Ostream operator overloading in C++ allows you to define how objects of your custom classes are outputted to streams, enabling intuitive printing of complex data types. Here's a code snippet demonstrating how to overload the `<<` operator:
#include <iostream>
class Point {
public:
int x, y;
Point(int x, int y) : x(x), y(y) {}
// Overload the << operator
friend std::ostream& operator<<(std::ostream& os, const Point& p) {
os << "Point(" << p.x << ", " << p.y << ")";
return os;
}
};
int main() {
Point p(3, 4);
std::cout << p; // Outputs: Point(3, 4)
return 0;
}
What is Operator Overloading?
Operator overloading is a powerful feature in C++ that allows you to redefine how operators (like `+`, `-`, `<<`, etc.) work with user-defined types. By overloading these operators, you can enhance the readability and usability of your code, making your classes easier to work with for other developers (or for yourself in the future).
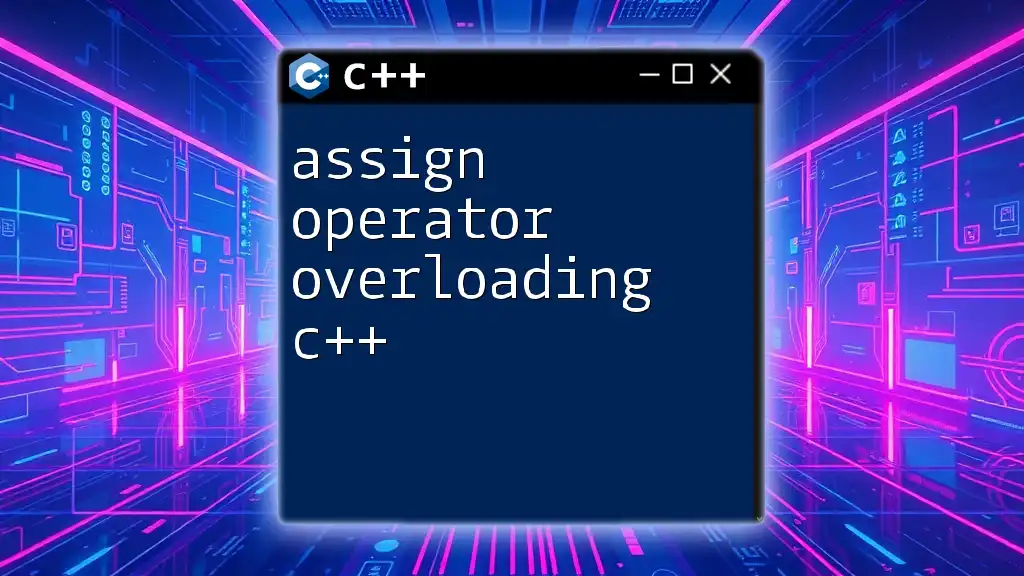
Why Use Ostream Operator Overloading?
When it comes to outputting information about objects in C++, the standard output stream (`std::cout`) often falls short for user-defined types. By implementing ostream operator overloading, you can define exactly how instances of your classes should be represented as strings when they are sent to an output stream. This improves code readability and simplifies debugging—imagine printing complex objects with just a few type-specific instructions rather than cumbersome member function calls.
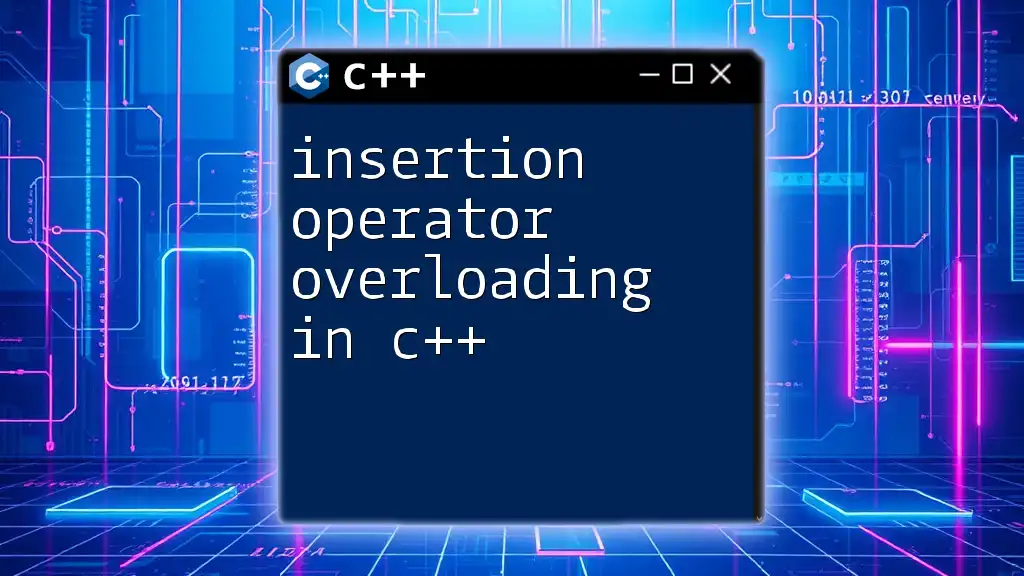
Understanding the Ostream Class
What is Ostream?
The `ostream` class in C++ is a part of the standard I/O library. It facilitates the output stream functionality, allowing you to send data to various output destinations, such as console or files. The `ostream` class is critical for displaying information and logs in your applications.
Components of Ostream
The `ostream` class consists of several key functions, including:
- `operator<<`: Used for outputting data.
- `flush()`: Flushes the output buffer.
- `precision(int)`: Sets the decimal precision for floating-point outputs.
These functions work together to provide a comprehensive toolset for formatted output.
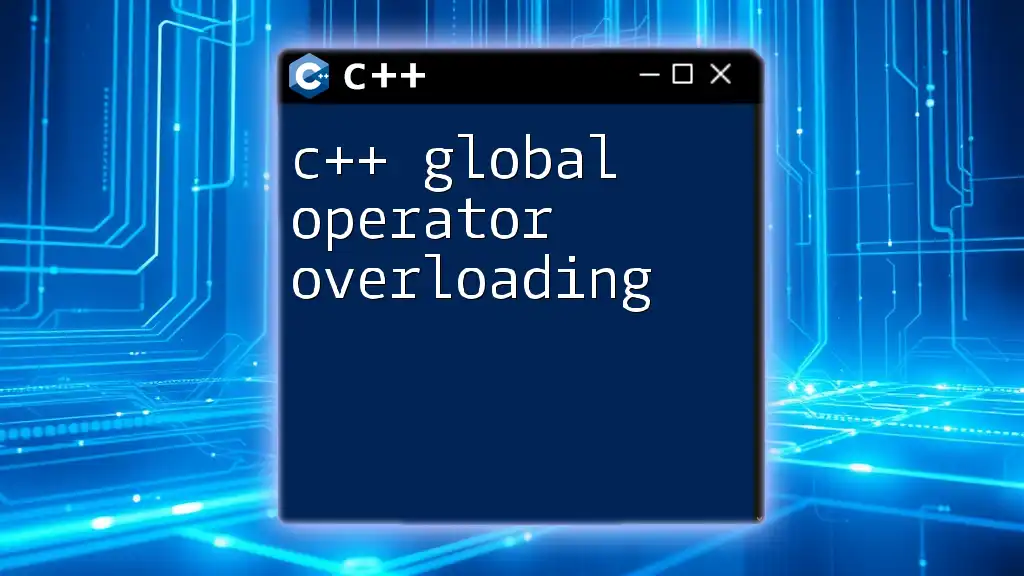
The Basics of Overloading `<<` Operator
What is the `<<` Operator?
The `<<` operator is primarily used for outputting data to streams. It’s a straightforward way to display different data types, and C++ allows you to extend this functionality to your custom types through operator overloading.
Syntax for Overloading the `<<` Operator
Overloading the `<<` operator in C++ involves creating a function that works with two parameters: an `ostream` reference and a constant reference to your class. The syntax is as follows:
std::ostream& operator<<(std::ostream &os, const YourClass &obj) {
// Implementation here
return os;
}
This method returns a reference to the `ostream`, allowing for chaining of outputs (e.g., `std::cout << obj1 << obj2;`).
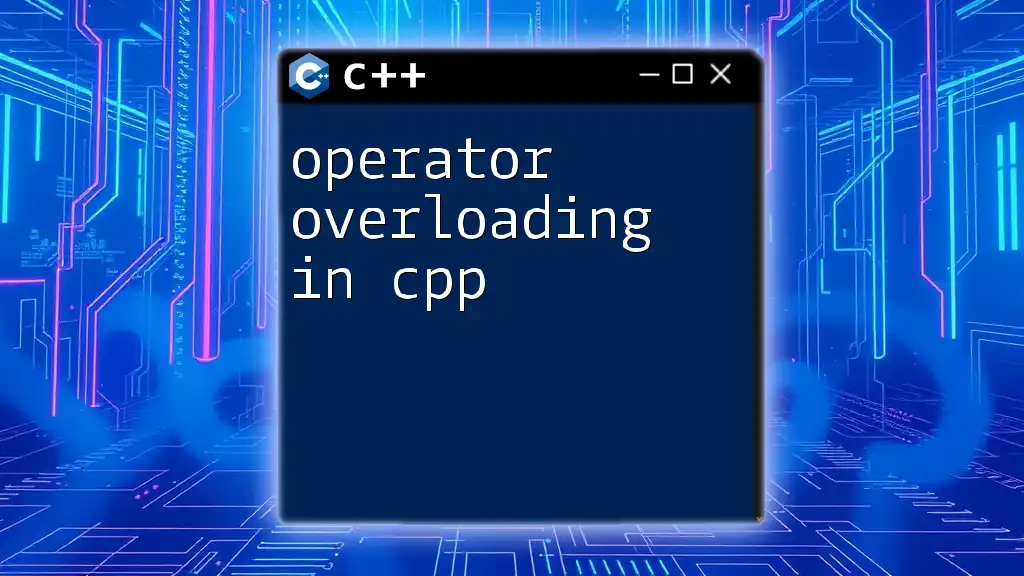
Step-by-Step Guide to Implementing Ostream Operator Overloading
Step 1: Defining a Custom Class
Start with defining a simple class. Here’s an example of a `Point` class that encapsulates a point in 2D space:
class Point {
public:
int x, y;
Point(int x, int y) : x(x), y(y) {}
};
Step 2: Overloading the `<<` Operator
Now, you’ll implement the `<<` operator for the `Point` class. This allows you to output `Point` objects directly to an output stream in a formatted manner.
std::ostream& operator<<(std::ostream &os, const Point &p) {
os << "(" << p.x << ", " << p.y << ")";
return os;
}
In this implementation, the `<<` operator is overloaded to output the `Point` object in the form of `(x, y)`, enhancing clarity.
Step 3: Using the Overloaded Operator
Finally, you can leverage the overloaded operator in your main program:
int main() {
Point p(3, 4);
std::cout << p; // Output: (3, 4)
return 0;
}
When you execute this code, the output is neatly formatted, making it clear and concise.
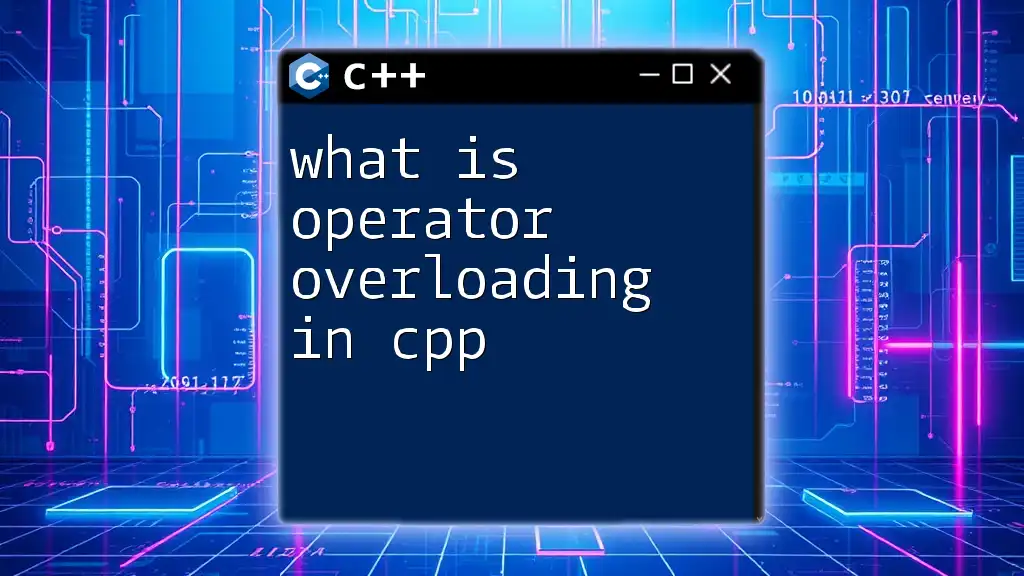
Common Pitfalls and Best Practices
Common Mistakes in Ostream Operator Overloading
While overloading the ostream operator can be simple, there are common mistakes to watch out for:
- Not returning `ostream&`: Remember to return the output stream reference to support chaining.
- Forgetting to handle output formatting: Proper formatting enhances readability, so make sure your output aligns with user expectations.
Best Practices for Overloading
When overloading operators, keep the following best practices in mind:
- Simplicity: Ensure that your overloads remain simple and easy to understand. Complex logic can detract from the readability of your class.
- Consistency: Make sure your operator overloading adheres to conventional behavior. For instance, the `<<` operator should consistently output a meaningful representation of your object's state.
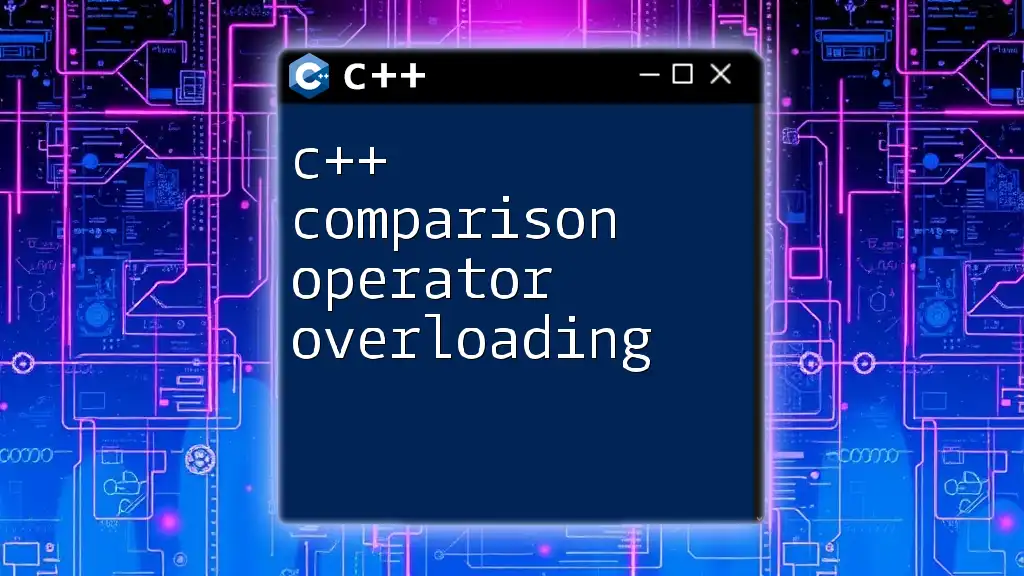
Conclusion
The importance of ostream operator overloading in C++ cannot be overstated. It allows you to represent your user-defined types in a manner that is both intuitive and effective, greatly enhancing the readability of your code. By following this guide, you can implement operator overloading in your own classes, paving the way for cleaner, more maintainable C++ code.
Additional Resources
For further reading on operator overloading, consider exploring C++ coding best practices and textbooks focused on advanced C++ concepts. The more familiar you become with these foundational topics, the better your proficiency in C++ will become.