Insertion operator overloading in C++ allows you to define how objects of your custom classes should be outputted to streams (like `cout`) by overriding the `<<` operator.
#include <iostream>
class Point {
public:
int x, y;
friend std::ostream& operator<<(std::ostream& os, const Point& p) {
os << "(" << p.x << ", " << p.y << ")";
return os;
}
};
int main() {
Point p{3, 4};
std::cout << p; // Output: (3, 4)
return 0;
}
What Is Operator Overloading?
Definition of Operator Overloading
Operator overloading in C++ is a powerful feature that allows developers to redefine the way operators work with user-defined types (classes or structs). This means you can specify the behavior of operators (like `+`, `-`, `*`, and, notably, the insertion operator `<<`) when they operate on your custom objects. By doing this, you can make your code more intuitive and easier to read.
Benefits of Operator Overloading
- Enhanced Readability: By overloading operators, your code can be more expressive and reflect mathematical or logical formulations naturally. For example, if you overload the `+` operator for a `Complex` number class, you can write `c1 + c2` instead of a method call like `c1.add(c2)`.
- User-Friendly Interfaces: Operator overloading allows users to interact with objects using familiar syntax. This makes the code more accessible to other developers and reduces the learning curve required to understand your custom types.
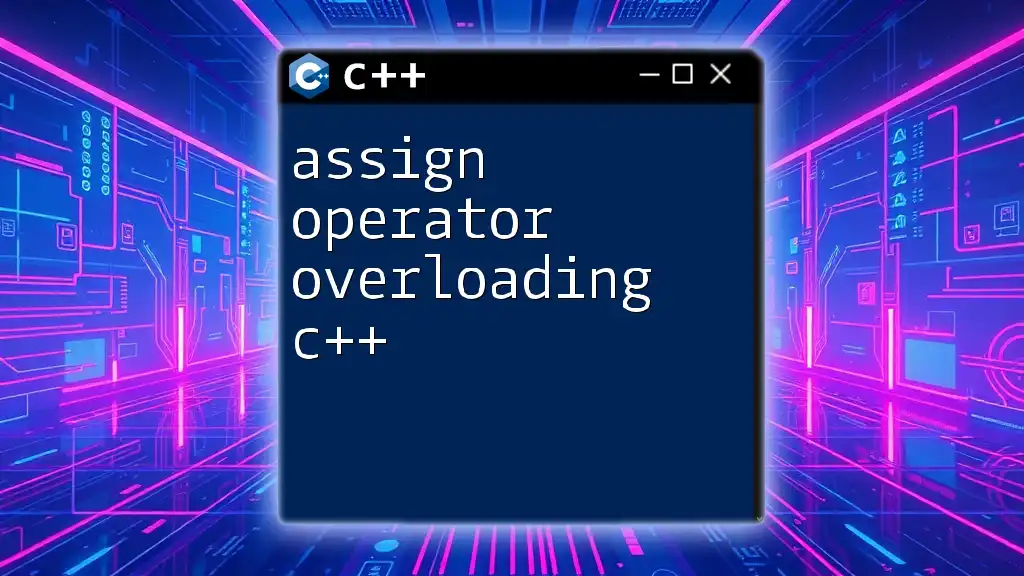
Understanding the Insertion Operator
What Is the Insertion Operator (`<<`)?
The insertion operator (`<<`), often used with output streams such as `std::cout`, is a fundamental part of C++'s input/output (I/O) mechanism. It is primarily responsible for sending data to output devices, such as the console.
How the Insertion Operator Works
The insertion operator takes the left operand as an output stream (like `std::cout`) and the right operand as the data to be printed. For example:
std::cout << "Hello, World!";
This line sends the string `"Hello, World!"` to standard output. The `<<` operator handles all sorts of data types, including built-in ones and custom classes, thanks to operator overloading.
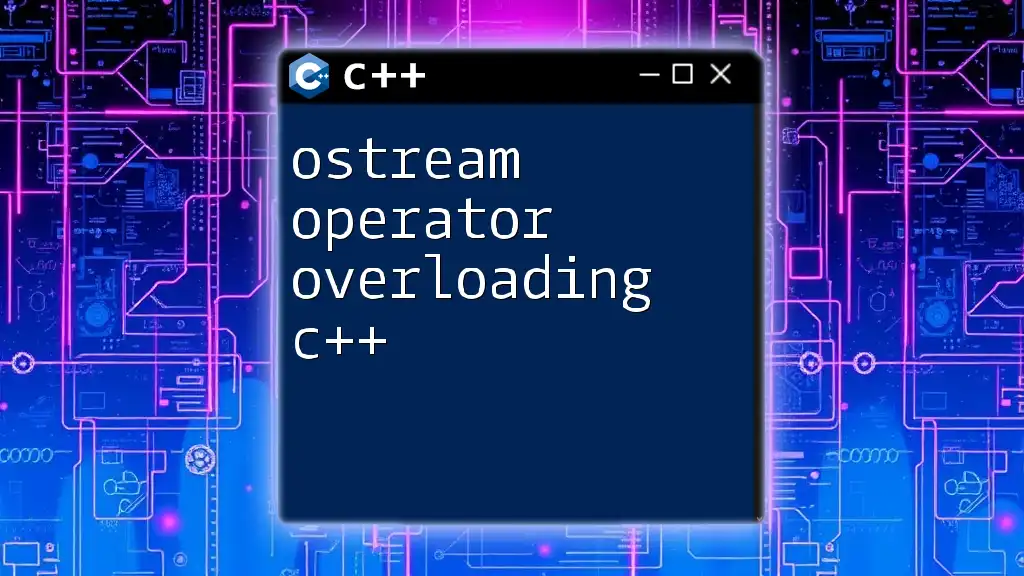
How to Overload the Insertion Operator
Syntax of Operator Overloading
To overload the insertion operator in C++, you'll typically define a friend function inside your class. The friend function allows you to access the private and protected members of the class from outside of it.
Steps to Overload the Insertion Operator
- Define the class for which the operator is being overloaded.
- Create a friend function that takes an `std::ostream` reference as its first argument and a const reference to your class as its second argument.
Example: Overloading the Insertion Operator
Here's an example demonstrating how to overload the insertion operator for a simple `Point` class representing a 2D point:
#include <iostream>
class Point {
public:
int x, y;
Point(int x, int y) : x(x), y(y) {}
// Overloading the insertion operator
friend std::ostream& operator<<(std::ostream& out, const Point& p) {
out << "(" << p.x << ", " << p.y << ")";
return out;
}
};
int main() {
Point p(10, 20);
std::cout << p;
return 0;
}
In this code:
- A `Point` class is defined with two integer attributes, `x` and `y`.
- The insertion operator `<<` is overloaded as a friend function, allowing it to access private members of the class.
- Inside the friend function, we define how an object of `Point` should be formatted when sent to an output stream.
- The `main` function demonstrates the overloaded operator by printing the point.
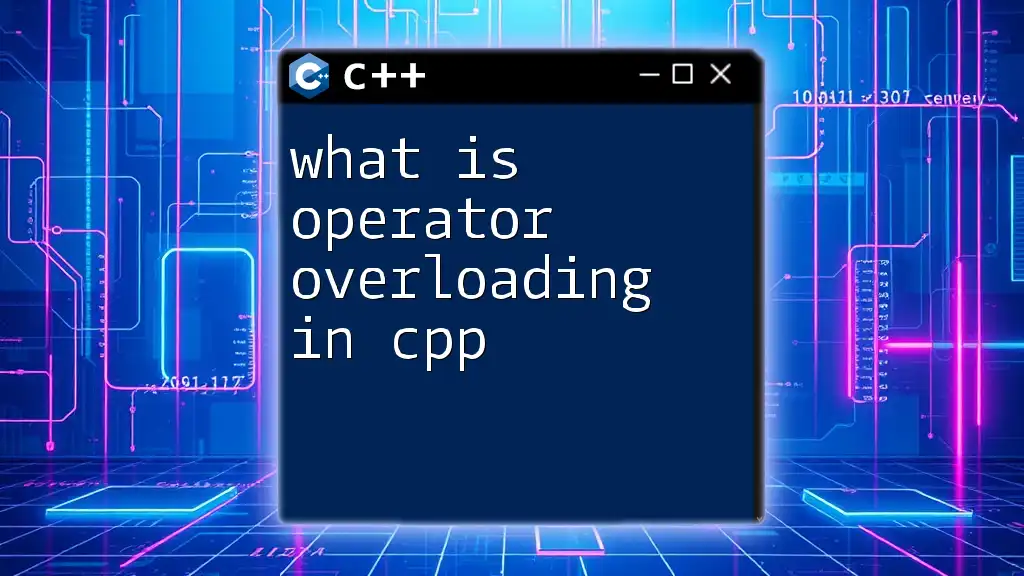
Best Practices for Overloading Operators
Keep It Intuitive
When overloading operators, make sure their behavior is intuitive. Users of your class should readily understand what an overloaded operator does without delving into its implementation. For instance, if you overload `+` for a vector class to perform vector addition, it's logical and expected behavior. Conversely, overloading `+` to perform subtraction might confuse users.
Maintain Consistency
It's essential to maintain consistent behavior when overloading operators. The user should expect similar results when using familiar operators across different class types. For example, if you've overloaded the `==` operator to compare objects, ensure that it behaves logically, akin to how built-in types behave.
Error Handling
When implementing operator overloading, consider incorporating error handling to prevent undefined behavior. For instance, if attempting to output an object without an overloaded insertion operator, ensure the function gracefully reports an error. This consideration greatly improves the robustness and usability of your code.
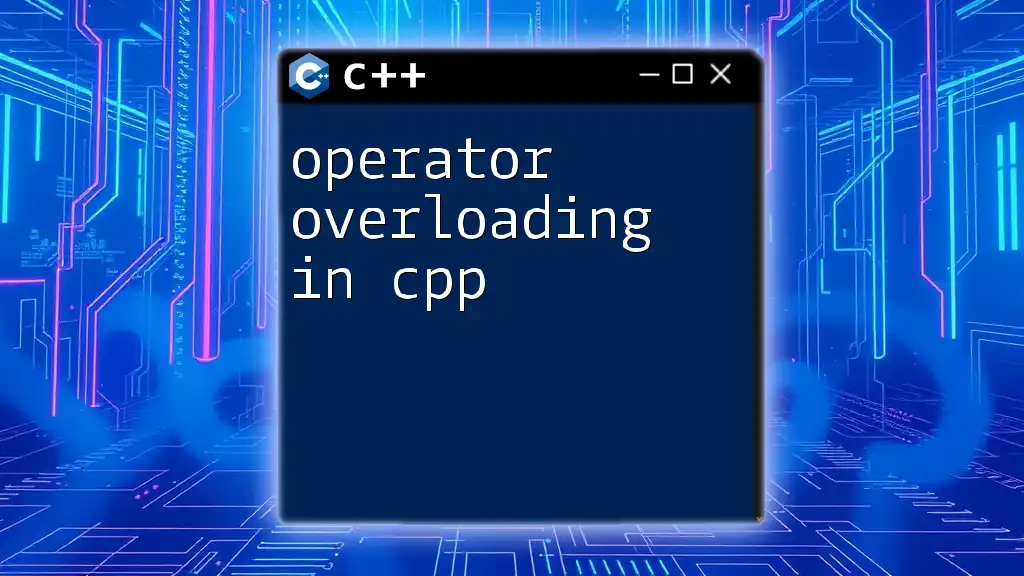
Real-World Applications of Insertion Operator Overloading
Data Representation
Operator overloading is particularly useful in creating user-friendly representations of complex data structures. When dealing with classes that encapsulate complex data—such as matrices or graphs—overloading the insertion operator can significantly enhance the output readability.
Code Snippet for a More Complex Data Type:
Here’s an example of overloading the insertion operator for a `Vector` class:
#include <iostream>
#include <vector>
class Vector {
public:
std::vector<double> elements;
Vector(std::initializer_list<double> list) : elements(list) {}
// Overloading the insertion operator
friend std::ostream& operator<<(std::ostream& out, const Vector& v) {
out << "[";
for (size_t i = 0; i < v.elements.size(); ++i) {
out << v.elements[i];
if (i != v.elements.size() - 1) out << ", ";
}
out << "]";
return out;
}
};
int main() {
Vector v{1.1, 2.2, 3.3};
std::cout << v;
return 0;
}
In this example, the `Vector` class stores a list of double values, and we overload the insertion operator to output the vector elements in a readable format. This makes it clear and efficient for users to visualize the data contained within the class.
Debugging and Logging
Overloading the insertion operator enhances debugging and logging processes. By customizing the output representation of objects, developers can easily log the state of the application at various points, providing clarity and context when reviewing logs. This practice streamlines troubleshooting and helps identify issues within complex systems.
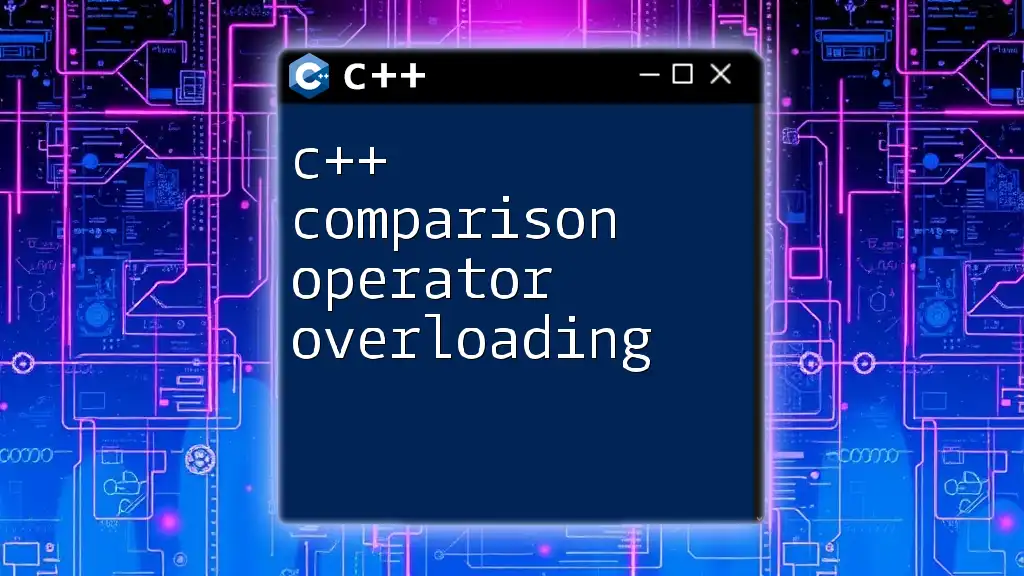
Conclusion
Insertion operator overloading in C++ is an essential skill that enables developers to create more intuitive and readable code. By following best practices and understanding how to implement this powerful feature, you can significantly enhance the usability of your classes. By applying the concepts discussed in this article, you can leverage operator overloading in various applications, from data representation to effective debugging. Embrace the art of operator overloading and take your C++ programming to new heights!
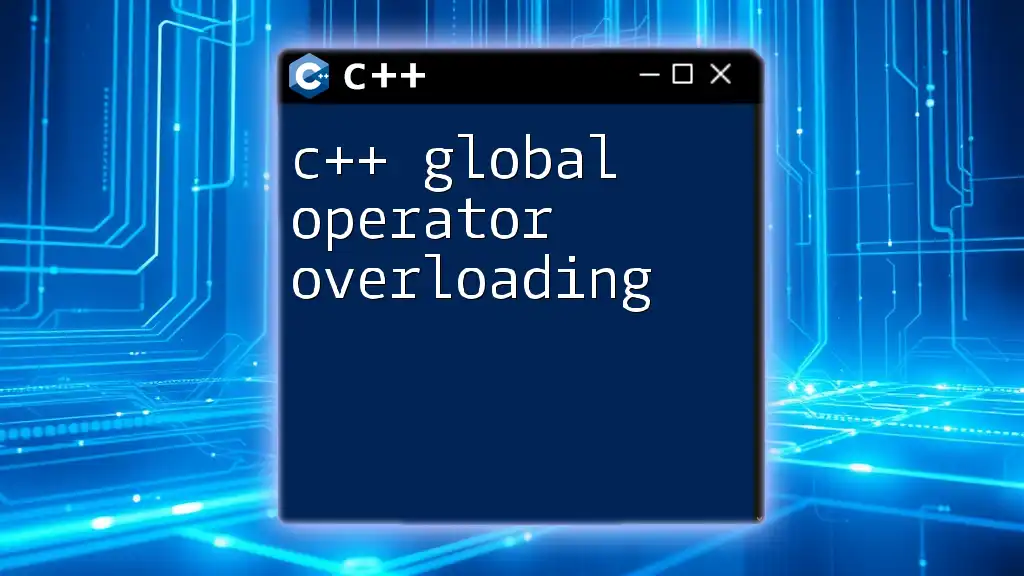
Additional Resources
For further reading, explore detailed resources on C++ operator overloading, including books, video tutorials, and online courses. Engaging with additional material can deepen your understanding and inspire innovative ways to apply operator overloading in your projects.