Compiler directives in C++ are special instructions that are processed by the compiler before the actual compilation begins, allowing you to include files, define constants, or conditionally compile code. Here's a code snippet illustrating the use of a compiler directive to include a header file:
#include <iostream>
Understanding the C++ Preprocessor
What is a Preprocessor?
A preprocessor is a program that processes the source code before it is compiled by the C++ compiler. It is a crucial part of the compilation process that prepares the code for the compiler by performing several tasks, such as macro expansion, file inclusion, and conditional compilation.
The distinction between the preprocessor and the compiler is essential: while the preprocessor handles directives, the compiler takes the preprocessed code and translates it into machine code.
How the C++ Preprocessor Operates
The C++ preprocessor performs its duties in stages:
- Macro Expansion: This step replaces predefined macros with their corresponding code.
- File Inclusion: It incorporates files into the source code.
- Conditional Compilation: This feature allows compiling parts of code based on certain conditions, which can be specified using directives.
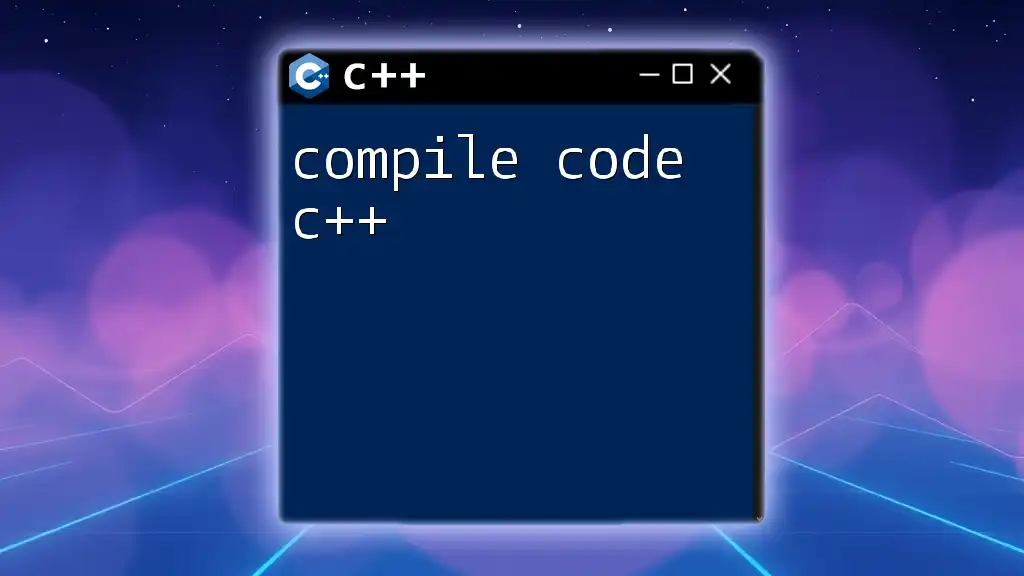
Types of Compiler Directives in C++
Understanding different types of compiler directives C++ will help you harness their power effectively. These directives are broadly categorized into several types, primarily focusing on preprocessor directives.
Preprocessor Directives in C++
Preprocessor directives are instructions that are processed by the preprocessor. Unlike normal C++ statements, they do not require a semicolon at the end and begin with the `#` symbol. Here are some commonly used preprocessor directives.
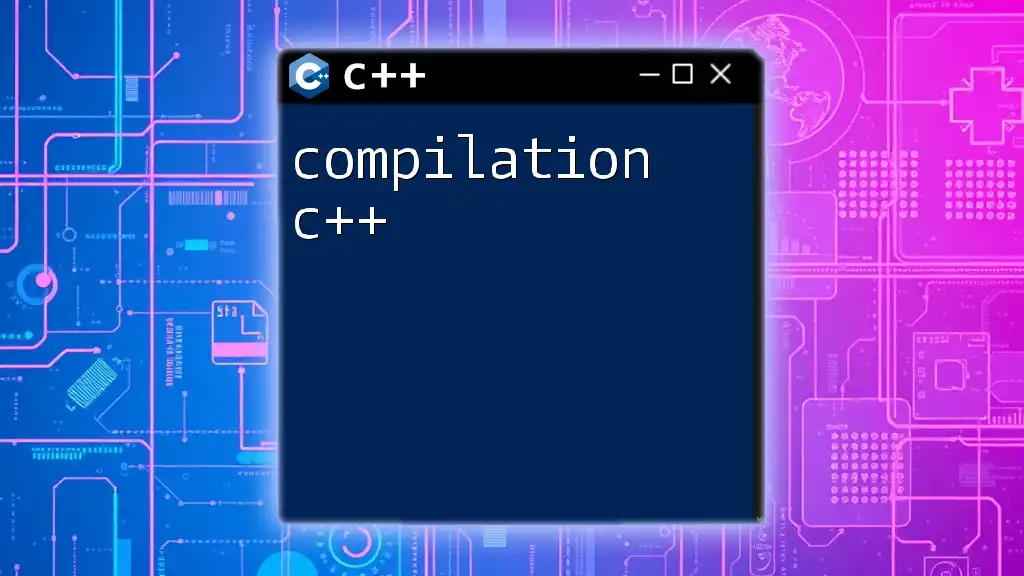
Common C++ Preprocessor Directives
Include Directives
The `#include` directive is perhaps the most commonly used directive in any C++ program. Its primary role is to include the contents of a specified file within the code. The syntax is as follows:
#include <filename>
This format is used for including standard library files. Conversely, using double quotes allows for the inclusion of user-defined files:
#include "filename"
The difference lies in the search paths used by the compiler; angle brackets refer to standard directories while double quotes check the current directory first.
Macro Directives
The `#define` directive is instrumental for defining constants or creating macros. A macro can be a simple constant or a function-like construct.
The syntax for defining a constant is straightforward:
#define PI 3.14
For a function-like macro, you might see:
#define SQUARE(x) ((x) * (x))
When using these macros, the preprocessor replaces every occurrence of the macro in the code with its definition, enabling a cleaner and more maintainable codebase.
Conditional Compilation
Conditional compilation directives allow you to compile certain parts of code based on specific conditions. They employ commands like `#ifdef`, `#ifndef`, `#if`, `#else`, `#elif`, and `#endif`.
Use cases for conditional compilation might include enabling or disabling debugging information. An example is shown below:
#ifdef DEBUG
std::cout << "Debugging mode" << std::endl;
#endif
In the above code, the debugging message will only appear if the `DEBUG` macro is defined.
Undefining Macros
Sometimes, you may need to remove a macro definition. The `#undef` directive does just that, allowing you to undefine a previously defined macro. This is particularly useful when managing scope or ensuring macros do not interfere with one another.
Here's an example:
#define MAX 100
#undef MAX
This code snippet first defines a constant `MAX`, and then undefines it, making it unusable afterwards in that context.
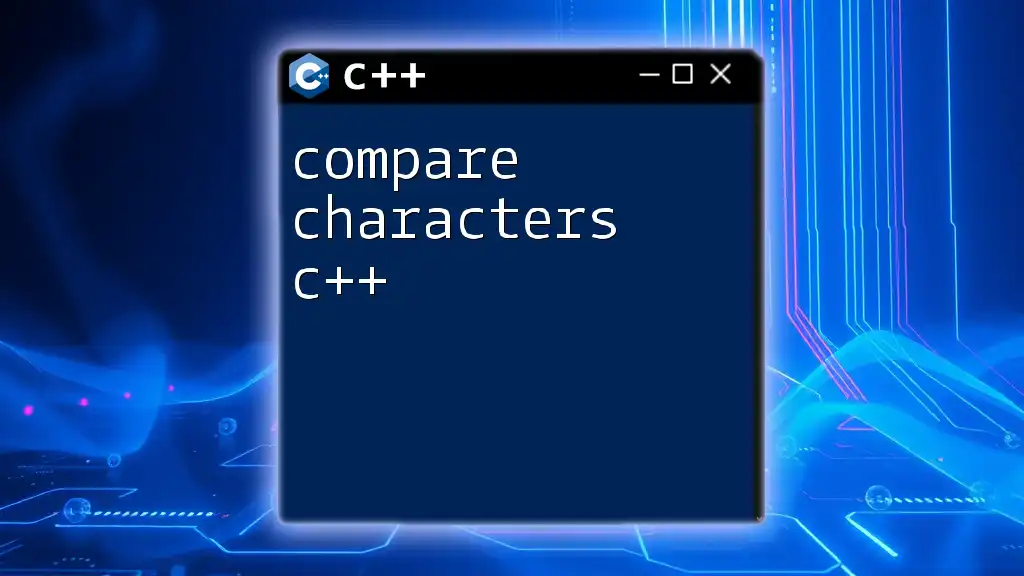
Advanced C++ Directives
File Inclusion Guards
File inclusion guards are a critical aspect of modern C++ programming. They prevent issues that arise from multiple inclusions of the same header file, which can lead to redefinition errors.
A typical structure using file inclusion guards would look like this:
#ifndef MY_HEADER_H
#define MY_HEADER_H
// Your header file content, such as function declarations or class definitions.
#endif
This pattern ensures that the header file's content is only included once, regardless of how many times it's referenced.
Diagnostic Directives
The `#pragma` directive provides a mechanism for issuing special instructions to the compiler. Unlike other directives, `#pragma` is compiler-specific; its behavior might vary based on the compiler being used. A common example is the following:
#pragma once
This directive tells the compiler to include the file only once during compilation. It simplifies the use of inclusion guards while achieving the same goal.
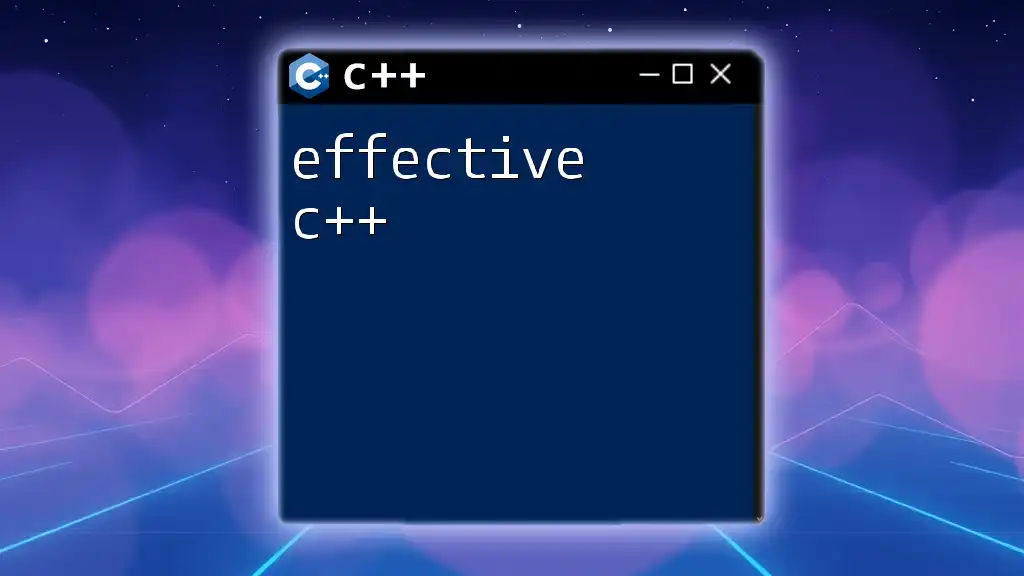
Best Practices for Using C++ Compiler Directives
To make the most of compiler directives C++, adhering to best practices is crucial:
-
Organize Directives for Readability: Position your directives at the top of your source files for better clarity.
-
Consistent Naming Conventions for Macros: Establish and stick to a naming convention, usually uppercase letters for macros, to differentiate them from variables.
-
Avoiding Overuse of Macros: While macros can enhance flexibility, overusing them may lead to code that is hard to debug and maintain. Prefer inline functions whenever possible.
-
Effectively Using Conditional Compilation: Use conditional compilation to manage platform-specific or feature-specific code, but keep this organized to avoid confusion.
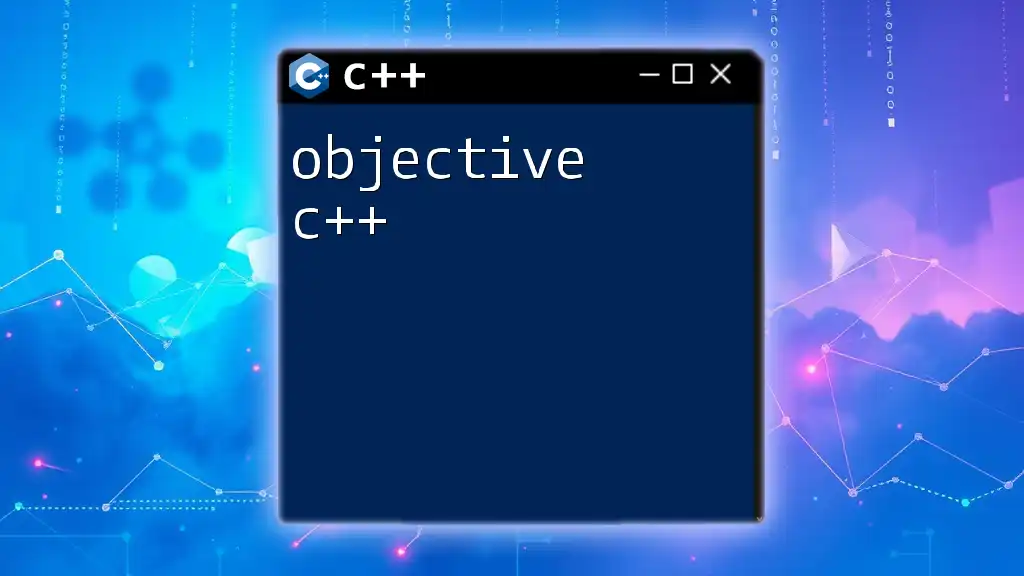
Conclusion
Compiler directives C++ are powerful tools that enable developers to write flexible, efficient, and maintainable code. From the basic `#include` to advanced `#pragma`, understanding and implementing these directives is essential for any serious C++ programmer.
By mastering compiler directives, you not only enhance your programming skills but also improve the overall quality of your code. Start applying these concepts in your projects today and watch your coding efficiency soar!
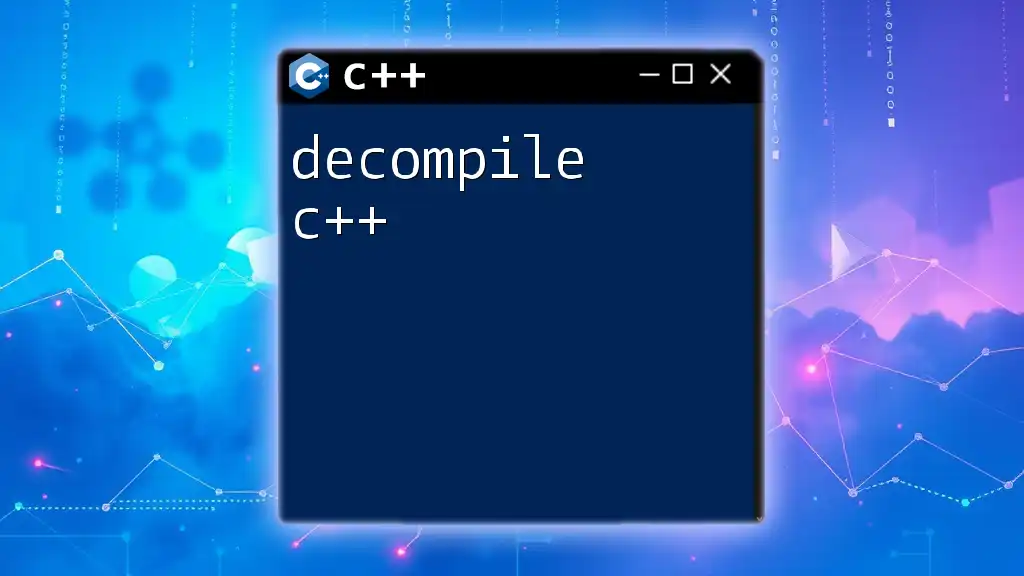
Additional Resources
- Recommended Books on C++: Look for titles that delve into advanced C++ programming techniques.
- Online Tutorials for Further Learning: Various platforms provide valuable resources and hands-on exercises.
- Community Forums for Discussion: Engage with other developers to share knowledge and experiences related to C++ and its directives.