In C++, the logical AND operator (`&&`) is used to combine two boolean expressions, returning `true` only if both expressions evaluate to `true`.
Here’s an example code snippet:
#include <iostream>
int main() {
bool a = true;
bool b = false;
if (a && b) {
std::cout << "Both a and b are true." << std::endl;
} else {
std::cout << "At least one of a or b is false." << std::endl;
}
return 0;
}
Understanding Logical Operators in C++
Logical operators in C++ are crucial for creating complex conditions in your code. They allow you to form expressions that can evaluate to true or false based on the values of other boolean expressions. Having a firm grasp on these operators enables you to control the flow of your programs more effectively.
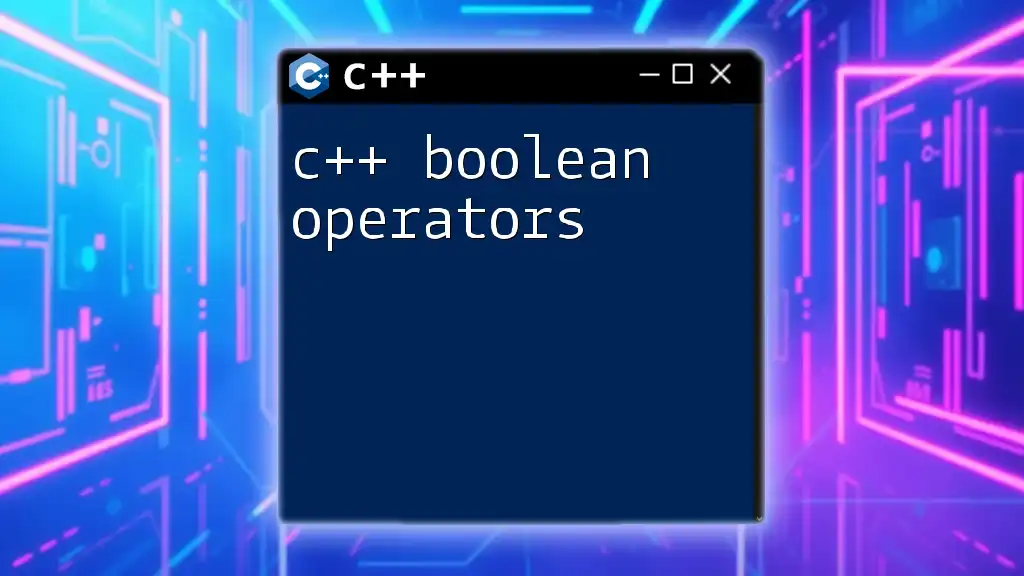
The AND Operator (`&&`)
What is the AND Operator?
The AND operator (`&&`) evaluates two boolean expressions and returns true only if both expressions are true. If either expression is false, the result will be false. This behavior is often described as short-circuit evaluation, where C++ will stop evaluating as soon as it determines the overall value.
Syntax and Usage
You can use the AND operator with the following syntax:
expression1 && expression2
For example:
bool a = true;
bool b = false;
if (a && b) {
cout << "Both are true";
} else {
cout << "At least one is false"; // This will execute
}
Common Use Cases
The AND operator is frequently applied in situations where multiple conditions need to be satisfied. For example, you might want to check if a user is both logged in and has admin privileges before allowing them access to certain features. This ensures both conditions must be met, promoting greater security and control over the program's flow.
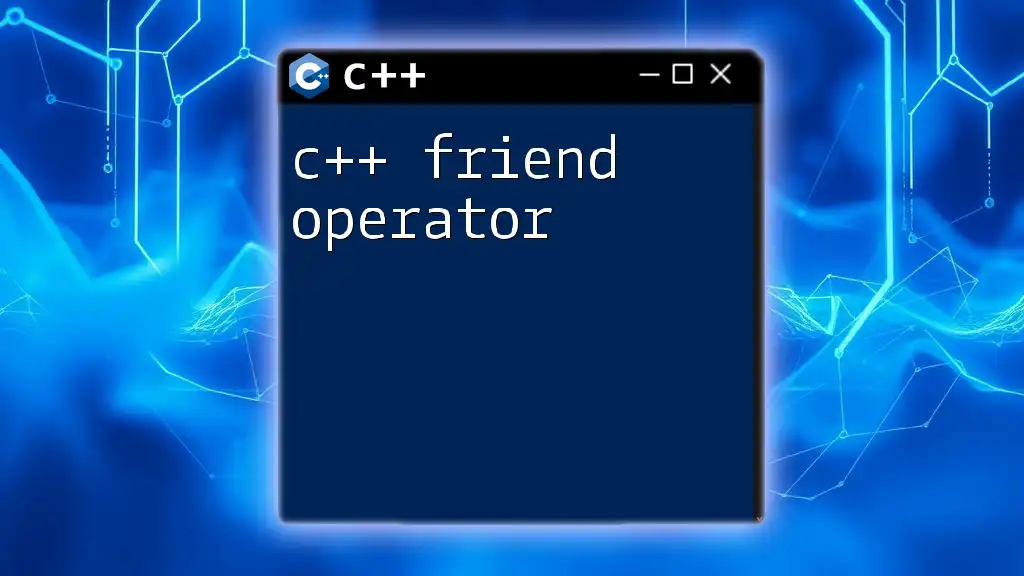
The OR Operator (`||`)
What is the OR Operator?
The OR operator (`||`) evaluates two boolean expressions and returns true if at least one of the expressions is true. If both are false, it will return false. Like the AND operator, the OR operator also employs short-circuit evaluation, meaning that if the first expression is true, the second one will not be evaluated.
Syntax and Usage
The OR operator can be implemented using the following syntax:
expression1 || expression2
Here’s an illustrative example:
bool a = true;
bool b = false;
if (a || b) {
cout << "At least one is true"; // This will execute
} else {
cout << "Both are false";
}
Common Use Cases
You’ll often find the OR operator useful in conditions where one of several options needs to be valid. A classic example is checking if a user is eligible for discounts based on membership levels, where they could qualify for a discount if they have either a premium or a student membership.
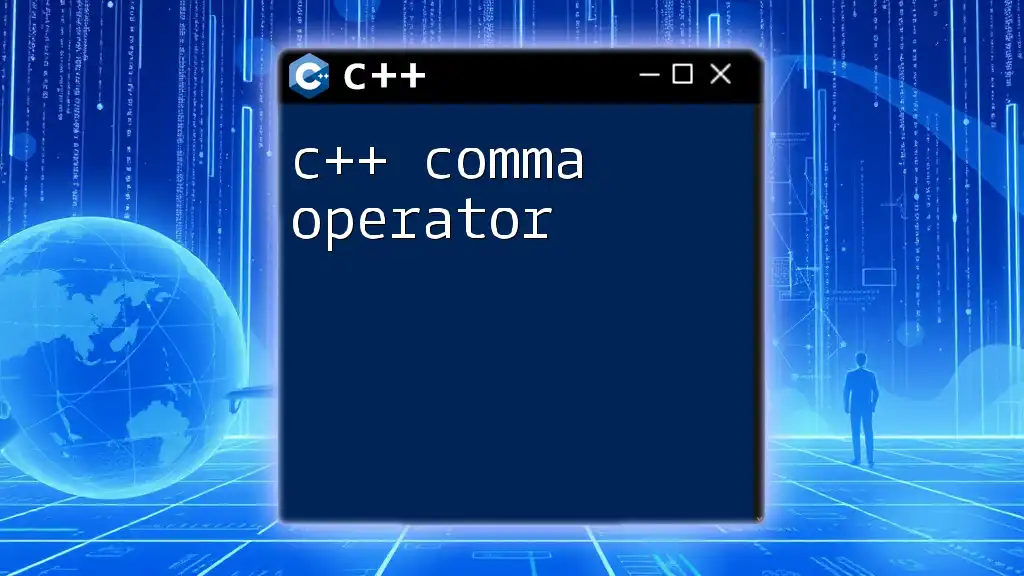
The NOT Operator (`!`)
What is the NOT Operator?
The NOT operator (`!`) is used to negate or invert the value of a boolean expression. If the expression evaluates to true, using the NOT operator will change it to false, and vice versa.
Syntax and Usage
The NOT operator is straightforward to use with the following syntax:
!expression
For example:
bool a = true;
if (!a) {
cout << "a is false";
} else {
cout << "a is true"; // This will execute
}
Common Use Cases
The NOT operator is especially handy for controlling program logic where certain conditions should not be met. For instance, you might want to ensure a game continues only if a player is not dead, thereby negating the condition to halt the game if they are alive.
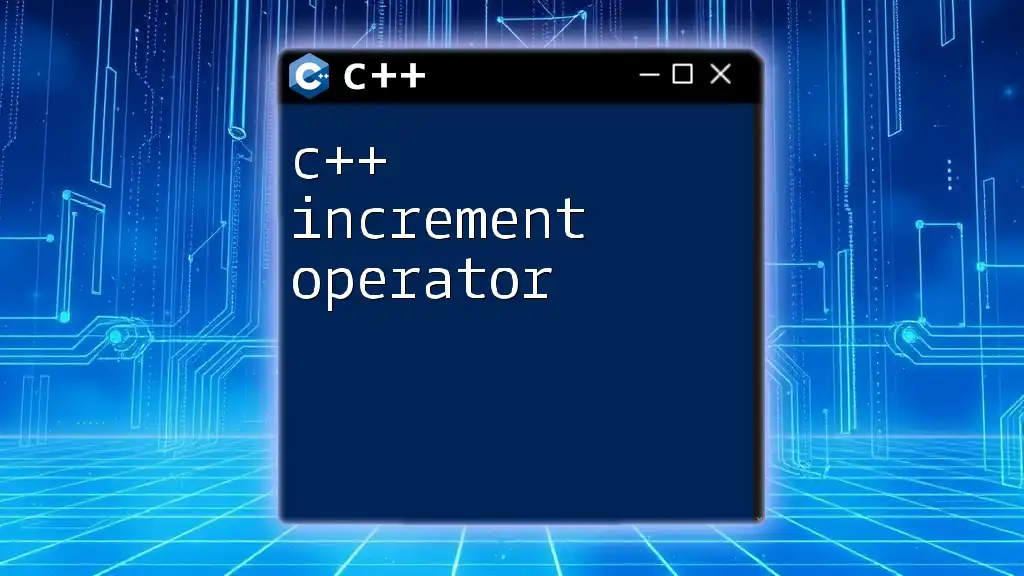
Operator Precedence
Understanding Operator Precedence
Operator precedence determines the order in which operations are evaluated in C++. Logical operators have their own levels of precedence that can affect the outcome of complex expressions.
Examples
Here's an example demonstrating how operator precedence influences evaluation:
bool a = true;
bool b = false;
bool c = true;
if (a || b && c) {
cout << "Condition is true"; // This will execute
}
In this example, `b && c` is evaluated first due to higher precedence, resulting in `false`, and then `a || false` evaluates to `true`.
Implicit vs Explicit Parentheses
To create clearer logic flows, using parentheses can help in situations where you want to enforce a specific order of evaluation:
if ((a || b) && c) {
// This ensures (a || b) is evaluated first
}
Using parentheses not only clarifies your intent but also prevents potential bugs and confusion regarding operator precedence.
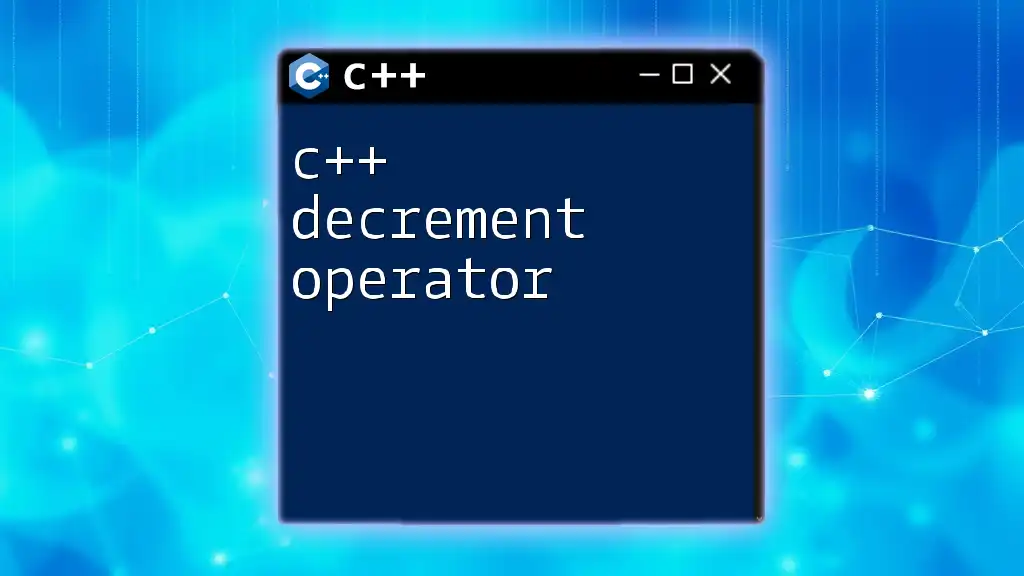
Best Practices for Using Logical Operators
When utilizing logical operators in your C++ code, keep these best practices in mind:
- Keep Expressions Simple: Simplify logical expressions to maintain code readability. Complex expressions can lead to confusion.
- Use Parentheses Wisely: Always consider using parentheses to reinforce order of evaluation, even if they are not strictly necessary.
- Avoid Redundant Logic: When using multiple operators, make sure you are not repeating conditions unnecessarily, which can lead to less efficient code.
- Comment Your Logic: When appropriate, add comments explaining the purpose of complex logical expressions to aid future maintainability.
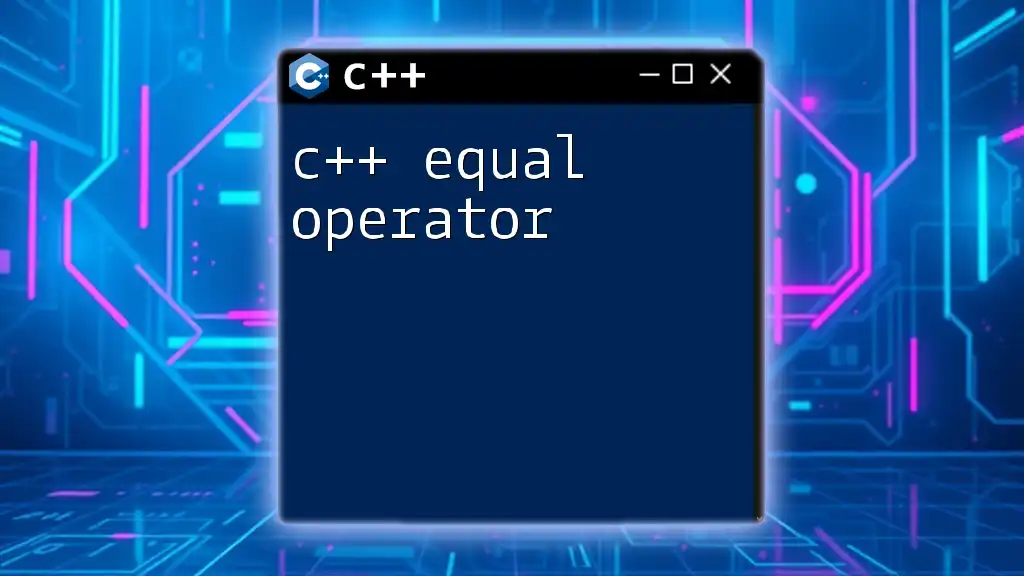
Conclusion
C++ logical operators play an essential role in forming effective conditional statements, allowing you to create intricate logic structures within your programs. Understanding how to use the AND, OR, and NOT operators, along with grasping operator precedence and best practices, will empower you to write cleaner, more robust code.
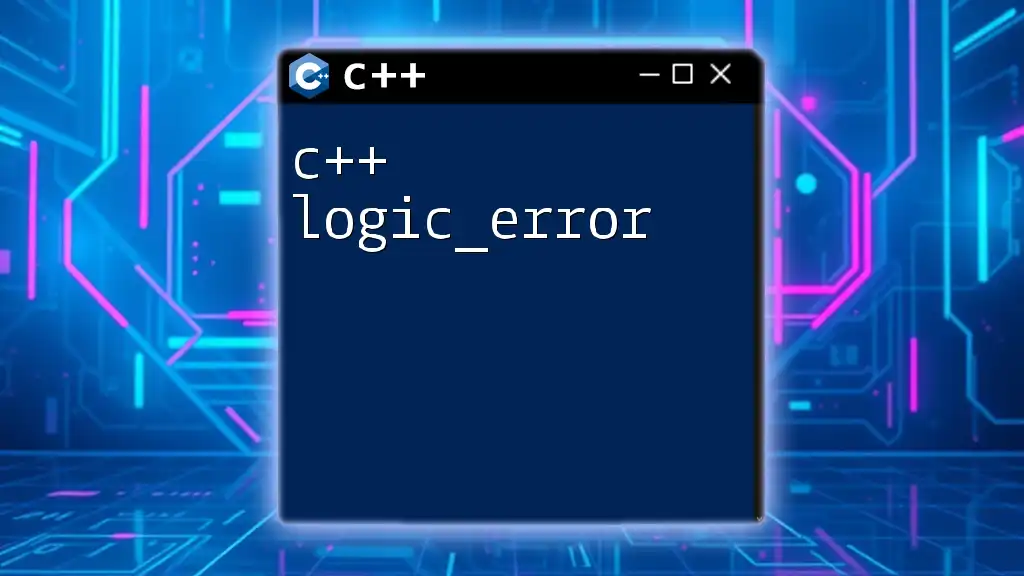
Additional Resources
For further learning, you may wish to explore additional readings on C++ logical operators through recommended books, online courses, and community forums where you can ask for advice and discuss logical constructs with peers.