C++ binary operations allow you to perform mathematical and logical operations on two operands using various operators like addition, subtraction, and bitwise operations.
Here's a simple example demonstrating addition and bitwise AND:
#include <iostream>
int main() {
int a = 5; // 0101 in binary
int b = 3; // 0011 in binary
// Addition
int sum = a + b; // 5 + 3 = 8
std::cout << "Sum: " << sum << std::endl;
// Bitwise AND
int bitwiseAnd = a & b; // 0101 & 0011 = 0001 (1 in decimal)
std::cout << "Bitwise AND: " << bitwiseAnd << std::endl;
return 0;
}
What are Binary Operators in C++?
Binary operators in C++ are operators that operate on two operands. These operators take two values and perform operations, returning a result. For example, when you use the addition operator (`+`), it adds two numbers together. Understanding binary operators is crucial for writing effective and efficient C++ code, as these operations form the backbone of any arithmetic or logic-driven task within a program.
While binary operators deal with two operands, there are unary operators that only require one. Knowing the difference is essential for proper coding practice and facilitates a better comprehension of how expressions are evaluated in C++.
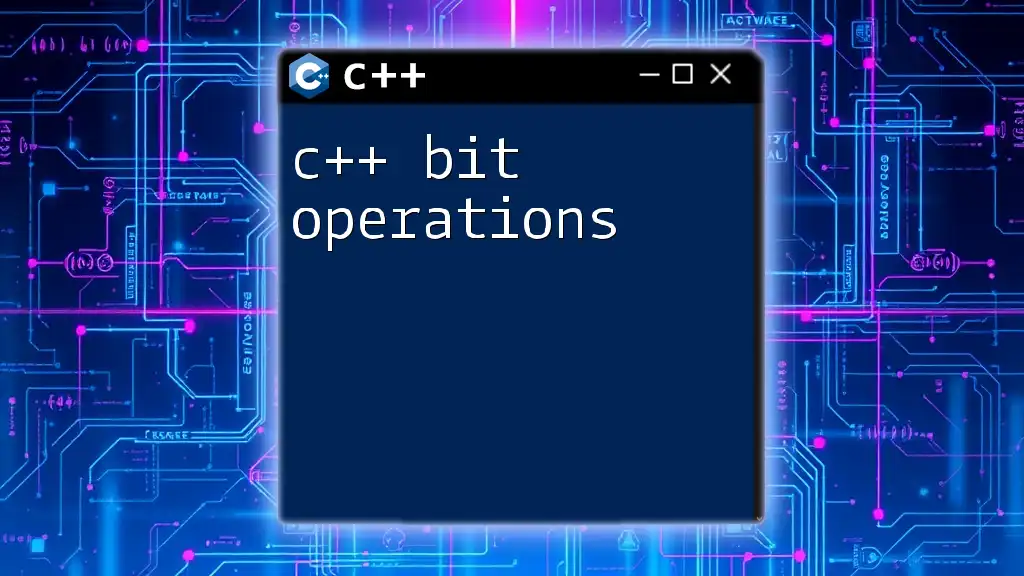
Types of C++ Binary Operators
Arithmetic Binary Operators
Arithmetic binary operators are the most commonly used operators. They allow you to perform mathematical operations such as addition, subtraction, multiplication, and division. Here are the primary arithmetic operators:
- Addition (`+`): This operator sums two values.
- Subtraction (`-`): This operator subtracts the second value from the first.
- Multiplication (`*`): This operator multiplies two values.
- Division (`/`): This operator divides the first value by the second.
- Modulus (`%`): This operator returns the remainder of the division.
Examples:
int a = 10, b = 5;
int addition = a + b; // 15
int subtraction = a - b; // 5
int multiplication = a * b; // 50
int division = a / b; // 2
int modulus = a % b; // 0
Relational Binary Operators
Relational binary operators are used to compare two values, allowing you to determine their relationship to one another. They yield boolean values: `true` or `false`. The common relational operators include:
- Equal to (`==`): Checks if two values are equal.
- Not equal to (`!=`): Checks if two values are not equal.
- Greater than (`>`): Checks if the left operand is greater than the right.
- Less than (`<`): Checks if the left operand is less than the right.
- Greater than or equal to (`>=`): Checks if the left operand is greater than or equal to the right.
- Less than or equal to (`<=`): Checks if the left operand is less than or equal to the right.
Examples:
bool isEqual = (a == b); // false
bool isGreater = (a > b); // true
Logical Binary Operators
Logical binary operators are used to perform logical operations on boolean inputs. The outcomes of these operations also yield boolean values, making them essential in decision-making processes within a program. The main logical operators are:
- Logical AND (`&&`): Returns `true` if both operands are true.
- Logical OR (`||`): Returns `true` if at least one of the operands is true.
Examples:
bool andResult = (a > b) && (a < 20); // true
bool orResult = (a < b) || (a > 0); // true
Bitwise Binary Operators
Bitwise binary operators manipulate the binary representations of integers. They are vital for tasks involving lower-level programming, hardware manipulation, and performance optimization. The most common bitwise operators include:
- Bitwise AND (`&`): Compares each bit, returning 1 if both bits are 1.
- Bitwise OR (`|`): Compares each bit, returning 1 if at least one of the bits is 1.
- Bitwise XOR (`^`): Compares each bit, returning 1 if the bits are different.
- Left shift (`<<`): Shifts bits to the left, adding zeros at the right.
- Right shift (`>>`): Shifts bits to the right, discarding the bits that go off the end.
Examples:
int x = 5; // 0101 in binary
int y = 3; // 0011 in binary
int bitwiseAnd = x & y; // 0001 -> 1
int bitwiseOr = x | y; // 0111 -> 7
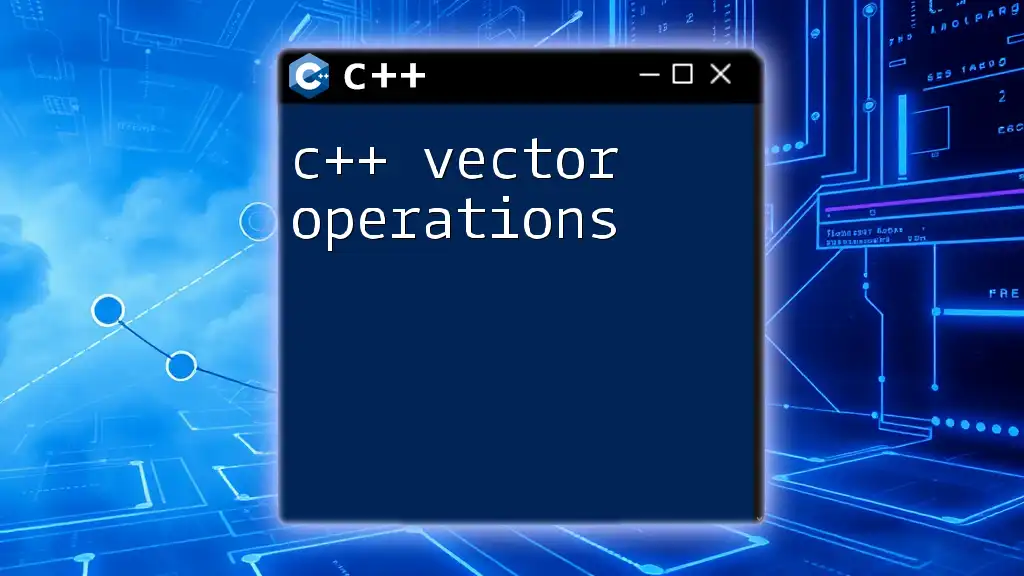
Precedence and Associativity of Binary Operators
Operator precedence refers to the rules governing the order in which operators are evaluated in an expression. The higher the precedence, the earlier the operator is evaluated. Associativity determines the order of evaluation in expressions with operators of the same precedence.
For instance, the multiplication operator (`*`) has a higher precedence than the addition operator (`+`). Therefore, in an expression like `10 + 5 * 2`, the multiplication will be performed first, resulting in `20` instead of `30`.
Formal understanding of precedence and associativity helps to avoid syntax errors and logical bugs in C++ programming.
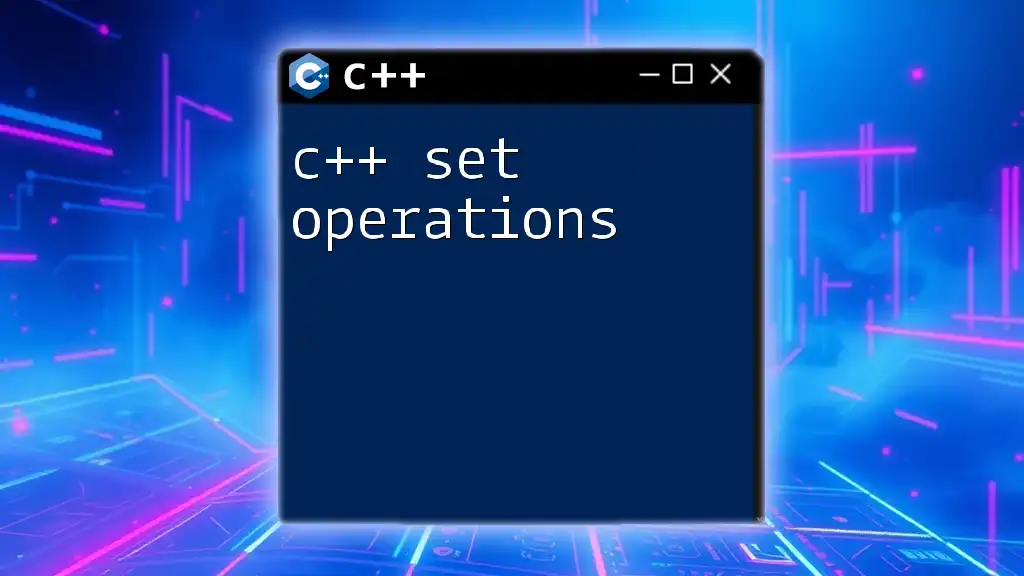
Common Mistakes with Binary Operators
Newcomers to C++ often face common pitfalls when using binary operators. Recognizing these can save time and frustration:
- Confusing `=` with `==`: The assignment operator (`=`) is not the same as the equality operator (`==`). Mistakenly using one for the other can lead to erroneous results.
- Misuse of Integer Division: Remember that dividing two integers in C++ yields an integer result—any fractional part is discarded.
- Bitwise versus Logical Operations: Using bitwise operators instead of logical ones can result in unexpected behavior, especially in conditional statements.
Examples and Corrections:
bool condition = (a = b); // Incorrect usage for comparison; uses assignment instead
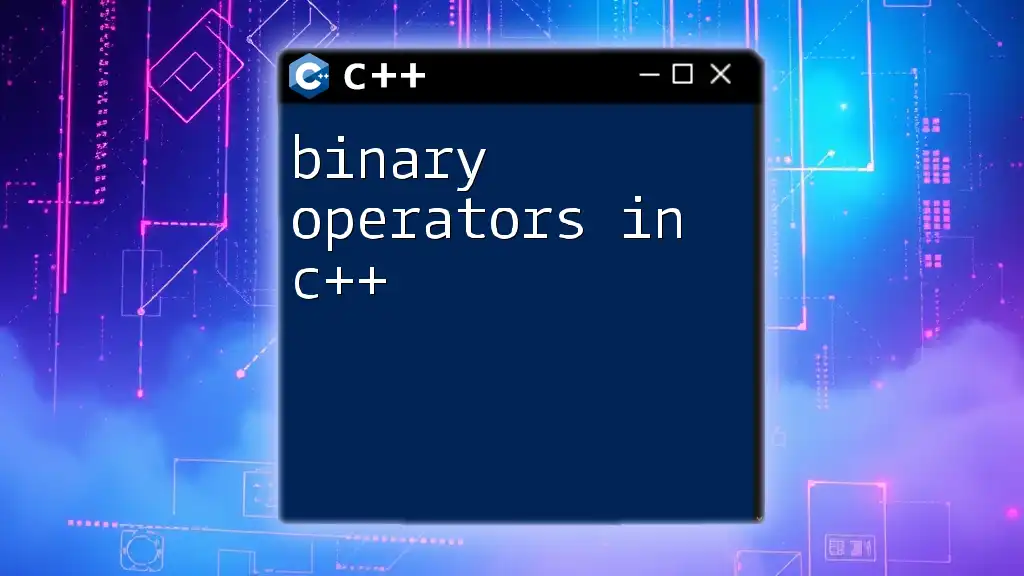
Best Practices for Using C++ Binary Operators
To write clear and maintainable code with binary operators, consider these best practices:
- Use Parentheses for Clarity: Don’t hesitate to use parentheses to explicitly define the order of operations. This reduces the chance of logical errors caused by operator precedence.
- Understand the Data Types Involved: Mistakes can arise when mixing data types, especially with integers and floating-point numbers.
- Test Edge Cases: Always test your code with edge cases to ensure your binary operations behave as expected.
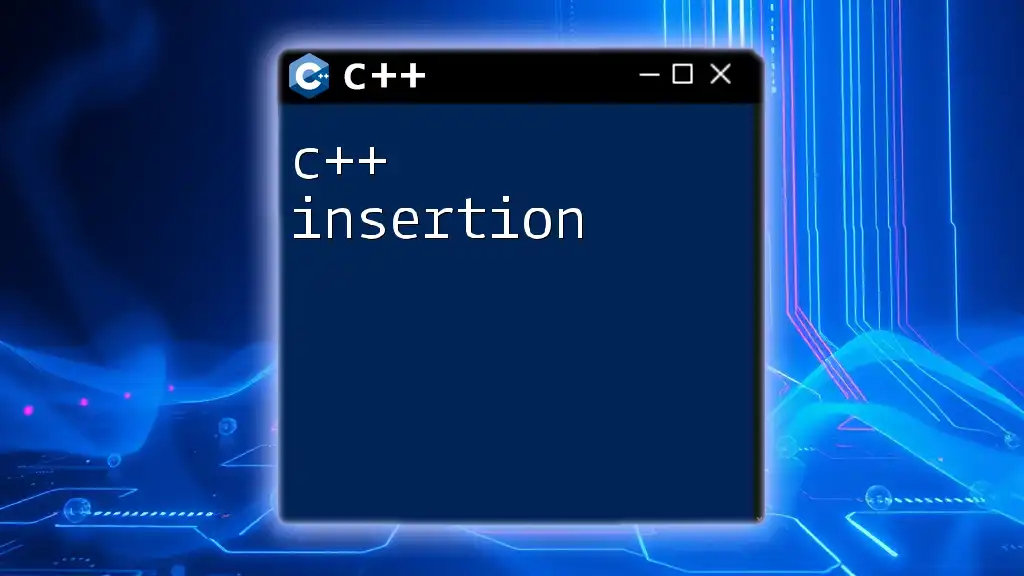
Conclusion
Understanding C++ binary operations is essential for effective programming. The diverse range of binary operators allows for flexible and efficient expression of logic and arithmetic. Familiarity with these operators, their precedences, and common mistakes will significantly enhance your programming skills and enable you to tackle C++ coding challenges more confidently. Continuous practice with examples will help solidify this knowledge, paving the way for more complex programming concepts.