The stream extraction operator (`>>`) in C++ is used to read data from input streams, such as `cin`, and store it into variables. Here's a code snippet demonstrating its usage:
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter a number: ";
cin >> number; // Using the stream extraction operator
cout << "You entered: " << number << endl;
return 0;
}
What is the Stream Extraction Operator?
The stream extraction operator in C++ is denoted by `>>`. It is a fundamental part of C++ input operations, allowing data to be extracted from input streams, such as `std::cin`. This operator is crucial for interacting with user input, enabling users to enter data which can then be processed by the program.
How the Stream Extraction Operator Works
The basic syntax for using the stream extraction operator is as follows:
std::cin >> variable;
In this syntax:
- `std::cin` refers to the standard input stream.
- `>>` is the extraction operator that reads data from the input stream.
- `variable` is where the extracted data will be stored.
When executed, this statement will wait for the user to input data and will store it in the specified variable after conversion to the appropriate type.
Using the Stream Extraction Operator
Basic Input with the Extraction Operator
Here’s a simple example that illustrates basic input using the stream extraction operator:
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter a number: ";
cin >> number;
cout << "You entered: " << number << endl;
return 0;
}
In this example:
- The program prompts the user to enter a number.
- Upon entering data and pressing Enter, the input is stored in the `number` variable.
- The entered number is then displayed back to the user.
Extracting Different Data Types
The stream extraction operator can be used to extract various data types:
- Integer Input: Works seamlessly for `int` type.
- Floating Point Input: It can also read decimal numbers (`float`, `double`).
- String Input: Extracting strings is slightly different, as it reads until the first whitespace.
For example:
double decimalNumber;
string text;
cout << "Enter a decimal number: ";
cin >> decimalNumber;
cout << "You entered: " << decimalNumber << endl;
cout << "Enter a string: ";
cin >> text; // Reads until the first whitespace
cout << "You entered: " << text << endl;
Handling Whitespaces and Input
The Role of Whitespaces
When using the stream extraction operator, it automatically skips leading whitespace characters, making it convenient for user input. This means you can enter numbers or strings with spaces, and the operator will correctly extract the input. For instance:
int a, b;
cout << "Enter two numbers separated by spaces: ";
cin >> a >> b; // Both numbers are successfully extracted, spaces ignored
However, if you want to read an entire line that contains spaces, you should use the `getline()` function instead of the extraction operator.
Common Issues with the Stream Extraction Operator
Input Failures
One of the most common pitfalls is a mismatch between the expected data type and the user's input. For example, if the user is prompted to enter a number and inputs text, this can lead to an error in input handling. When such an error occurs, the stream enters a fail state, which must be managed to avoid program crashes.
Here’s an example of error handling for input type mismatches:
int number;
cout << "Enter a number: ";
if (!(cin >> number)) {
cout << "Invalid input! Please enter a valid number." << endl;
// Clear error state
cin.clear();
// Discard invalid input
cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n');
}
In this snippet:
- `cin.clear()` resets the error state.
- `cin.ignore()` discards the invalid input, allowing the program to prompt for input again.
Stream State Management
It's essential to check if the extraction was successful. Here’s how you can do that:
if (cin >> number) {
cout << "You entered: " << number << endl;
} else {
cout << "Failed to read the number." << endl;
}
This ensures that you can react appropriately if input fails.
Overloading the Stream Extraction Operator
Custom Types and the Extraction Operator
In C++, you can also overload the stream extraction operator to work with user-defined types. This allows you to define how objects of your class should be read from an input stream.
Here’s a brief overview of how to implement this:
class Point {
public:
int x, y;
friend std::istream& operator>>(std::istream& is, Point& p);
};
std::istream& operator>>(std::istream& is, Point& p) {
is >> p.x >> p.y;
return is;
}
In this code, the `>>` operator is overloaded to read the `x` and `y` coordinates of a `Point` object from an input stream.
Example Usage of Overloaded Operator
You can now use this overloaded operator for input operations:
Point p;
cout << "Enter coordinates (x y): ";
cin >> p;
cout << "You entered: (" << p.x << ", " << p.y << ")" << endl;
Best Practices for Using the Stream Extraction Operator
To maximize the utility of the stream extraction operator, consider these best practices:
- Safety Tips: Always validate the input after attempting to extract it. This can prevent many runtime errors.
- Validate Input: Validate the data received using checks before proceeding with logic that depends on that data.
- Handle Edge Cases: Be prepared for various types of input, such as empty entries, non-numeric characters, or unexpected formats.
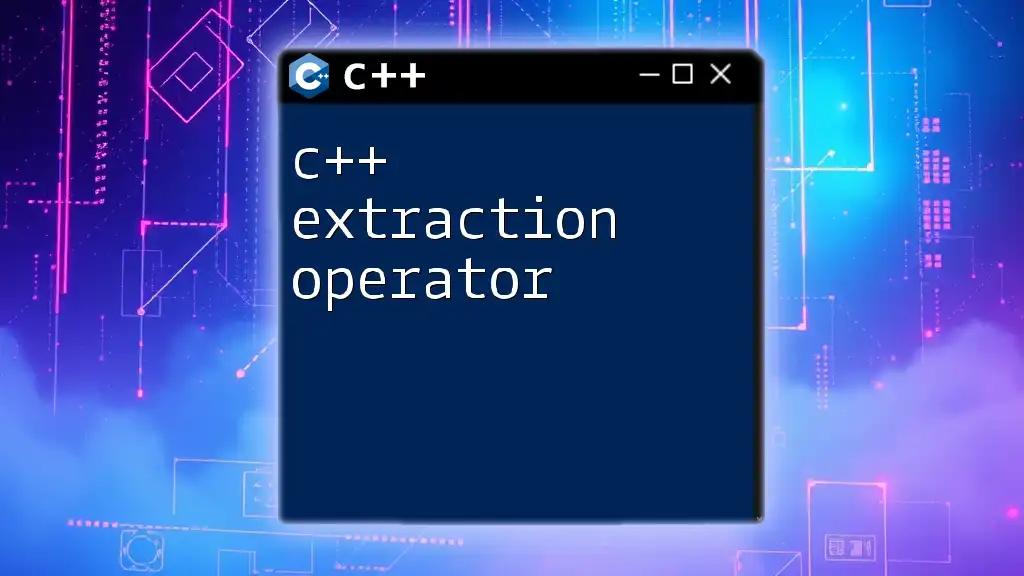
Conclusion
Understanding the stream extraction operator in C++ is crucial for effective input handling. By mastering this operator, you can create user-friendly applications that gracefully handle various inputs, improve data validation, and manage errors. Continuous practice, along with implementing overloading for custom types, will enhance your programming skills and efficiency in using C++.