The error "no operator matches these operands" in C++ typically occurs when you are trying to perform an operation with incompatible data types, such as adding an integer to a string.
Here's an example that illustrates this error:
#include <iostream>
#include <string>
int main() {
int number = 5;
std::string text = "Hello";
// This line will cause a "no operator matches these operands" error
std::string result = number + text;
std::cout << result << std::endl;
return 0;
}
Understanding Operator Overloading
What is Operator Overloading?
Operator overloading in C++ allows developers to define custom behavior for operators (+, -, *, etc.) when they are applied to user-defined types (classes and structures). This feature enhances the expressiveness of code, making it possible to write operations on user-defined types in a manner that feels natural and intuitive, similar to built-in types.
Benefits of Operator Overloading
One of the primary benefits of operator overloading is increased code readability. Instead of calling a method, one can simply use an operator symbol. For instance, instead of using `add(obj1, obj2)`, one can simply write `obj1 + obj2`. This allows developers to maintain a clear and concise syntax.
Another notable advantage is the enhanced functionality of user-defined types, enabling users to express complex operations in straightforward terms. Examples include mathematical operations for complex numbers or vector mathematics, where clarity and succinctness are paramount.
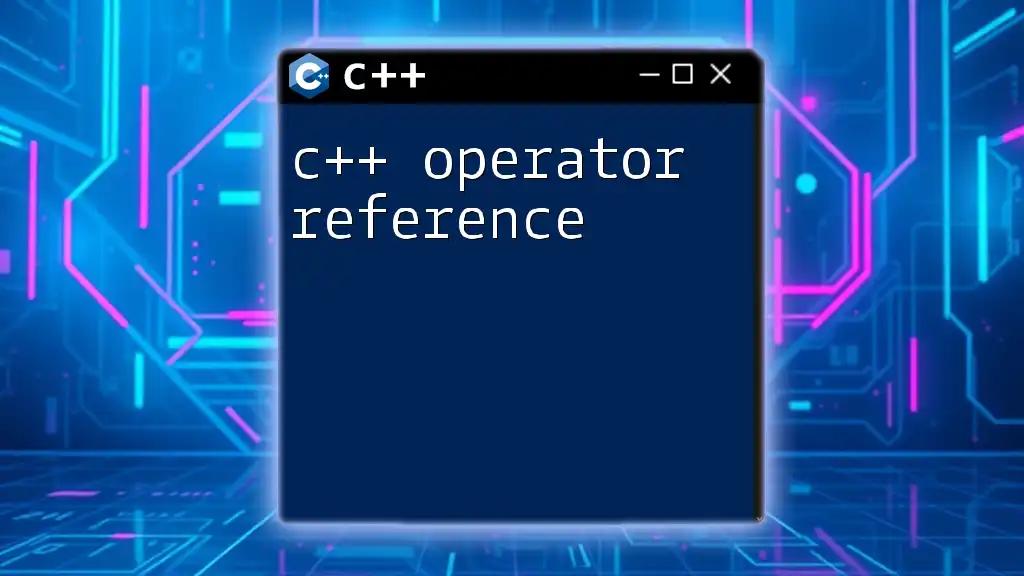
The Error: "No Operator Matches These Operands"
What Does It Mean?
When you encounter the error "no operator matches these operands," it generally indicates that an attempt has been made to use an operator on two operands where the operator has not been defined or overloaded for the given data types. In practical terms, it means that C++ does not know how to process that particular operation for those specific types.
Common Causes
Type Mismatch
A type mismatch occurs when the operands involved in an operation are of incompatible types. For example, if you're trying to add an integer to a floating-point number, C++ might struggle to resolve that operation if the data types are custom classes.
Here’s an example that triggers this error:
class MyClass {
// Some member variables and methods
};
MyClass obj1, obj2;
auto result = obj1 + obj2; // Error: no operator matches these operands
In this snippet, `MyClass` has no overloaded `+` operator defined, hence the compiler cannot match the operands.
Non-Overloaded Operators
Another common cause is the use of operators that are not overloaded by default. If a user-defined type is involved in an operation without a corresponding operator implemented, this error will arise.
Here’s an example of this issue:
class Point {
public:
int x, y;
Point(int a, int b) : x(a), y(b) {}
};
Point p1(1, 2), p2(3, 4);
// auto p3 = p1 + p2; // Error: no operator matches these operands
In this case, attempting to add two `Point` objects without an overloaded `+` operator leads to the stated error.
Troubleshooting Steps
Step 1: Checking Types
The first step in resolving the "C++ no operator matches these operands" error is to check the types of the operands involved in the operation. It’s crucial to be familiar with the data types being used. You can utilize the `typeid` operator in C++ to get information about the data types at runtime.
#include <iostream>
#include <typeinfo>
class MyClass {
// ...
};
int main() {
MyClass obj1, obj2;
std::cout << typeid(obj1).name() << "\n"; // Output the type of obj1
std::cout << typeid(obj2).name() << "\n"; // Output the type of obj2
}
Step 2: Implementing Operator Overloading
Once you identify the cause, the most effective way to resolve the issue is to implement operator overloading for the required operations. Here’s how you can overload operators:
For example, to overload the `+` operator for the `Point` class:
class Point {
public:
int x, y;
Point(int a, int b) : x(a), y(b) {}
Point operator+(const Point& other) {
return Point(this->x + other.x, this->y + other.y);
}
};
With this overloaded operator, you can now perform the addition of `Point` objects seamlessly, like so:
Point p1(1, 2), p2(3, 4);
Point p3 = p1 + p2; // Now this works!
Best Practices for Operator Overloading
Balancing Clarity and Functionality
While operator overloading can improve code clarity, it is vital to be judicious about its use. Operators should only be overloaded when they provide a clear benefit. Overloading operators in ways that deviate from their typical use can lead to confusion. Always ensure that overloaded operators maintain intuitive behavior.
Consistency
Another essential practice is maintaining consistency across overloaded operators. If you define an operator such as `+`, consider also overloading the corresponding `-` operator while keeping semantic meaning intact. Here’s an example:
Point operator-(const Point& other) {
return Point(this->x - other.x, this->y - other.y);
}
This consistency makes the operators more predictable for users of your classes, which is crucial for writing understandable and maintainable code.
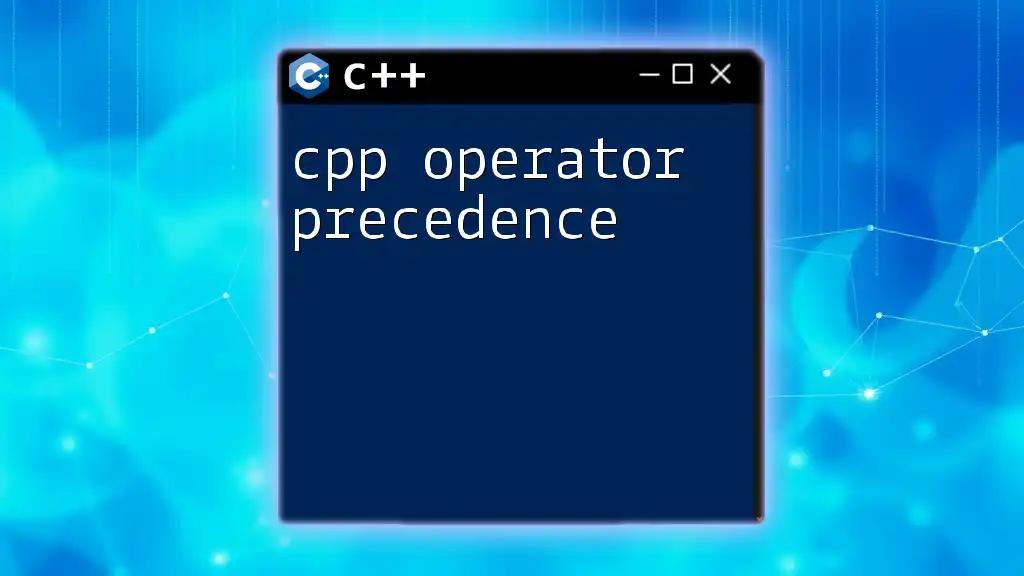
Common Mistakes Leading to "No Operator Matches These Operands"
Forgetting to Include a Header File
One common mistake that leads to the "no operator matches these operands" error is forgetting to include necessary header files that define the types you are working with, especially when using standard types or classes defined in libraries.
Using Incompatible Types
Another frequent pitfall is using incompatible types without realizing it. Always ensure that the types are compatible and that any necessary conversion is handled properly if dealing with user-defined types.

Conclusion
Understanding the nuances of operator overloading in C++ is crucial for developing robust applications. The error message "no operator matches these operands" serves as an important reminder of the complexities involved when using operators with user-defined types. By familiarizing yourself with the principles of operator overloading and what causes this error, you can enhance your C++ programming skills significantly.
Experimenting with operator overloading and following best practices will lead to clearer, more maintainable code. Remember to stay engaged with the C++ community, share your experiences, and seek guidance when needed. Happy coding!

Resources for Further Learning
For those looking to delve deeper into the topic, consider reading classic C++ texts or visiting community forums and websites that specialize in C++ programming. Engaging with videos, tutorials, and even hackathons can provide practical experience and foster valuable connections in your programming journey.