The casting operator in C++ allows you to convert a variable from one data type to another, ensuring proper type handling and compatibility in expressions. Here's a simple example of using the static_cast operator:
#include <iostream>
int main() {
double num = 5.5;
int convertedNum = static_cast<int>(num); // Casting double to int
std::cout << "Converted number: " << convertedNum << std::endl; // Output: Converted number: 5
return 0;
}
What are Casting Operators?
Casting operators are essential when it comes to converting one data type to another in C++. They enable developers to manipulate types, ensuring that operations between different data types can be performed effectively. This foundational concept is crucial for various programming scenarios, including polymorphism, interfacing with legacy code, and optimizing performance.
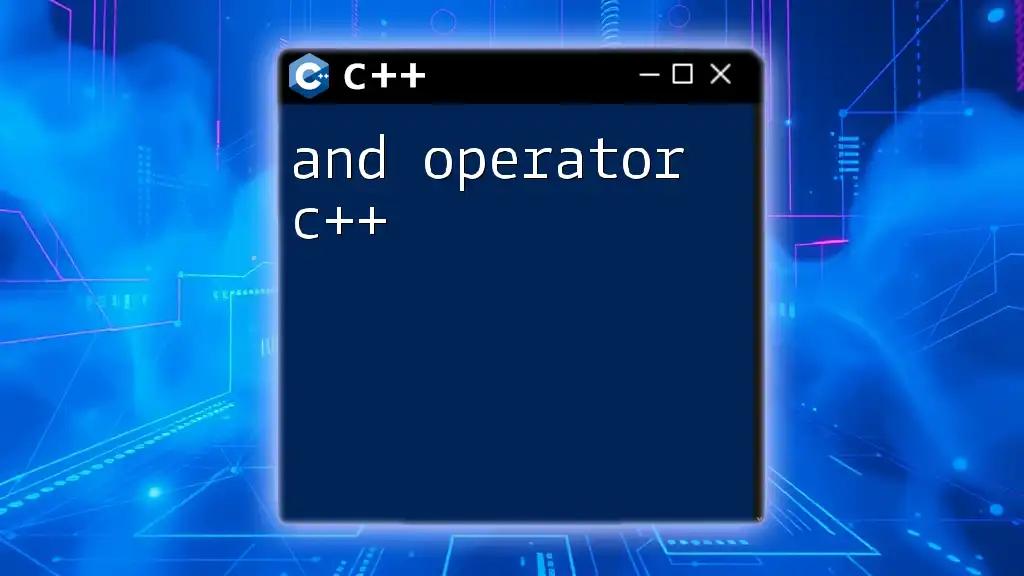
Understanding C++ Casting Operators
A C++ cast is primarily used to convert variables of one type into another type. This is crucial in a language that is heavily typed, as improper type assignments can lead to errors and undefined behaviors.
Types of Casting Operators in C++
C++ provides several casting operators, each tailored for specific scenarios:
Dynamic Cast
Dynamic cast is utilized mainly for safe downcasting in polymorphic class hierarchies. It checks the validity of the cast at runtime and returns a null pointer if the conversion fails, which helps ensure type safety.
-
Syntax and Requirements: To use `dynamic_cast`, the types involved in the cast must be pointers or references to polymorphic types (i.e., types with at least one virtual function).
-
Example Use Case: Here's an example demonstrating the use of `dynamic_cast`:
class Base { virtual void foo() {} }; class Derived : public Base { void foo() override {} }; Base* b = new Derived(); // Base pointer pointing to Derived object Derived* d = dynamic_cast<Derived*>(b); // Attempting to downcast if(d) { // d is safe to use as it successfully casted }
Static Cast
Static cast is the most commonly used cast in C++. It's used for conversions that are known to be safe at compile time, such as implicit conversions or conversions between related types.
-
Different Scenarios for Static Casting: You would typically use `static_cast` for conversions that do not involve complex relationships, such as converting an integer to a double or between class hierarchies that are statically verifiable.
-
Example: Here's a simple example of using `static_cast` for type conversion:
int a = 10; double b = static_cast<double>(a); // Safe conversion from int to double
Const Cast
Const cast is particularly useful when dealing with constant data. It allows you to add or remove the `const` modifier from a variable.
-
Importance of Const Correctness: Const-correctness prevents unintended modifications to data, which helps avoid bugs in large codebases. However, sometimes you may need to modify a constant value in specific situations.
-
Example: Here’s how you can use `const_cast`:
const int a = 20; int* b = const_cast<int*>(&a); // Removing const-ness *b = 30; // This can lead to undefined behavior if 'a' is used afterwards
Reinterpret Cast
Reinterpret cast is a powerful yet dangerous tool that allows direct conversion between any pointer type. It can change the interpretation of a bit pattern, effectively allowing any pointer to become any other type.
-
Risks and Precautions: Using `reinterpret_cast` requires caution because it can lead to undefined behavior, particularly if the object types being cast do not match, or if the alignment is incorrect.
-
Example of Reinterpret Cast Usage: Here’s a simple example demonstrating its potential use:
long p = 123456; int* ptr = reinterpret_cast<int*>(p); // Casting a long to a pointer type
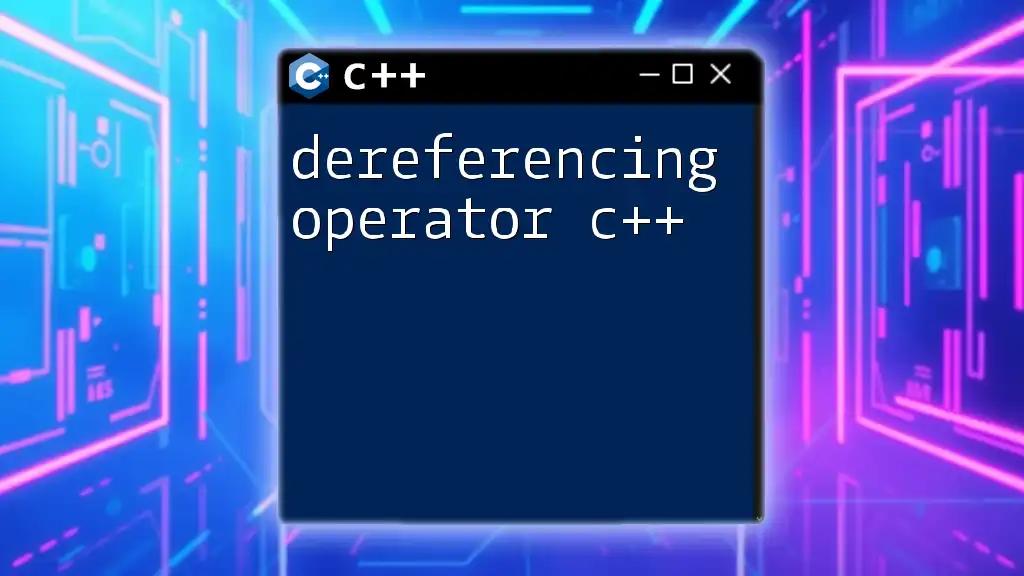
Comparing the Casting Operators
When selecting a casting operator, it's essential to understand when to use each type:
- Dynamic Cast: Ideal for downcasting when polymorphism is in play. Always prefer this when you can due to its safety checks.
- Static Cast: Use this for conversions that are well-defined and safe at compile time. It offers better performance than `dynamic_cast`.
- Const Cast: Best for modifying the `const` qualifier on data types when necessary. However, be wary of changing constants unexpectedly.
- Reinterpret Cast: Reserve this for low-level programming or specific performance cases, as it bypasses the type system.
In general, safety versus performance should guide your choice; prefer safer casts if performance is not an immediate concern.
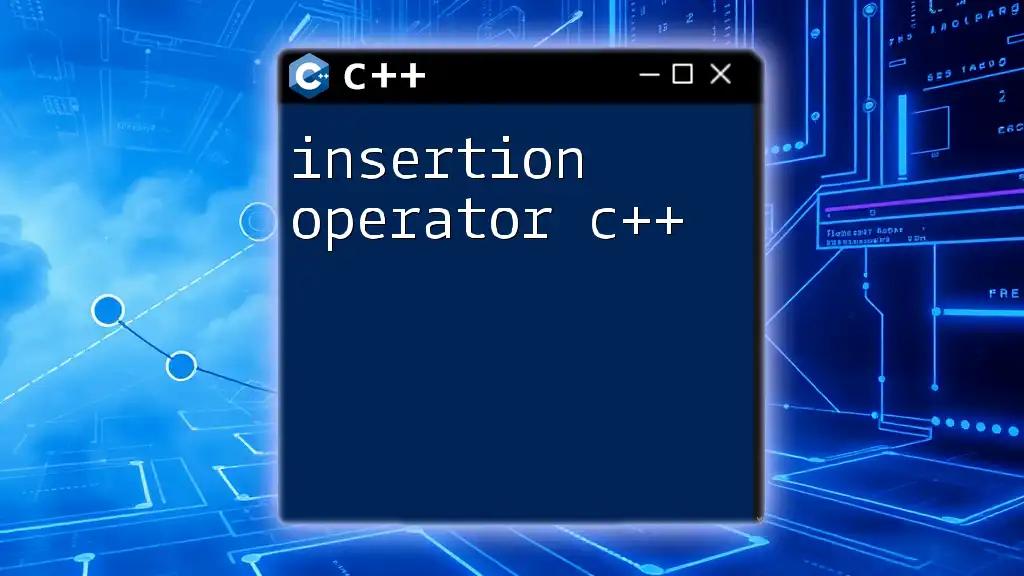
Best Practices with C++ Casting Operators
Being cautious with casting is vital for writing robust, maintainable code:
-
Avoid Common Pitfalls:
- Always use `dynamic_cast` in polymorphic classes to avoid invalid casts.
- Use `static_cast` for straightforward conversions but ensure they are safe.
-
Writing Clean and Safe Code:
- Make it a habit to minimize casting where possible. If you require frequent casts, it might indicate design issues.
- Utilize smart pointers and object-oriented principles to help manage type safety and reduce the need for explicit casts.
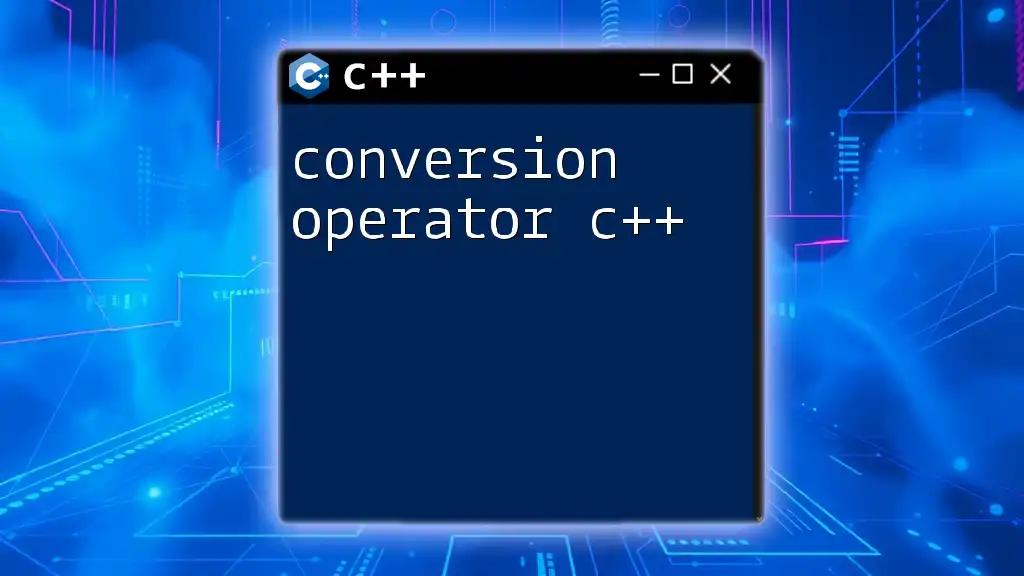
Conclusion
Casting operators in C++ are powerful tools that help manage type conversions, but they must be used with care. Each casting operator serves a distinct purpose, from the safety of `dynamic_cast` in polymorphic scenarios to the low-level freedom granted by `reinterpret_cast`. Understanding these operators and their applications will enable you to write cleaner and safer code in your C++ programming endeavors.

Additional Resources
Here are some suggested resources for further exploration of C++ casting and programming practices:
- Books on C++ programming and type safety.
- Websites such as cppreference.com and learncpp.com that provide in-depth C++ tutorials.
- Member communities in platforms like Stack Overflow for discussing casting-related queries and seeking advice.
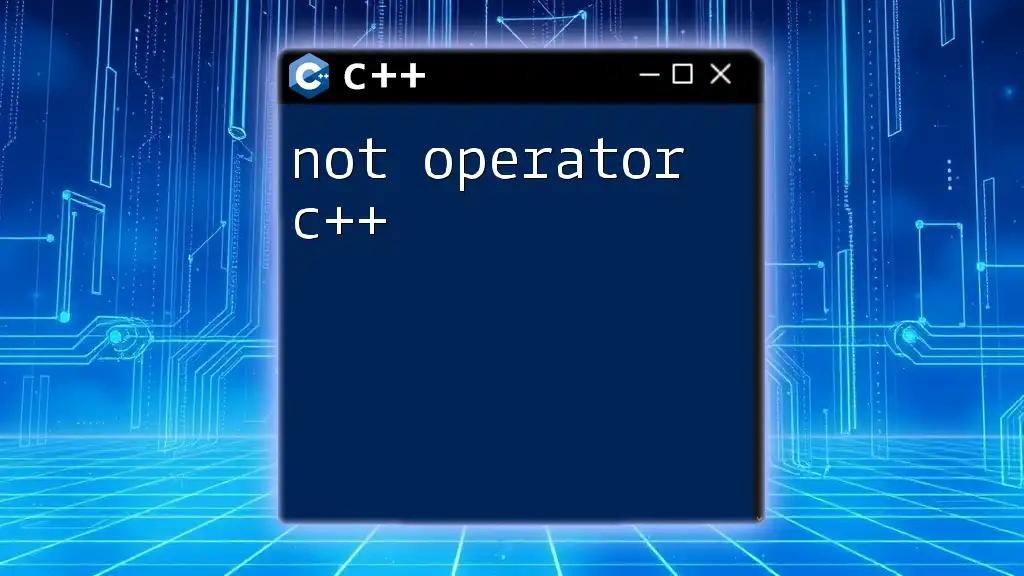
FAQs on C++ Casting Operators
While delving into casting operators in C++, several commonly asked questions arise:
- What is the difference between `dynamic_cast` and `static_cast`?
- Is it safe to use `reinterpret_cast`?
- When should I avoid using casting operators altogether?
By thoroughly understanding casting operators, you will enhance your programming skills and improve your C++ code's reliability and efficiency.