In C++, a dynamic allocation array allows you to create arrays whose size can be determined at runtime using pointers and the `new` operator.
Here's an example:
#include <iostream>
int main() {
int size;
std::cout << "Enter the size of the array: ";
std::cin >> size;
int* dynamicArray = new int[size]; // Dynamically allocate array
// Example of populating the array
for (int i = 0; i < size; i++) {
dynamicArray[i] = i * 2; // Assigning values
}
// Displaying the array elements
for (int i = 0; i < size; i++) {
std::cout << dynamicArray[i] << " ";
}
delete[] dynamicArray; // Freeing allocated memory
return 0;
}
What is Dynamic Allocation?
Dynamic allocation in C++ refers to the process of allocating memory during the runtime of a program, as opposed to compile time. This method allows developers to create arrays that can change in size based on user input or other factors, providing flexibility that static arrays cannot offer.
Importance of Dynamic Allocation
Dynamic allocation is crucial in various programming scenarios. For instance, when the size of an array cannot be determined before runtime—such as when working with user input or reading data from a file—dynamic allocation becomes essential. This capability enables programs to be more efficient in memory usage, as it allows for the allocation of only the necessary amount of memory.
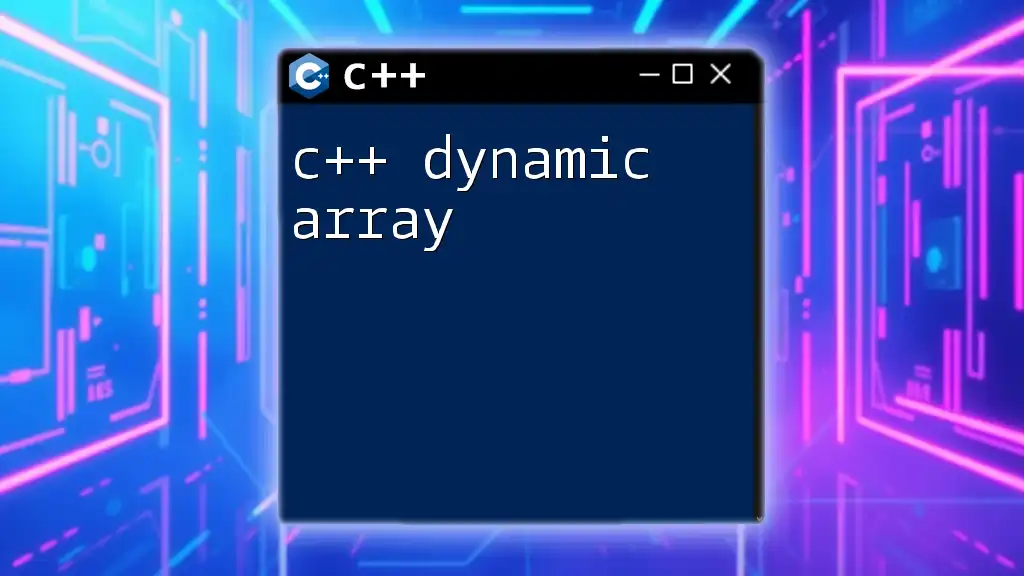
Understanding Arrays in C++
Overview of Arrays
In C++, an array is a collection of elements, all of the same data type, stored in contiguous memory locations. Arrays are static by nature, meaning their size must be declared at compile time. This can lead to memory wastage when arrays are either too large for the intended use or too small, requiring workarounds.
Static vs. Dynamic Arrays
The primary difference between static and dynamic arrays lies in how memory is allocated. Static arrays have a fixed size determined at compile time, whereas dynamic arrays can change in size during execution. This makes dynamic arrays significantly more versatile. For example, if an application needs to store a list of items whose quantity may vary, dynamic allocation provides the necessary flexibility.
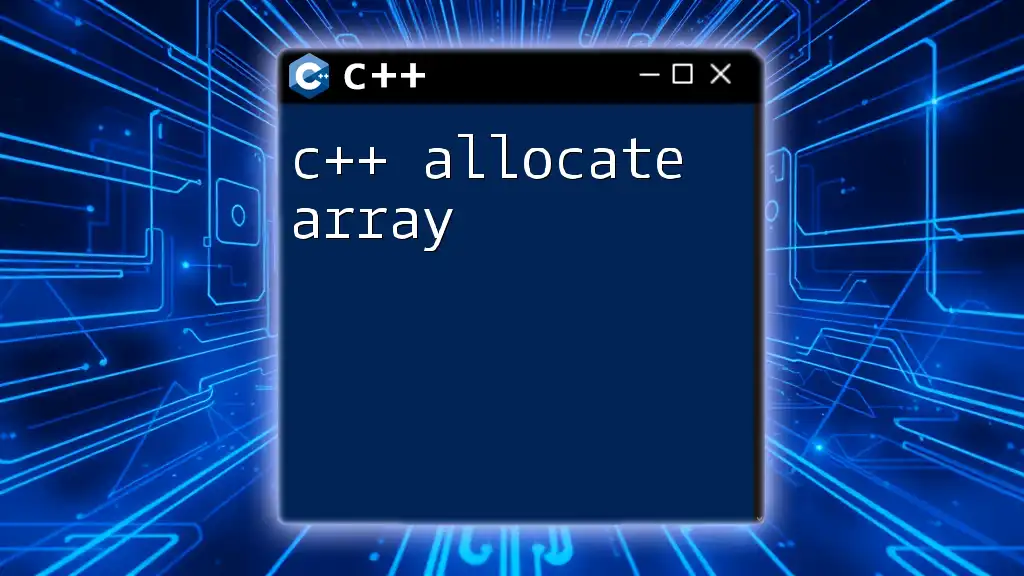
How to Dynamically Allocate an Array in C++
Syntax for Dynamic Allocation
The syntax for dynamically allocating an array in C++ utilizes the `new` keyword. This process creates a block of memory on the heap, which is suitable for objects whose lifetime will exceed the scope in which they were created.
type* arrayName = new type[arraySize];
Where `type` is the data type of the elements (e.g., `int`, `float`, etc.), `arrayName` is a pointer to the array, and `arraySize` is the desired number of elements.
Steps for Dynamic Allocation
To dynamically allocate an array, follow these essential steps:
-
Choose an Appropriate Data Type: Determine the data type of the array elements you will use.
-
Define the Array Size: Establish how many elements you need. It can be obtained from user input, allowing your program to adjust dynamically.
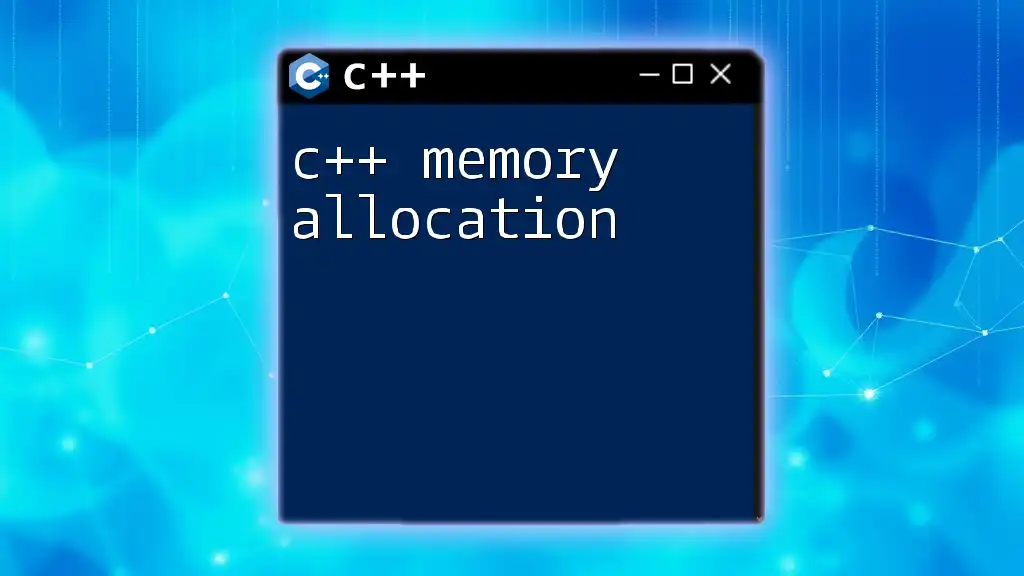
Example: Dynamically Allocate Array
Code Snippet
Here’s a simple example of dynamically allocating an array:
int* arr = new int[5]; // creates an array of 5 integers
Explanation of the Code
In this snippet, `arr` is a pointer that holds the memory address of the first element of an integer array containing 5 elements. The `new` operator allocates memory on the heap for the array, and `arr` points to this allocated memory. It’s important to remember that by using dynamic allocation, resources remain available even once the program exits the scope where the allocation occurred.
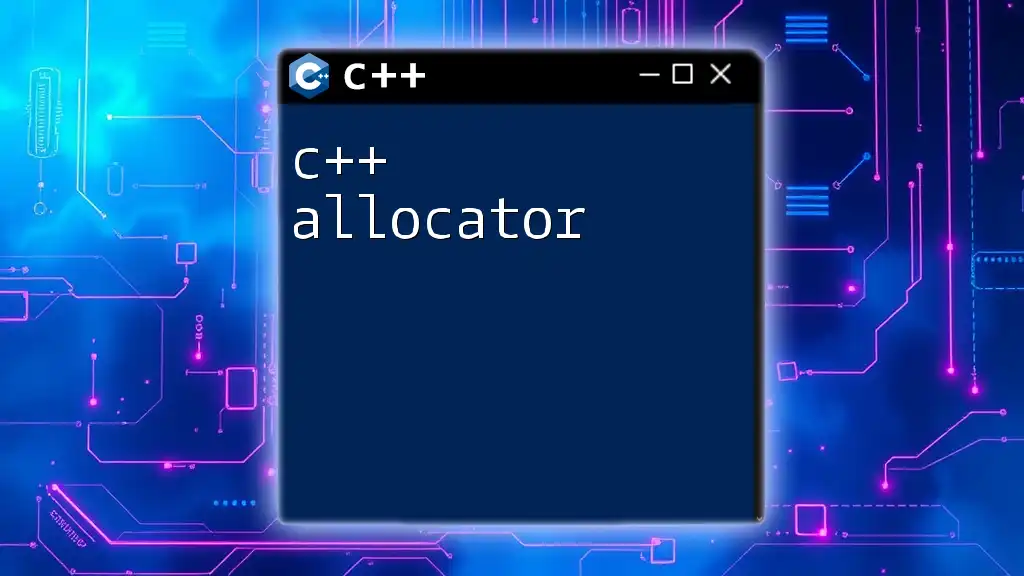
Accessing and Using Dynamically Allocated Arrays
Accessing Elements
You can access elements in a dynamically allocated array much like you would with a static array:
arr[0] = 10; // Assign value to the first element
In this line, the first element of the array `arr` is assigned a value of 10. This syntax makes it easy to interact with array elements.
Looping Through the Array
To iterate through a dynamically allocated array, you can use a loop, as shown in the following snippet:
for (int i = 0; i < 5; ++i) {
std::cout << arr[i] << " ";
}
This loop starts with `i = 0`, and continues until `i` is less than 5, printing each element in the array. This allows you to effectively manage and utilize the contents of your dynamically allocated array.
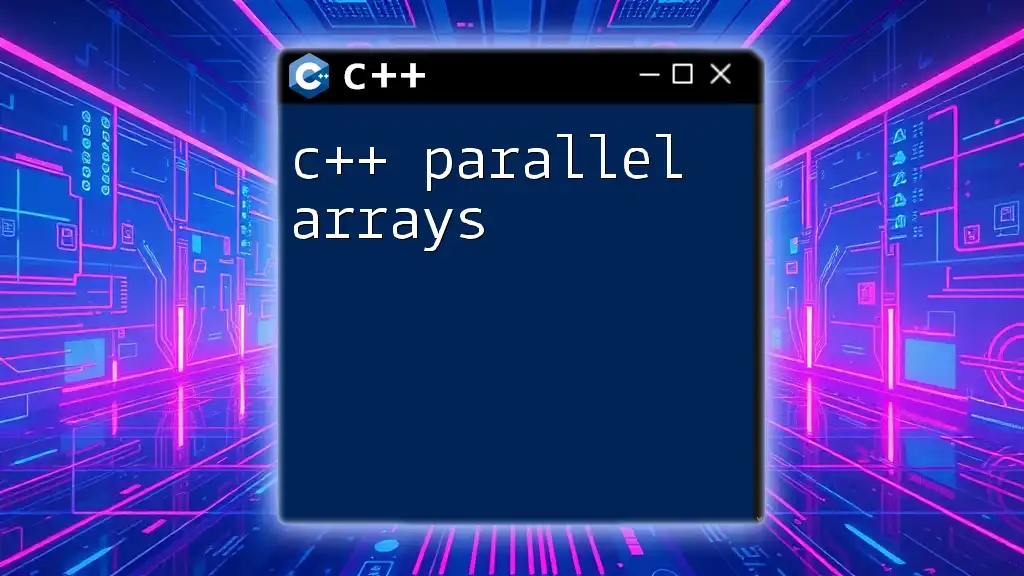
Deallocating Dynamic Arrays
Importance of Deallocation
When you dynamically allocate memory, it is imperative to deallocate it after use to avoid memory leaks. Memory leaks occur when allocated memory is not freed, leading to wasted resources which can cripple larger applications.
Syntax for Deallocation
The syntax to deallocate a dynamically allocated array utilizes the `delete[]` operator:
delete[] arrayName;
This action releases the memory back to the system, making it available for other processes.
Example: Safe Deallocation
Combining allocation and deallocation, here is a complete example demonstrating the usage of dynamic arrays:
int* arr = new int[5]; // Dynamically allocate array
// Use the array
delete[] arr; // Free memory
In this code, we allocate an array for 5 integers, can manipulate it as needed, and finally deallocate it to prevent memory leaks.
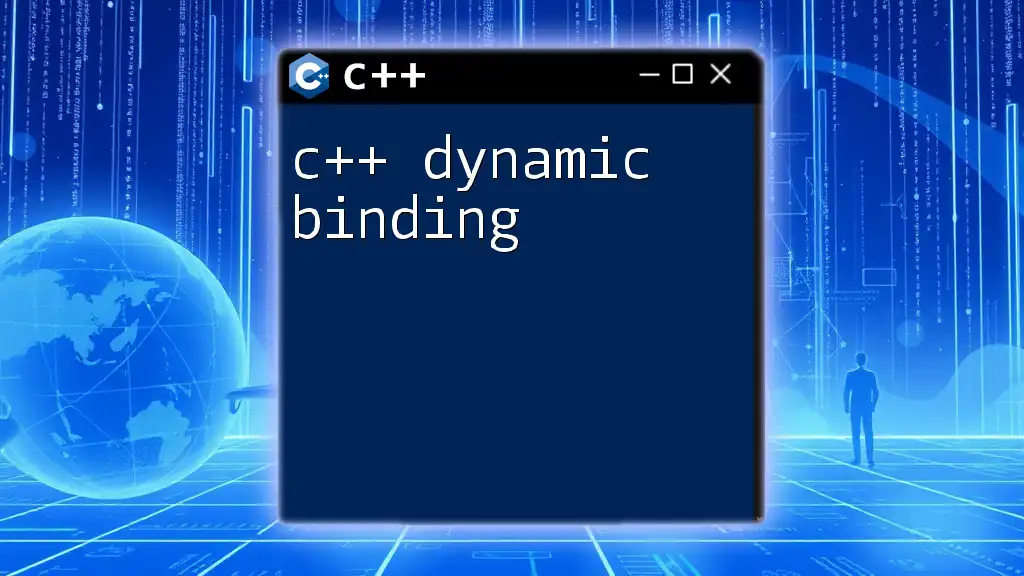
Best Practices for Dynamic Memory Management
Avoiding Memory Leaks
To prevent memory-related issues when using dynamic allocations, it is critical to ensure that every `new` operation has a corresponding `delete` operation. Always keep track of pointers and make sure they are deallocated properly after their usage.
Smart Pointers as an Alternative
C++ offers smart pointers like `std::unique_ptr` and `std::shared_ptr`, which can help manage dynamic memory automatically. These pointers clean up memory once they go out of scope, significantly reducing the risk of memory leaks. Using smart pointers is often a best practice in modern C++ programming.
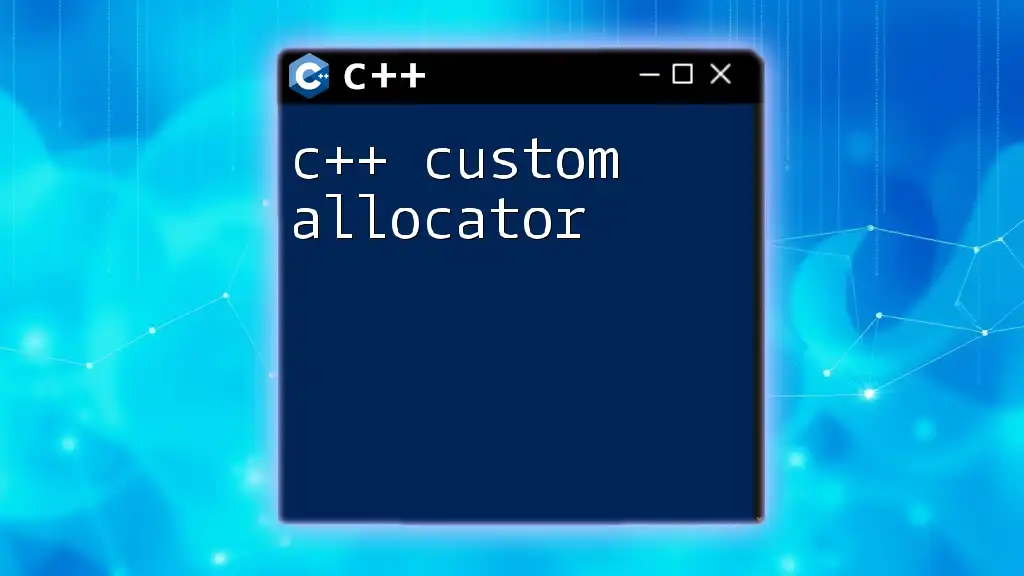
Common Errors in Dynamic Array Allocation
Memory Allocation Failures
One common error with dynamic arrays is memory allocation failure, which can occur if the system runs out of memory. Always ensure to check whether the pointer is `nullptr` after allocation:
if (arr == nullptr) {
std::cerr << "Memory allocation failed!" << std::endl;
}
Pointer Mismanagement
Improper handling of pointers with dynamic arrays can lead to undefined behavior. Avoid using deleted pointers or failing to deallocate memory, as this can lead to program crashes and unpredictable errors.
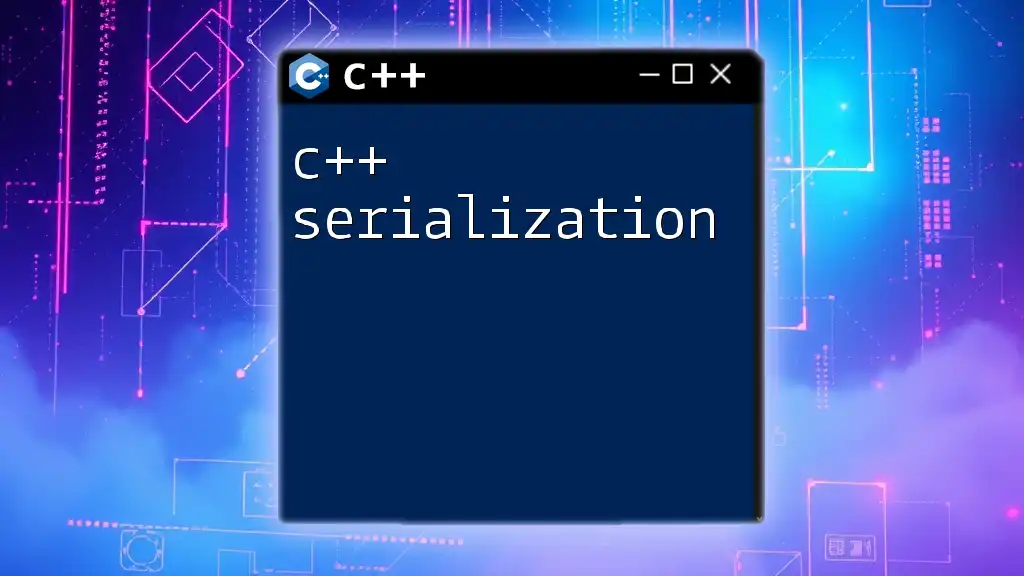
Conclusion
Understanding C++ dynamic allocation arrays is essential for effective memory management and program performance. By enabling the flexibility to allocate memory at runtime, dynamic arrays empower developers to create more adaptable and efficient code. Practicing with examples and employing best practices will solidify your knowledge in using dynamically allocated arrays efficiently.
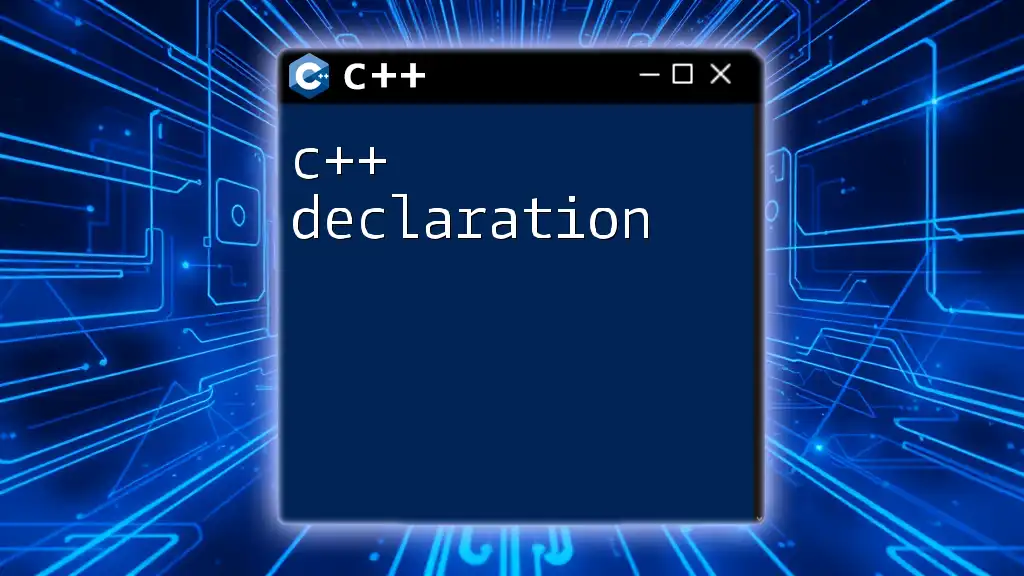
Additional Resources
For readers keen on expanding their knowledge on C++ dynamic memory management, consider exploring various programming books, online courses, and forums that focus on advanced C++ practices. Engaging with community resources can significantly enhance your understanding and proficiency.
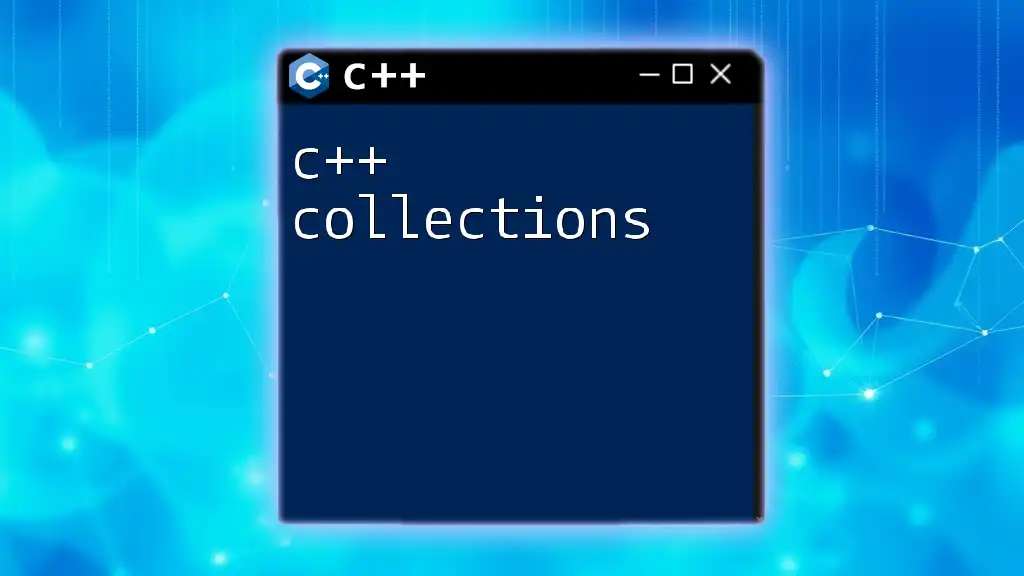
Frequently Asked Questions (FAQs)
What is the difference between new and malloc in C++?
While both `new` and `malloc` allocate memory, `new` also initializes the memory and calls constructors for objects, making it suitable for C++. In contrast, `malloc` allocates raw memory without initialization.
Can I use a dynamically allocated array without deallocation?
Using a dynamically allocated array without deallocation can lead to memory leaks, which can degrade performance over time.
How can I resize a dynamically allocated array?
To resize a dynamic array, you would typically allocate a new array of the desired size, copy the contents from the old array to the new one, and then deallocate the old array. This is often where smart pointers can help with management.
By following the guidance in this article, you’ll become proficient in working with C++ dynamic allocation arrays, allowing for more efficient programming practices.