The C++ extraction operator (`>>`) is used to read input from streams, such as `std::cin`, and can be overloaded for user-defined types to facilitate custom input handling.
#include <iostream>
int main() {
int number;
std::cout << "Enter a number: ";
std::cin >> number; // Using the extraction operator
std::cout << "You entered: " << number << std::endl;
return 0;
}
What is the C++ Extraction Operator?
Definition
The C++ extraction operator, denoted as `>>`, is a key operator used in input streams. It is primarily utilized to read data from standard input, such as the keyboard, but can also be integrated with file streams. The extraction operator is fundamental in C++ for obtaining user input or reading data from files, making it a cornerstone of input handling in the language.
Purpose
The primary purpose of the extraction operator is to facilitate the reading of data from input streams. It allows programmers to extract values from a stream and store them in variables. This functionality is essential for interactive applications where user data needs to be processed in real time, as well as for batch processing of data from files.
Common Use Cases
The C++ extraction operator appears in various scenarios, including:
- Reading User Input: It is frequently used to capture information from users in console applications.
- File Handling: When reading structured data from files, in conjunction with file streams.

Syntax of the Extraction Operator
Basic Syntax
The basic syntax for using the C++ extraction operator is straightforward:
std::cin >> variable;
Supported Data Types
The extraction operator supports an array of primitive data types, allowing for versatile input operations. You can read integers, floating-point numbers, strings, and characters seamlessly. For example, here's how multiple data types can be read from standard input:
int age;
float height;
std::cin >> age >> height;
In this snippet, the program captures an integer for `age` and a `float` for `height`, demonstrating how the extraction operator efficiently handles multiple variables in one line.
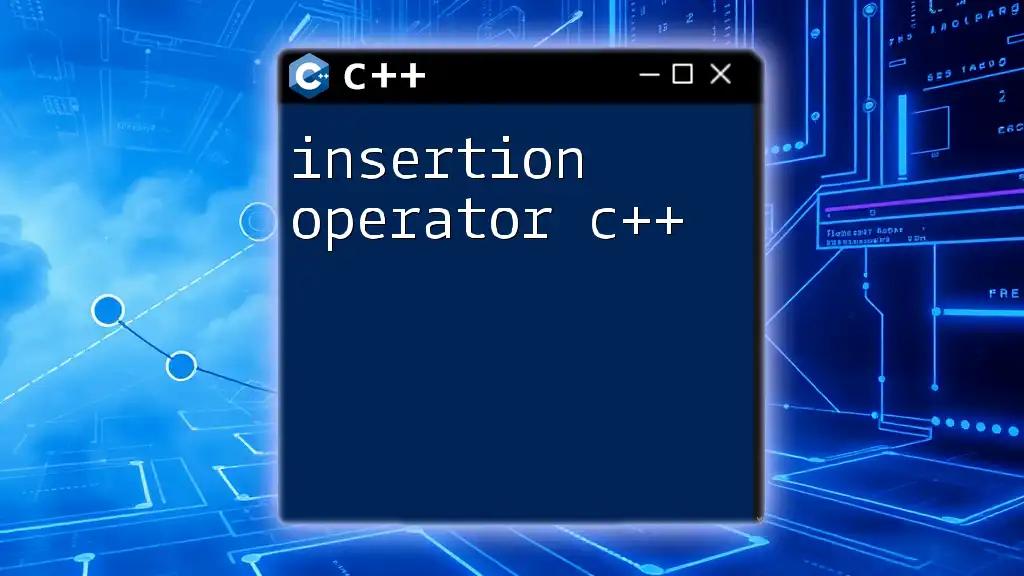
How the Extraction Operator Works
Input Streams
The extraction operator operates with input streams, which are crucial for reading data. Specifically, `std::istream` is the class responsible for input stream operations in C++. When you use the `>>` operator, it interacts directly with a stream object (like `std::cin` or file input streams) to process user input or data.
Flow of Data
Understanding the internal workflow of the extraction operator is vital:
- Reading from Input Buffer: The operator reads data from the input buffer that is generated when the user types data and presses enter.
- Type Conversion and Storage: It converts the input data into the specified variable type and stores it in the given variable.
- Error Handling: If the extraction operator encounters invalid input (e.g., entering text when expecting a number), it sets a fail state on the stream.
Example of Input Handling
Here's a robust example demonstrating how to handle input effectively while dealing with potential errors:
#include <iostream>
#include <limits>
int main() {
int number;
std::cout << "Enter a number: ";
while (!(std::cin >> number)) {
std::cin.clear(); // Clear the error flag
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n'); // Ignore invalid input
std::cout << "Invalid input. Please enter a number: ";
}
std::cout << "You entered: " << number << std::endl;
return 0;
}
In this example, the program prompts the user for a number. If the user enters an invalid input, it clears the error state and discards the invalid data, allowing the program to continue until valid input is received.

Overloading the Extraction Operator
Why Overload Operators?
Operator overloading in C++ allows developers to redefine the behavior of operators for user-defined types. This capability enhances the usability of custom classes, enabling them to seamlessly integrate with standard input/output practices.
How to Overload the Extraction Operator
To overload the extraction operator for a custom class, you typically follow these steps:
- Create a class with relevant member variables.
- Define a friend function that overloads the `>>` operator. This function should handle the input for the class's member variables.
Here is a comprehensive example that illustrates how to perform this:
#include <iostream>
#include <string>
class Person {
public:
std::string name;
int age;
friend std::istream& operator>>(std::istream &in, Person &p) {
std::cout << "Enter name: ";
in >> p.name;
std::cout << "Enter age: ";
in >> p.age;
return in;
}
};
int main() {
Person p;
std::cin >> p;
std::cout << "Name: " << p.name << ", Age: " << p.age << std::endl;
return 0;
}
In this example, the `Person` class overloads the `>>` operator. When an object of `Person` is inputted, the program prompts the user to enter the name and age, demonstrating how the extraction operator can be customized for specific types.
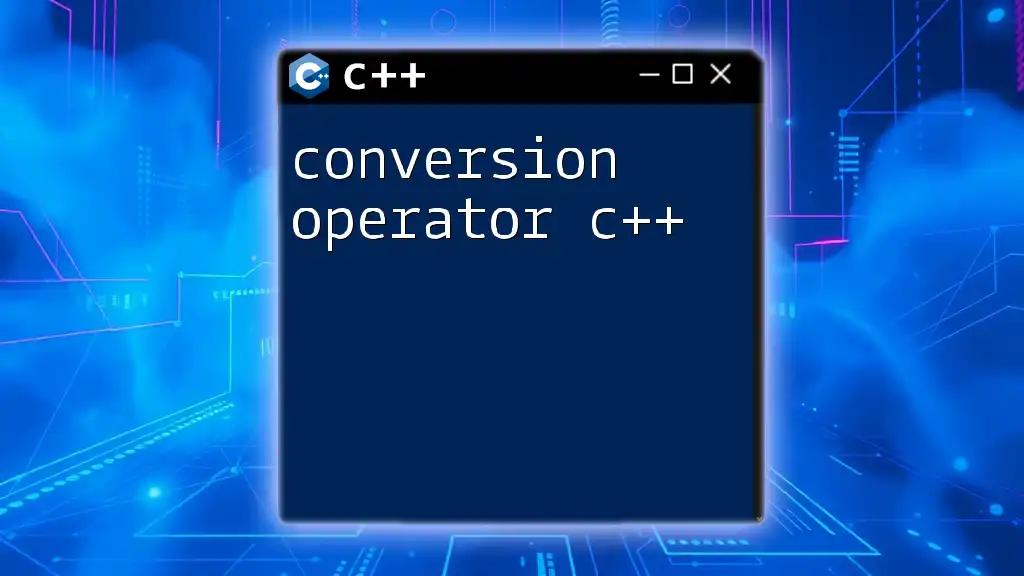
Best Practices for Using the Extraction Operator
Input Validation
It’s crucial to validate user input to avoid unexpected behavior or program crashes. Always check the input to ensure that the data being read matches the expected type. This practice prevents runtime errors and enhances the robustness of your applications.
Handling Stream States
Managing stream states effectively is significant when using the extraction operator. You can make use of `std::cin.clear()` to reset the stream state and `std::cin.ignore()` to discard invalid input from the buffer. Proper stream state management ensures that your program can recover from errors and continue functioning as intended.
Efficiency Considerations
When reading data, especially in performance-critical applications, consider the implications of excessive input operations. Buffering strategies and reading input in bulk when feasible can improve efficiency.

Common Pitfalls and How to Avoid Them
Not Checking for Input Failure
Neglecting to handle input failures can lead to bugs that are difficult to trace. Always check if an extraction operation was successful using `std::cin.fail()` to ensure reliable program execution.
Misuse with Complex Data Types
When using the extraction operator with complex or structured data types, ensure meticulous management of how the data is read and parsed. This is particularly important when the data consists of multiple fields or requires specific formatting.

Conclusion
Understanding the C++ extraction operator is essential for effective input handling in your applications. This operator not only simplifies the process of reading user input but also allows for extensibility through operator overloading for custom types. By employing best practices and avoiding common pitfalls, you can ensure that your input operations are both robust and efficient. Experimenting with examples and writing your own classes will deepen your understanding and mastery of C++ input handling techniques.

Additional Resources
For further knowledge, refer to official C++ documentation and reputable C++ programming books. Engaging in online courses and community forums can also provide valuable insights and support.

FAQs
What happens if I input a wrong data type?
If a wrong data type is entered, such as a character when an integer is expected, the extraction operation will fail, and the input stream will enter a fail state. You should handle this state appropriately to prompt users for valid input.
Can I use the extraction operator with user-defined types?
Yes, you can overload the extraction operator for user-defined types, allowing for customized input handling similar to built-in types.
What library do I need to include to use the extraction operator?
To use the extraction operator, include the `<iostream>` header, as it defines the stream classes and operators used for input and output.
How do I handle multiple data inputs efficiently?
You can prompt and read multiple inputs in a single line using the extraction operator, as demonstrated in the supported data types section. For structured data or instances requiring complex input management, consider reading data in a buffered manner or implementing custom parsing strategies.
By adhering to the insights provided in this article, you'll develop a deeper comprehension of the C++ extraction operator and its usage, positioning yourself to create more effective input-handling applications.