In C++, the "no match for operator" error occurs when the compiler cannot find a suitable operator overload for a given operation involving user-defined types or incompatible types.
Here's an example of how this error might occur:
#include <iostream>
class MyClass {
public:
int value;
MyClass(int v) : value(v) {}
};
// Attempting to add two MyClass objects without an overloaded operator+
MyClass obj1(10);
MyClass obj2(20);
MyClass result = obj1 + obj2; // Error: no match for 'operator+'
What are Operators in C++?
Operators are fundamental building blocks in programming, enabling developers to perform operations on variables and values. In C++, operators can be categorized into several groups:
- Arithmetic Operators: Perform basic mathematical operations (e.g., `+`, `-`, `*`, `/`).
- Relational Operators: Used to compare values (e.g., `<`, `>`, `==`, `!=`).
- Logical Operators: Facilitate logical operations (e.g., `&&`, `||`, `!`).
- Bitwise Operators: Operate on bits and perform bit-level operations (e.g., `&`, `|`, `^`, `~`).
- Assignment Operators: Assign values to variables (e.g., `=`, `+=`, `-=`).
Understanding these operators is crucial for creating functional and efficient C++ applications, as they significantly impact program logic and flow.
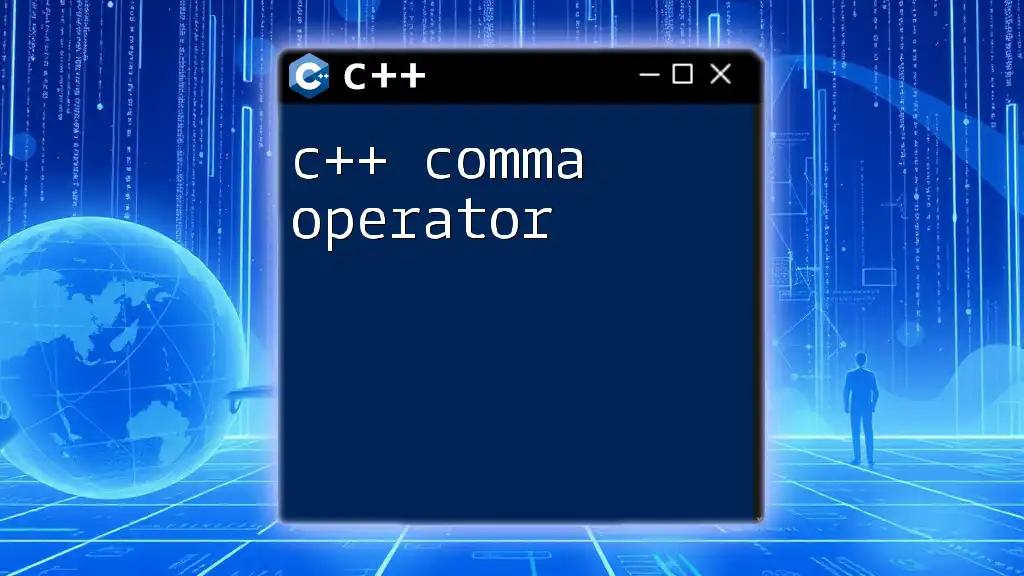
Understanding the "No Match for Operator" Issue
What Does "No Match for Operator" Mean?
The "No match for operator" error is a compilation issue that arises when the compiler cannot find a suitable operator for the given operand types. This typically happens during operations involving overloaded operators or custom classes. When the requested operation cannot be resolved, developers encounter this frustrating error.
Common Causes of "No Match for Operator"
Type Mismatches
One of the primary causes of encountering the c++ no match for operator error is mismatched data types. When you try to use an operator with two operands of incompatible types, the compiler cannot deduce how to perform the operation.
For example:
#include <iostream>
struct MyStruct {
int value;
};
int main() {
MyStruct a;
MyStruct b;
a.value = 10;
b.value = 20;
// This will generate a "no match for operator+" error
std::cout << (a + b) << std::endl; // Error: no match for 'operator+'
return 0;
}
In this snippet, trying to add two `MyStruct` instances leads to a compilation error because there is no defined operator `+` for `MyStruct`.
Missing Operator Overloads
Operator overloading is essential when working with custom types. If an operator is needed but hasn’t been defined for a specific type, the result is a no match for operator error.
Here's a simple example of a class missing operator overloads:
#include <iostream>
class Vector {
public:
int x, y;
Vector(int x, int y) : x(x), y(y) {}
};
int main() {
Vector v1(1, 2);
Vector v2(3, 4);
// This line will cause a "no match for operator+" error
Vector v3 = v1 + v2; // Error: no match for 'operator+'
return 0;
}
In this instance, without an overloaded `+`, there’s no way to add two `Vector` instances.
Conflicting Types
Conflicting types can also result in the c++ no match for operator issue. If a user-defined operator conflicts with standard operators, this can confuse the compiler and lead to errors.
Example:
class CustomInt {
public:
int value;
CustomInt(int v) : value(v) {}
// Overloading the operator= incorrectly
CustomInt operator=(const int rhs) {
value = rhs; // valid for assignment from int
return *this;
}
};
int main() {
CustomInt ci(10);
// This will lead to a conflict, resulting in an error
ci + 2; // Error: no match for 'operator+'
return 0;
}
In this example, the `CustomInt` class can handle assignments, but lacks an overloaded `+` operator, causing issues when trying to use it with an integer.
C++ Template and Operator Issues
Templates add flexibility to C++, but they can also lead to no match for operator errors if operators are not defined or appropriately specialized for specific types.
For instance, consider a template class that uses an operator without the correct overload:
template <typename T>
class Wrapper {
public:
T value;
Wrapper(T v) : value(v) {}
};
int main() {
Wrapper<int> wi(10);
// Assuming T = double gives no match for operator error
Wrapper<double> wd(20.5);
// This will produce an error due to lack of operator overloads
Wrapper<double> sum = wi + wd; // Error: no match for 'operator+'
return 0;
}
In this case, the operation cannot be completed because the correct operator overloads for template instantiation are missing.
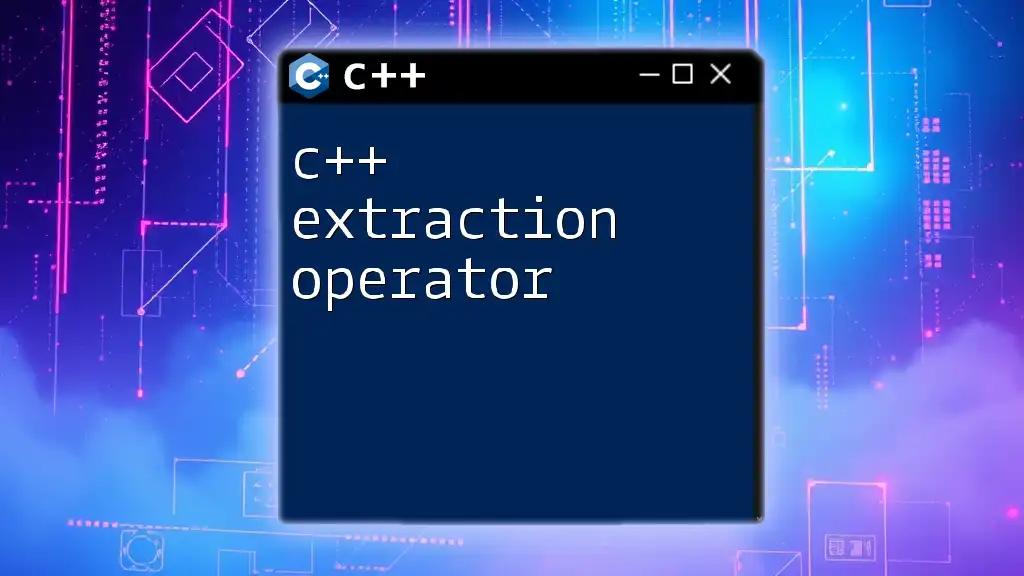
Troubleshooting the "No Match for Operator" Error
Reading Compiler Error Messages
When encountering the c++ no match for operator compilation message, pay close attention to the specific error details provided by the compiler. The message typically includes the types of the operands involved, allowing you to identify which operator and what types are problematic.
Step-by-Step Troubleshooting Approach
Check Data Types
Begin by verifying the data types involved in the operation. Ensure they are compatible and expected by the operator in use. For instance, updating types to match the expected operator functionality can resolve many issues.
Example solution:
class MyStruct {
public:
int value;
MyStruct operator+(const MyStruct& other) {
return MyStruct{value + other.value}; // Overloading for addition
}
};
Implementing Operator Overloading
To eliminate the no match for operator error due to missing overloads, implement the required operator overloads explicitly.
Consider the following corrected example:
#include <iostream>
class Vector {
public:
int x, y;
Vector(int x, int y) : x(x), y(y) {}
Vector operator+(const Vector& other) {
return Vector(x + other.x, y + other.y); // Define how addition works
}
};
int main() {
Vector v1(1, 2);
Vector v2(3, 4);
Vector v3 = v1 + v2; // Now works with operator+ defined
std::cout << "Result: (" << v3.x << ", " << v3.y << ")" << std::endl;
return 0;
}
By providing an appropriate overload for `operator+`, the operation can successfully proceed without errors.
Checking Template Specialization
If using templates, make sure that your operator definitions are well-formed and accessible for specific types. You might need to explicitly specialize if your template class relies on operators.
template <typename T>
class Wrapper {
public:
T value;
Wrapper(T v) : value(v) {}
// Ensure you can work with Wrapper types
Wrapper<T> operator+(const Wrapper<T>& other) {
return Wrapper<T>(value + other.value);
}
};
int main() {
Wrapper<int> wi(10);
Wrapper<int> wd(20);
Wrapper<int> sum = wi + wd; // Now valid
std::cout << "Sum: " << sum.value << std::endl;
return 0;
}

Best Practices to Avoid “No Match for Operator”
Using Proper Data Types
To reduce the chances of encountering no match for operator errors, always select appropriate data types for your operations. If your classes need to interact with built-in types, implement necessary operator overloads to facilitate this interaction smoothly.
Regularly Reviewing Operator Overloads
Take time to review your class definitions for operator overloads regularly. Ensure that all necessary operations are defined, especially if your class evolves or you add new functionalities.
Utilizing Smart Pointers and Other Modern C++ Features
Leverage modern C++ features like smart pointers and standard library utilities. These can minimize type conflicts and help encapsulate ownership semantics, reducing the need for manual operator definition or complex overload scenarios.

Conclusion
Understanding the c++ no match for operator error is crucial for any C++ developer. By identifying common causes and following the outlined troubleshooting strategies, you can effectively resolve these issues. Employ best practices to avoid such pitfalls in your future programming endeavors. Through practice and experimentation, you will gain deeper insights into how operators work in C++ and enhance your programming skills significantly.

Additional Resources
For further reading and assistance, consider exploring dedicated C++ resources, forums, and communities that focus on operator usage and troubleshooting complexities in C++. Engaging with other developers can provide additional insights and innovative solutions to your coding challenges.