In C++, memory allocation allows you to dynamically reserve memory during runtime using operators like `new` and `delete`, facilitating efficient management of resources.
int* ptr = new int; // Allocates memory for an integer
*ptr = 42; // Assigns value to the allocated memory
delete ptr; // Frees the allocated memory
What is Memory Allocation?
Memory allocation refers to the process of reserving a certain amount of memory in a program while it's running. This process is crucial in programming, as it directly impacts how efficiently your application can utilize resources, manage data, and perform tasks. A well-managed memory allocation can lead to improved performance and reduced risk of program crashes.
In C++, dynamic memory allocation is particularly important, given the language's flexibility and the need for efficient resource management. C++ provides developers with significant control over memory management, allowing for both static and dynamic allocation. There are three primary types of memory allocation:
- Static Allocation: Memory is allocated at compile-time and remains fixed throughout the program's life.
- Stack Allocation: Memory is allocated for function calls, and it is automatically managed when functions are exited.
- Heap Allocation: Memory is allocated at runtime, allowing for more flexible and potentially larger allocations. This is where C++ memory allocation shines.
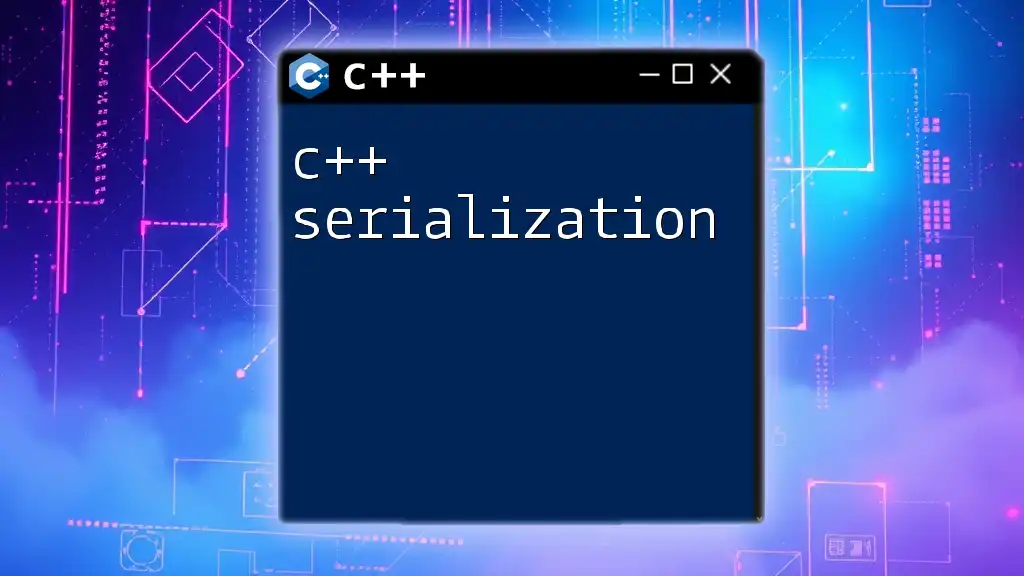
Dynamic Memory Allocation in C++
What is Dynamic Memory Allocation?
Dynamic memory allocation allows you to request memory at runtime, providing flexibility in managing your application's memory needs. This is especially useful when the amount of memory required cannot be determined beforehand, such as when handling variable-sized data structures like linked lists or dynamic arrays.
C++ Memory Allocation Functions
`new` Operator
The `new` operator is the primary mechanism for allocating memory in C++. It allocates memory for a variable of a specific type and returns a pointer to that memory.
Basic Usage: You can use the `new` operator to allocate a single variable and access it via a pointer.
Example:
int* ptr = new int;
*ptr = 42; // Now ptr points to an integer with the value of 42
`new[]` Operator
For allocating an array of objects, you can use the `new[]` operator. This operator provides the ability to allocate memory for multiple objects at once.
When to Use: Whenever the size of an array is not known in advance.
Example:
int* arr = new int[5]; // Allocates an array of 5 integers
Deallocating Memory in C++
After allocating memory, it's essential to free that memory when it is no longer needed to avoid memory leaks.
`delete` Operator
The `delete` operator is used to deallocate memory that was previously allocated with `new`.
Usage: Make sure to free memory when it is no longer needed.
Example:
delete ptr; // Deallocates memory pointed to by ptr
`delete[]` Operator
To deallocate an array that was allocated with `new[]`, you need to use `delete[]`.
When to Use: Whenever you allocate memory for an array, use `delete[]` to free it.
Example:
delete[] arr; // Deallocates the array memory
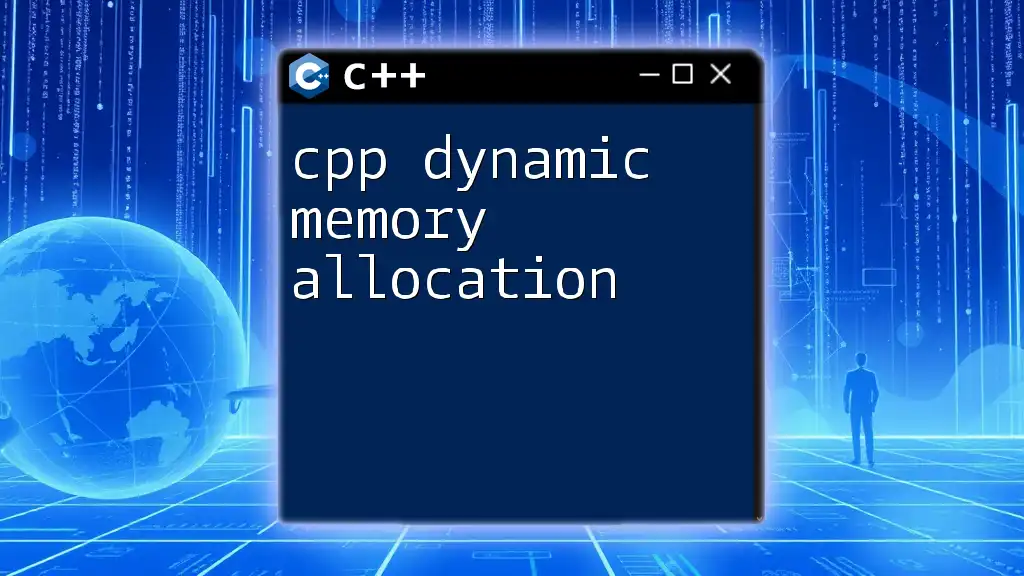
Best Practices for Memory Allocation in C++
Avoiding Memory Leaks
Memory leaks occur when the program allocates memory but fails to deallocate it after use, leading to wasted resources and potential application failure. To avoid memory leaks, follow these guidelines:
- Always match `new` with `delete`: Every allocation should have a corresponding deallocation.
- Use smart pointers, which automatically manage memory and help prevent leaks.
Using Smart Pointers in C++
Smart pointers are an important feature in modern C++ that automate memory management and significantly reduce the risk of memory leaks.
What are Smart Pointers?
Smart pointers are objects that manage memory via pointers and automatically handle memory deallocation.
Types of Smart Pointers
- `std::unique_ptr`: A smart pointer that owns a dynamically allocated object exclusively. It's non-copyable but movable.
Example:
#include <memory>
std::unique_ptr<int> ptr(new int(42)); // Automatically deallocates when ptr goes out of scope
- `std::shared_ptr`: A smart pointer that allows multiple pointers to own the same object. The object is deallocated when the last `shared_ptr` goes out of scope.
Example:
#include <memory>
std::shared_ptr<int> ptr = std::make_shared<int>(42); // Memory is freed when the last shared_ptr goes out of scope
- `std::weak_ptr`: A smart pointer that holds a non-owning reference to an object managed by `shared_ptr`. It's useful to prevent circular references.
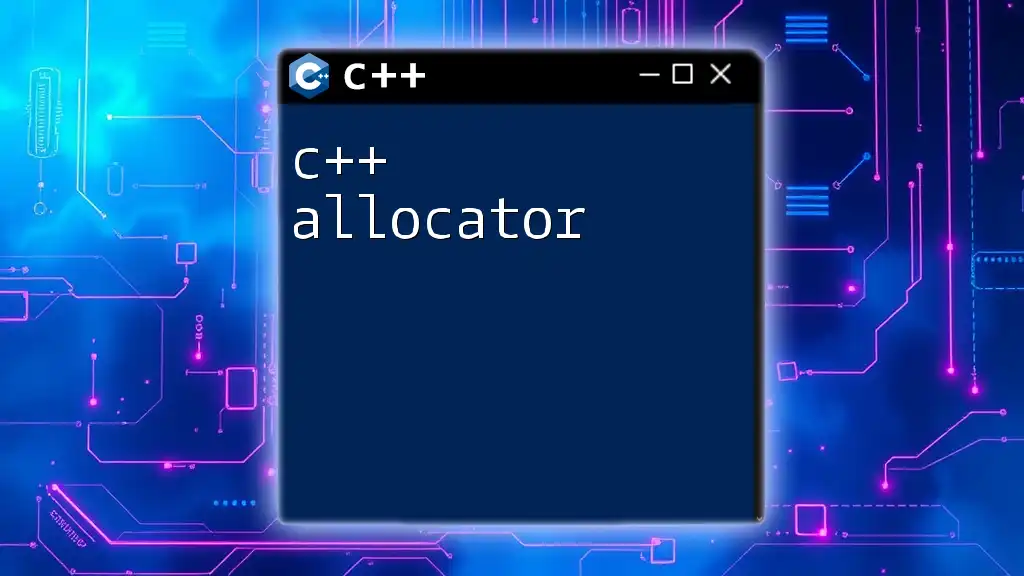
Error Handling During Memory Allocation
Handling Memory Allocation Failures
Failing to allocate memory can lead to serious issues in C++. When using `new`, if memory allocation fails, it throws a `std::bad_alloc` exception.
To avoid crashes caused by memory allocation failures, incorporate error handling in your code. Always validate the result of a memory allocation.
Example of safe dynamic allocation:
int* array = nullptr;
try {
array = new int[1000]; // Attempt to allocate memory for an array of 1000 integers
} catch (const std::bad_alloc& e) {
std::cerr << "Memory allocation failed: " << e.what() << '\n';
}
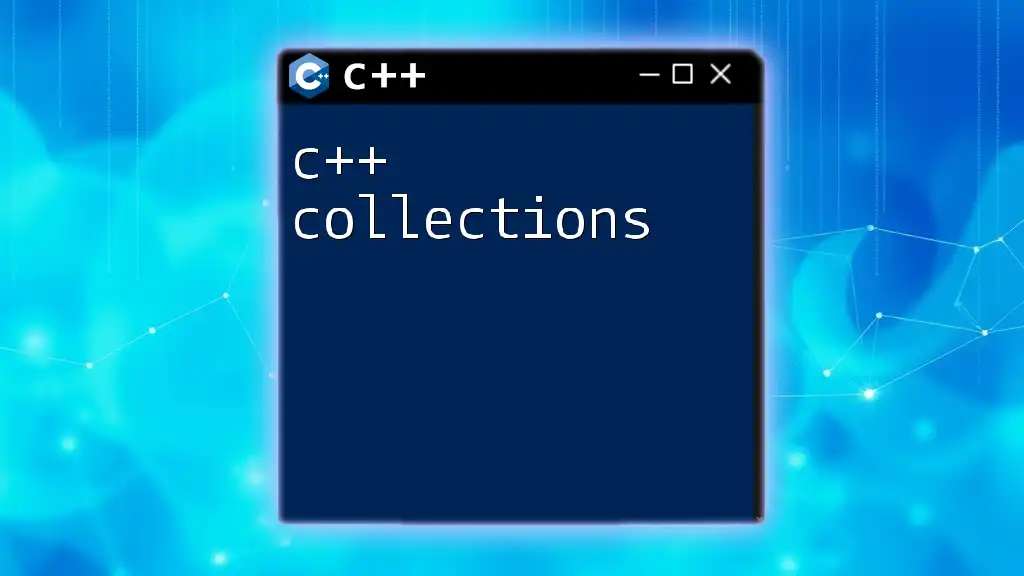
Common Mistakes in Memory Allocation
Misuse of Pointers
One common mistake in memory management is dealing with dangling pointers—pointers that point to freed memory. This can lead to unpredictable behavior and crashes. Always ensure that pointers are properly managed.
Not Deallocating Memory
Failing to deallocate memory can lead to degraded performance and crashes in long-running applications. Be vigilant about freeing memory when it is no longer needed. Using smart pointers can significantly mitigate this risk.
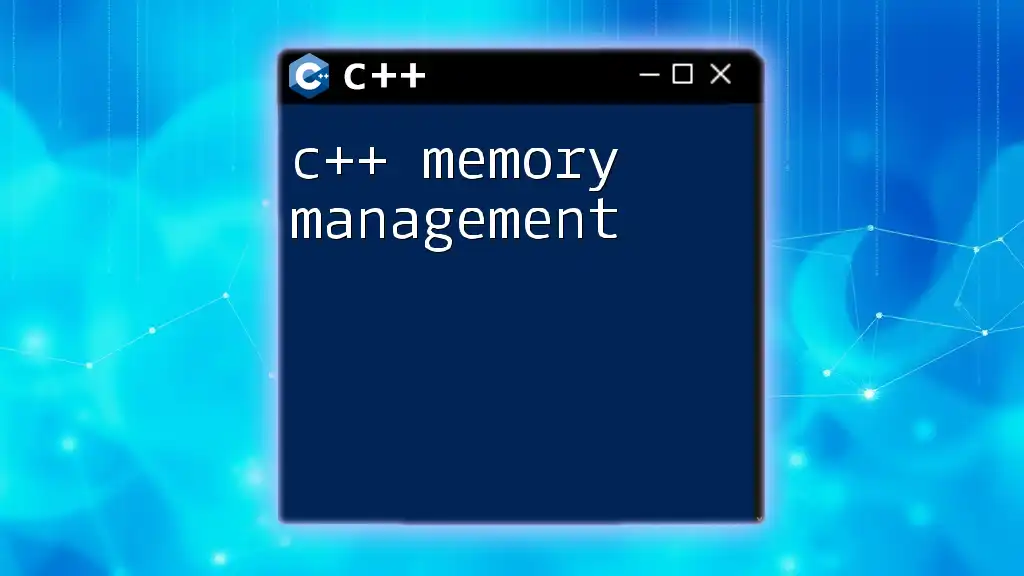
Conclusion
In summary, understanding C++ memory allocation is critical for any developer looking to utilize the language effectively. Proper dynamic memory allocation practices enhance performance and prevent memory leaks. Always utilize features like smart pointers to streamline memory management and encourage safer coding practices. Mastering the art of memory allocation is a valuable skill that will greatly improve the reliability and efficiency of your C++ applications.
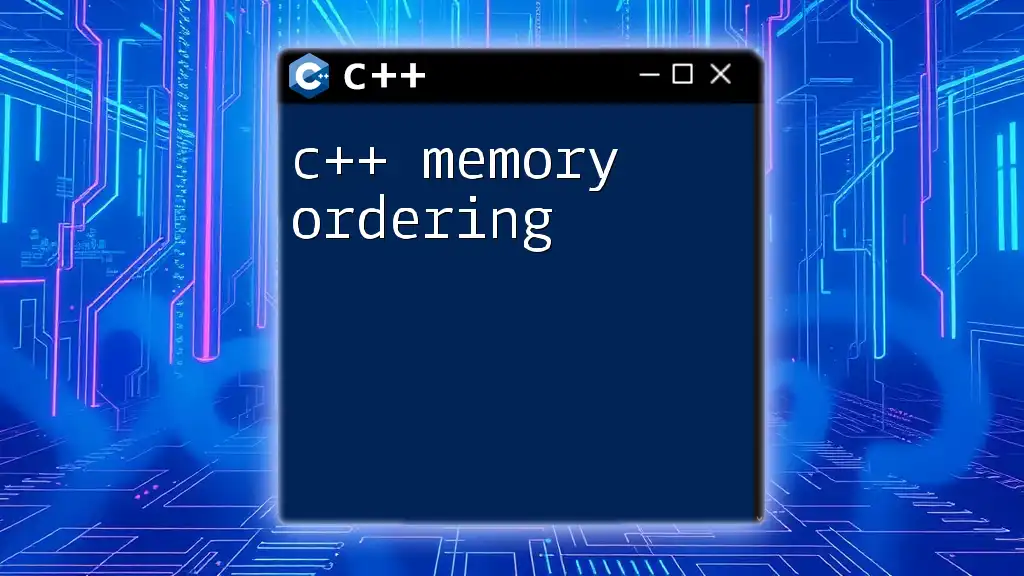
Further Reading and Resources
For those eager to explore more about C++ memory management, consider diving into the following:
- Books on C++ programming and memory management
- Online courses focusing on advanced C++ techniques
- Community forums and discussion boards, where you can engage with fellow developers
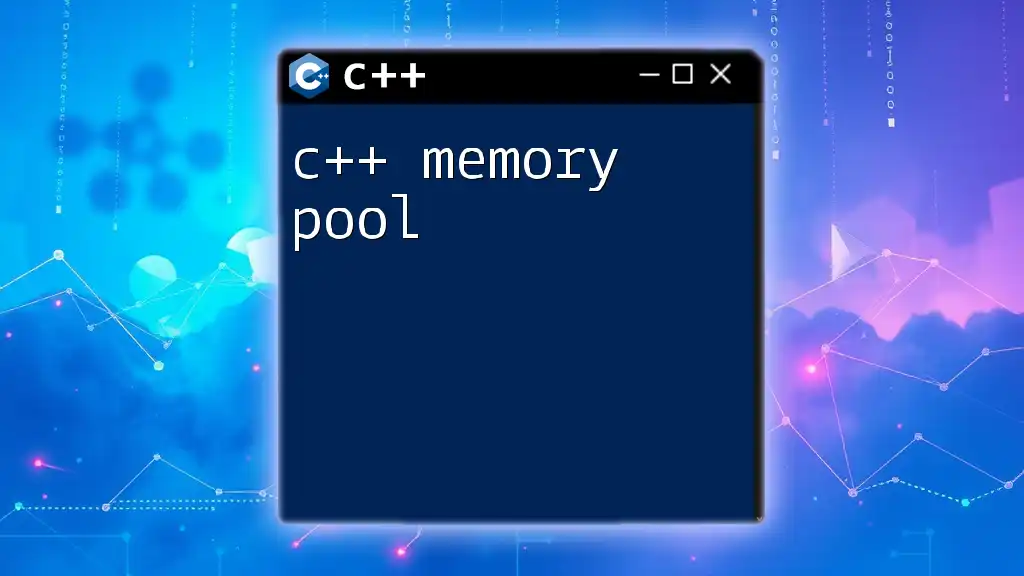
Engage With Us!
Have you faced challenges with C++ memory allocation? Share your experiences and questions in the comments below!