Relational operators in C++ are used to compare two values, resulting in a boolean value indicating whether the comparison is true or false.
// Example of relational operators in C++
int a = 5;
int b = 10;
bool isEqual = (a == b); // Checks if a is equal to b
bool isNotEqual = (a != b); // Checks if a is not equal to b
bool isGreater = (a > b); // Checks if a is greater than b
bool isLess = (a < b); // Checks if a is less than b
bool isGreaterEqual = (a >= b); // Checks if a is greater than or equal to b
bool isLessEqual = (a <= b); // Checks if a is less than or equal to b
What Are Relational Operators?
Relational operators are essential tools in programming that allow developers to compare values. They play a critical role in decision-making processes, enabling the execution of specific code segments based on the results of these comparisons. In C++, relational operators evaluate the relationship between two operands and return a boolean value—either true or false.
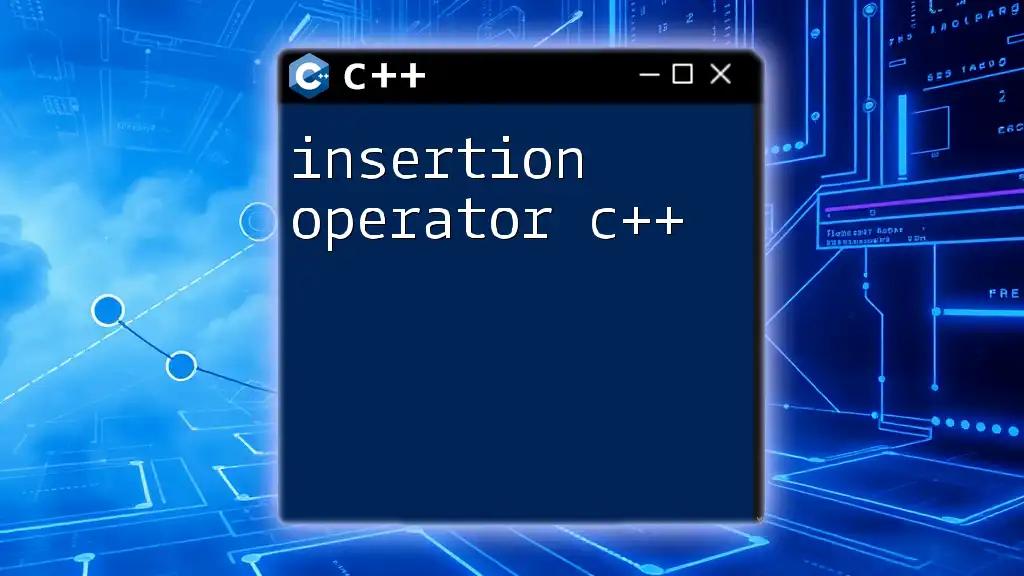
Overview of Relational Operators in C++
In C++, six primary relational operators can be used within your code:
- Equal to (==): Checks if two values are equal.
- Not equal to (!=): Checks if two values are not equal.
- Greater than (>): Checks if the left operand is greater than the right.
- Less than (<): Checks if the left operand is less than the right.
- Greater than or equal to (>=): Checks if the left operand is greater than or equal to the right.
- Less than or equal to (<=): Checks if the left operand is less than or equal to the right.
Understanding these operators is crucial for effective programming, as they form the basis of many logical conditions and flow control structures, including loops and conditional statements.
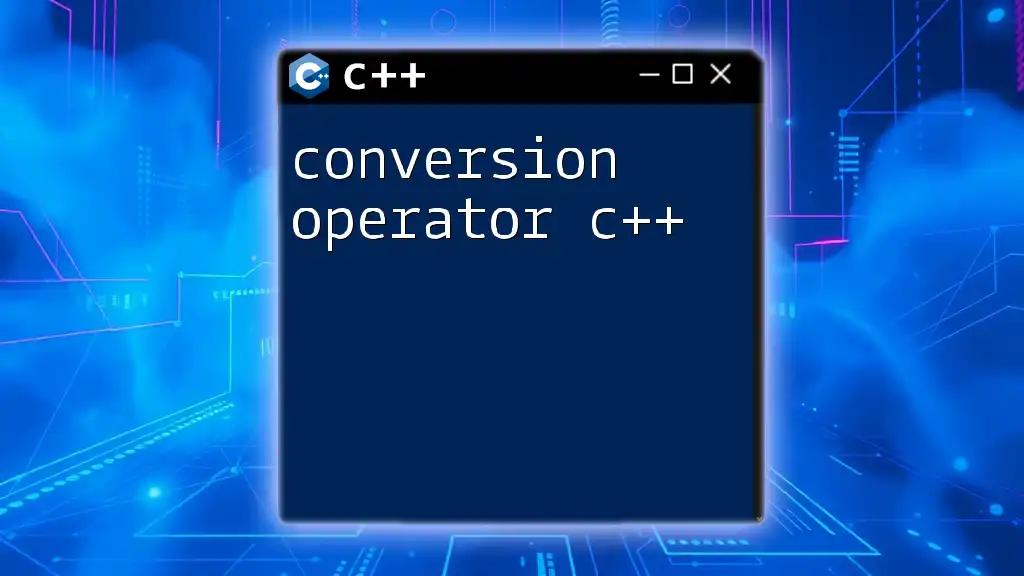
How Relational Operators Work
The Basic Concept
At its core, a relational operator compares two values to determine their relationship. For example, consider the following simple comparisons:
int a = 10;
int b = 20;
if (a < b) {
cout << "a is less than b" << endl;
}
This code will print "a is less than b" because the condition evaluates to true. The result of the relational operation can be used to control the flow of the program.
Data Types and Relational Operators
C++ relational operators are versatile and can be applied to various data types, including integers, floats, and even characters. Here's how they function across different types:
char x = 'A';
char y = 'B';
if (x < y) {
cout << "A comes before B" << endl; // This evaluates to true
}
Relational operators are designed to return true or false, making them essential in control statements regardless of the data type being compared.
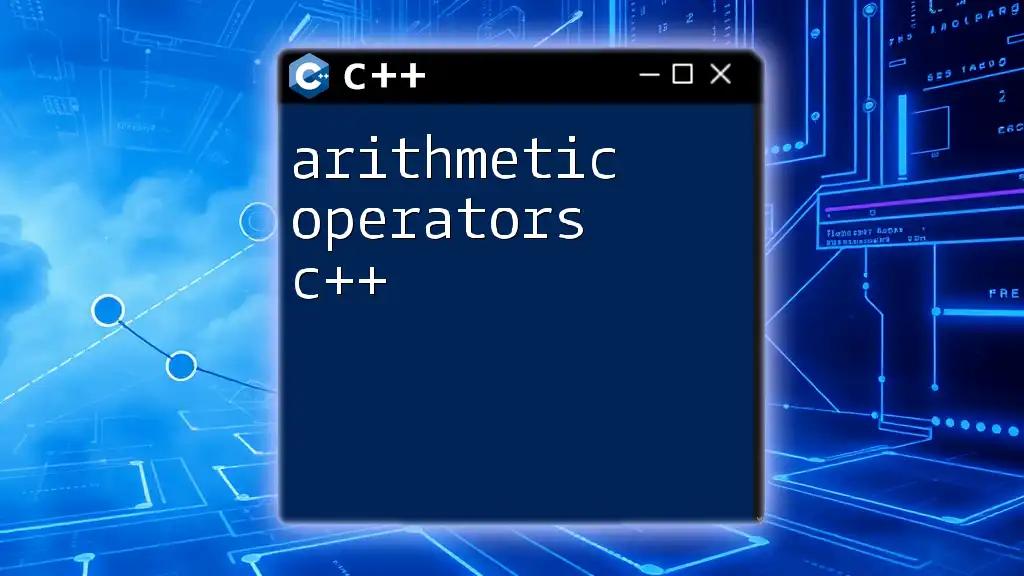
Practical Use Cases of Relational Operators
Decision-Making with if Statements
One of the most common applications of relational operators is within if statements. These operators help in making decisions based on certain conditions. Here’s an example using relational operators within an if statement:
int age = 18;
if (age >= 18) {
cout << "You are eligible to vote" << endl;
}
In this snippet, the program checks if age is greater than or equal to 18 to determine if a user is eligible to vote.
Loops and Relational Operators
Relational operators are also frequently used in loops to control iterations. For example, consider a for loop that prints values from 0 to 4, but only if they are greater than 2:
for (int i = 0; i < 5; i++) {
if (i > 2) {
cout << i << " is greater than 2" << endl; // This will print 3, 4
}
}
In this loop, the relational operator helps determine whether to execute the inner block, showcasing its powerful role in controlling program flow.
Compound Conditions
By combining relational operators with logical operators (AND, OR), you can create more complex conditions. For instance, if you want to check if a person is eligible for work based on age constraints:
int age = 20;
bool hasJob = true;
if (age >= 18 && age < 65) {
cout << "Eligible for work" << endl; // This evaluates to true
}
This code demonstrates how relational operators can work alongside logical operators to create compound conditions.
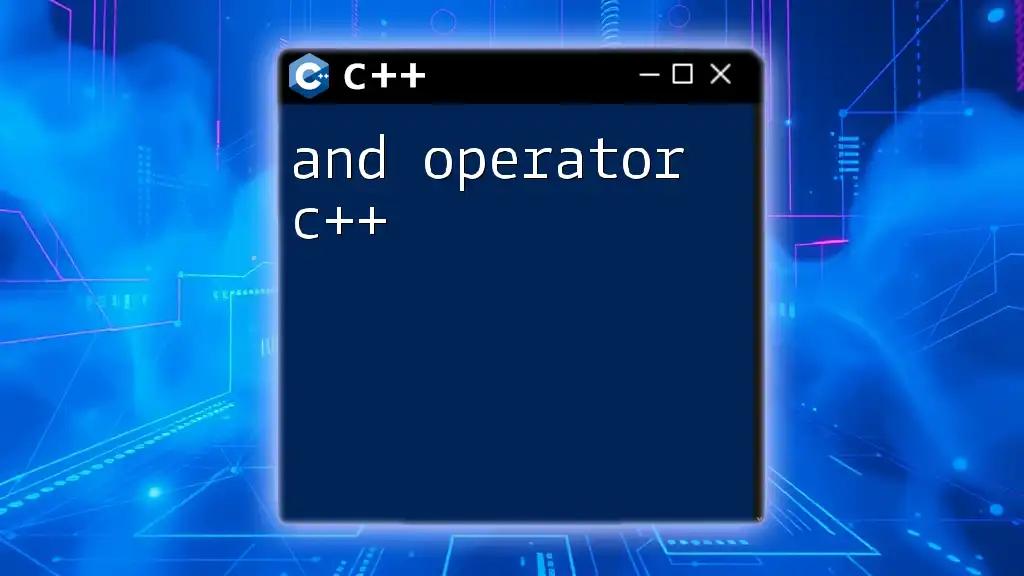
Contrast with Other Operators
Relational Operators vs. Arithmetic Operators
Understanding the difference between relational operators and arithmetic operators is essential. While relational operators compare values, arithmetic operators perform mathematical operations. Here’s an example that uses both types:
int height = 150; // in cm
if (height >= 120) {
cout << "Height is sufficient." << endl; // Evaluates if height is sufficient
}
In this example, the relational operator checks if a certain condition is met, while arithmetic operators can be used for calculations in other contexts.
Understanding Logical Operators
Logical operators complement relational operators by enabling complex logical expressions. For example, using both relational and logical operators together can yield comprehensive conditions:
bool hasPermission = true;
int age = 20;
if (hasPermission && (age >= 18)) {
cout << "Access granted!" << endl; // Will execute if both conditions are true
}
This code checks both the hasPermission and age conditions, allowing for more nuanced control over program behavior.

Common Errors and Troubleshooting
Common Mistakes with Relational Operators
A frequent mistake programmers encounter involves confusing the assignment operator (`=`) with the equality operator (`==`). For example, consider this faulty piece of code:
if (a = b) { // Incorrect usage: assignment instead of comparison
// This will always evaluate to true if b is non-zero
}
This mistake can lead to unexpected behavior, emphasizing the need to differentiate between the two operators clearly.
Debugging Techniques
When debugging code that uses relational operators, it's helpful to include print statements to trace the values being compared. For instance:
cout << "a: " << a << ", b: " << b << endl;
This demonstrates the current state of variables, making it easier to identify logic errors during comparison operations.
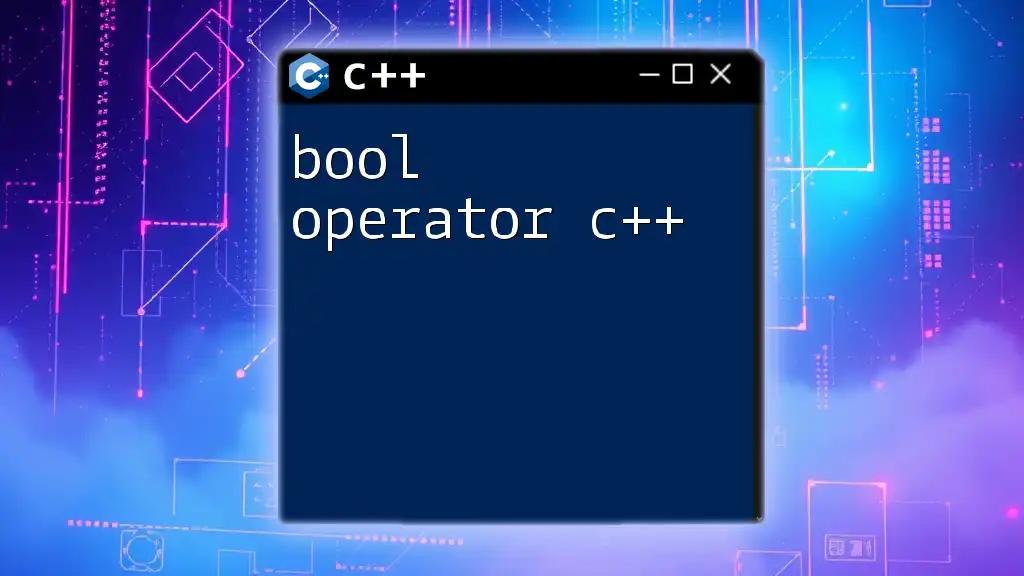
Advanced Applications of Relational Operators
Utilizing in Data Structures
Relational operators play a crucial role in data structure manipulations, such as sorting and searching within arrays or lists. Here’s a simple example of sorting elements using a relational operator:
#include <algorithm>
#include <vector>
#include <iostream>
int main() {
std::vector<int> arr = {5, 3, 8, 1};
// Sort using a relational operator
std::sort(arr.begin(), arr.end(), [](int a, int b) {
return a < b; // Using < for ascending order
});
for (int num : arr) {
std::cout << num << " "; // Will print sorted numbers
}
return 0;
}
In this example, the `<` relational operator determines how the sorting should occur, showcasing advanced applications in algorithms.
Contextual Applications in Functions
Another powerful use of relational operators is within functions that may take conditions as parameters. For example, you could create a function to compare two values:
bool compareValues(int a, int b) {
return a > b; // Returns true if a is greater than b
}
if (compareValues(10, 5)) {
cout << "10 is greater than 5" << endl;
}
This demonstrates how relational operators can be encapsulated in functions, allowing for cleaner and more reusable code.
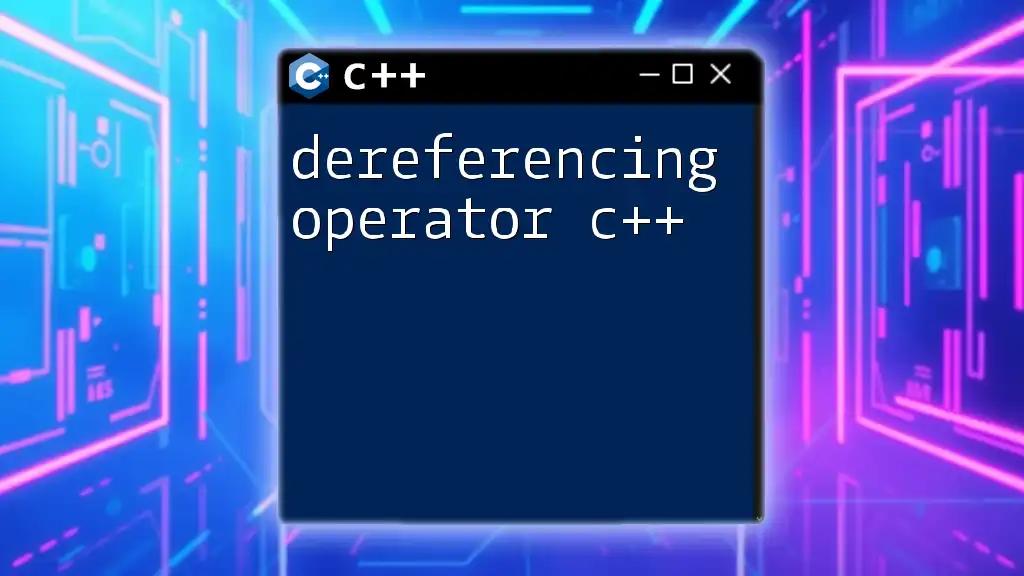
Conclusion
In summary, relational operators in C++ are fundamental tools that enhance decision-making capabilities within your code. From controlling flow with if statements to driving iterations in loops, they are indispensable in a programmer's toolkit. As you practice using these operators, you'll discover their profound impact on writing cleaner and more efficient code. To deepen your understanding, continue exploring resources that cater to C++ programming and relational operations specifically. Happy coding!