference between static and dynamic linking. To link against an external library with GCC, you might use commands like this:
g++ main.cpp -o main -lm
In the example above, the `-lm` flag links the math library, allowing you to use mathematical functions defined in that library, such as `sin()` or `cos()`.
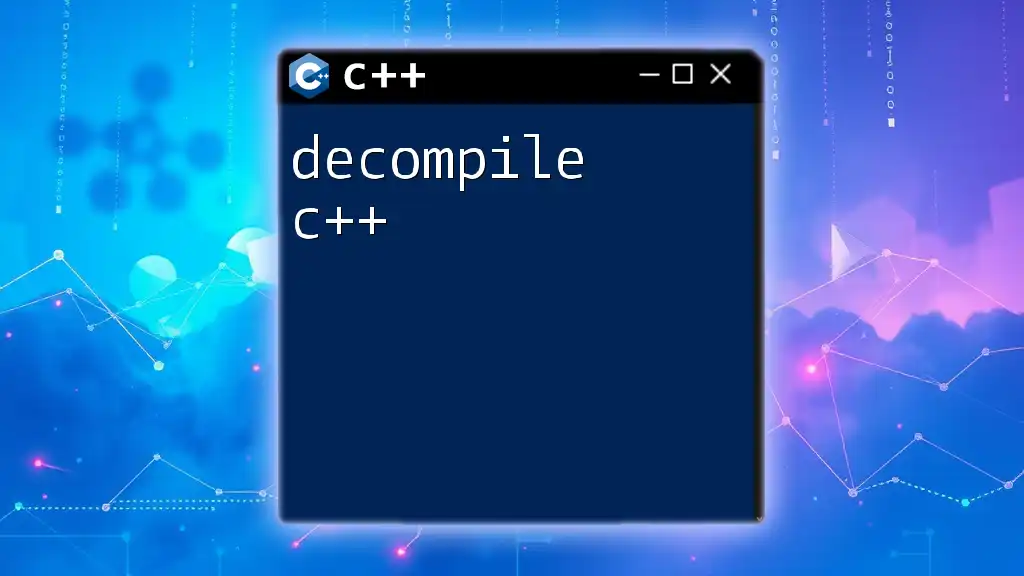
Debugging with GCC
Using the GDB Debugger
The GNU Debugger (GDB) integrates seamlessly with GCC and is invaluable for debugging C++ programs. Using GDB allows you to set breakpoints, inspect variable states, and control the execution flow of your program.
Here’s how to use GDB with your compiled code:
-
Compile your C++ code with debugging information included:
g++ -g hello.cpp -o hello
-
Start GDB with the compiled executable:
gdb ./hello
-
At the GDB prompt, you can set breakpoints and run your program. For instance, to set a breakpoint at the `main` function:
break main
-
To run the program, type:
run
-
When execution hits the breakpoint, you can inspect variables or step through the code line by line.
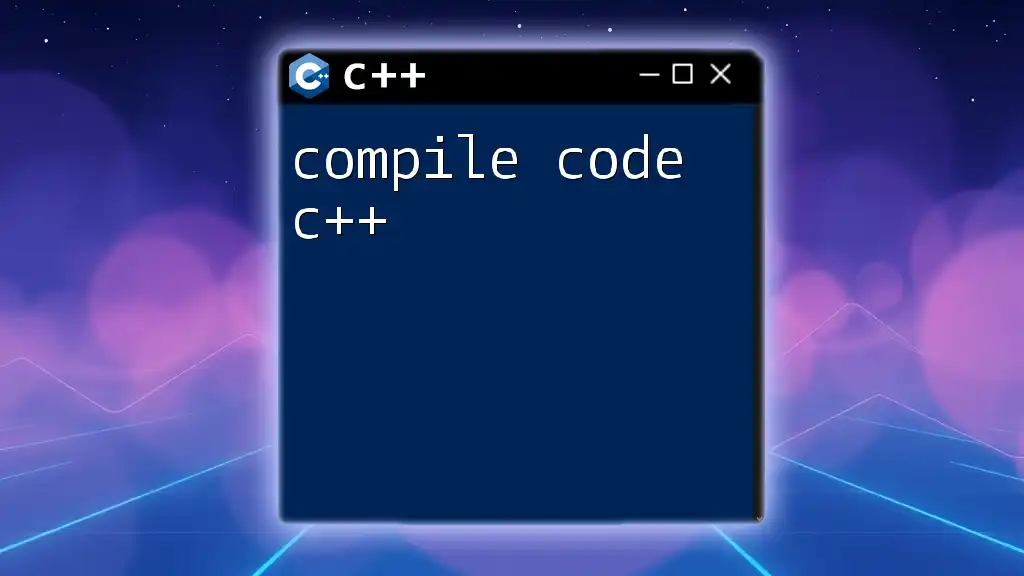
Common Errors and Their Solutions
When using the gcc compiler for C++, it's common to encounter errors. Here are a few typical issues and solutions you might face:
- Compilation errors: Common issues include missing semicolons or unmatched braces. Ensure that every statement ends correctly and that all braces are matched.
- Linking errors: These may arise from undefined references or libraries not being found. Double-check that you have included all necessary files and libraries using the correct flags.
- Example of a linking error:
Make sure you’ve compiled all relevant files and linked the correct libraries.undefined reference to 'function_name'
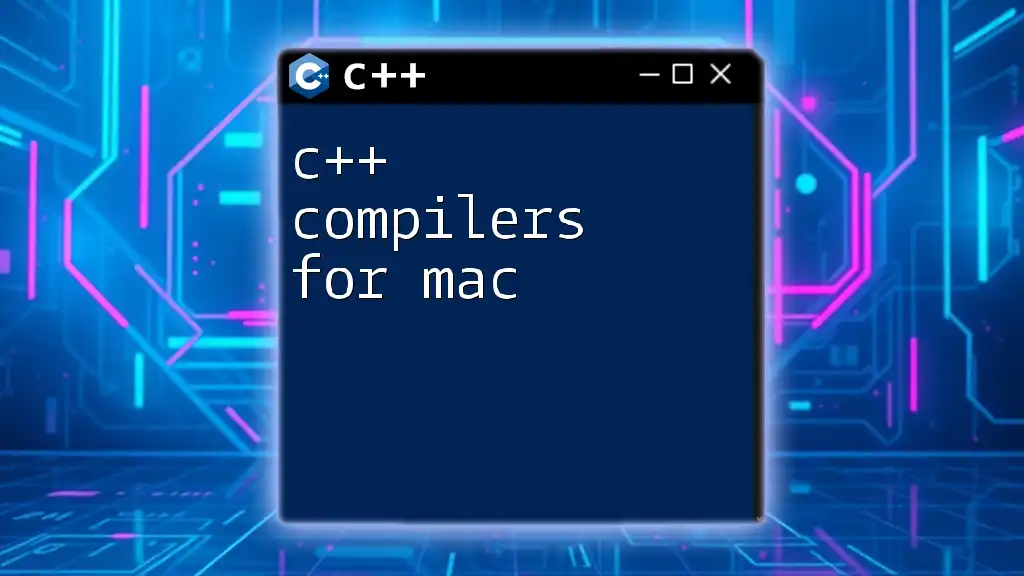
FAQs about GCC and C++ Compilation
-
What is the difference between GCC and G++?
GCC generally refers to the GNU Compiler Collection and is used for C code. G++ is the specific driver for compiling C++ code. -
Can GCC be used to compile C++ code for embedded systems?
Yes, GCC has been adapted for various architectures, including those used in embedded systems. You may need to cross-compile depending on your target environment. -
What are some common alternatives to GCC?
Alternatives include Clang, MSVC (Microsoft Visual C++), and Intel C++ Compiler. Each has unique features and optimizations.
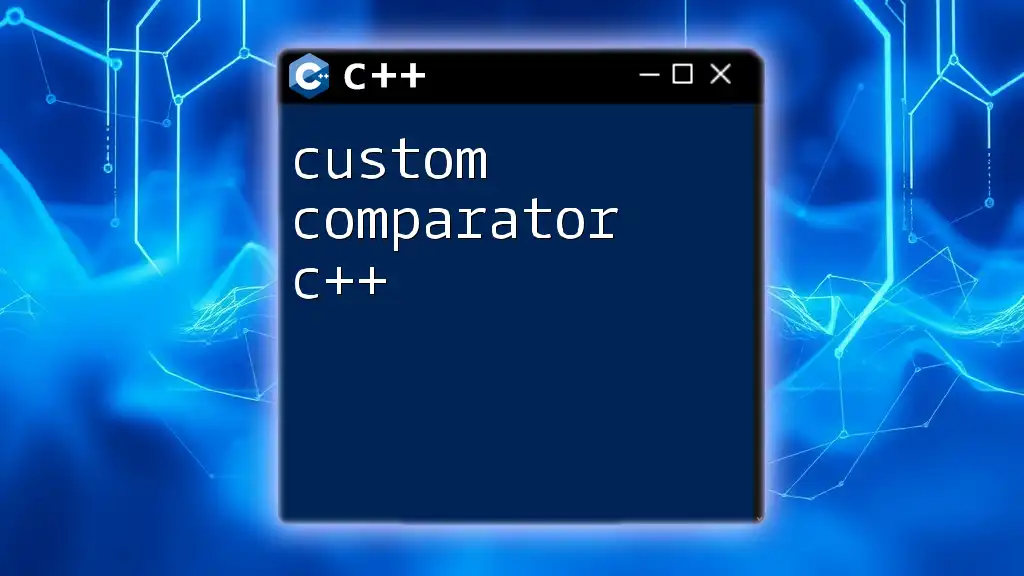
Conclusion
Mastering the gcc compiler for C++ is crucial for anyone aspiring to write efficient and robust C++ applications. Its vast feature set and active community support provide a strong foundation for developers. By familiarizing yourself with GCC, you'll be well-equipped to tackle both simple and complex C++ programming challenges.
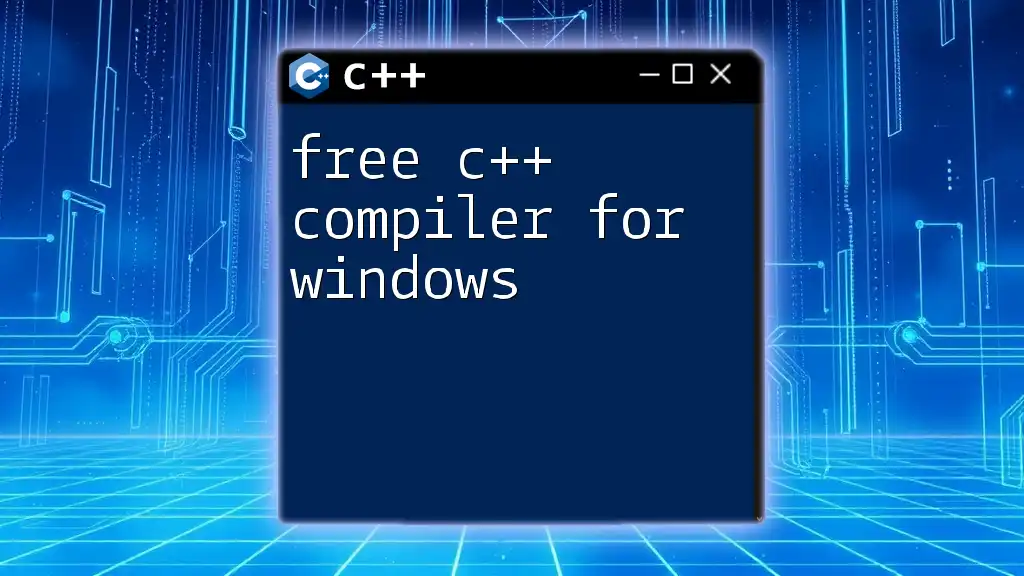
Additional Resources
For further learning:
- Visit the official [GCC documentation](https://gcc.gnu.org/onlinedocs/gcc/).
- Explore online tutorial platforms and forums focused on C++ and GCC.
- Consider books and online courses that delve deeper into C++ programming and GCC usage.