Learning C++ offers a more advanced set of features and object-oriented paradigms compared to C, making it a versatile choice for modern software development.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
The Foundation: Understanding C and C++
What is C?
C is a powerful general-purpose programming language that has stood the test of time since its creation in the early 1970s. It is a procedural programming language, which means it follows a linear step-by-step approach to execute instructions. The simplicity and efficiency of C make it ideal for system software, embedded systems, and performance-critical applications.
Key Features of C:
- Low-Level Memory Access: Provides direct access to memory, allowing detailed hardware manipulation.
- Portability: Programs written in C can be executed on different machines with minimal modification.
- Rich Library Support: Offers a rich set of built-in functions for various tasks.
C is widely used in operating systems, embedded systems, and high-performance applications. It remains a cornerstone language for understanding low-level programming.
What is C++?
Developed as an extension of C in the early 1980s, C++ incorporates object-oriented programming features without sacrificing C's efficiency and flexibility. C++ allows developers to create complex applications while managing code through reusable objects.
Key Features of C++:
- Object-Oriented Programming: Supports encapsulation, inheritance, and polymorphism.
- Standard Template Library (STL): Offers a set of generic classes and functions to facilitate data structure and algorithm implementation.
- Performance: Retains C's performance characteristics while providing higher-level abstractions.
C++ finds its applications in game development, large-scale systems, and graphics rendering, making it a versatile language for various fields.
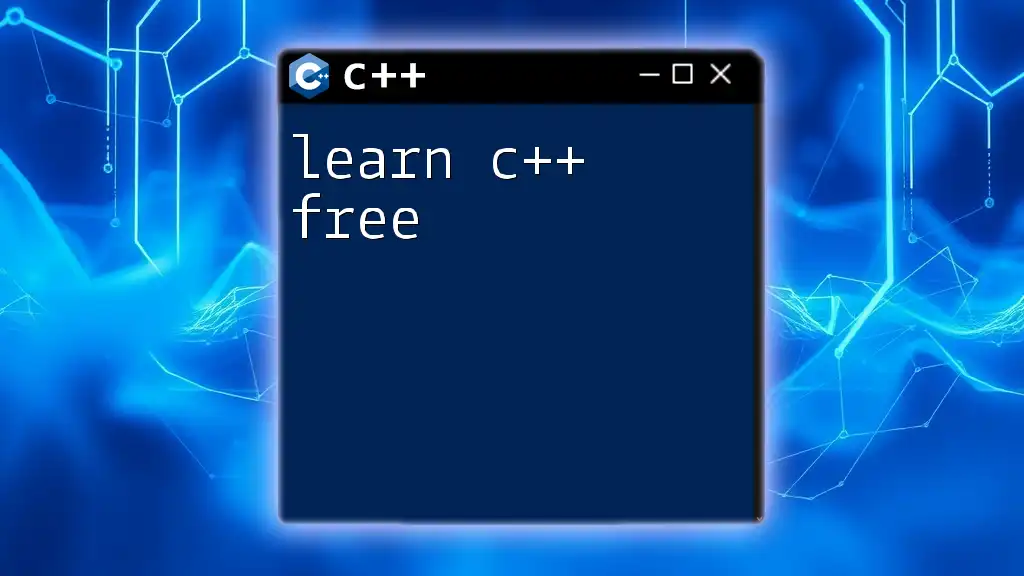
Key Differences Between C and C++
Language Paradigms
While C is a procedural programming language, C++ introduces object-oriented programming. This distinction allows C++ to model real-world entities as objects, making the code more modular and maintainable.
Code Example - Procedural vs. Object-Oriented:
Procedural approach in C:
#include <stdio.h>
void greet() {
printf("Hello, World!\n");
}
int main() {
greet();
return 0;
}
Object-oriented approach in C++:
#include <iostream>
class Greeter {
public:
void greet() {
std::cout << "Hello, World!" << std::endl;
}
};
int main() {
Greeter greeter;
greeter.greet();
return 0;
}
Syntax and Complexity
In terms of syntax, C is more straightforward due to its procedural nature. C++ introduces nuances such as class definitions, access specifiers, and function overloading, which add complexity but enhance functionality.
For instance, C requires separate function declarations, while C++ can provide default parameters:
// C function
void display(int n);
// C++ function with default parameter
void display(int n = 5);
Memory Management
Memory management is a critical aspect where C and C++ differ significantly. C relies on manual memory management using pointers:
int *arr = (int*)malloc(5 * sizeof(int));
free(arr);
In contrast, C++ provides advanced features like smart pointers to manage memory automatically, reducing the risk of leaks:
#include <memory>
std::unique_ptr<int[]> arr(new int[5]);
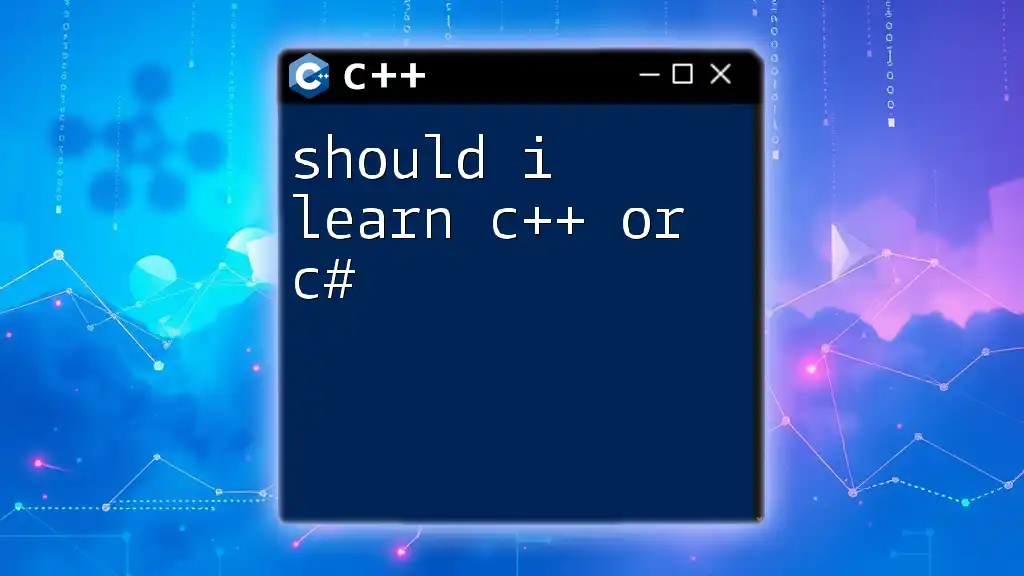
Should I Learn C or C++ First?
Learning Curve
The choice between learning C or C++ first largely depends on your goals. C is often recommended for beginners due to its simpler syntax and focus on fundamental programming concepts. However, if you're interested in developing applications using an object-oriented approach from the start, then C++ may be appropriate.
Career Opportunities
While both C and C++ offer strong job prospects, they cater to different niches:
- C Developers: Primarily sought in embedded systems, network devices, and operating systems.
- C++ Developers: Preferred in game development, applications where performance and efficiency matter, and systems programming.
Understanding your intended career path can guide your decision on which language to learn first.
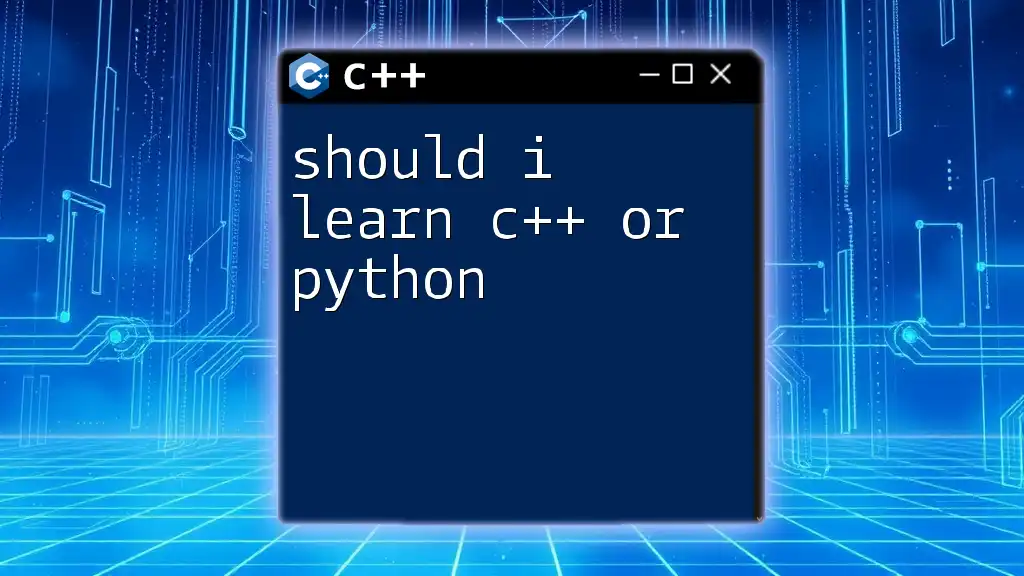
Real-World Applications of C and C++
C in Embedded Systems
C is the dominant language in embedded systems, largely due to its efficiency and direct hardware manipulation capabilities. Microcontrollers and real-time operating systems extensively use C to meet performance requirements.
Code Example - C in Embedded Systems:
#include <avr/io.h>
void setup() {
// Set pin 13 as output
DDRB |= (1 << DDB5);
}
void loop() {
// Blink LED on pin 13
PORTB |= (1 << PORTB5);
_delay_ms(1000);
PORTB &= ~(1 << PORTB5);
_delay_ms(1000);
}
C++ in Game Development
C++ holds a prominent place in the game development industry, with many game engines, such as Unreal Engine, built on its principles. The language's performance capabilities, along with its ability to manage complex graphics systems, make it an essential tool for developers in this field.
Code Example - C++ in Game Logic:
#include <SFML/Graphics.hpp>
class Player {
public:
void move(float deltaX, float deltaY) {
position.x += deltaX;
position.y += deltaY;
}
private:
sf::Vector2f position;
};
int main() {
sf::RenderWindow window(sf::VideoMode(800, 600), "Game");
Player player;
while (window.isOpen()) {
// Handle input and update game state
// ...
window.clear();
// Render player
window.display();
}
return 0;
}
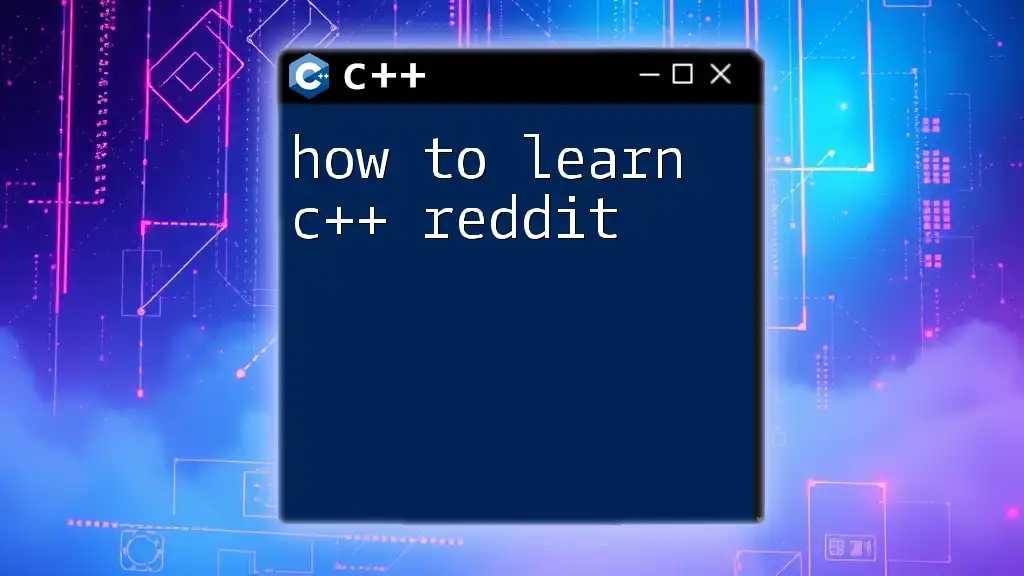
Resources for Learning C and C++
Online Courses
Several platforms offer comprehensive courses tailored to C and C++. Look for courses on sites like Coursera, Udemy, or edX, focusing on practical programming exercises to get hands-on experience.
Books
For a more in-depth understanding, several books stand out:
- "The C Programming Language" by Brian W. Kernighan and Dennis Ritchie: A classic for C learners.
- "C++ Primer" by Stanley B. Lippman: Essential for grasping C++ concepts. These texts cater to both beginners and advanced programmers.
Community and Support
Engaging with communities such as Stack Overflow, Reddit’s r/C_Programming, or specific forums for C and C++ can enhance your learning experience. Networking with peers will expose you to various coding styles and solutions.
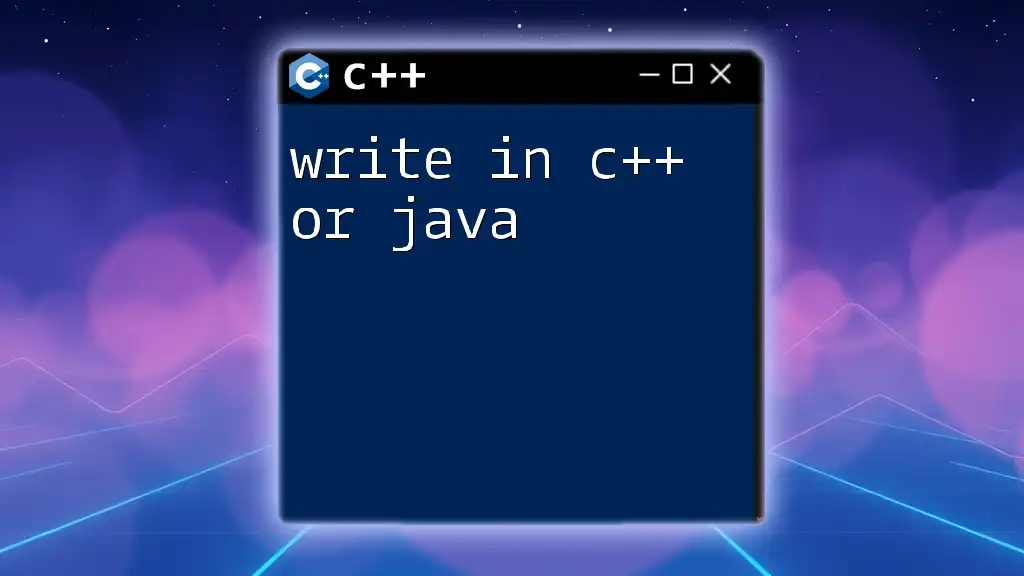
Conclusion: Making Your Decision
Choosing Based on Goals
Your decision about whether to learn C++ or C should align with your career aspirations and personal interests. Consider the types of projects that excite you and the industries that inspire you.
Final Recommendations
Regardless of your choice, embrace hands-on practice. Building projects, solving coding challenges, or contributing to open-source software will cement your learning and enhance your skills.
Call to Action
Begin your coding journey today! Explore resources, start a project, or join a community—your path to C or C++ mastery awaits!
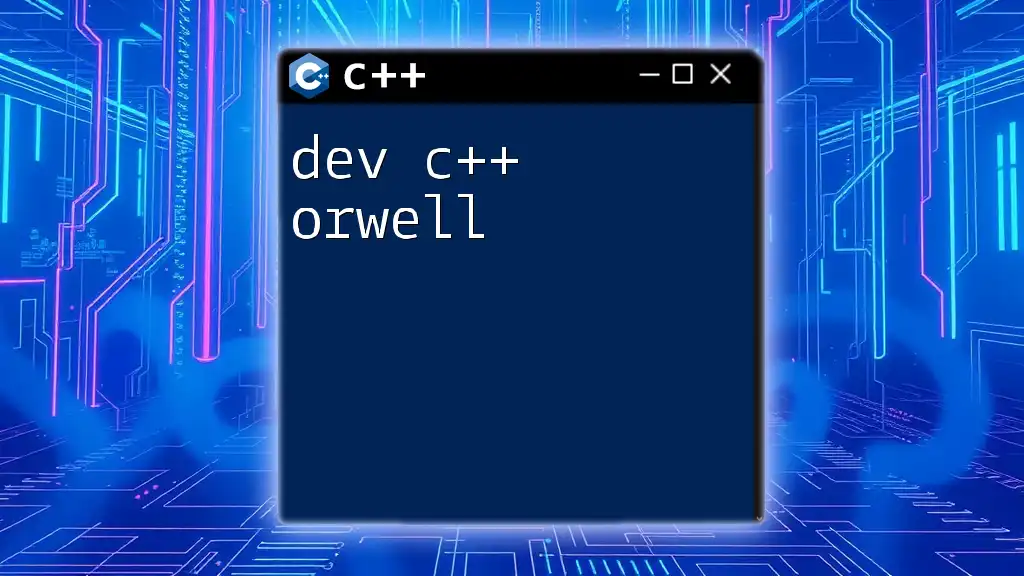
Additional Resources
Helpful Websites and Blogs
Look for websites dedicated to C and C++ programming, such as LearnCPP.com, and CodeProject. Following programming blogs can provide insights and updates on best practices.
Code Repositories
Visit GitHub to find a multitude of C and C++ projects where you can observe coding styles and practices. Contributing to these repositories will deepen your understanding and experience.
Continued Learning
Once you've grasped the basics, consider exploring advanced topics such as memory management techniques, multithreading, or design patterns. A commitment to continuous learning is essential in the ever-evolving coding landscape.