Xcode is a powerful Integrated Development Environment (IDE) for macOS that facilitates C++ programming by providing tools for code completion, debugging, and project management.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Setting Up Xcode for C++
Downloading and Installing Xcode
To get started with Xcode and C++, you must first download and install Xcode on your Mac. The simplest way to do this is through the Mac App Store. Here's a quick guide to ensure you get Xcode up and running smoothly:
- Access the Mac App Store: Open the Mac App Store from your Applications folder or Dock.
- Search for Xcode: Use the search bar to find "Xcode" and click on it.
- Download and Install: Click the "Get" button to download and then "Install." Once the installation is complete, Xcode will be ready for use.
During the installation process, you may encounter prompts requesting data usage and permissions. Ensure you accept these to enable all features of Xcode.
Configuring Xcode for C++
Creating Your First C++ Project
Once Xcode is installed, you can start your first C++ project easily:
- Open Xcode: Launch the application.
- Select Create a New Project: From the welcome dialog, choose the option to create a new project.
- Choose the Right Template: Navigate to macOS and select the "Command Line Tool" template, which is ideal for C++ projects. Here, you can set your project's name and ensure C++ is selected as the language.
- Location and File Setup: Choose a location for your new project and click "Create."
Upon creating your project, you will be presented with a default main file. You can modify it to run your first C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding Xcode Interface for C++
Navigating the Xcode interface is crucial for maximizing productivity when working with Xcode and C++. Familiarize yourself with the key sections:
- Navigator: Located on the left, it allows you to view all files, the project structure, and the version control.
- Editor: The central area where you write your C++ code. It includes syntax highlighting, and indentation helps maintain code consistency.
- Debug Area: At the bottom of Xcode, this area lets you see output and debug your application step-by-step.
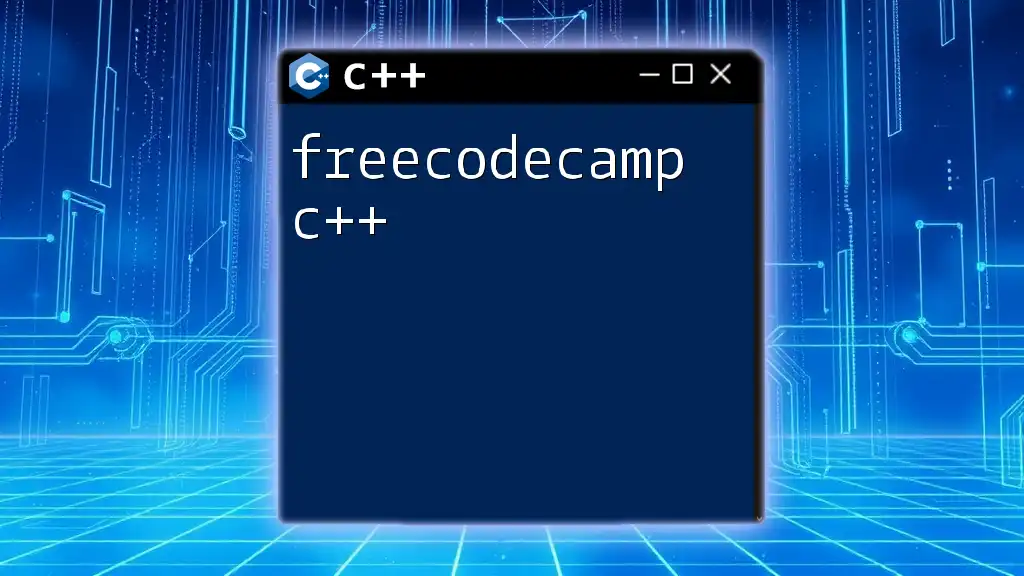
Writing C++ Code in Xcode
Project Structure in Xcode
Understanding the project structure within Xcode is essential as it determines how your files interact with each other. Your primary components are:
- Source Files (.cpp): This is where the implementation of your functions and classes resides.
- Header Files (.h): Used for defining your classes, constants, and function declarations.
This separation of files helps maintain organization and clarity in your projects.
Key Features for C++ Development
Xcode comes with several features to enhance your C++ coding experience:
Code Completion and Suggestions
One of the most helpful aspects of Xcode is its code completion feature. As you type, Xcode provides suggestions for variables, functions, and methods. This not only speeds up your coding process but also reduces the likelihood of syntax errors.
Error Highlighting and Diagnostics
When you make an error in your code, Xcode immediately highlights the problematic line and provides a description of the error. This feature efficiency allows you to focus on solving issues without wasting time looking for bugs.
Working with Libraries and Frameworks
Integrating External Libraries
Often, you may want to incorporate additional functionality into your projects by using external libraries. To do this in Xcode:
- Navigate to Project Settings: Click on your project in the Navigator, select the "General" tab, and find "Frameworks, Libraries, and Embedded Content."
- Add Libraries: Use the "+" button to add desired libraries (e.g., Boost, OpenCV) either installed through package managers or downloaded manually.
Using Built-in C++ Libraries
C++ has a wealth of built-in libraries that streamline development processes. The Standard Template Library (STL) is especially noteworthy as it offers data structures and algorithms like vectors, lists, and maps, which can significantly ease your coding efforts.
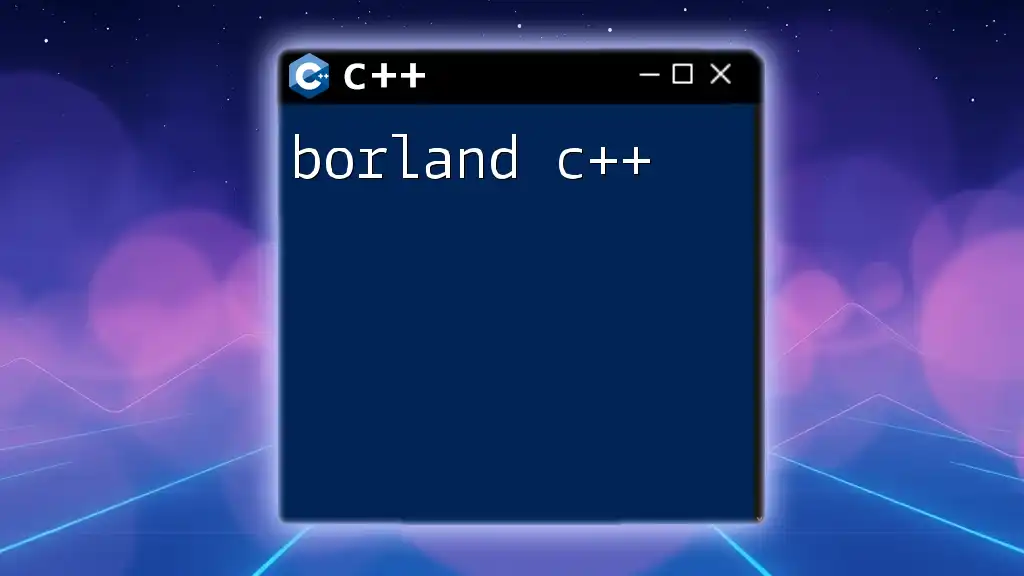
Debugging C++ Code in Xcode
Setting Breakpoints
Debugging is a fundamental part of software development. With Xcode, setting breakpoints is intuitive:
-
Set Breakpoints: Click in the gutter next to the line numbers in your code to create a breakpoint. This tells Xcode to pause execution at that line, allowing you to inspect the values at that moment.
-
Remove Breakpoints: Simply click again to toggle them off.
Using the Debugger
The debugger within Xcode is powerful. You can step through your code line by line, observing how variables change and watching for logical errors.
- Take advantage of watch variables to monitor specific data points.
- Use expression evaluation to run quick checks on your variables in real time.
Analyzing Performance
To ensure efficiency, utilizing the Instruments tool for profiling your C++ application can highlight performance bottlenecks. You can track memory usage, CPU load, and understand how resources are being allocated throughout your program’s lifecycle.
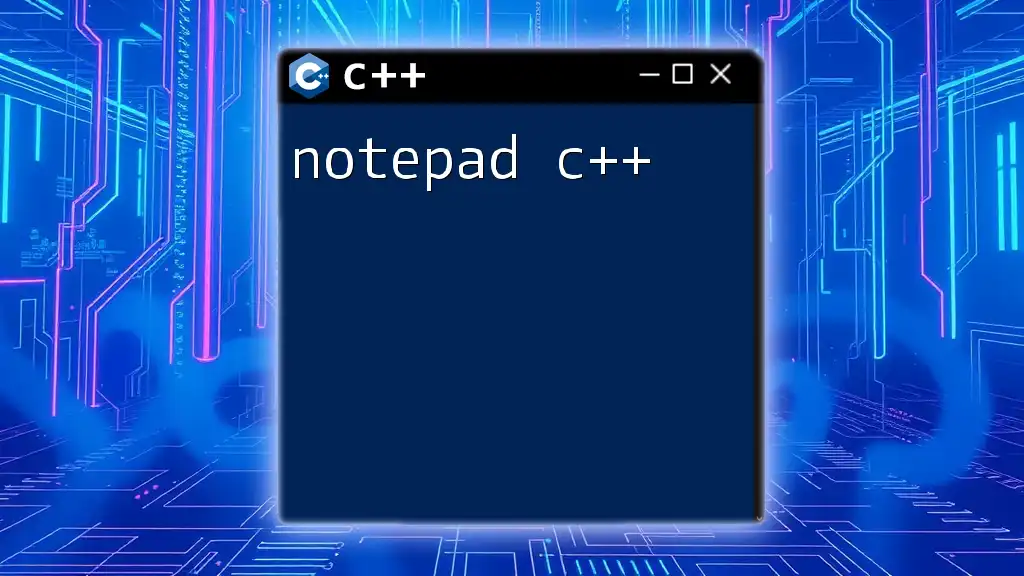
Building and Running C++ Applications
Compiling Your Code
When you click on the "Run" button or use the appropriate keyboard shortcut, Xcode compiles your code. Understanding this process is vital because:
- Compilation transforms your source code into machine-readable format.
- Linking connects various code sections and libraries, creating a single executable application.
Common Build Configurations
Xcode allows you to choose between different build configurations. The most common are Debug and Release modes:
- Debug mode includes additional debugging information, making it easier to test your application.
- Release mode optimizes the executable, stripping away any debug-related metadata to generate lean, efficient code for production.
Running and Testing Your Application
To run your project, simply click a button or select the corresponding menu option. Xcode will compile, link, and execute your application seamlessly.
Moreover, unit testing is crucial, especially using frameworks like Google Test. This will help ensure your functions perform as expected.
#include <gtest/gtest.h>
TEST(SampleTest, AssertionTrue) {
ASSERT_TRUE(true);
}
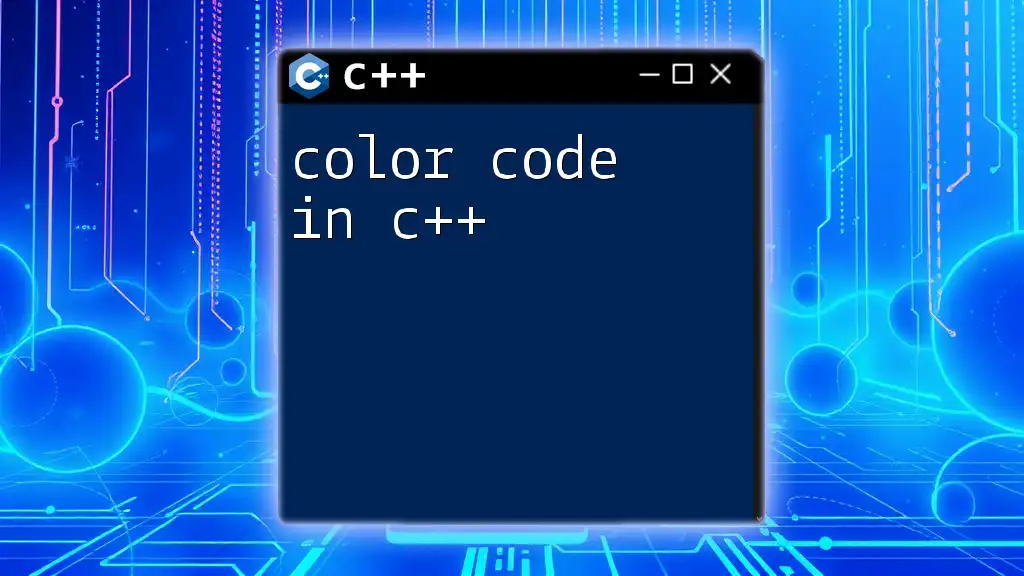
Advanced C++ Techniques in Xcode
Using Templates and STL
Templates and the STL are pillars of C++. Templates allow you to create functions and classes that can operate with any data type, enhancing code reusability.
Common STL Containers
Utilizing STL also provides you the flexibility of various containers:
- Vector: A dynamic array that can resize itself automatically.
- List: A collection that allows fast insertions and deletions.
- Map: A key-value store that works efficiently for lookups.
Object-Oriented Programming in C++
Understanding Object-Oriented Programming (OOP) principles in C++—such as encapsulation, inheritance, and polymorphism—is vital. Here’s a simple example demonstrating classes and inheritance:
class Animal {
public:
virtual void speak() = 0; // pure virtual function
};
class Dog : public Animal {
public:
void speak() override { std::cout << "Woof!" << std::endl; }
};
This code allows you to define different animals with their behaviors while enabling polymorphism—a core tenet of OOP.
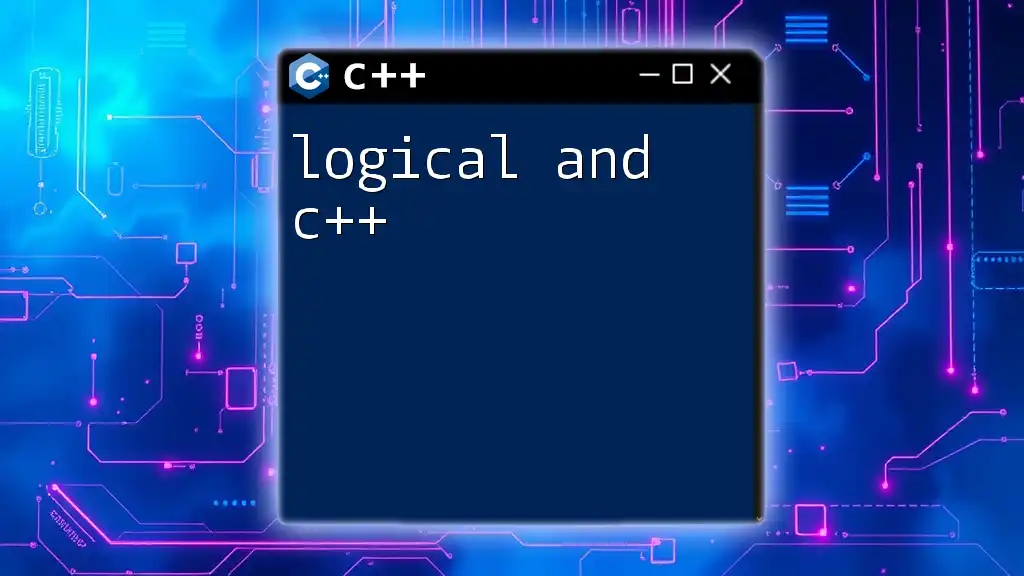
Conclusion
Mastering Xcode and C++ can significantly enhance your programming capabilities. By understanding how to set up your development environment, write efficient code, debug effectively, and utilize advanced features, you are well on your way to becoming a proficient C++ developer.
The journey doesn’t stop here! Maintain a continual learning path and experiment with your own projects to solidify your understanding.
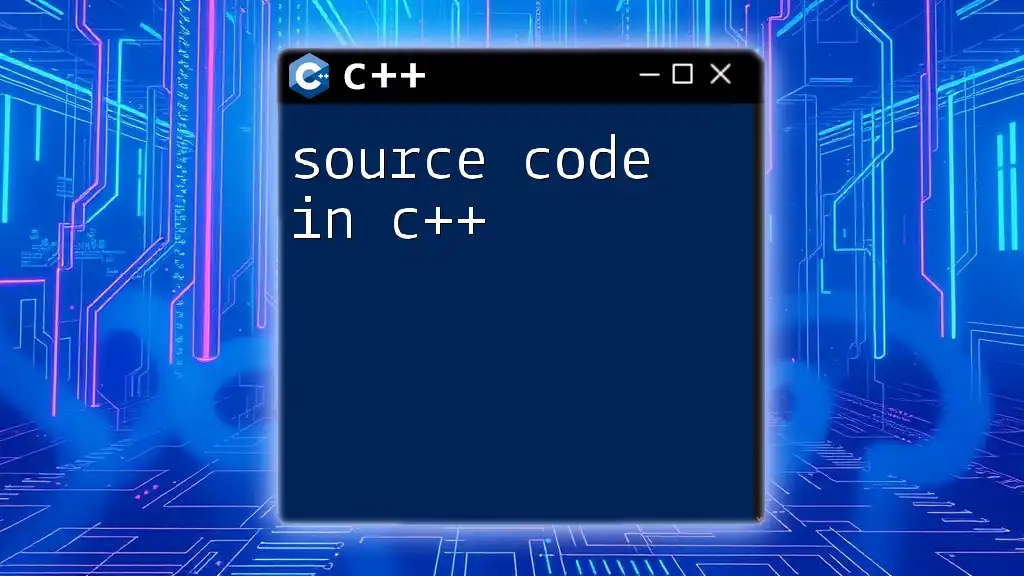
Call to Action
Join our community to explore more tips and tricks on mastering C++ in Xcode. Share your experiences or any challenges you face; we’re here to help you become a better C++ programmer!