The C++ div operator (represented by the `/` symbol) performs integer division, returning the quotient of two integers, discarding any remainder.
Here's a code snippet demonstrating its use:
#include <iostream>
int main() {
int a = 10;
int b = 3;
int result = a / b; // result will be 3
std::cout << "The result of " << a << " / " << b << " is " << result << std::endl;
return 0;
}
Understanding Division in Programming
Division is one of the fundamental operations in programming, enabling developers to perform mathematical calculations and manipulate numeric data. Understanding how to effectively use division in C++ is essential for building robust applications, as it is often used in algorithms, formulas, and data processing tasks.
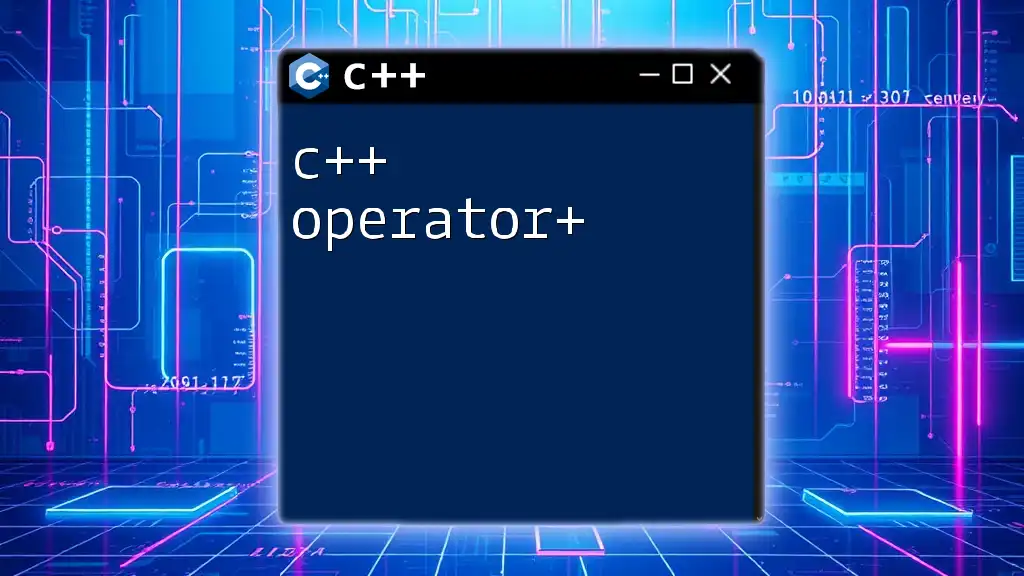
What is the Div Operator?
In C++, the div operator is represented by the forward slash (`/`). It is used to divide two numbers and return the result. This operator plays a crucial role in arithmetic operations within C++ code. It is important to note how the division operator behaves with various data types, as the outcomes can significantly differ between integers and floating-point numbers.
![Unlocking the C++ [] Operator: A Step-by-Step Guide](/images/posts/c/cpp-operator.webp)
C++ Div Operator Syntax
Basic Syntax of the Div Operator
The syntax for using the div operator is straightforward. You simply use the forward slash (`/`) between two operands:
int result = a / b;
In the example above, `a` and `b` are variables representing numeric values. The operation divides `a` by `b` and stores the quotient in `result`.
Advanced Syntax: Div in C++
When utilizing division in C++, it is essential to understand how different types of operands interact with the div operator. The operator can work with integers, floats, and doubles, leading to varied outcomes. For instance, consider the following code snippet:
int a = 5, b = 2;
double result1 = static_cast<double>(a) / b; // Outputs 2.5
In this case, we explicitly cast `a` to a `double` before division. This prevents truncation and ensures that the result is a floating-point number.
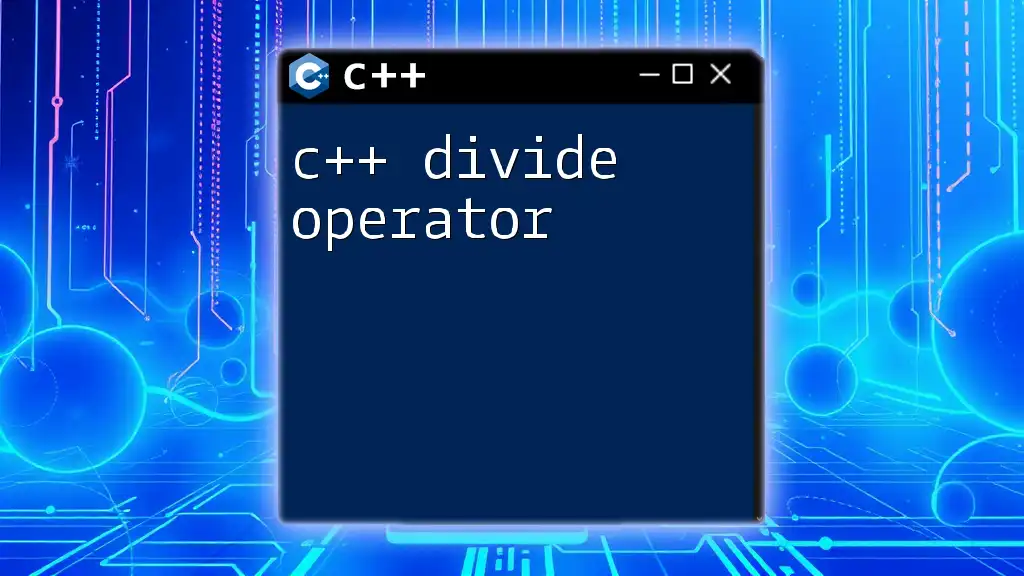
Types of Division in C++
Integer Division
One of the most notable behaviors of the div operator in C++ is its handling of integer division. When both operands are integers, C++ performs integer division, which truncates the decimal part. For example:
int a = 7, b = 2;
int result = a / b; // Outputs 3, not 3.5
In this example, though the mathematical division yields 3.5, the result in C++ is truncated to 3 due to integer division.
Floating-Point Division
Conversely, when using floating-point numbers, the division operator yields a more precise result. When at least one operand is a floating-point number, C++ performs floating-point division. Consider the following example:
double a = 7.0, b = 2.0;
double result = a / b; // Outputs 3.5
Here, both operands are doubles, and the division result maintains the decimal, reflecting the true mathematical outcome.
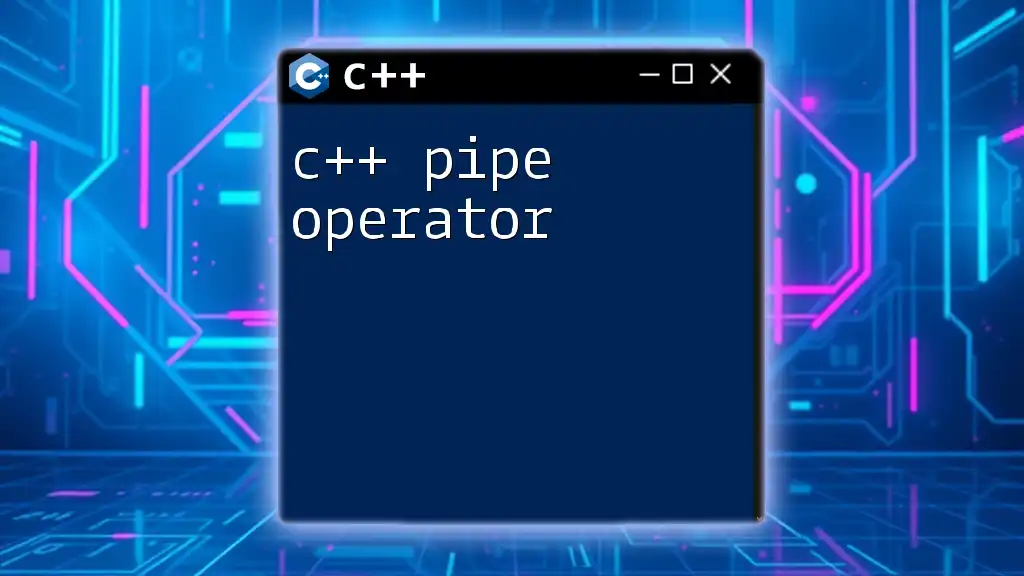
Handling Division by Zero
Importance of Zero Division Check
One critical aspect of using the div operator in C++ is the risk associated with division by zero. Attempting to divide by zero leads to runtime errors, which can crash the program. Therefore, it is vital to implement checks before performing division operations.
Implementing Safe Division
To safely handle dividing by zero, you can use a simple if-else statement. Here's an example:
if (b != 0) {
int result = a / b;
} else {
std::cout << "Division by zero error!" << std::endl;
}
In this code snippet, we check whether `b` is zero before performing the division. If it is zero, a warning message is displayed instead of attempting the division.
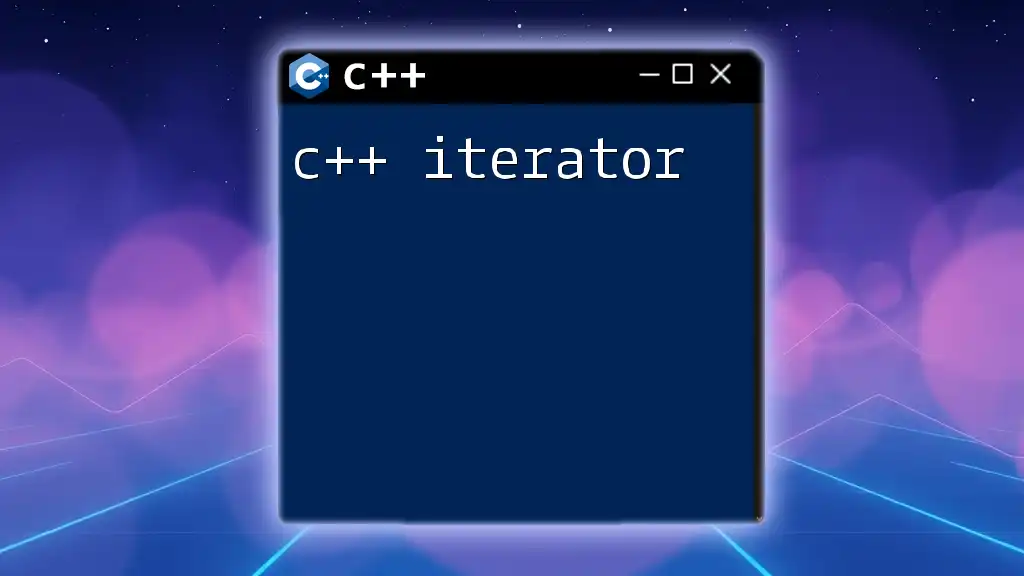
Dividing with Different Data Types
Using the Div Operator with Integers, Floats, and Doubles
Understanding how the div operator behaves with different data types is essential for accurate calculations.
int a = 10, b = 4;
double c = 10.0, d = 4.0;
int intResult = a / b; // Outputs 2
double floatResult = c / d; // Outputs 2.5
In the above code, integer division results in 2, whereas the floating-point division accurately provides a result of 2.5.
Implicit and Explicit Type Conversion
While dividing, C++ automatically promotes smaller types to match the type of larger operands. This behavior can sometimes lead to unexpected results if not understood properly.
float f = 10 / 4; // Outputs 2.0 due to integer division
float preciseResult = 10.0 / 4; // Outputs 2.5
In the first instance, since both 10 and 4 are integers, integer division occurs and truncates the result to 2. In the second case, explicit floating-point representation ensures that the division yields the expected decimal.
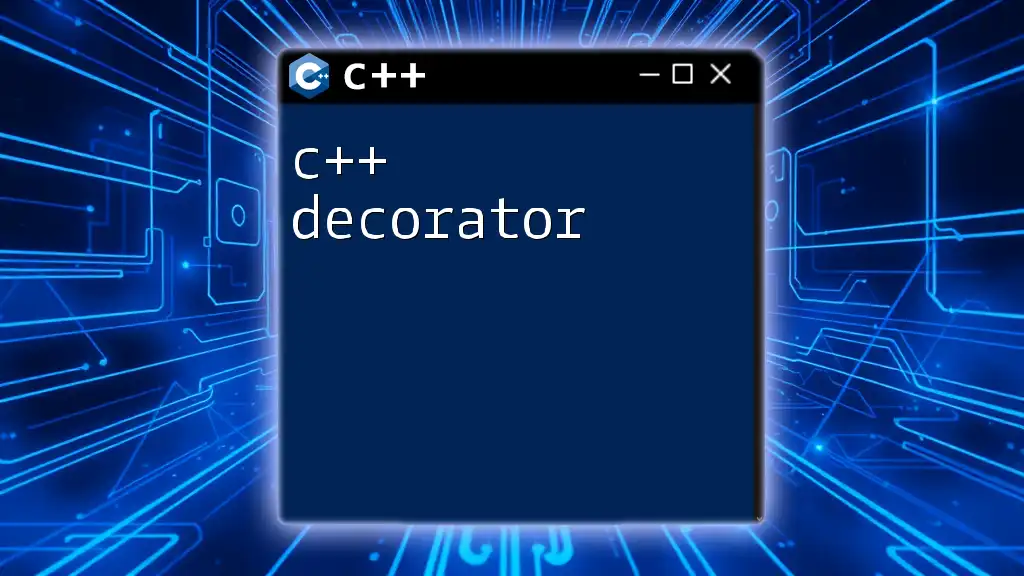
Practical Examples of the Div Operator in C++
Real-World Applications of Division
Division is not only a basic arithmetic operation; it is often integral to real-world applications, such as calculating averages, scaling factors, and ratios in data analysis or financial calculations. The div operator seamlessly integrates into these programming processes.
Sample C++ Program Demonstrating the Div Operator
Let’s take a look at a simple C++ program that demonstrates division:
#include <iostream>
using namespace std;
int main() {
int num1, num2, result;
cout << "Enter two integers: ";
cin >> num1 >> num2;
if (num2 != 0) {
result = num1 / num2;
cout << "The result is: " << result << endl;
} else {
cout << "Error: Division by zero!" << endl;
}
return 0;
}
In this program, the user inputs two integers for division. The program checks for division by zero before performing the calculation, ensuring safe execution.
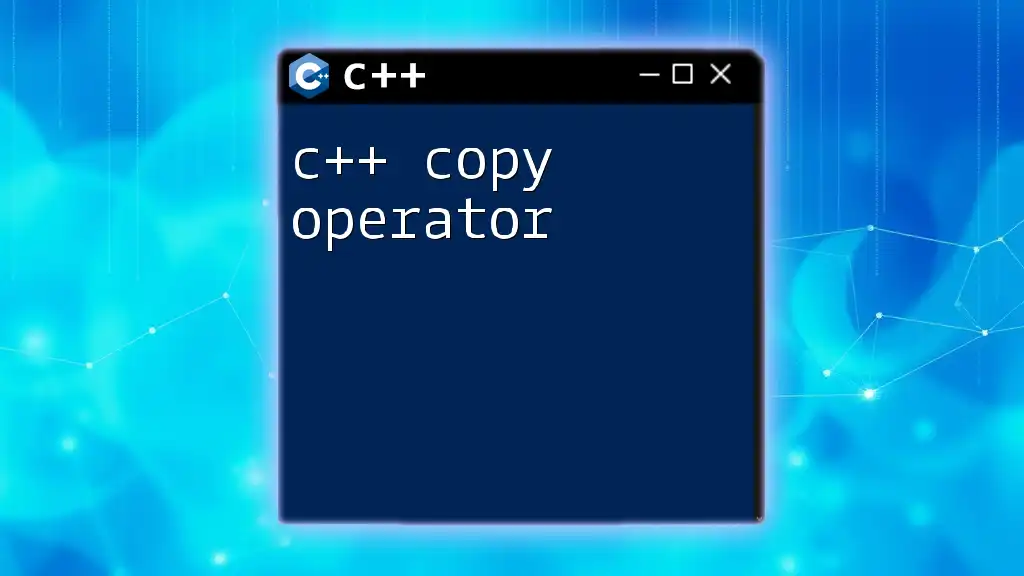
Best Practices When Using the Div Operator
Code Readability and Maintenance
To foster code readability, write clear and concise division expressions. Using well-named variables and structuring your division operations logically can improve overall code maintenance.
Avoiding Common Pitfalls
Common misconceptions surrounding the div operator include assuming that division will always yield a floating-point result. It is crucial to be mindful of the data types being divided and to handle any potential errors, particularly with zero division.
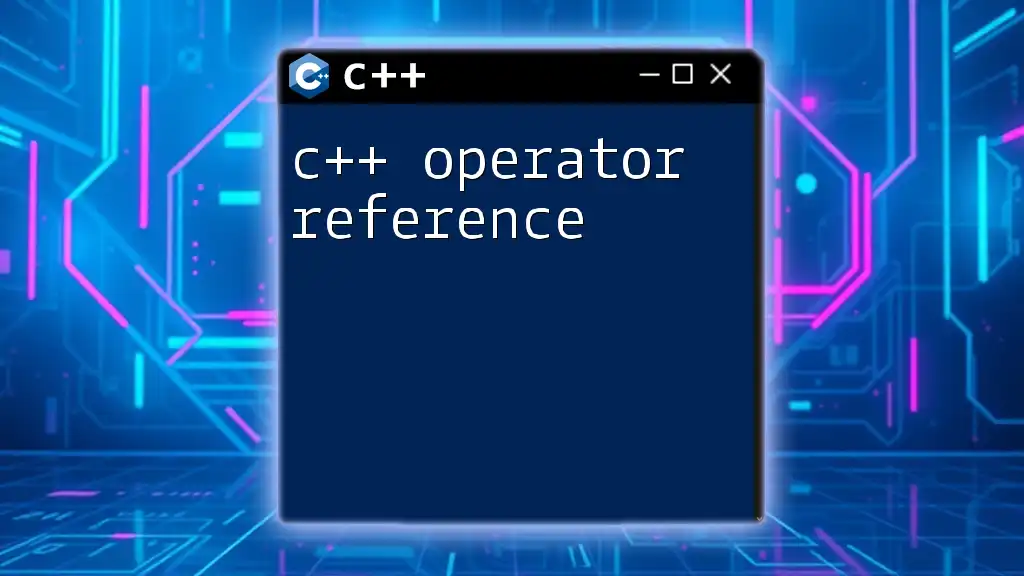
Conclusion
Understanding the C++ div operator is essential for effective programming, as it enables developers to perform vital mathematical operations accurately and efficiently. By grasping the nuances between integer and floating-point division, handling division by zero safely, and adhering to best programming practices, developers can confidently implement division across their applications. Happy coding!