To compile and run C++ code in Visual Studio Code, you need to set up the C++ extension and use the integrated terminal with the g++ command.
Here’s a sample command to compile and run your `main.cpp` file:
g++ main.cpp -o main && ./main
Setting Up Your Visual Studio Code Environment
Download and Install Visual Studio Code
To begin with how to compile and run C++ in Visual Studio Code, you first need to have the software installed. Visit the [Visual Studio Code website](https://code.visualstudio.com/) and download the latest version suitable for your operating system (Windows, macOS, or Linux). Follow the installation prompts and ensure that the installation completes successfully.
Install C++ Compiler
Next, you'll need a C++ compiler to convert your C++ code into executable programs. The most common compilers include GCC (GNU Compiler Collection) and Clang.
Installing GCC on Windows
For Windows, installing MinGW, the Minimalist GNU for Windows, is a common approach. After downloading MinGW from its [official page](https://osdn.net/projects/mingw/releases/), follow these steps:
- Run the installer and check the box for the C++ compiler.
- Complete the installation.
- Add MinGW to your system PATH:
- Right-click on "This PC" > "Properties" > "Advanced system settings" > "Environment Variables".
- Under "System variables", find the Path variable, select it, and click "Edit".
- Click "New" and add the path to the `bin` directory of your MinGW installation (e.g., `C:\MinGW\bin`).
Installing GCC on macOS
If you're using macOS, the easiest way to install GCC is through Homebrew. If you don't have Homebrew installed, you can do so with the following command in your terminal:
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
Once Homebrew is installed, run:
brew install gcc
Installing GCC on Linux
Installing GCC on Linux is typically straightforward. You can use the package manager available on your distribution. For example, on Ubuntu, you can install it by running:
sudo apt update
sudo apt install build-essential
Install C/C++ Extension in Visual Studio Code
To streamline your C++ programming experience, installing the C/C++ extension by Microsoft is essential.
- Open Visual Studio Code.
- Navigate to the Extensions Marketplace by clicking on the square icon in the left sidebar or pressing `Ctrl + Shift + X`.
- Search for "C/C++" and click Install.
- Additionally, consider installing extensions such as CodeLLDB for debugging and CMake Tools if you plan to use CMake for your build process.
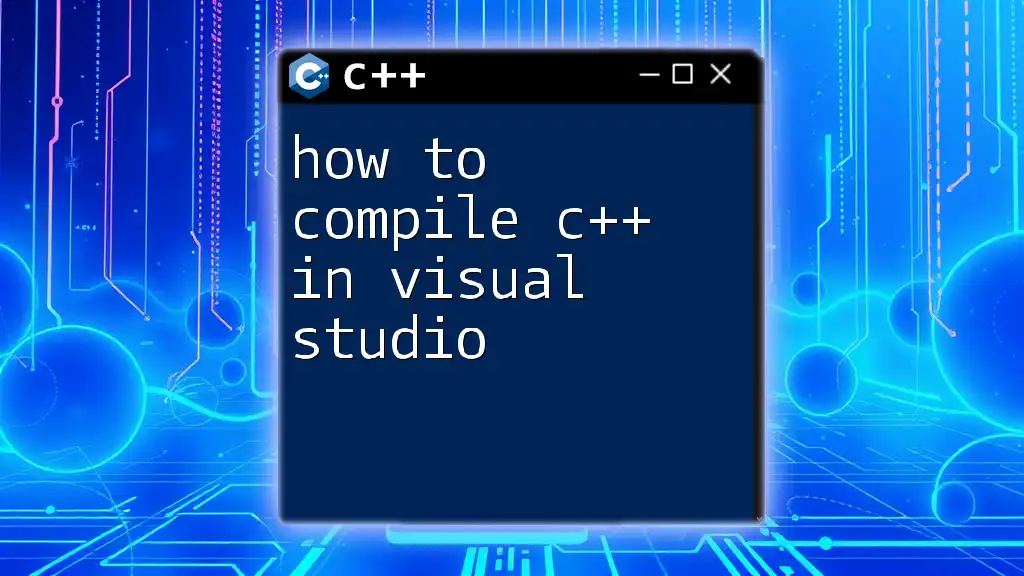
Creating Your First C++ Project
Set Up a New Project Folder
To keep your projects organized, create a new directory for your C++ files. Use your terminal or file explorer to create a folder named `MyCPPProject` or any name that suits your project.
Create a New C++ File
Inside your project folder, create a new file and name it `main.cpp`. This file will contain your C++ code.
Writing Your First C++ Program
In your `main.cpp` file, write the following simple C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This basic program outputs "Hello, World!" to the console. Understanding the structure of this program is fundamental for all C++ programs. The `#include <iostream>` line imports the input-output stream library, allowing you to use `std::cout` for printing to the console.
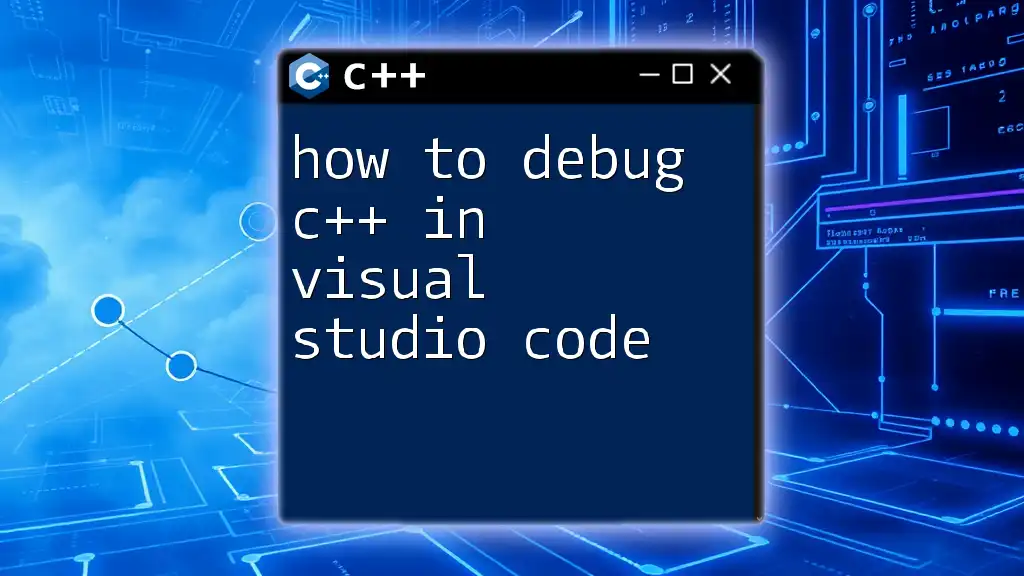
Compiling Your C++ Code
Using the Integrated Terminal
To compile your C++ program, you will utilize the integrated terminal in Visual Studio Code. To open the terminal, go to the top menu and select `Terminal > New Terminal`, or use the shortcut `Ctrl + ` (backtick).
Compiling the Code
With the terminal open, navigate to your project directory using the `cd` command. Then, compile your C++ code by running:
g++ main.cpp -o main
Here, `g++` is the command for the GNU C++ compiler, `main.cpp` is the source file, and `-o main` specifies the name for the output file (the compiled program).
Understanding Compiler Commands: The `-o` flag allows you to name the output executable, making it easier to identify and run the program later.
Understanding Compilation Errors
If there are any errors in your code, the terminal will output messages to help you debug the issues. Common errors include missing semicolons, undeclared variables, or syntax mistakes. For instance, forgetting a semicolon will generate an error similar to:
error: expected ‘;’ before ‘return’
Focus on reading these error messages carefully; they guide you on what to fix.
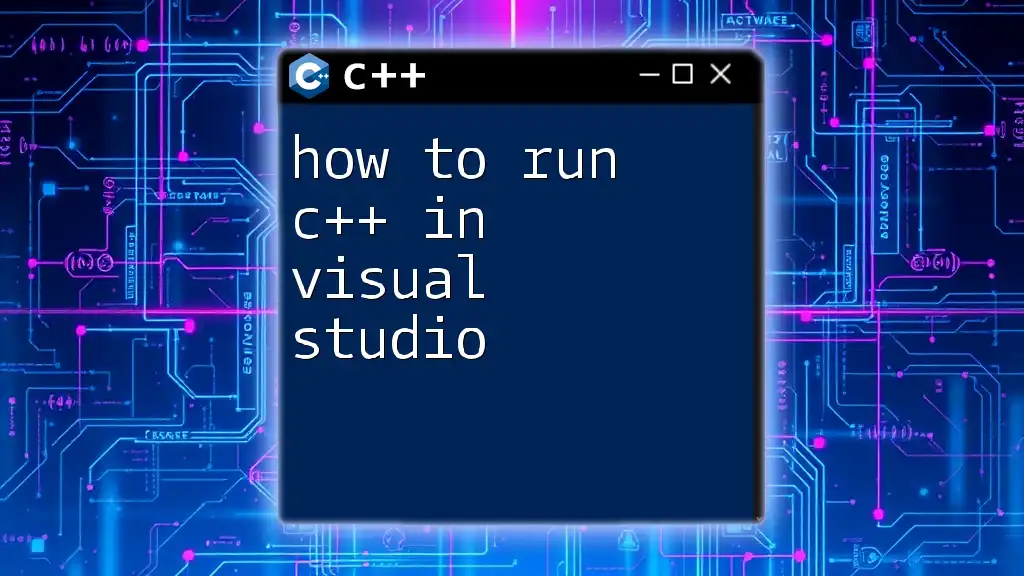
Running Your C++ Program
Executing the Compiled Code
Now that your code is successfully compiled, it's time to run the executable. In the terminal, type the command to execute your program. This differs slightly depending on your operating system:
- On Unix-based systems (Linux, macOS):
./main
- On Windows:
main.exe
Executing this will run your program, and you should see "Hello, World!" printed in the terminal.
Using VS Code Debugger
Visual Studio Code has built-in debugging capabilities, allowing you to set breakpoints and inspect variables as your program runs. To get started with debugging:
- Open the Debug pane by clicking on the play icon in the left sidebar.
- Click on create a launch.json file.
- Follow the prompts to choose the C++ (GDB/LLDB) option, which will automatically create a `launch.json` file with default settings.
Example Debugging Configuration
For more advanced debugging, your `launch.json` configuration might look like this:
{
"version": "0.2.0",
"configurations": [
{
"name": "C++ Debug",
"type": "cppdbg",
"request": "launch",
"program": "${workspaceFolder}/main",
"args": [],
"stopAtEntry": false,
"cwd": "${workspaceFolder}",
"environment": [],
"externalConsole": false,
"MIMode": "gdb",
"setupCommands": [
{
"description": "Enable pretty-printing for gdb",
"text": "-enable-pretty-printing",
"ignoreFailures": true
}
],
"preLaunchTask": "build"
}
]
}
This configuration sets up your debugging session to run `main`, while `MIMode` specifies the debugging protocol. Becoming familiar with debugging tools is crucial, as they enhance your ability to resolve issues efficiently within your code.
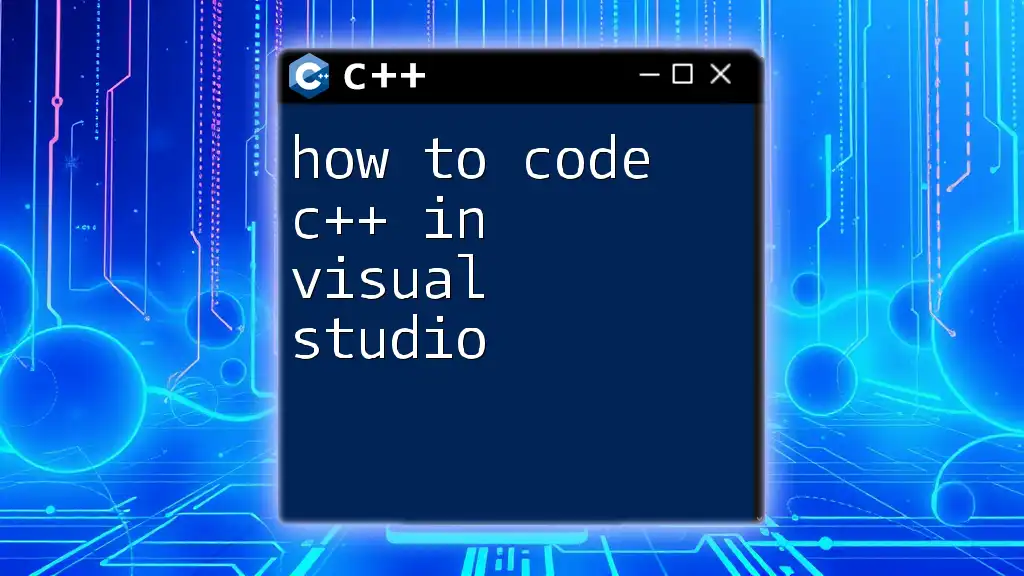
Best Practices and Tips for Using C++ in Visual Studio Code
Use Code Formatting Tools
For cleaner and more maintainable code, consider using formatting tools and linters that help enforce coding standards. The C/C++ extension in VS Code often includes options to format your code on save.
Version Control with Git
Incorporating version control into your workflow is invaluable. Use Git for managing changes in your code. It allows you to track modifications, revert to earlier versions, and collaborate with others. Setting up Git in VS Code involves:
- Opening the terminal.
- Cloning a repository or initializing a new one with:
git init
- Committing changes regularly using:
git add .
git commit -m "Initial commit"
Resources for Further Learning
While mastering C++ takes time, numerous online resources can help you along the way. Websites like Codecademy, Udacity, and Coursera offer comprehensive C++ courses. Community forums such as Stack Overflow and Reddit can be invaluable for troubleshooting and gaining insights from experienced developers.
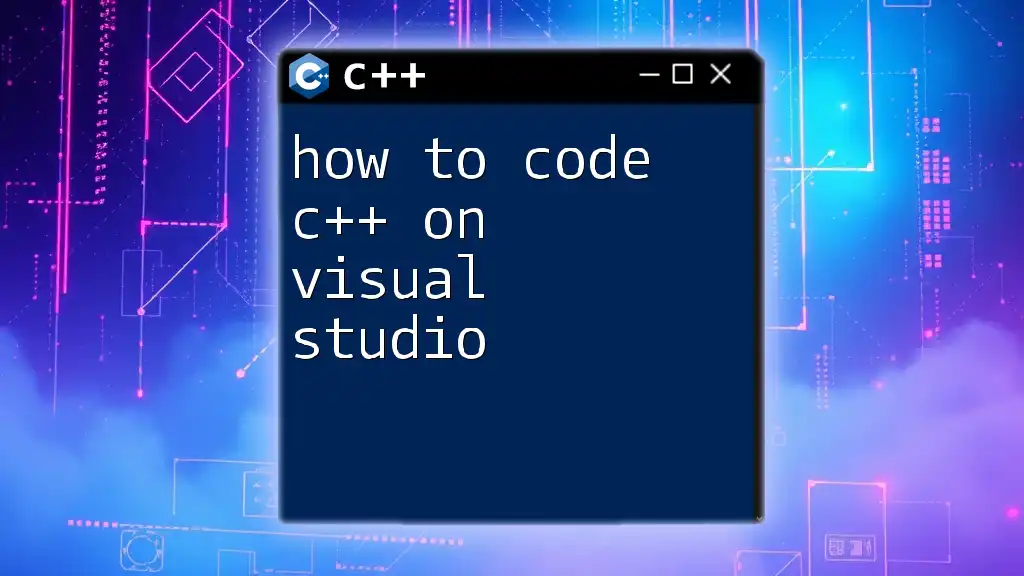
Conclusion
In this article, we've explored how to compile and run C++ in Visual Studio Code. By setting up a proper environment, creating C++ programs, compiling them, and utilizing debugging tools, you are now equipped to tackle your C++ development projects confidently. The ability to efficiently compile and run code in an integrated development environment like VS Code will significantly enhance your programming experience.
With these foundational skills, you're encouraged to dive deeper into the world of C++ and unlock new programming capabilities!