C++ memory management involves allocating and deallocating memory dynamically using operators like `new` and `delete` to optimize resource usage while preventing memory leaks.
Here's a code snippet demonstrating basic memory management in C++:
#include <iostream>
int main() {
int* ptr = new int; // allocate memory
*ptr = 42; // assign value
std::cout << *ptr << std::endl; // output value
delete ptr; // deallocate memory
return 0;
}
Understanding Memory Management in C++
What is Memory Management?
Memory management refers to the process of allocating and deallocating memory for a program during its execution. In C++, effective memory management is crucial, as it directly impacts both performance and resource utilization. A well-managed memory space allows a program to run efficiently, while poor memory management can lead to issues such as memory leaks, performance degradation, and even crashes.
C++ offers a level of control over memory that many higher-level programming languages do not. In languages with automatic garbage collection, programs don’t need to explicitly manage memory; however, C++ developers must handle memory manually. This necessity stems from the desire for speed and efficiency, giving developers more direct access to memory operations, at the cost of additional complexity.
Why C++ Requires Manual Memory Management
C++ differentiates itself by prioritizing performance. Automatic memory management comes with overhead; the garbage collector consumes time and resources ensuring that memory is freed when it's no longer needed. In contrast, C++ enables developers to allocate and release memory as needed, optimizing resource use and performance.
This manual memory management allows for finer control over how memory is utilized, which is particularly useful in systems programming or applications where performance is critical, such as real-time systems.
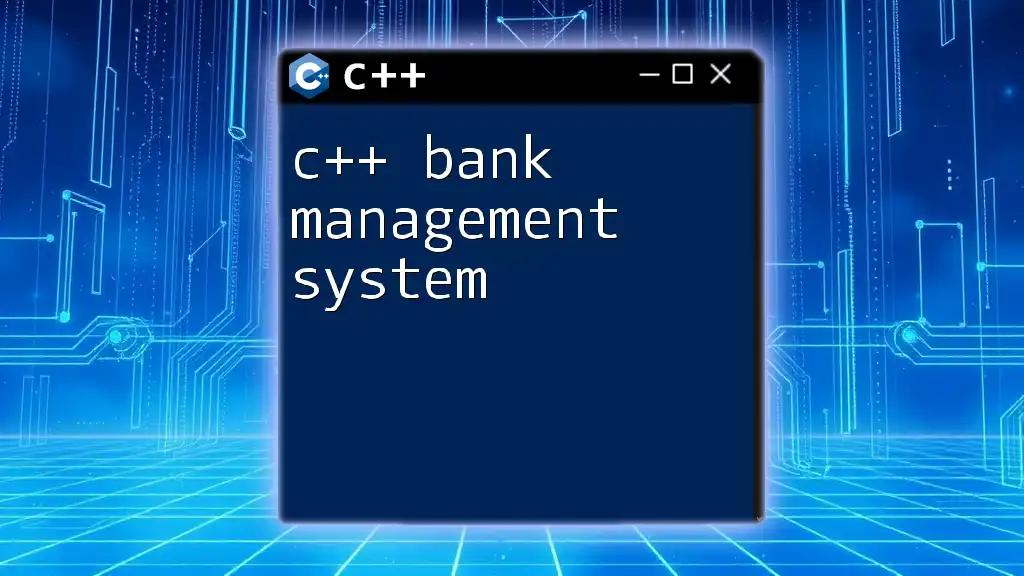
Types of Memory in C++
Stack Memory
Stack memory is a region where local variables are stored. It operates on a Last In, First Out (LIFO) principle. The primary characteristics of stack memory include:
- Automatic allocation and deallocation: Memory for local variables is allocated on entering a function and deallocated upon exiting.
- Limited size: Stack space is often smaller compared to heap space and may lead to stack overflow if abused, such as with too many nested function calls.
For example, consider the following code snippet:
void stackExample() {
int a = 10; // 'a' is allocated on the stack
// Do something with 'a'
} // 'a' is deallocated here when the function exits
In this snippet, the integer variable `a` is allocated on the stack when `stackExample` is called. It ceases to exist once the function returns.
Heap Memory
Unlike stack memory, heap memory is allocated dynamically at runtime. This type of memory allows for larger allocations and persists until explicitly deallocated, making it more flexible but also more prone to errors if not managed correctly.
Heap memory is characterized by:
- Manual allocation and deallocation: Resources continue to stay allocated until they are explicitly freed using `delete`.
- Global accessibility: Variables allocated on the heap can be accessed throughout the program, making them suitable for varied data structures.
The following example illustrates heap allocation:
void heapExample() {
int* p = new int; // Allocate memory on the heap
*p = 20; // Assign value
// Use 'p'
delete p; // Free the allocated memory
}
In this example, an integer is allocated on the heap. Remember to use `delete` to free this memory to avoid leaks.

Memory Allocation in C++
Dynamic Memory Allocation
Dynamic memory allocation in C++ is achieved using `new` and `delete` operators. This process allows allocating memory during runtime based on the program’s needs.
When using dynamic allocation, consider the following:
- Allocate single variables: `int* p = new int;`
- Allocate arrays: `int* array = new int[5];`
Here’s an example:
void dynamicAllocation() {
int* array = new int[5]; // Allocate an array on the heap
// Use array...
delete[] array; // Free the memory allocated for the array
}
In this case, `delete[]` is essential for freeing the memory allocated for the array to prevent memory leaks.
Memory Leaks and How to Avoid Them
Memory leaks occur when allocated memory is not deallocated, leading to a gradual reduction of available memory in your application. Common causes of memory leaks include:
- Forgetting to call `delete` after using `new`.
- Losing references to dynamically allocated memory.
Consider the following example that demonstrates a memory leak:
void memoryLeakExample() {
int* leak = new int(30); // Memory leak if not deleted
// No delete here, leading to memory waste
}
To prevent leaks, ensure all dynamically allocated memory is freed. Utilize tools like Valgrind to track memory usage and identify leaks during development.

Smart Pointers: Modern C++ Memory Management
What are Smart Pointers?
Smart pointers are a modern C++ feature that simplifies memory management by automatically handling resource cleanup. They reduce the chances of memory leaks and dangling pointers by employing reference counting and scoped lifetimes.
Unique Pointers
The `std::unique_ptr` type embodies ownership, ensuring that only one unique pointer can own a resource. This structure eliminates the need for manual deallocation as the memory is released automatically when the pointer goes out of scope.
Here is how you can use `std::unique_ptr`:
#include <memory>
void uniquePointerExample() {
std::unique_ptr<int> ptr(new int(40)); // Automatic memory management
// No need to manually delete, goes out of scope automatically
}
Shared Pointers
`std::shared_ptr` allows multiple pointers to share ownership of a dynamically allocated object. It uses reference counting to manage resources, with the memory being freed when the last `shared_ptr` referencing it goes out of scope.
An example might look like this:
#include <memory>
void sharedPointerExample() {
std::shared_ptr<int> ptr1(new int(50));
{
std::shared_ptr<int> ptr2 = ptr1; // Shared ownership
} // Memory still exists here since ptr1 is still valid
}
Weak Pointers
`std::weak_ptr` complements `shared_ptr` by providing a way to reference an object without affecting its reference count. This mechanism helps prevent cyclic references, which can lead to memory leaks.
Consider the following example:
#include <memory>
void weakPointerExample() {
std::shared_ptr<int> sharedPtr(new int(60));
std::weak_ptr<int> weakPtr = sharedPtr; // Does not affect reference count
// Use weakPtr...
}
In this example, `weakPtr` allows access to `sharedPtr` without taking ownership, safeguarding against potential memory leaks in circular references.

Conclusion: Best Practices in C++ Memory Management
Effective C++ memory management is essential for crafting high-performance applications. Understanding the differences between stack and heap memory, leveraging smart pointers, and practicing proper allocation and deallocation techniques are vital for any developer.
Summary of Key Points
- Memory Types: Differentiate between stack and heap memory along with their characteristics.
- Dynamic Allocation: Utilize `new` and `delete` operators correctly.
- Smart Pointers: Integrate `std::unique_ptr`, `std::shared_ptr`, and `std::weak_ptr` for safer resource management.
- Prevent Leaks: Always ensure appropriate memory deallocation to avoid leaks.
Resources for Further Learning
For those eager to expand their knowledge, numerous resource options exist. Consider diving into books like "Effective C++" by Scott Meyers or exploring online platforms such as Codecademy and Coursera.
Final Thoughts
Mastering C++ memory management not only elevates your programming skills but also equips you with the insights needed to tackle performance issues and resource allocation challenges. Practice these concepts continually, and feel free to explore advanced memory management techniques to deepen your understanding.