The assignment operator overloading in C++ allows you to define how the assignment operator (`=`) behaves when assigning one object of a class to another.
class MyClass {
public:
int data;
// Assignment operator overload
MyClass& operator=(const MyClass& other) {
if (this != &other) { // Check for self-assignment
data = other.data; // Copy the value
}
return *this; // Return current object
}
};
Understanding Assignment Operator Overloading in C++
What is Operator Overloading?
Operator overloading in C++ allows programmers to redefine the way operators work in a class context. This means you can create intuitive interfaces for classes and use operators like `+`, `-`, or `=` to manipulate objects naturally. Overloading improves code readability and usability, making classes easier to work with, especially in complex systems.
Introduction to Assignment Operators in C++
Assignment operators are fundamental to variable manipulation in C++. The default behavior of the assignment operator (`=`) is to perform a shallow copy, meaning it copies the value of one variable into another. However, this can lead to significant issues, particularly when managing resources like dynamic memory, where shallow copying might not suffice.
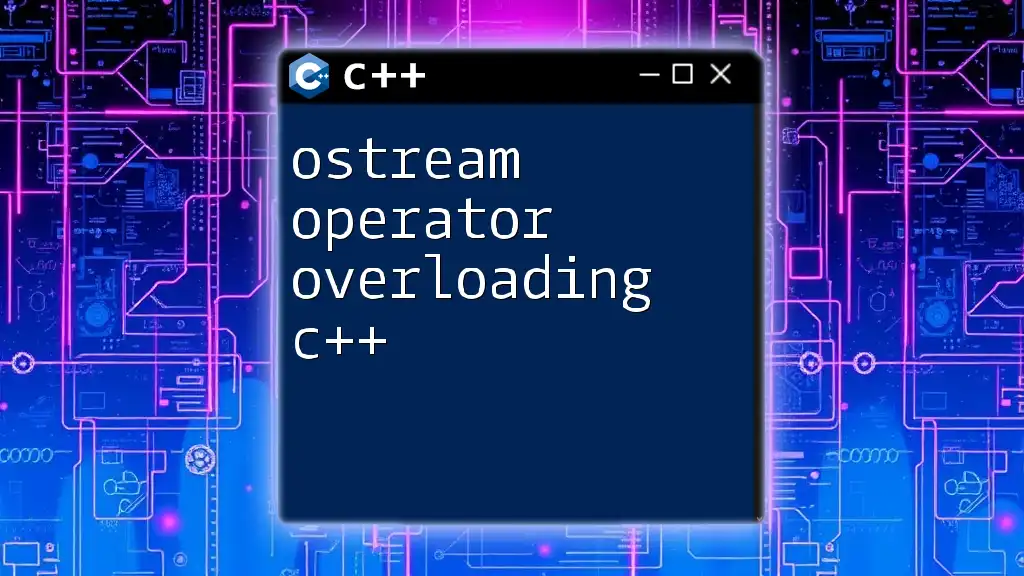
The Need for Assignment Operator Overloading
Why Overload the Assignment Operator?
Overloading the assignment operator becomes crucial when the default shallow copy does not meet the needs of your class. For example, if your class allocates memory dynamically, a shallow copy could result in multiple variables referencing the same memory location. This can lead to double deletion if two variables go out of scope and attempt to free the same resource. Customizing the assignment behavior allows for proper resource management, reducing potential memory leaks and segmentation faults.
Common Use Cases
Overloading the assignment operator is particularly beneficial in several scenarios, including:
- Managing Resources: Classes that handle pointers or file descriptors need to control how resources are assigned.
- Enhancing Performance: For large data structures, overloaded assignment can avoid costly copies by reusing existing resources.
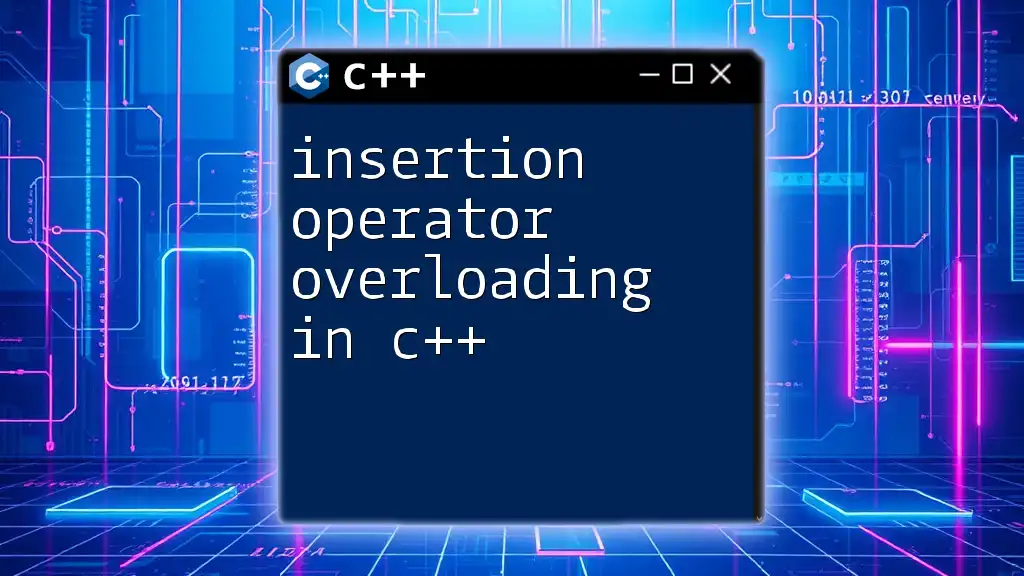
How to Overload the Assignment Operator in C++
Syntax for Overloading the Assignment Operator
To overload the assignment operator in C++, you use the `operator=` keyword. The typical structure resembles the following:
ClassName& operator=(const ClassName& other) {
// Assignment logic
}
Example: Overloading the Assignment Operator for a Simple Class
Here is an example that demonstrates how to overload the assignment operator effectively:
class Example {
public:
int* data;
// Constructor
Example(int value) {
data = new int(value);
}
// Destructor
~Example() {
delete data;
}
// Copy Assignment Operator Overloading
Example& operator=(const Example& other) {
if (this == &other) return *this; // Check for self-assignment
// Free existing resource
delete data;
// Deep copy assignment
data = new int(*other.data);
return *this;
}
};
In this example, the `Example` class contains a pointer to an integer. The overloaded `operator=` checks for self-assignment, releases any existing memory before copying the data from the `other` object. This ensures that the internal state remains consistent and valid.
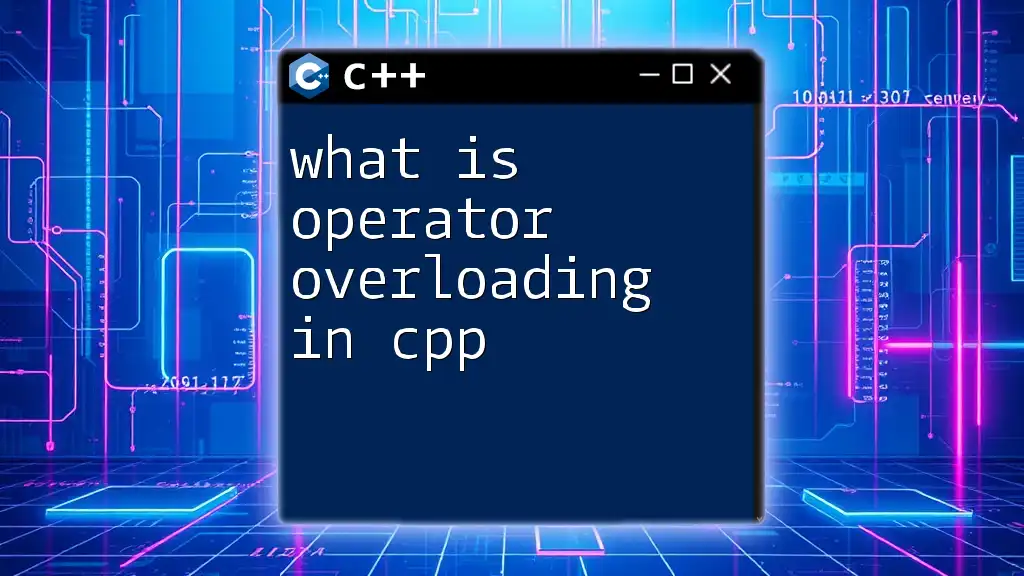
Best Practices for Overloading the Assignment Operator
The Rule of Three
When overloading the assignment operator, it is paramount to follow the Rule of Three. This rule states that if a class requires a user-defined destructor, copy constructor, or copy assignment operator, it likely requires all three. Consistent management of resources across these functionalities is essential to avoid undefined behavior.
Handling Self-Assignment
Always check for self-assignment in your overloaded assignment operator:
if (this == &other) return *this;
This line ensures that if the object is copied to itself, it aborts the operation early. Failing to do this may result in unintentionally deleting the object’s own resources.
Returning a Reference to `*this`
Returning a reference to `*this` allows for chain assignment to work effectively:
return *this;
This means you can use statements like `a = b = c;`, where `b` is assigned the value of `c`, and then `a` receives the updated value of `b`.
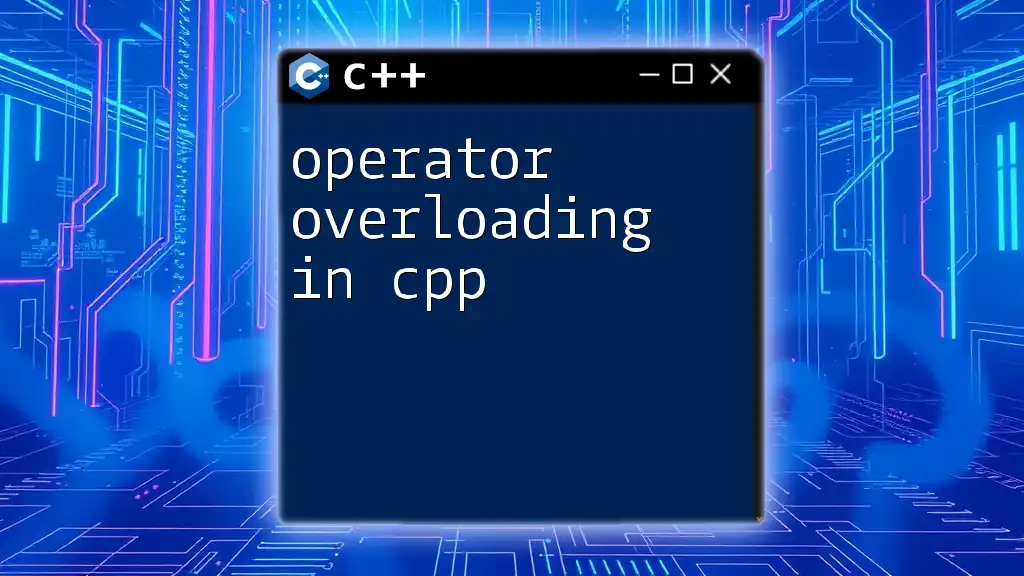
Common Pitfalls to Avoid
Forgetting to Release Resources
A frequent problem when overloading is failing to release previously allocated resources. This could lead to memory leaks, where memory remains allocated even after it is no longer needed. Always ensure resources are properly released before reassigning!
Not Implementing Copy Constructor
In relation to the assignment operator, if you implement one, you should also implement a copy constructor. If you fail to do this, you might use the default one, leading to shallow copy problems. Here’s a basic outline of a proper copy constructor:
Example(const Example& other) {
data = new int(*other.data);
}
Confusion with the Assignment vs Copy Constructor
Understanding when to use the assignment operator versus the copy constructor is vital. The assignment operator is called for existing objects, while the copy constructor is called when a new object is created from an existing one.
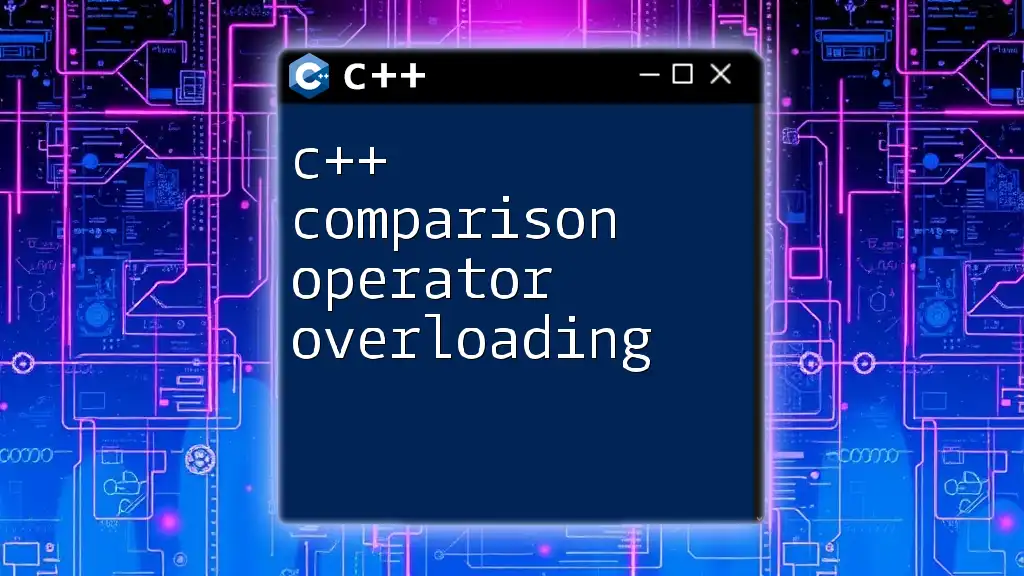
Conclusion
Recap of Key Points
Assign operator overloading in C++ is an essential skill for any developer looking to manage resources effectively and ensure code integrity. By understanding the mechanics of copying and overloading the assignment operator, you enhance both the functionality and safety of your classes.
Further Learning Resources
For those seeking deeper knowledge, consider exploring books like "Effective C++" by Scott Meyers or online tutorials that cover advanced C++ topics. These resources will help reinforce the concepts introduced in this article.
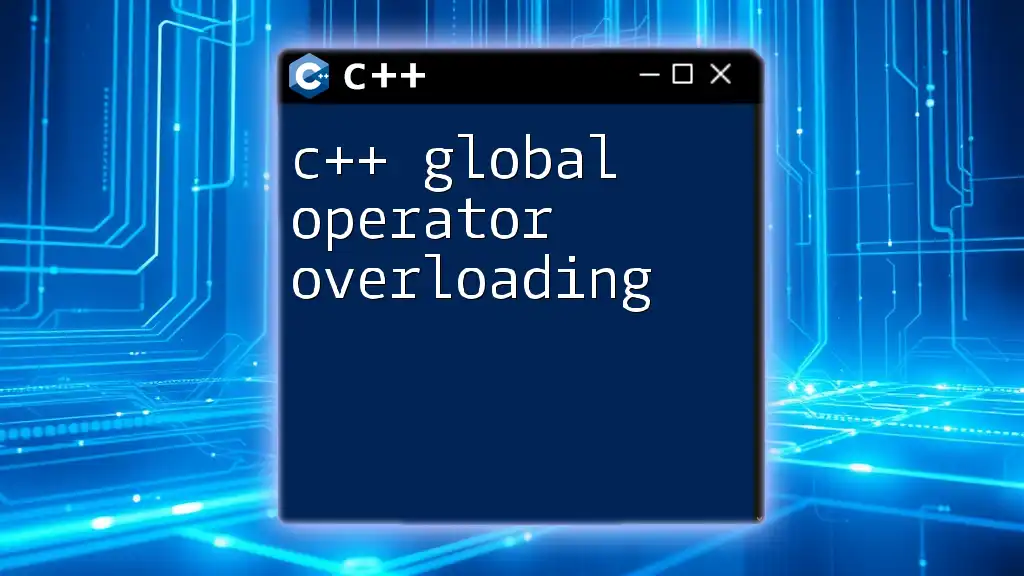
FAQs on C++ Assignment Operator Overloading
What is the purpose of overloading the assignment operator in C++?
Overloading the assignment operator allows for tailored object behavior when assigning one object to another, particularly in contexts where resource management is critical.
Can you overload the assignment operator for built-in types?
No, built-in types like `int`, `float`, etc., cannot be overloaded since they are intrinsic to the language. However, you can create custom classes for more complex behavior.
Are there any performance impacts of overloading the assignment operator?
The performance impact largely depends on how well the operator is implemented, particularly with resource management. Efficient handling can lead to performance gains while poor handling can result in overhead due to frequent memory allocations and deallocations.
This concludes the comprehensive guide on assign operator overloading in C++. Implement these practices in your projects to write safer and more efficient code!