Learning C++ for game development equips you with the necessary skills to create high-performance games by utilizing the language's powerful features and object-oriented principles.
Here’s a simple example of a class in C++ that could be used for a game character:
class GameCharacter {
public:
GameCharacter(std::string name, int health) : name(name), health(health) {}
void takeDamage(int damage) {
health -= damage;
}
void displayStatus() {
std::cout << name << " has " << health << " health left." << std::endl;
}
private:
std::string name;
int health;
};
Understanding C++ in Game Development
C++ is one of the most widely used programming languages in the game development industry due to its unparalleled performance and efficiency. It provides a rich set of features, allowing developers to create complex game mechanics and systems while ensuring smooth gameplay. The advantages of learning C++ for game development include:
-
Performance and Efficiency: C++ allows developers to optimize their code for speed, letting them create high-performance games that run efficiently on various hardware.
-
Object-Oriented Programming (OOP): C++ supports OOP principles, enabling developers to create scalable and manageable code bases through encapsulation, inheritance, and polymorphism.
-
Rich Library Support: With extensive libraries and frameworks like Unreal Engine and SFML available, C++ developers have access to powerful tools that can significantly speed up game development processes.
Why Choose C++ Over Other Languages?
When considering learning C++ for game development, it's essential to understand why C++ is often preferred over languages like Python or C#. Some notable comparisons include:
-
Speed: C++ is generally faster than interpreted languages like Python, making it an ideal choice for resource-intensive applications such as games.
-
Control over System Resources: C++ gives developers direct access to the hardware and system resources, which is crucial for optimizing performance in games.
-
Industry Standard: Many commercial game engines, such as Unreal Engine, are built using C++, making it a common language in the game development industry.
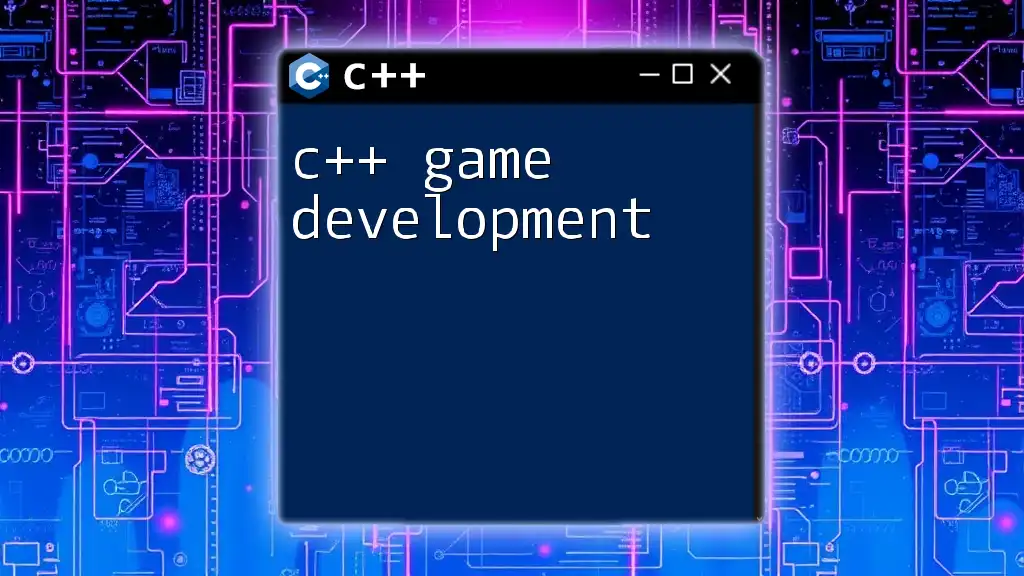
Getting Started with C++
Setting Up Your Development Environment
Before diving into coding, you need to set up your development environment. A few essential tools and software are necessary for successful C++ video game development:
-
Integrated Development Environments (IDEs): Popular choices include Visual Studio (especially for Windows), CLion, or Code::Blocks. Each IDE comes with tools that streamline coding, debugging, and project management.
-
Compilers: While an IDE will greatly assist in coding, you will also require a compiler such as GCC or MSVC to convert your source code into an executable program.
To set up your IDE, follow the provided installation guidelines, ensuring you have all necessary libraries installed (like graphics and sound libraries) that may be required for game development.
Basic Syntax and Concepts
Once your environment is ready, familiarize yourself with basic C++ syntax. Understanding variables, data types, loops, and conditionals is crucial for building game mechanics. Here's a simple example:
#include <iostream>
int main() {
int score = 0; // Initialize score
std::cout << "Your score is: " << score << std::endl;
return 0;
}
In this code, we declare an integer variable named `score`, initialize it to zero, and then print the score to the console. Grasping these fundamentals is essential as they serve as the building blocks for more complex game logic.
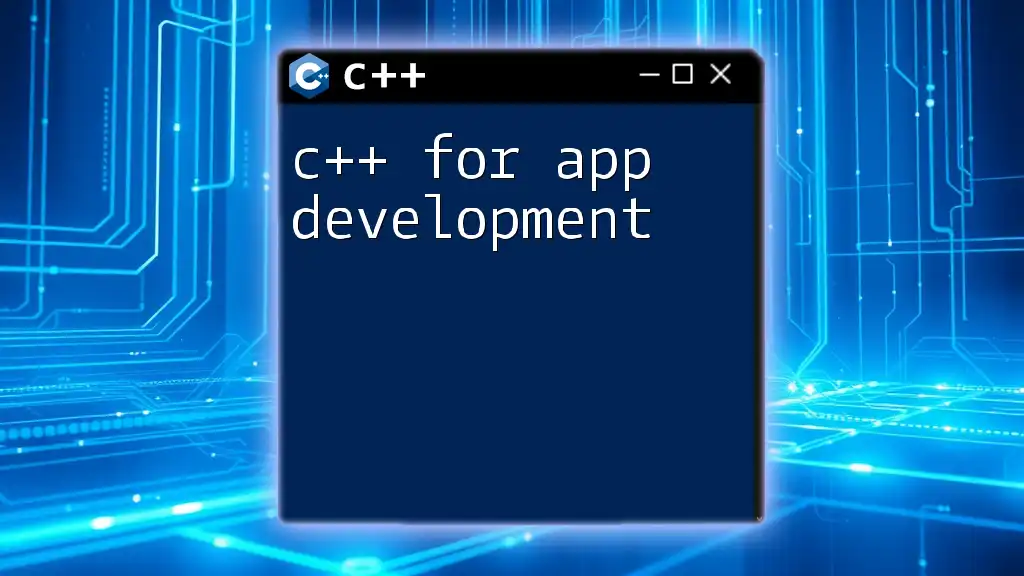
Exploring Advanced C++ Concepts for Game Development
Object-Oriented Programming (OOP)
Object-oriented programming is a paradigm that helps to organize code in a way that mimics real-world interactions. Understanding its principles is crucial for learning C++ for game development. Some core concepts include:
-
Encapsulation: Bundling data and methods that operate on the data into a single unit or class.
-
Inheritance: Creating new classes based on existing classes, promoting code reusability.
-
Polymorphism: The ability for different classes to be treated as instances of the same class through shared interfaces.
Here is an example of a simple class definition in C++:
class Player {
public:
std::string name;
int health;
void takeDamage(int amount) {
health -= amount;
}
};
In this example, we define a `Player` class with properties for `name` and `health`, along with a method to reduce health when the player takes damage. Understanding such OOP concepts aids in designing more complex game elements.
Memory Management
Memory management is a crucial aspect of C++ that can significantly impact game performance. Understanding pointers and dynamic memory allocation will lead to better game resource management.
Here's how you can allocate and deallocate memory in C++:
Player* player = new Player(); // Allocate memory for a new Player object
player->health = 100; // Set health for the player
delete player; // Free the allocated memory
In this example, we dynamically allocate memory for a `Player` instance using `new`, manipulate it, and then free that memory with `delete`. Mastering these concepts is essential for optimizing performance and preventing memory leaks in your games.
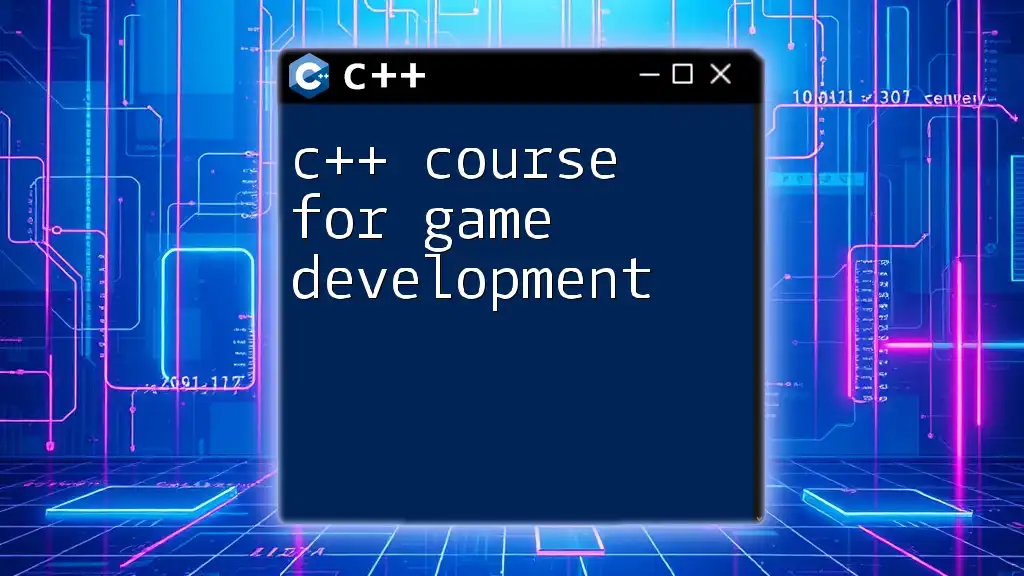
Game Development Frameworks and Libraries
Overview of Game Engines and Their Language Support
When you venture into game development, utilizing existing game engines can save time and help streamline the development process. Many engines are built with C++ as their core language:
-
Unreal Engine: One of the most popular engines, renowned for its high fidelity and robustness.
-
Cocos2d: A lighter engine suitable for 2D game development, offering C++ support.
By learning C++, you open yourself to a wealth of resources and support from these platforms, magnifying your capabilities in game development.
Libraries to Enhance Your C++ Game Development
In addition to game engines, numerous libraries enhance C++ game development. One popular choice is SFML (Simple and Fast Multimedia Library). Here’s a basic setup for initializing an SFML window:
#include <SFML/Graphics.hpp>
int main() {
sf::RenderWindow window(sf::VideoMode(800, 600), "My Game");
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
window.display();
}
return 0;
}
In this code, we create a simple game window that responds to close events. Utilizing libraries like SFML simplifies graphics handling and input management, allowing you to focus on game mechanics.
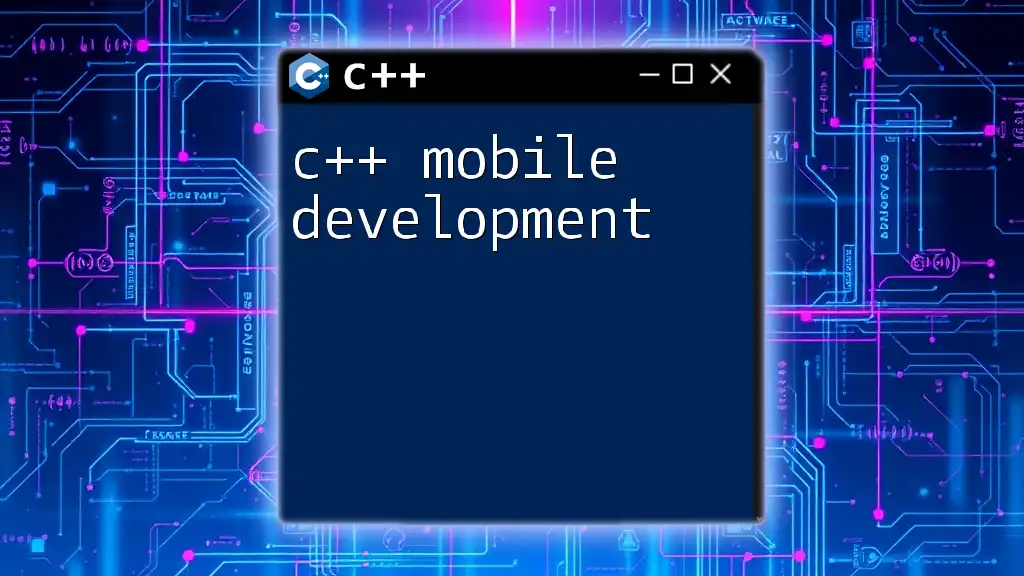
Designing Your First Game
Game Concept and Planning
Every successful game begins with a solid concept. Take the time to brainstorm and outline your game's key elements, including its core mechanics, story, and player objectives. Storyboarding your ideas visually can help clarify your vision and guide your development process.
Building the Game Loop
The game loop is a fundamental concept in game programming. It's the core cycle your game will run through, processing inputs, updating game states, and rendering graphics. Here’s a basic structure for a game loop:
while (running) {
handleInput();
updateGame();
render();
}
In this loop, each iteration processes user input, updates the game state (like player movements or interactions), and renders the new state to the screen.
Implementing Game Mechanics
Implementing gameplay features, including player controls, enemy AI, and scoring systems, is where your creativity will shine. Here’s a simple function for handling player input:
void handleInput() {
// Code to handle player input
}
Your input handling function will facilitate dynamic interactions, such as responding to keyboard events to move a character or trigger game actions.
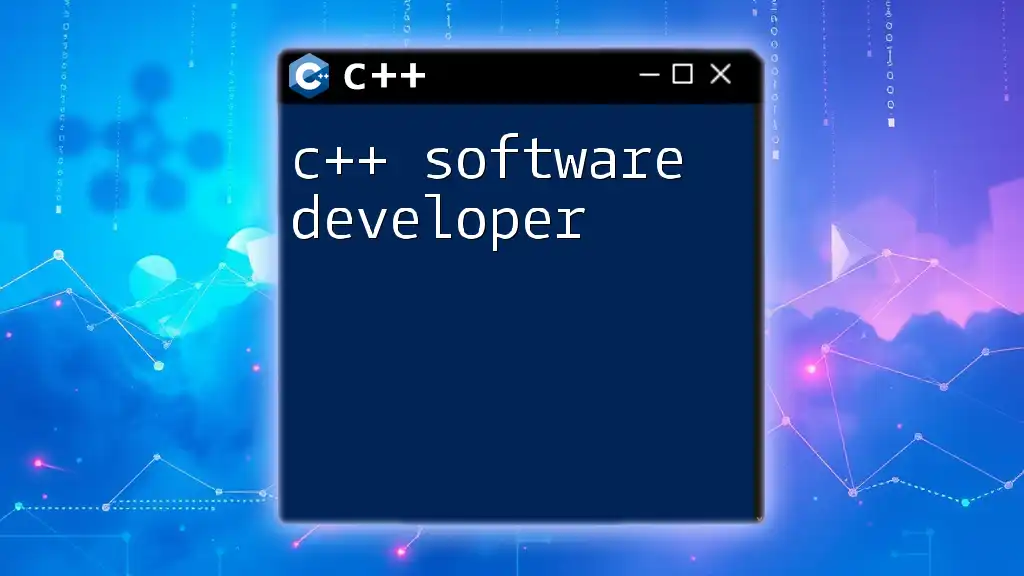
Resources for Learning C++ for Game Development
Online Courses and Tutorials
To effectively learn C++, consider enrolling in structured online courses. Platforms like Udemy and Coursera offer comprehensive programs, while YouTube features valuable tutorials from knowledgeable creators. Engaging with these resources can give you practical experience and help solidify your understanding.
Books and Documentation
Books such as "C++ Primer" and "Effective C++" provide in-depth insights into the language, while game development-specific texts can convey practical application. Always check official documentation for the libraries and engines you choose to use, as they provide essential information about implementation and best practices.
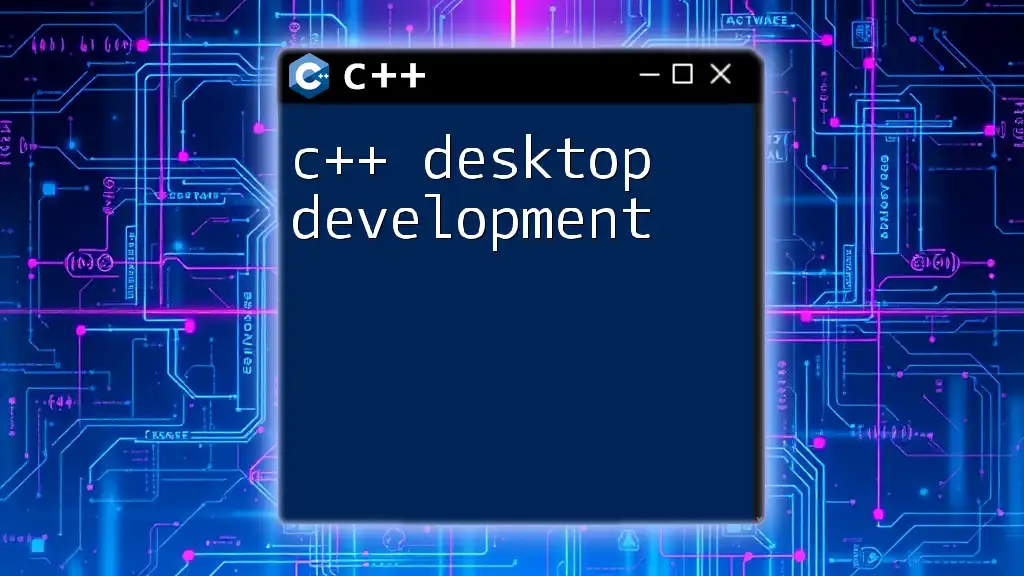
Conclusion
Embarking on the journey of learning C++ for game development can feel daunting, but it is incredibly rewarding. By building a solid foundation in C++ principles and practices, exploring game engines and libraries, and engaging with learning resources, you position yourself to create engaging and dynamic games.
As you venture into your game development projects, remember to practice, experiment, and remain active in the gaming community. Your unique ideas and creativity are the keys to creating memorable gaming experiences.