Global operator overloading in C++ allows you to define how operators (like +, -, *, etc.) behave for user-defined types, enabling intuitive interactions with your objects.
Here’s an example of overloading the addition operator for a custom `Point` class:
#include <iostream>
class Point {
public:
int x, y;
Point(int x, int y) : x(x), y(y) {}
// Global operator overload
friend Point operator+(const Point& p1, const Point& p2) {
return Point(p1.x + p2.x, p1.y + p2.y);
}
};
int main() {
Point p1(1, 2);
Point p2(3, 4);
Point p3 = p1 + p2; // Uses overloaded operator+
std::cout << "Result: (" << p3.x << ", " << p3.y << ")\n"; // Output: (4, 6)
return 0;
}
Understanding Operator Overloading
What is Operator Overloading?
Operator overloading allows C++ programmers to redefine the way operators work for user-defined types (like classes). This functionality enables developers to specify how operators interact with their objects, which can enhance the readability and intuitiveness of the code. For instance, say you have a `Complex` number class; by overloading the `+` operator, you can easily add two complex numbers using the `+` symbol rather than calling a function.
Types of Operator Overloading
C++ supports two primary methods for operator overloading: member function operator overloading and global operator overloading. While member function overloading is done within the class itself (where the object itself acts on the operator), global operator overloading allows operators to work on two distinct objects, making the function independent of the class. Each method has its own use cases and advantages.
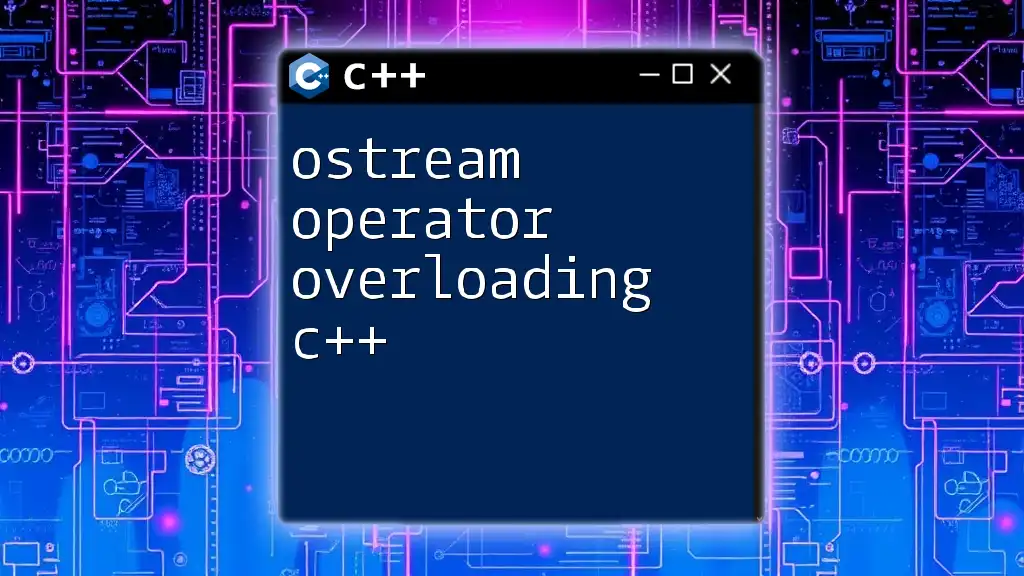
Global Operator Overloading in C++
What is Global Operator Overloading?
Global operator overloading refers to defining operator functions outside the class scope. It allows you to operate with two types or classes that may not be related through inheritance. This is particularly useful when you want to allow interaction between different data types or classes.
Syntax for Global Operator Overloading
The syntax for global operator overloading follows a straightforward structure. Here’s the basic form:
ReturnType operator OpType(parameters) {
// implementation
}
Example: Let’s consider an implementation where we define a global `+` operator to add two objects of a class named `MyClass`.
class MyClass {
public:
int value;
MyClass(int v) : value(v) {}
};
// Overloading '+' operator globally
MyClass operator+(const MyClass& a, const MyClass& b) {
return MyClass(a.value + b.value);
}
In this example, we define how the `+` operator behaves when used with objects of `MyClass`.
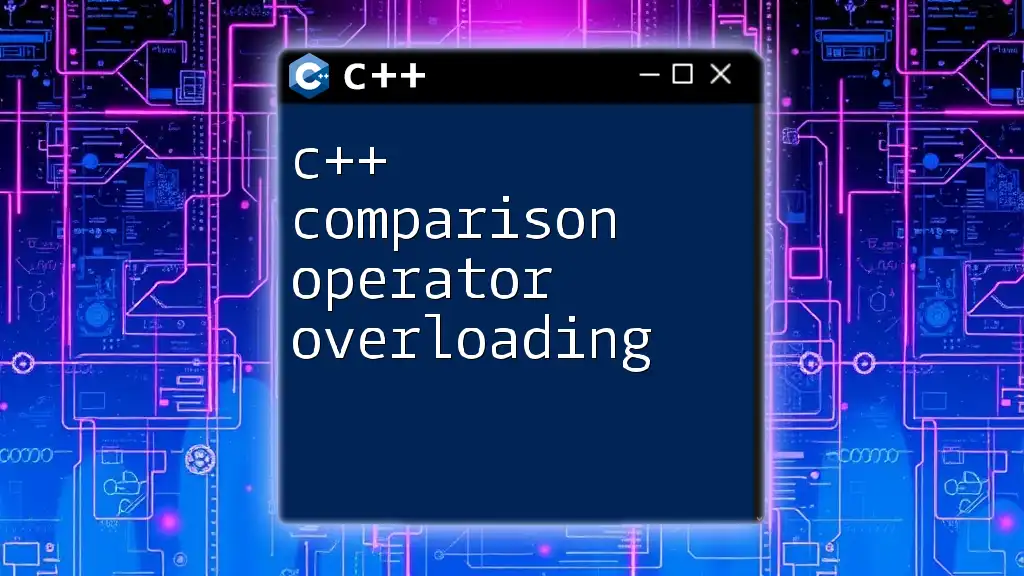
How to Implement Global Operator Overloading
Step-by-step Implementation
- Define a class: Create a class that encapsulates the desired properties.
- Create overloaded operator function: Define the operator function outside the class, specifying how it interacts with class instances.
Example Implementation
Let’s explore a practical example where we create a class to represent complex numbers and overload the `+` operator.
class Complex {
public:
float real;
float imag;
Complex(float r, float i) : real(r), imag(i) {}
};
// Overloading '+' operator globally to add two complex numbers
Complex operator+(const Complex& c1, const Complex& c2) {
return Complex(c1.real + c2.real, c1.imag + c2.imag);
}
In this snippet, we define a `Complex` number class where `real` and `imag` are floating-point numbers, representing the real and imaginary part of the complex number. The global `+` operator function returns a new `Complex` object, expressing the sum of two complex numbers intuitively.
Benefits of Using Global Operator Overloading
Implementing global operator overloading can significantly improve code readability and make interactions between your objects more intuitive. For instance, it allows you to express operations like addition or multiplication directly using operators rather than calling functions, enhancing the overall syntax of your code.
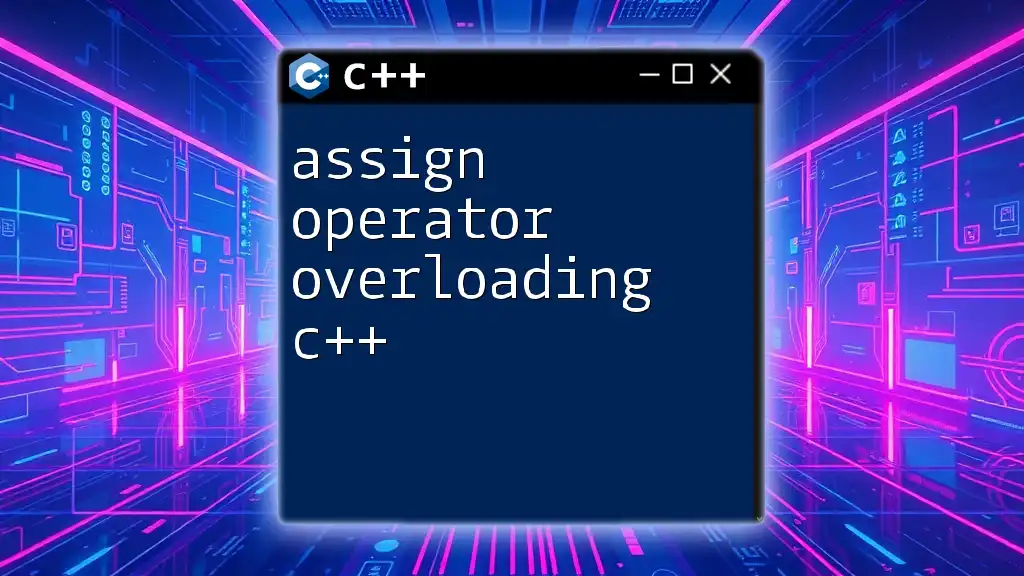
Common Mistakes to Avoid
Ignoring Const Qualifiers
A common pitfall when overloading global operators is neglecting const correctness. Failing to declare the parameters as constant references can lead to unnecessary copying or performance issues.
Example of a potential mistake:
Complex operator+(Complex& c1, Complex& c2) { ... } // Not const-correct
In this case, both `c1` and `c2` should be passed as constant references to avoid any unintended modifications and optimize performance.
Confusing Global and Member Overloading
It’s easy to confuse the two overload methods. Global operator overloading can often come off as a less popular choice due to potential confusion about syntax. However, understanding when to use each is crucial.
As a rule of thumb, use global operator overloading when you need to combine different types and member operator overloading when working exclusively with instances of a single class.
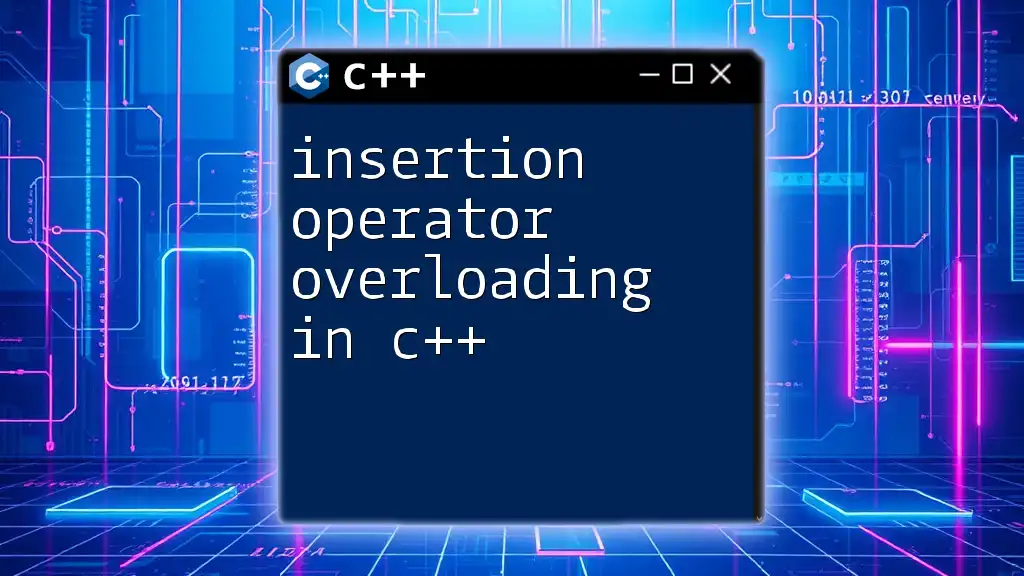
Best Practices for Global Operator Overloading
Maintain Consistency
When overloading operators globally, it’s vital to maintain consistency in the behavior of operators. For instance, if you overload `+` to add two objects, ensure that the same logic applies to other related operators like `+=` for coherence in your code.
Keep It Simple
Overloaded operators should ideally be simple. Avoid complex logic within operator overload definitions to keep the intention clear. A clean and maintainable operator overload enhances the developer experience for anyone using your class.
For example, compare the following:
Complex Example:
Complex operator+(const Complex& c1, const Complex& c2) {
// This may contain too much logic
if (c1.real > c2.real) {
return Complex(c1.real + c2.real, c1.imag + c2.imag);
}
// Additional conditions can complicate understanding.
}
Simple Example:
Complex operator+(const Complex& c1, const Complex& c2) {
return Complex(c1.real + c2.real, c1.imag + c2.imag);
}
The simple example is clearer, easier to read, and therefore preferable.
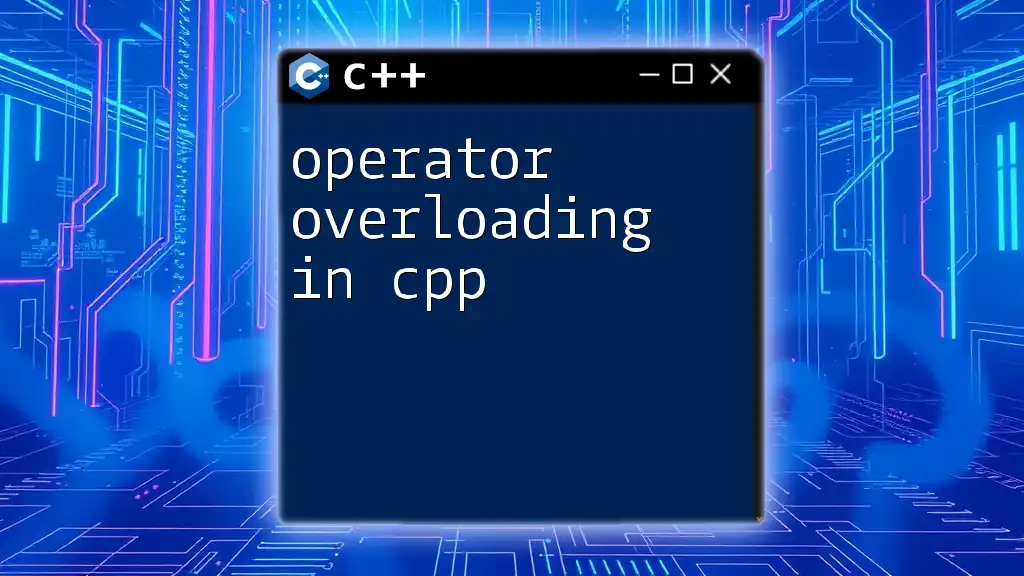
Performance Considerations
Efficiency in Global Operator Overloading
Global operator overloading can introduce overhead, particularly when dealing with temporary objects or performing deep copies. To mitigate this, it may be essential to utilize move semantics available in C++11 and later.
Conclusion on Performance
Incorporating move semantics in your operator overloads can reduce unnecessary copy overhead, improving runtime efficiency. This is especially relevant in scenarios where operations lead to temporary objects, as it allows them to take ownership of resources rather than duplicating them.
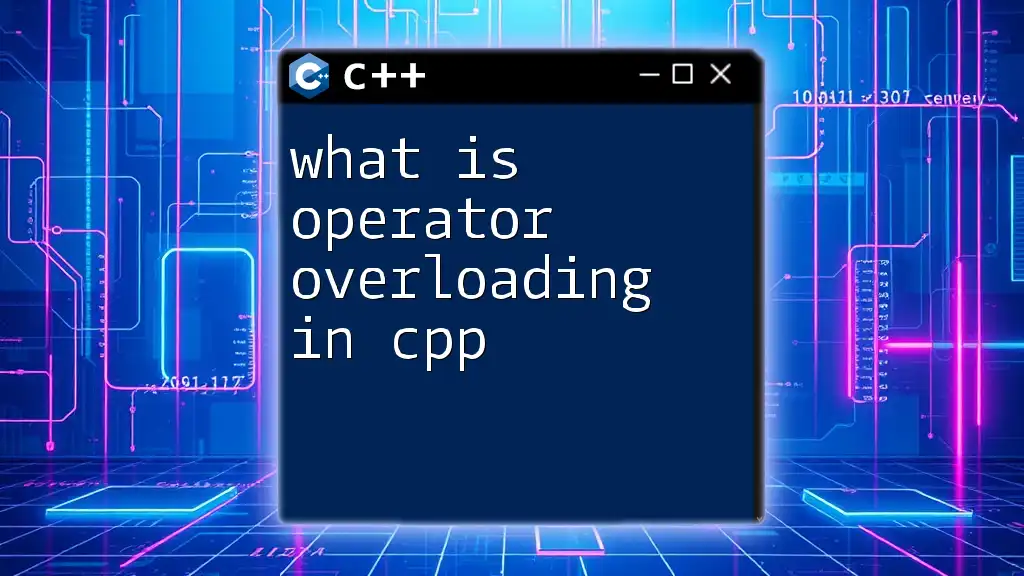
Conclusion
C++ global operator overloading enhances the way objects interact, allowing for more intuitive code without the need to write verbose function calls. By implementing these strategies effectively, you can harness the full power of C++ and create readable, maintainable, and efficient applications.
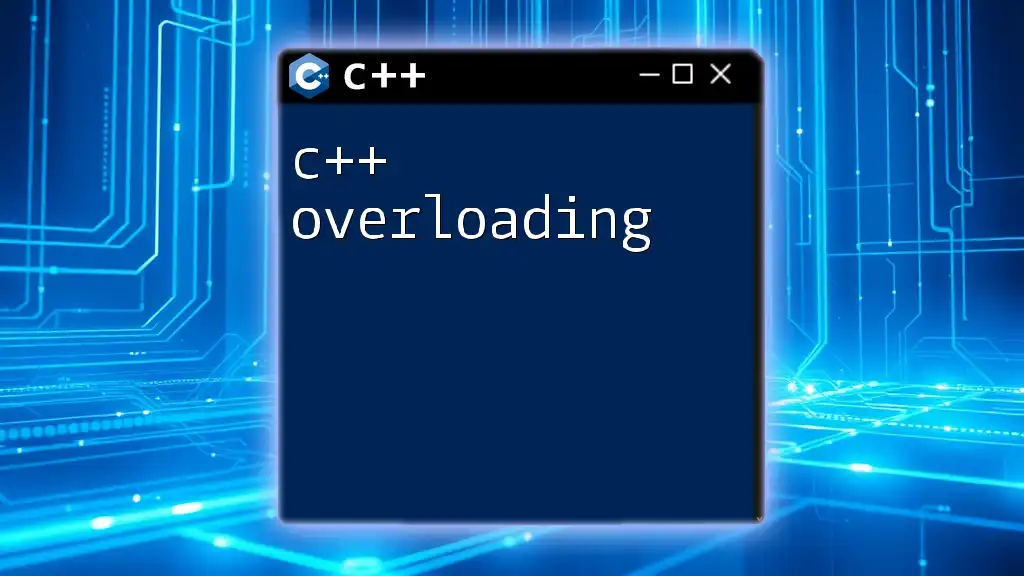
Additional Resources
If you’re looking to deepen your understanding of C++ global operator overloading, consider exploring documentation and tutorials that offer practical examples and case studies.
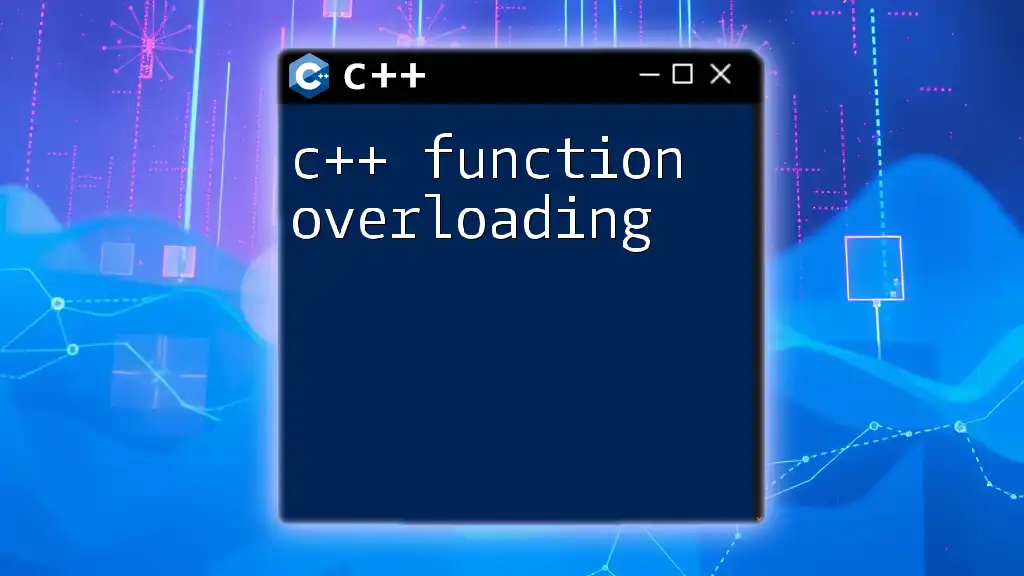
FAQs
What are the benefits of using global operator overloading?
Global operator overloading allows for smoother interactions between disparate types, improving code clarity and making concepts easier to grasp.
Can I overload all operators globally?
Most operators can be overloaded globally; however, operators such as `.` (member access), `.*` (pointer-to-member), and `::` (scope resolution) cannot be overloaded.
How do global overloaded operators interact with member overloaded operators?
Global and member overloaded operators can coexist, but it is essential to maintain clarity on which operator type is being used in different contexts to prevent confusion.
By following these guidelines and considerations, you can successfully implement C++ global operator overloading in your projects, leading to cleaner, more manageable code and an improved overall experience for developers and users alike.