Dynamic memory allocation in C++ allows developers to allocate memory during runtime using the `new` keyword, enabling the creation of variables and arrays whose size is determined at execution time.
Here’s a simple code snippet demonstrating dynamic memory allocation for an integer array:
#include <iostream>
int main() {
int* arr = new int[5]; // Allocate an array of 5 integers
for (int i = 0; i < 5; ++i) {
arr[i] = i * 10; // Initialize the array
}
for (int i = 0; i < 5; ++i) {
std::cout << arr[i] << " "; // Output the array values
}
delete[] arr; // Free the allocated memory
return 0;
}
What is Dynamic Memory Allocation?
Dynamic memory allocation refers to the process of allocating memory at runtime as opposed to compile time. In C++, this is done using operators such as `new` and `delete`. This approach allows programmers to create variables that can grow or shrink in size, providing far greater flexibility when managing memory.
The importance of dynamic memory management in C++ cannot be overstated—it allows for more efficient use of memory, as you can allocate exactly the amount of memory you need and release it when it is no longer in use.
Differences Between Static and Dynamic Memory Allocation
In static memory allocation, the size of the data structure must be determined at compile time, and the memory is allocated on the stack. In contrast, dynamic memory allocation occurs in the heap, where memory is allocated at runtime.
-
Static Memory Allocation:
- Memory is defined at compile time.
- Limited flexibility and possible wasted space.
-
Dynamic Memory Allocation:
- Memory allocation occurs at runtime.
- Provides flexibility and optimal memory usage.
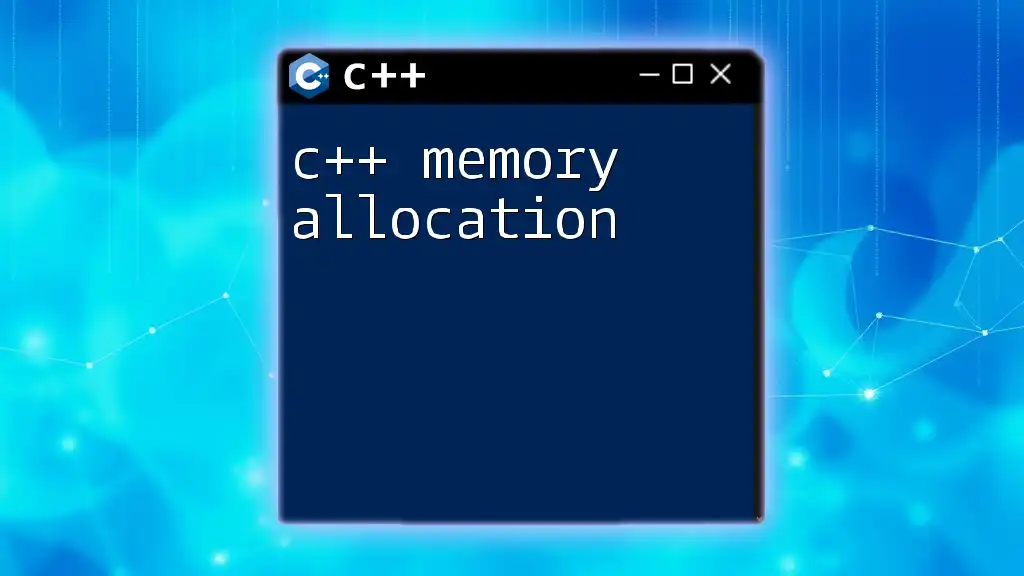
Why Use Dynamic Memory Allocation?
Dynamic memory allocation offers several benefits:
- Flexibility: You can allocate memory based on the actual needs of your program, allowing for structures of varying sizes.
- Efficient Memory Use: Memory can be allocated and deallocated as required, ensuring that you use only what you need.
- Complex Data Structures: It facilitates the creation of complex data structures such as linked lists, trees, and graphs, which require dynamically sized nodes.
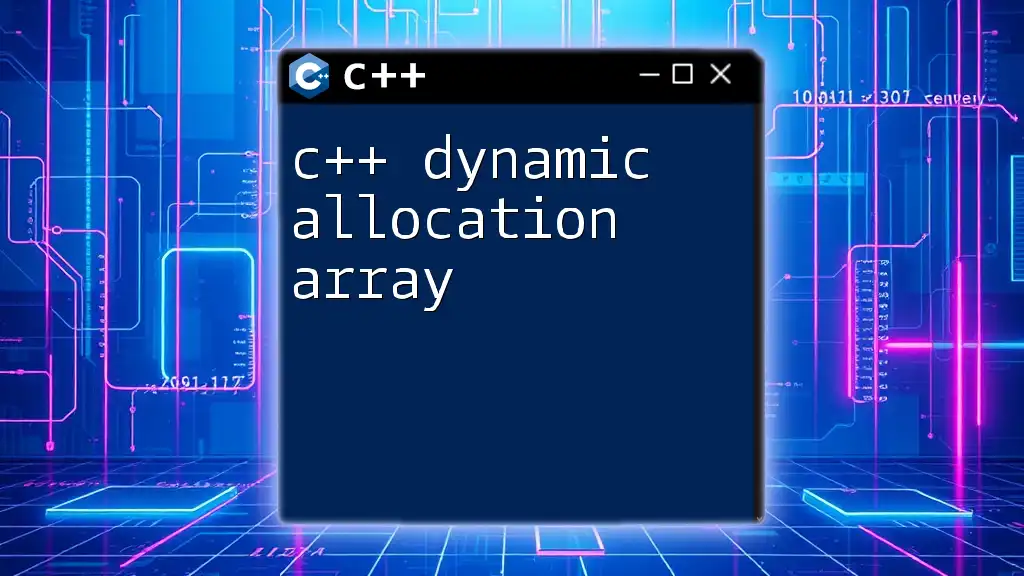
Understanding Memory Management in C++
The Memory Model
C++ utilizes a memory model that consists mainly of the stack and heap.
- Stack: Automatically managed memory that is used for static memory allocation. It has a limited size and is faster to allocate and deallocate than heap memory.
- Heap: Memory that is manually managed by the programmer. It is more flexible and can grow as needed, but it requires careful management to avoid memory leaks.
How Dynamic Memory Works
Pointers play a critical role in dynamic memory management. A pointer is a variable that stores the address of another variable.
When you allocate memory dynamically using the `new` operator, it returns a pointer to the beginning of the allocated memory block. This pointer can then be used to access and manipulate the memory.
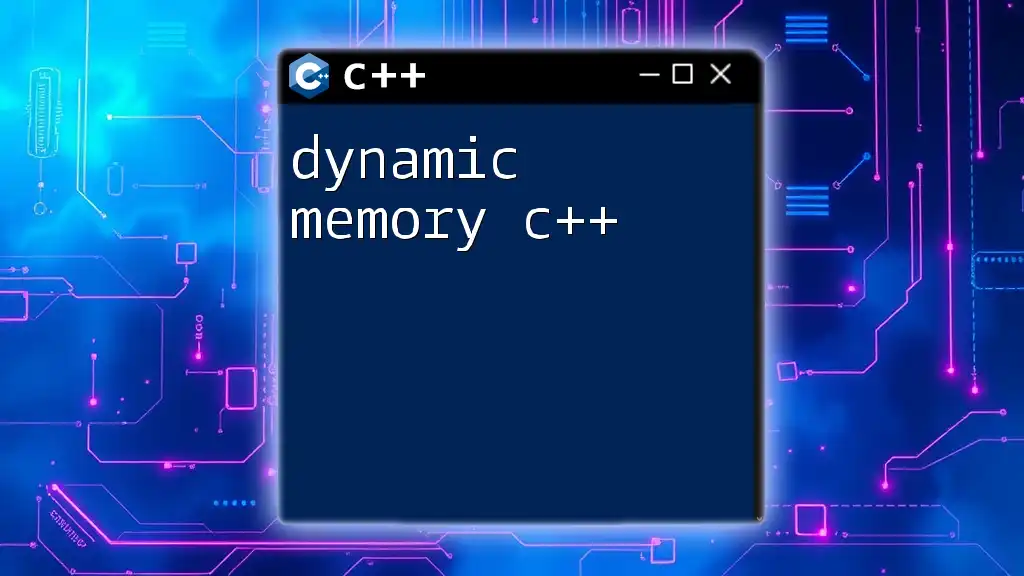
Methods of Dynamic Memory Allocation in C++
Using the `new` Operator
Dynamic memory allocation in C++ is primarily done through the `new` operator. This operator allocates memory on the heap.
Allocating Memory with `new`
You can allocate memory for both single objects and arrays:
int* singleInt = new int; // Allocates memory for a single integer
int* arrayInt = new int[10]; // Allocates memory for an array of 10 integers
Explanation: In the first line, `new int` allocates enough memory to store a single integer and returns a pointer to the allocated memory. In the second line, `new int[10]` allocates memory for an array of 10 integers.
Using the `delete` Operator
After allocating memory, it is crucial to deallocate it using the `delete` operator to avoid memory leaks.
Deallocating Memory with `delete`
delete singleInt; // Deleting a single integer
delete[] arrayInt; // Deleting an array of integers
Explanation: The `delete` operator releases the memory allocated for a single object, while `delete[]` is used for arrays. Failing to deallocate memory can lead to memory leaks, where the program consumes more memory over time than it actually needs.
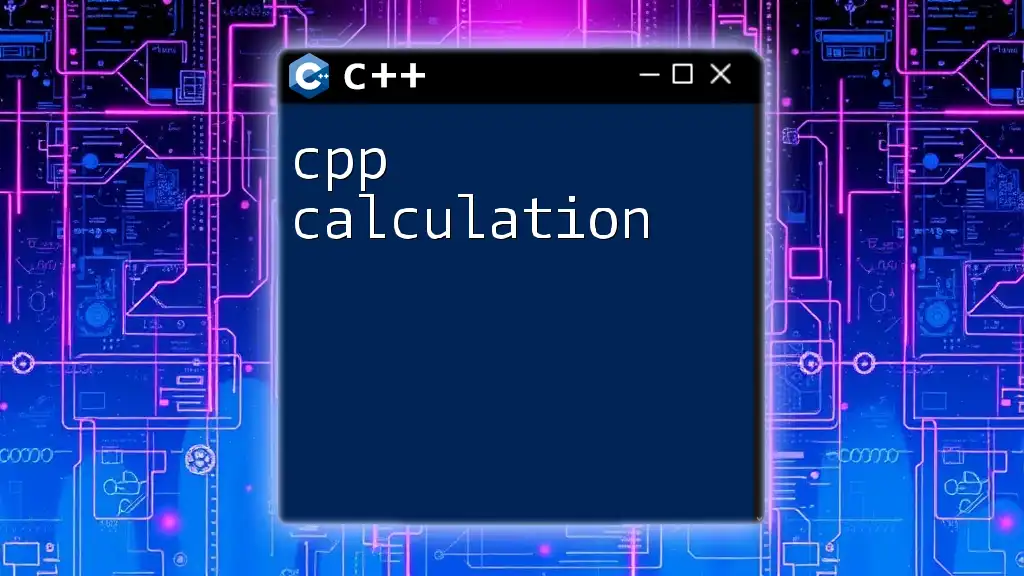
Advanced Dynamic Memory Allocation Techniques
Smart Pointers in C++
Smart pointers provide an alternative to raw pointers and help manage memory automatically. They significantly reduce the risks of memory leaks and dangling pointers.
Overview of Different Smart Pointers
- `std::unique_ptr`: A smart pointer that maintains exclusive ownership of the object it points to. Once a `unique_ptr` goes out of scope, its destructor releases the owned memory.
#include <memory>
std::unique_ptr<int> uniqueInt = std::make_unique<int>(5); // Allocates memory for an integer
- `std::shared_ptr`: A smart pointer that allows multiple pointers to own the same object. The memory is deallocated when no `shared_ptr` points to it anymore.
#include <memory>
std::shared_ptr<int> sharedInt = std::make_shared<int>(10); // Allocates memory for an integer
- `std::weak_ptr`: A smart pointer that does not affect the reference count of a `shared_ptr`. It is used to break circular references.
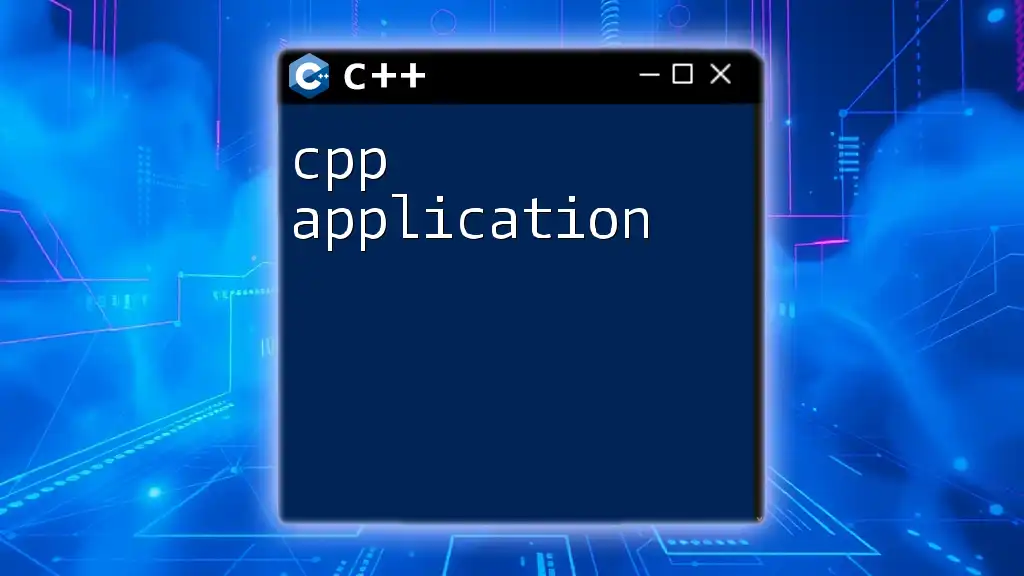
Common Issues with Dynamic Memory Allocation
Memory Leaks
A memory leak occurs when you allocate memory but fail to release it. This can lead to gradual increases in memory usage, ultimately slowing down or crashing your application.
To avoid memory leaks, always ensure that you paired each `new` operator with a corresponding `delete` operator.
Dangling Pointers
A dangling pointer is one that points to memory that has already been deallocated. Using a dangling pointer can lead to undefined behavior.
Tip: After freeing memory, set the pointer to `nullptr` to avoid dereferencing it.
Double Deletion
Double deletion occurs when you attempt to delete the same memory twice. This can lead to runtime errors or unpredictable behavior.
To prevent this, always ensure that pointers are not deleted more than once and consider using smart pointers to manage memory automatically.
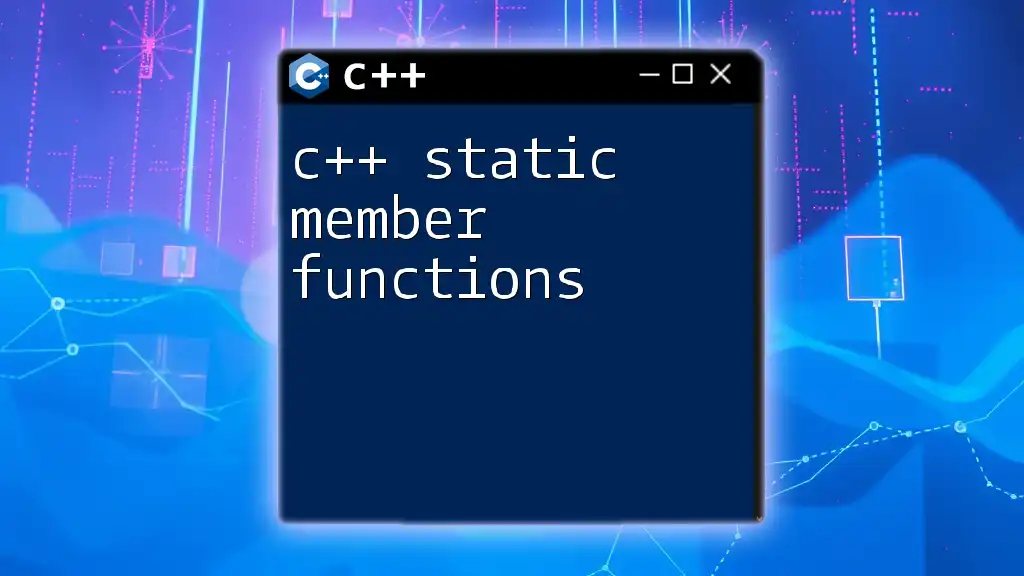
Effective Debugging Techniques for Dynamic Memory Issues
Using appropriate debugging tools can help identify dynamic memory problems.
Using Tools to Manage Dynamic Memory
- Valgrind: A powerful tool for detecting memory leaks, memory corruption, and invalid memory usage. It provides detailed reports to help you identify issues.
- AddressSanitizer: A fast memory error detector that can be used with GCC and Clang to check for memory errors in real-time.
These tools can help you write more robust and efficient C++ code by catching memory-related errors early in the development process.
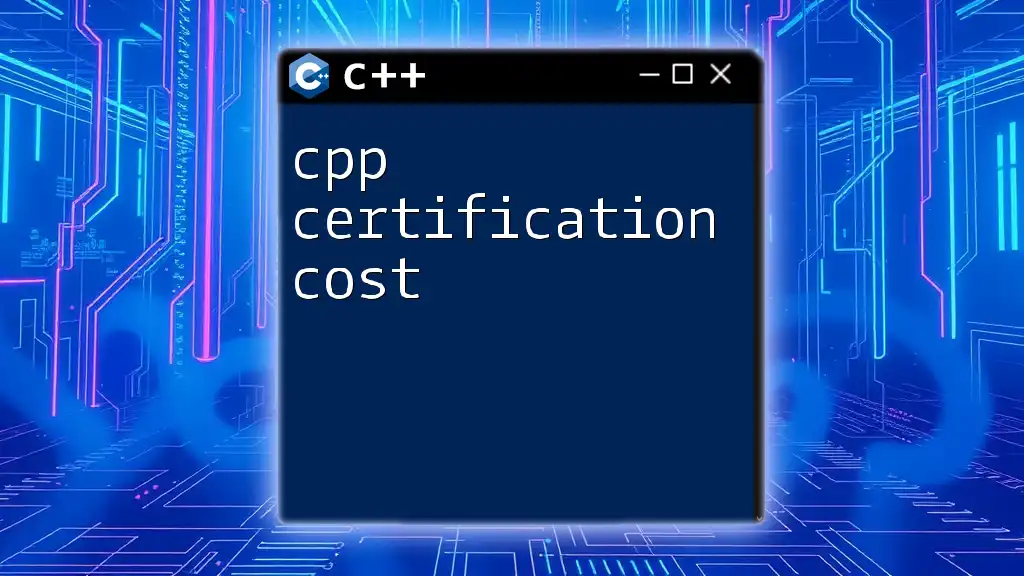
Conclusion
Understanding C++ dynamic memory allocation is crucial for effective programming in the language. By mastering the concepts of dynamic memory management, utilizing smart pointers, and avoiding common pitfalls, you can write safer and more efficient C++ applications.
Further Learning Resources
Consider exploring various books, tutorials, and courses that delve deeper into dynamic memory management and best practices in C++. This foundational knowledge will serve you well as you advance in C++ programming.
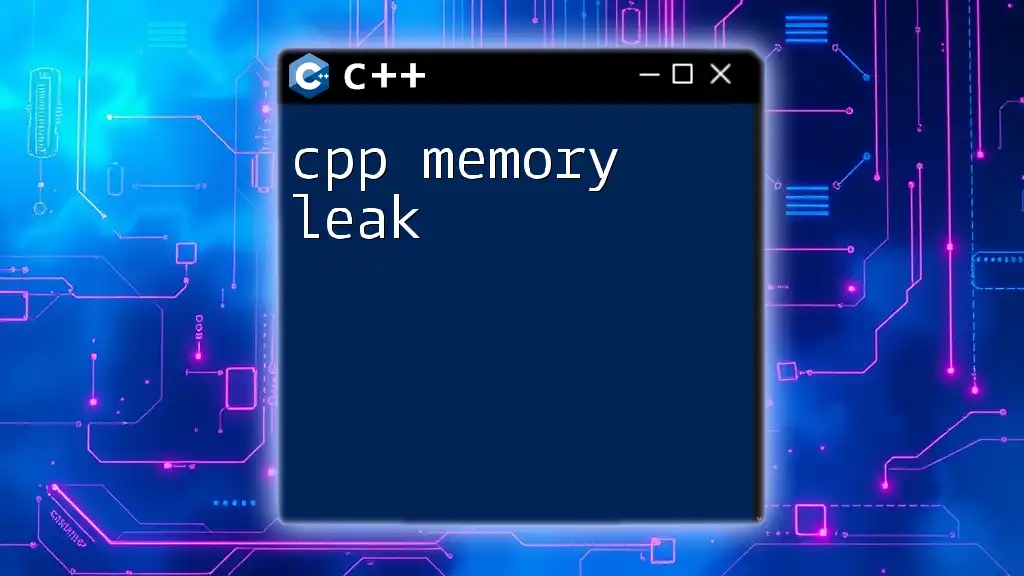
Call to Action
Join our community of learners to gain deeper insights into C++ and improve your skills in dynamic memory allocation and more!