Unity primarily uses C# as its scripting language, but it allows the integration of C++ through plugins for performance-critical tasks. Here's an example of C# code in Unity:
using UnityEngine;
public class HelloWorld : MonoBehaviour
{
void Start()
{
Debug.Log("Hello, World!");
}
}
If you're integrating C++, it might look like this:
#include <iostream>
extern "C" void HelloFromCPP()
{
std::cout << "Hello from C++!" << std::endl;
}
Unity Uses C# or C++
Overview of Programming Languages in Unity
Unity, as a powerful game development engine, implements multiple programming languages, with C# and C++ being the most prominent. While C# is the primary language used for scripting gameplay and other game elements, C++ forms the backbone of the Unity engine itself. Understanding the roles that C# and C++ play helps developers leverage Unity more effectively.
Does Unity Use C#?
Understanding C# in Unity
C# (pronounced C-sharp) is a versatile, high-level programming language developed by Microsoft as part of its .NET initiative. Widely known for its ease of use, it's particularly prevalent in game development due to its robust features and integrations within the Unity framework.
Unity’s choice of C# as its primary scripting language stems from several factors:
- Strongly Typed Language: C# is statically typed, meaning errors can be caught at compile time, reducing runtime issues.
- Object-Oriented: The object-oriented structure of C# enables developers to create modular and reusable code, which promotes better organization.
Why C# is the Primary Language for Unity
Utilizing C# in Unity offers numerous advantages for game developers:
-
Ease of Use and Accessibility: C# offers a syntax that is approachable for beginners while still powerful for seasoned developers. Its clear syntax and structure reduce the learning curve, making it ideal for those new to programming.
-
Rich Libraries and Frameworks: C# comes with extensive libraries that streamline various aspects of game development, from graphics rendering to complex mathematical calculations, allowing developers to focus on creativity rather than reinventing the wheel.
-
Supportive Community: C# has a vibrant community of developers who contribute to forums, tutorials, and documentation, making it easier for newcomers to find help and resources.
Example Code Snippet
using UnityEngine;
public class PlayerMovement : MonoBehaviour
{
public float speed = 5.0f;
void Update()
{
float moveHorizontal = Input.GetAxis("Horizontal");
float moveVertical = Input.GetAxis("Vertical");
Vector3 movement = new Vector3(moveHorizontal, 0.0f, moveVertical);
transform.Translate(movement * speed * Time.deltaTime);
}
}
This code snippet demonstrates basic player movement in a Unity game. The `Update()` method is called once per frame; it captures player input and translates the player object accordingly. This exemplifies how C# simplifies real-time gameplay mechanics and responsiveness.
Does Unity Use C++?
Understanding C++ in Unity
C++ is a general-purpose programming language that offers high-performance capabilities. In Unity, C++ is primarily utilized for developing engine functionalities. This means that while end-users predominantly write scripts in C#, the engine's underlying architecture is built with C++ to maximize efficiency and performance.
Use Cases for C++
C++ plays a crucial role in specific scenarios in Unity, especially where performance is paramount. Here are some contexts in which C++ is indispensable:
-
Performance-Critical Operations: Unity uses C++ to handle complex calculations and rendering tasks that require efficiency. This is particularly evident in the physics engine and graphics rendering layers, where C++ provides lower-level access to hardware optimization.
-
Custom Plugins and Native Code: If developers wish to create custom functionality that requires speed or interacts with platform-specific features, they may write native plugins in C++. This allows for functionality in Unity that goes beyond what is readily available through C#.
Example Code Snippet
// Example of a native plugin written in C++
extern "C" {
__declspec(dllexport) void MyFunction()
{
// Your C++ code here
}
}
This C++ code snippet creates a simple function within a native plugin. By declaring the function with `extern "C"`, Unity can interface with the C++ code seamlessly. Such plugins enhance Unity’s capabilities, especially for tasks that require direct system access or high-speed performance.
The Relationship Between C++ and C#
How C++ and C# Work Together in Unity
Understanding the roles of C# and C++ in Unity reveals how the two languages complement one another. While C# is used for scripting gameplay and interaction, C++ underpins the engine’s architecture and performance.
-
Scripting vs. Engine Development: C# focuses on game logic—controlling characters, managing game states, and handling user input. In contrast, C++ deals with lower-level tasks that are crucial for optimizing performance.
-
Interfacing with Native Code: Unity allows developers to call C++ code from C# scripts using plugins. This feature is particularly beneficial when performance or hardware-specific functions are needed that C# cannot deliver as efficiently.
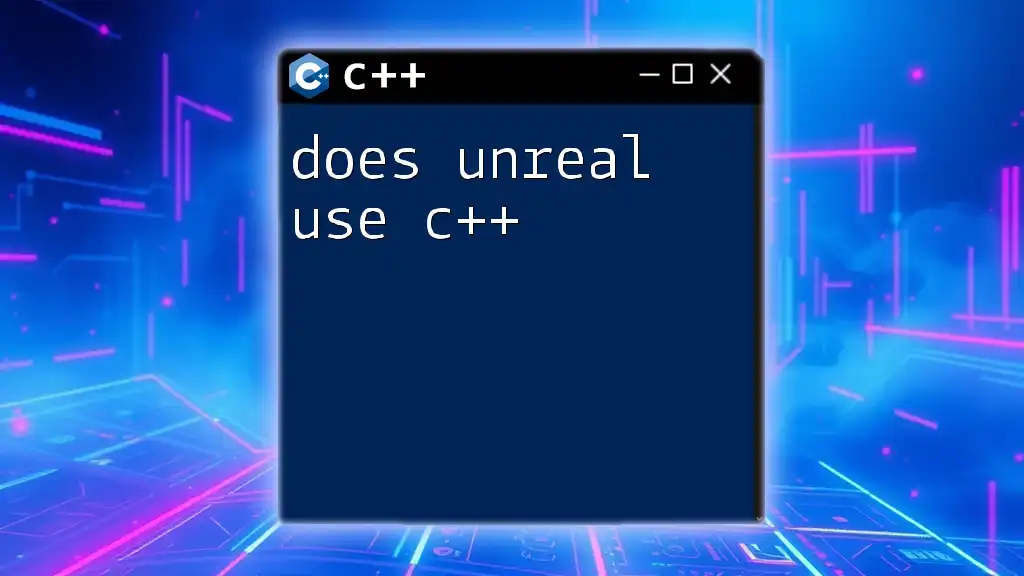
Conclusion
In conclusion, Unity primarily uses C# for its scripting needs due to its ease of use, rich library support, and community backing. C++, while not the primary language for game development within Unity, serves as the foundation on which the engine is built and performs critical functions where speed and performance are essential. Understanding the distinct yet interconnected roles of both languages can empower developers to create more sophisticated and optimized games.
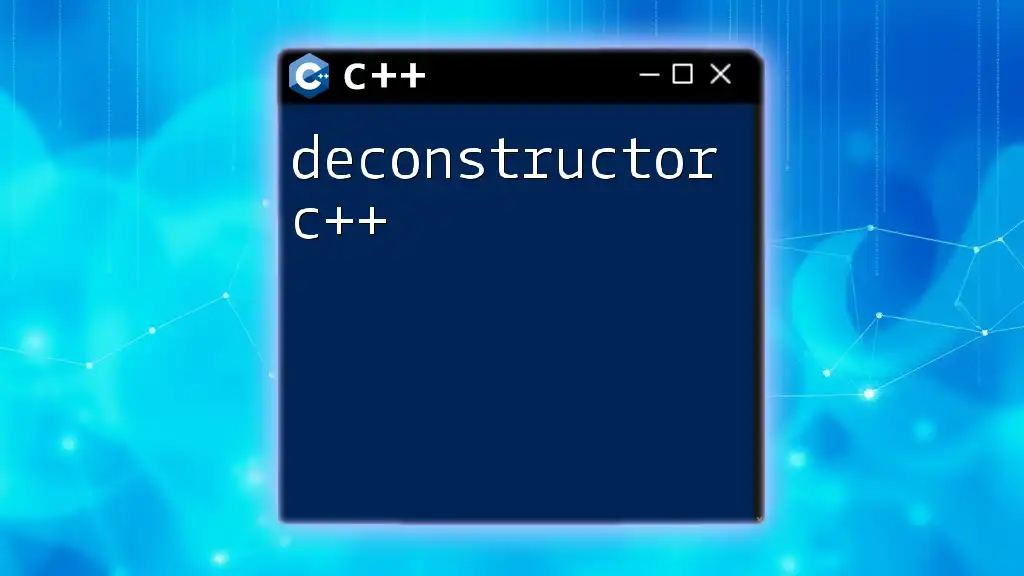
FAQs
Is C# easier to learn than C++ for Unity development?
Absolutely! C# is widely regarded as more user-friendly, especially for beginners, as it has a clearer syntax and structure, along with a focus on modern programming paradigms.
Can you write a complete game in C++ using Unity?
While Unity is built on C++, the scripting side primarily uses C#. However, developers can implement specific components or plugins in C++ to enhance performance or functionality.
What are some best practices when using both C# and C++ in Unity?
When employing both languages, it's vital to keep your code organized. Use C# for game logic and C++ for performance-critical operations, and be sure to document your code when interfacing between them to maintain clarity.
Feel free to enhance the structure with visuals, code samples, and additional context where needed. This comprehensive overview serves to pave the way for understanding Unity's usage of C# and C++ effectively.