Explore our C++ course for game development, where you'll learn essential commands to create engaging games efficiently, like setting up a simple game loop below:
#include <iostream>
using namespace std;
int main() {
while (true) {
// Game logic here
cout << "Running game loop..." << endl;
}
return 0;
}
Introduction to C++ in Game Development
Understanding the Importance of C++
When it comes to game development, C++ is unrivaled in its flexibility and power. Its popularity stems from several key attributes:
- Performance Efficiency: C++ allows for low-level memory manipulation and is compiled directly into machine code, resulting in fast execution. This is crucial for real-time applications such as games.
- Memory Management: It provides developers control over memory allocation and deallocation, which is important for resource-intensive applications like games where performance can be affected by inefficient memory use.
- Object-Oriented Principles: C++ supports object-oriented programming, which is ideal for managing complex game systems through encapsulation, inheritance, and polymorphism.
Given these advantages, a C++ course for game development equips aspiring developers with the necessary tools to create engaging and high-performing games.
What This Course Will Cover
This course is structured to take you from basic concepts through to advanced techniques critical for game development:
- Basic Concepts: An introduction to C++ syntax, data types, and control structures.
- Intermediate Topics: A dive into classes, inheritance, and object-oriented design.
- Advanced Techniques: Insights into game engine architecture, frameworks, and implementing complex systems like physics.
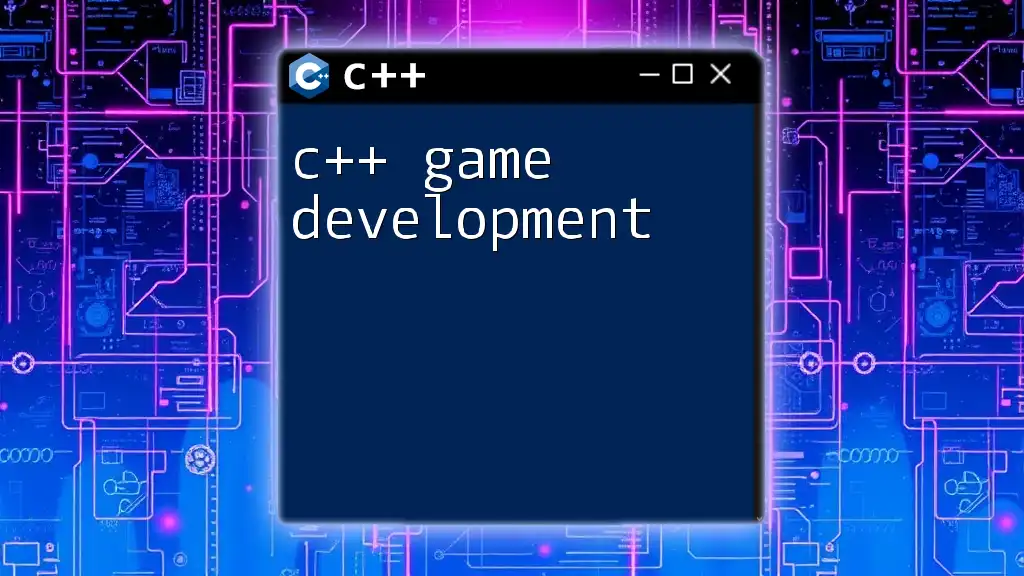
Setting Up Your Development Environment
Choosing the Right IDE
An integrated development environment (IDE) is essential for efficient coding. Several popular IDEs exist for C++ game development:
- Visual Studio: Highly recommended due to its powerful debugging tools and integration with game development frameworks.
- Code::Blocks: A lightweight, versatile option suitable for beginners.
- CLion: Ideal for modern C++ development with smart features like code analysis.
Installation and Setup Instructions: Follow the installation wizards for these IDEs, and ensure that you also install any necessary plugins that enhance game development capabilities.
Installing Necessary Libraries and Frameworks
Choosing the right libraries can significantly simplify game development. Here are a couple of essential libraries:
-
SDL (Simple DirectMedia Layer): This library provides a simple interface to various multimedia components. Follow the official documentation to install it and link it to your project.
-
SFML (Simple and Fast Multimedia Library): SFML is another great option for 2D game development. To install and configure SFML, download the binaries, link them properly in your project settings, and start utilizing its rich set of features.
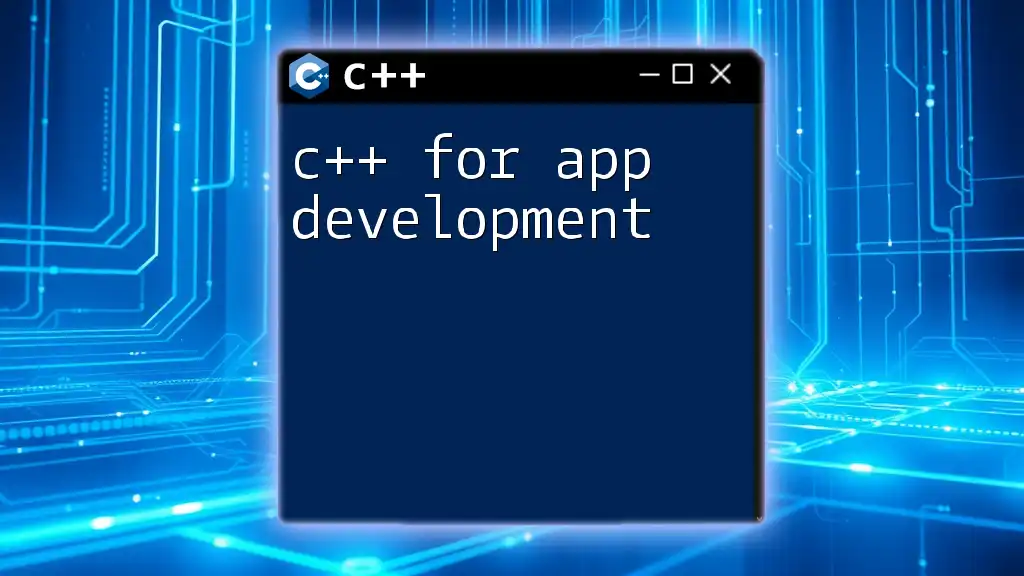
Basic C++ Concepts for Game Development
Understanding Basic Syntax and Data Types
Getting started with C++ requires familiarization with its syntax and data types:
-
Variables and Data Types: Understanding how to declare variables is crucial. For instance:
int playerScore = 0; // Represents the player's current score float playerHealth = 100.0f; // Represents the health, initialized to 100 char playerName = 'A'; // A character representing the player's identifier
-
Control Structures: Implement if-else statements to control game flow, for instance:
if (playerHealth <= 0) { // Logic for when the player’s health reaches zero }
Introduction to Functions and Input/Output
Functions in C++ serve as modular blocks of code that perform specific tasks. Here's how you can define a simple function and use input/output:
#include <iostream>
void displayScore(int score) {
std::cout << "Current Score: " << score << std::endl;
}
int main() {
int score = 10;
displayScore(score);
}
Utilizing `std::cout` for output and `std::cin` for input is vital for interactive gaming experiences.
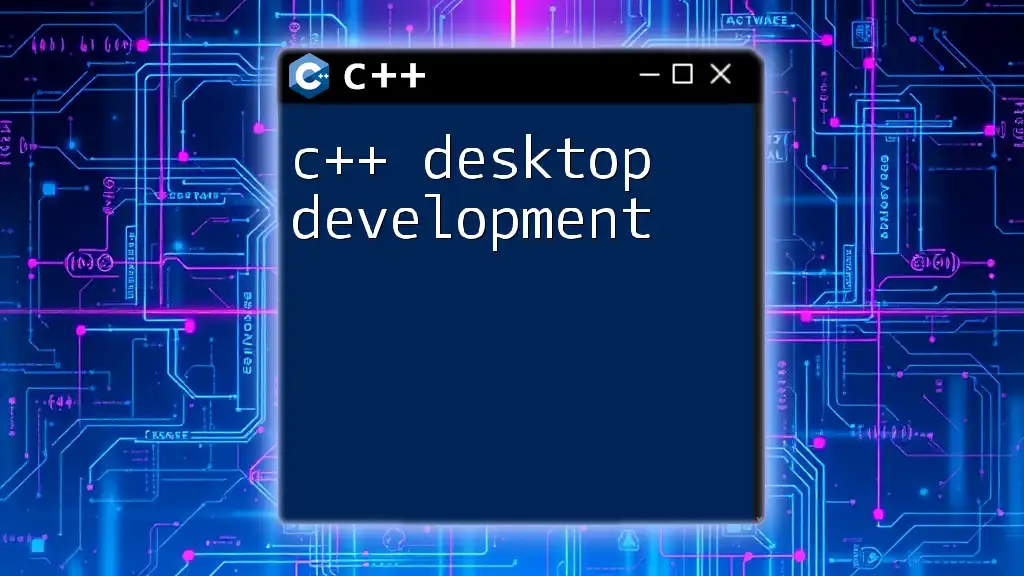
Object-Oriented Programming in C++
Classes and Objects: The Fundamentals
C++ shines through its support for object-oriented programming. To create a class and an object, follow this:
class Player {
public:
int health;
int score;
void displayStatus() {
std::cout << "Health: " << health << ", Score: " << score << std::endl;
}
};
Using Objects: You can instantiate an object of the class:
Player player1;
player1.health = 100; // Initialize the player's health
player1.score = 0; // Set the initial score
player1.displayStatus(); // Call the method to display the status
Inheritance and Polymorphism
Inheritance allows you to create new classes based on existing ones. This can simplify code and enhance reusability:
class Enemy : public Player {
public:
void takeDamage(int damage) {
health -= damage; // Subtract from health
}
};
This establishes a hierarchy where `Enemy` inherits properties from `Player`, demonstrating the power of polymorphism when combined with virtual functions.
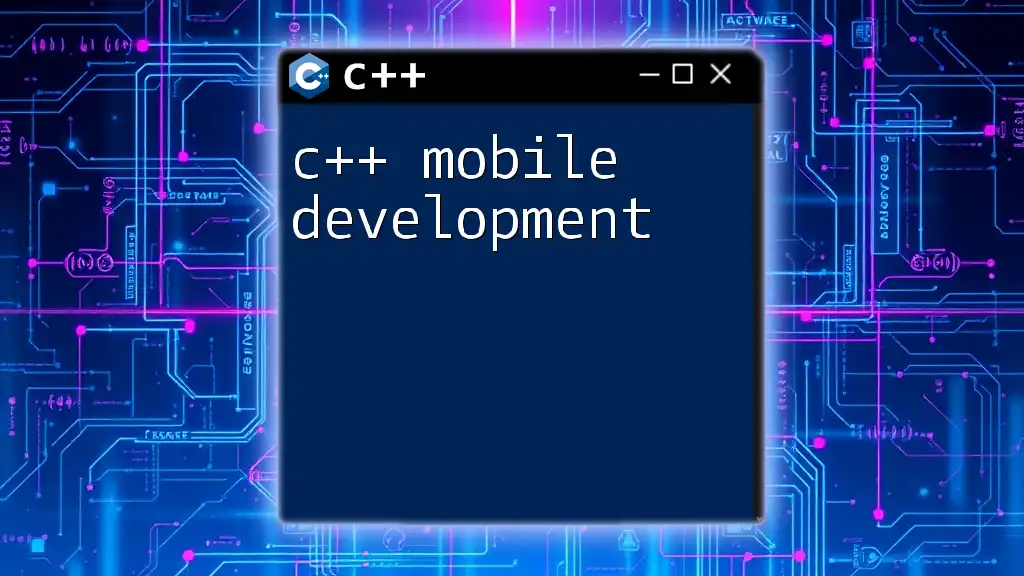
Intermediate Game Development Techniques
Introduction to Game Loops
Every game relies on a core structure known as the game loop, which cycles between processing input, updating the game state, and rendering graphics:
while (gameRunning) {
processInput(); // Capture player actions
updateGame(); // Update game state based on those actions
renderGraphics(); // Draw the current game state to the screen
}
Working with Graphics
Creating visuals in a game starts with setting up a window and rendering graphics. Using SFML, you might do the following:
sf::RenderWindow window(sf::VideoMode(800, 600), "Game Window");
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
// Add rendering logic here
window.display();
}
Rendering shapes or sprites is essential for building an engaging visual experience within your game.
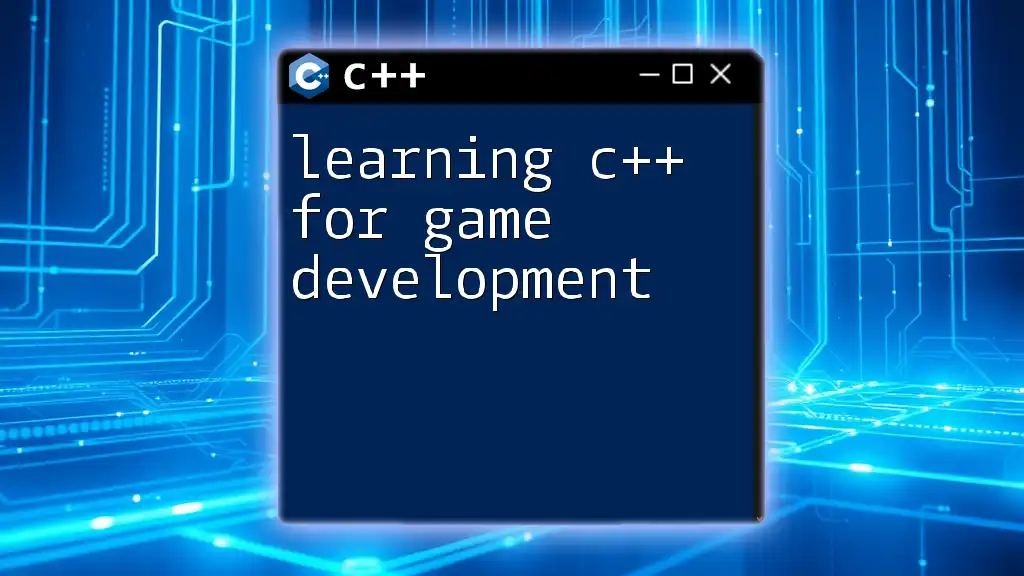
Advanced Concepts in Game Development
Building Game Engines
Understanding the architecture of game engines can significantly streamline your development process. You'll often encounter two types of engines:
- Micro Engines: Lightweight and focused on specific functionality. Great for simpler projects.
- Macro Engines: Full-featured engines like Unreal or Unity that encompass libraries for every aspect of game design.
Engine Architecture Overview: Key components include game states, resource management, and event handling. A popular structure to follow is the entity-component-system (ECS) model, which promotes clean separation of logic and data.
Implementing Game Physics
Incorporating physics enhances realism in games. A basic understanding of gravity, collision detection, and how to respond to interactions is critical.
- Using Physics Libraries: Libraries such as Box2D can handle complex physics calculations. Implementing these systems on game objects adds realism without extensive coding from scratch.
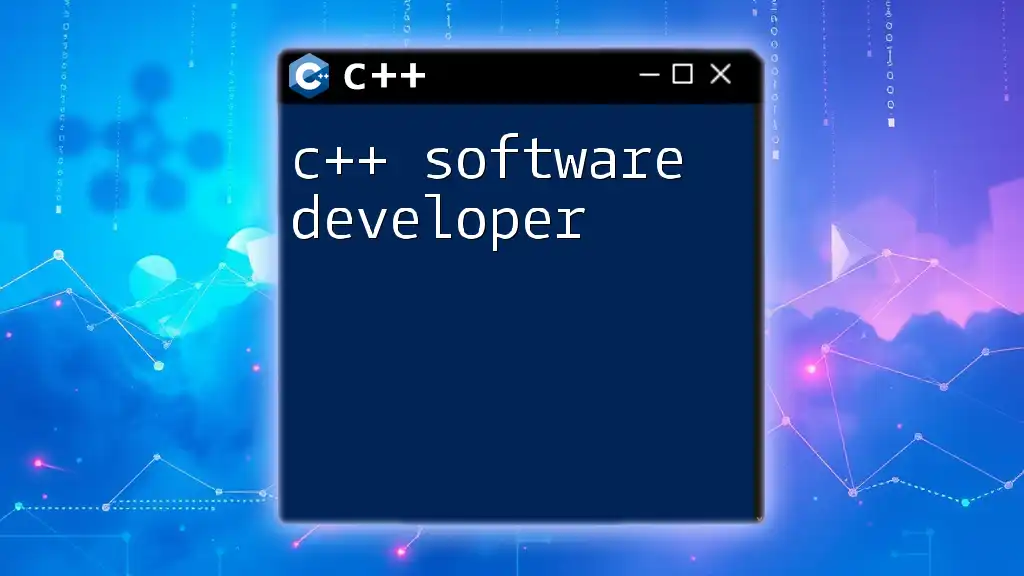
Practical Project: Creating a Simple Game
Step-by-Step Project Overview
Deciding on a game type is crucial. Whether you choose to develop a 2D platformer, shooter, or puzzle game, the approach will generally follow a similar structure.
Code Snippets for Each Stage
-
Creating the Game Window: Start with setting up your window as shown in previous examples.
-
Adding Player Controls: To handle player inputs, implement keyboard event listeners that modify the player's position based on key presses.
-
Implementing Scoring and Health Systems: Incorporate score increments based on gameplay events (like collecting coins) and create methods for managing player health (such as receiving damage from enemies).
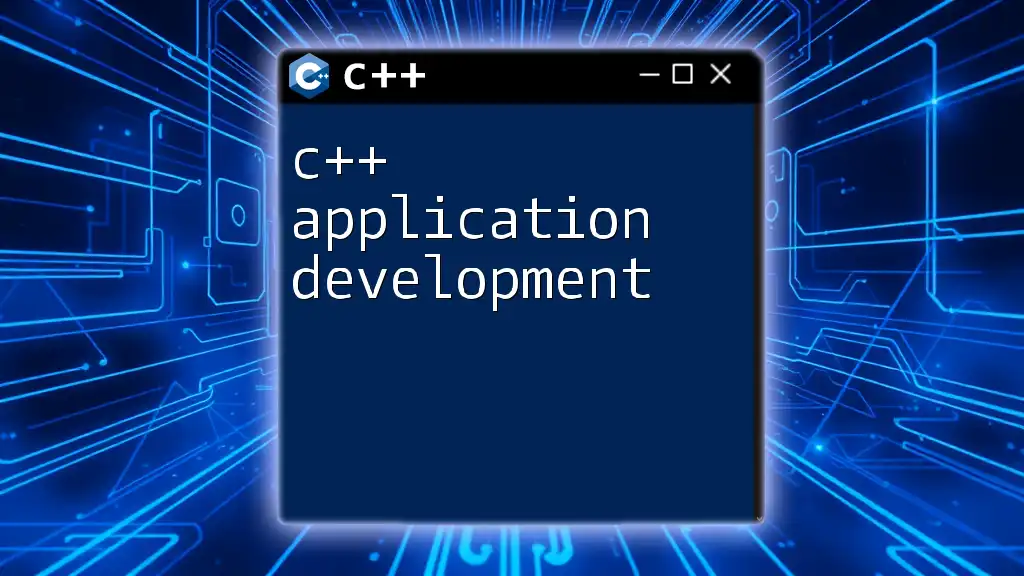
Debugging and Optimization Techniques
Common Debugging Strategies
Debugging is an integral part of game development. Utilizing the built-in debugging tools of your IDE allows for breakpoints and variable tracking, which can help identify issues directly.
- Logging and Error Handling: Implement robust logging to track game events and errors. Use `std::cout` to output messages that can assist in debugging and maintain system health while the game is running.
Optimizing Game Performance
As games become more complex, optimizing performance is essential:
- Memory Management Tips: Use smart pointers and avoid memory leaks. Regularly monitor memory usage to ensure efficient resource allocation.
- Profile Your Game: Use profiling tools to identify performance bottlenecks in graphics rendering or game logic.
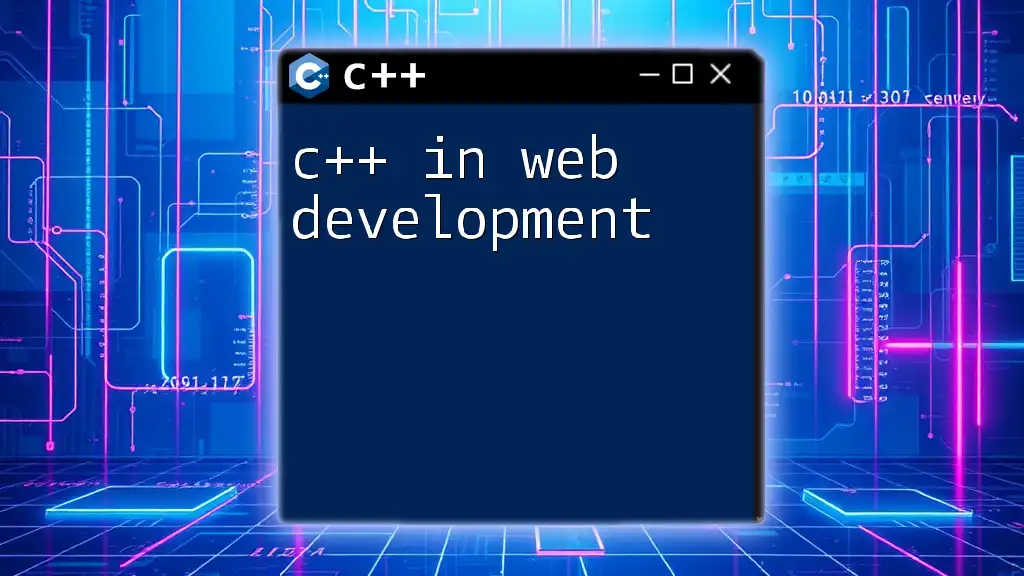
Conclusion
Recap of Key Points
Throughout this course on C++ for game development, we covered critical concepts, from basic syntax and OOP principles to advanced game engine architecture and real-time physics.
Resources for Further Learning
Continue your journey with books, online tutorials, and active participation in game development communities. This will further solidify your skills and provide invaluable networking opportunities.
Call to Action
Join our C++ game development course today! Learn, grow, and create engaging games that capture audiences worldwide. Subscribe for updates and stay informed about new tips, tricks, and resources!