The C++ arrow operator (`->`) is used to access members of an object through a pointer, enabling you to dereference the pointer and access its attributes or methods in a concise manner.
class Point {
public:
int x, y;
Point(int x, int y) : x(x), y(y) {}
};
Point* p = new Point(5, 10);
std::cout << "X: " << p->x << ", Y: " << p->y << std::endl; // Outputs: X: 5, Y: 10
Introduction to the C++ Arrow Operator
What is the Arrow Operator?
The C++ arrow operator (`->`) is a powerful feature of the language that allows you to access members (variables and functions) of a class or structure via a pointer to that class or structure. This operator plays a crucial role in object-oriented programming, enabling encapsulation and abstraction while providing easy member access.
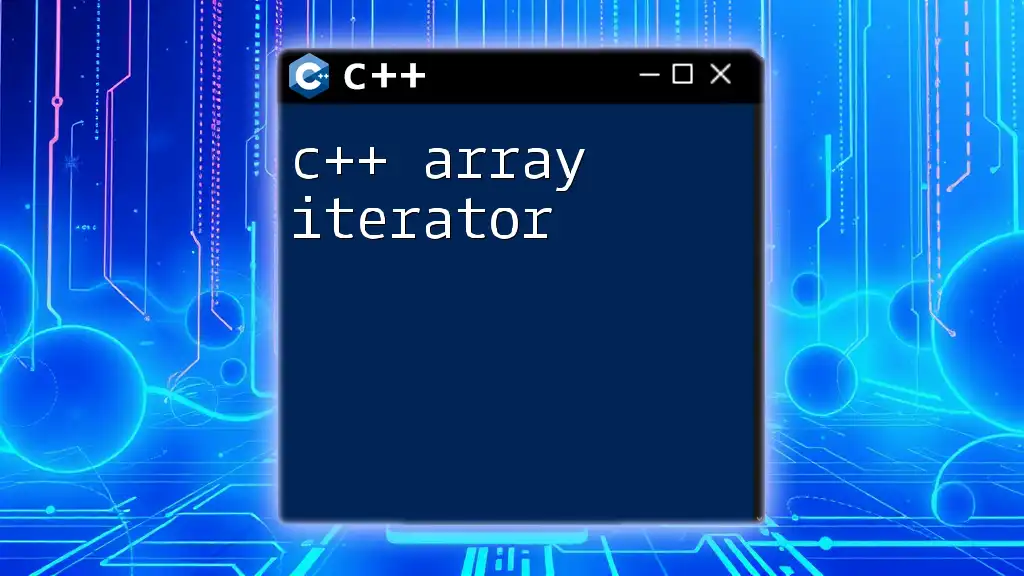
Understanding Pointers and References
What are Pointers?
Pointers are variables that store the memory address of another variable. They are vital for dynamic memory management and enable the creation of complex data structures. To illustrate pointers, consider the following example:
int a = 10;
int* p = &a; // Pointer p holds the address of a
std::cout << "Value of a: " << *p << std::endl; // Dereference p to get the value of a
In this example, `p` is a pointer to `a`, and using the dereference operator (``), we can access the value of `a` through `p`.*
What are References?
References provide an alternative way to refer to a variable. Unlike pointers, references must be initialized when declared and cannot be reassigned later. Here's how you can define a reference:
int b = 20;
int& ref = b; // Ref is a reference to b
std::cout << "Value of b: " << ref << std::endl; // Access b via reference
References act like aliases for the variables they reference, making code clearer and safer by avoiding null pointer issues.
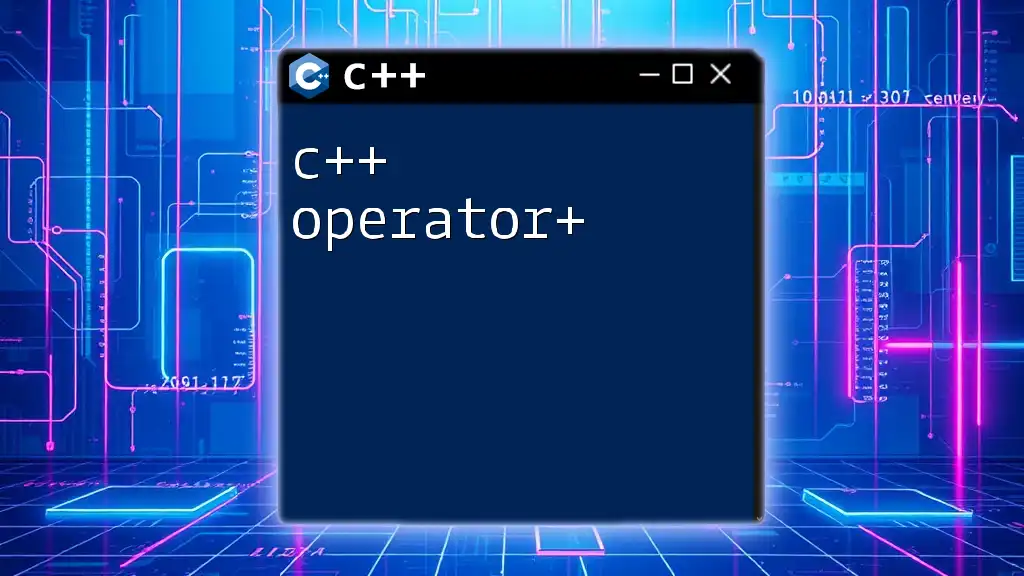
The Basics of the Arrow Operator in C++
Syntax of the Arrow Operator
The syntax for using the C++ arrow operator is straightforward: `pointer->member`. Here, `pointer` is a pointer to a class or structure, and `member` is the variable or function you want to access. Consider the following example:
struct Point {
int x;
int y;
};
Point* p = new Point{10, 20};
std::cout << "X: " << p->x << ", Y: " << p->y << std::endl;
In this example, `p->x` accesses the `x` member of the `Point` structure using the arrow operator.
When to Use the Arrow Operator
The arrow operator is particularly useful when working with pointers to objects. If you have an instance of a class or structure and you manage it through pointers, the arrow operator allows direct member access. Here's a comparison illustrating when to use the arrow operator versus the dot operator (`.`):
Point pt{5, 10}; // Direct instance of Point
std::cout << pt.x << std::endl; // Using dot operator
Point* pPoint = new Point{5, 10}; // Pointer to Point
std::cout << pPoint->x << std::endl; // Using arrow operator
This distinction is essential for writing clear and efficient C++ code.
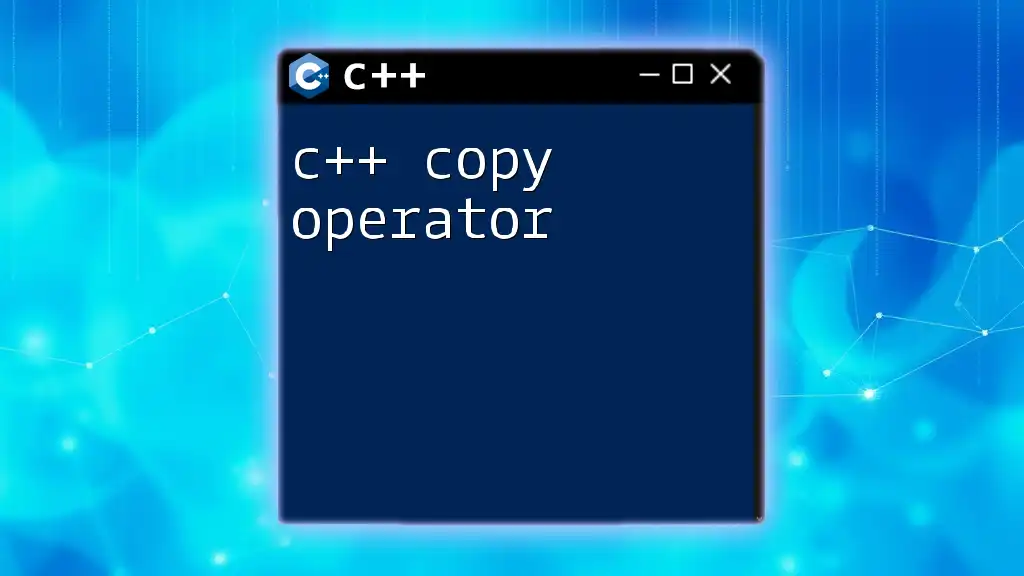
The Role of the Arrow Operator with Classes
Use with Class Member Functions
The arrow operator is often used to invoke member functions of a class through a pointer. Consider the following class definition:
class Circle {
public:
int radius;
Circle(int r) : radius(r) {}
int getArea() {
return 3.14 * radius * radius;
}
};
Circle* c = new Circle(5);
std::cout << "Area: " << c->getArea() << std::endl;
Here, `c->getArea()` uses the arrow operator to call the member function `getArea()` of the `Circle` class. This is a common pattern in C++ programming, especially when handling dynamic objects.
![Unlocking the C++ [] Operator: A Step-by-Step Guide](/images/posts/c/cpp-operator.webp)
The Arrow Operator in Smart Pointers
What are Smart Pointers?
Smart pointers are advanced pointers provided by C++ to help manage dynamic memory and prevent memory leaks. They automatically handle memory deallocation and come in various forms, such as `std::unique_ptr` and `std::shared_ptr`.
Using Arrow Operator with Smart Pointers
Smart pointers can also utilize the arrow operator for accessing members. Here’s an example using `std::shared_ptr`:
#include <memory>
std::shared_ptr<Circle> sc = std::make_shared<Circle>(5);
std::cout << "Area using smart pointer: " << sc->getArea() << std::endl;
In this snippet, `sc->getArea()` accesses the member function of the `Circle` instance managed by the smart pointer. This demonstrates how modern C++ has streamlined memory management while preserving the utility of the arrow operator.
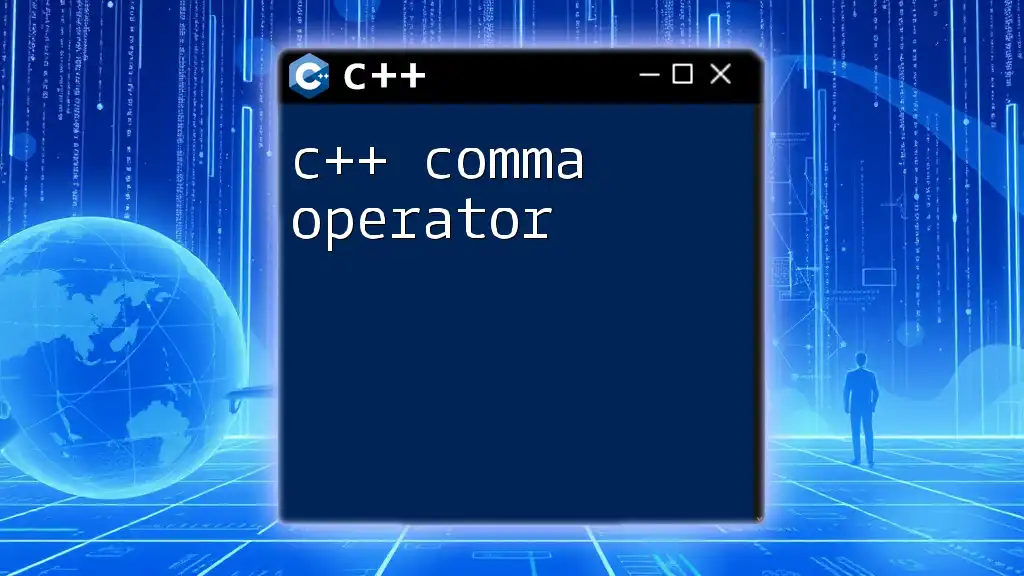
Advanced Concepts with the Arrow Operator
Arrow Operator with Structs and Classes
The arrow operator seamlessly applies to both structs and classes. For a deeper understanding, here’s an example that uses a linked list structure:
struct Node {
int data;
Node* next;
};
Node* head = new Node{1, nullptr};
head->next = new Node{2, nullptr};
Here, we define a linked structure where `head` points to the first node. The arrow operator is crucial for accessing the `next` member and linking nodes.
Challenges and Common Mistakes
While using the arrow operator, common pitfalls can arise, particularly when dealing with pointers. One such example is dereferencing a null pointer, which can lead to runtime errors:
Node* nullNode = nullptr;
std::cout << nullNode->data; // This will result in a segmentation fault
To avoid such mistakes, always ensure your pointer is valid before using the arrow operator.
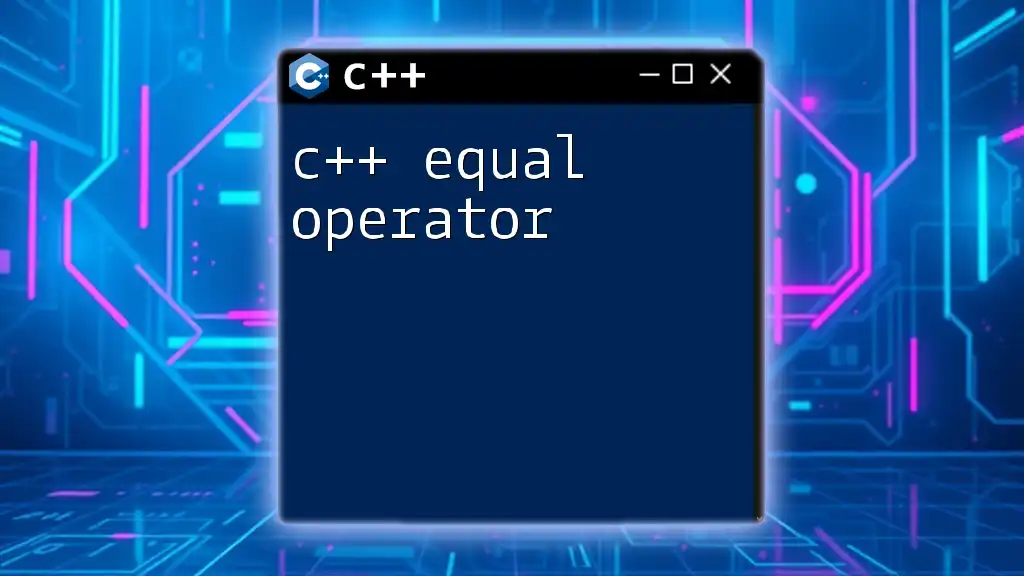
Conclusion
The C++ arrow operator is a key tool within the language that enables efficient member access for pointers to classes and structures. Understanding when and how to use it correctly not only enhances your programming skills but also helps in writing cleaner and safer code. As you grow in your C++ journey, practicing these concepts will solidify your understanding and improve your coding proficiency in object-oriented programming.
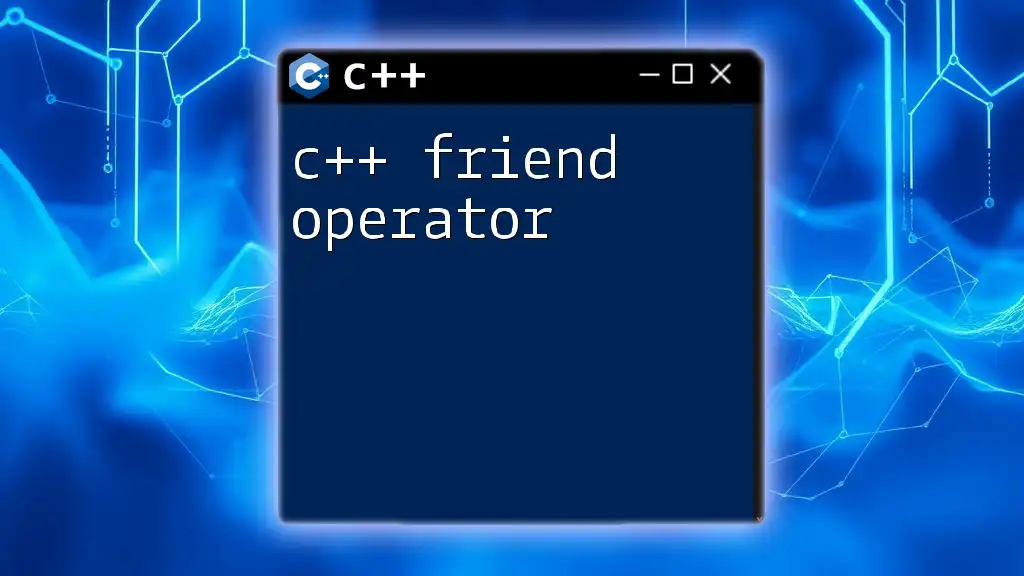
Further Resources and Reading
For those wishing to expand their knowledge further, various online tutorials, books, and documentation can provide deeper insights into pointers, memory management, and advanced C++ programming techniques.
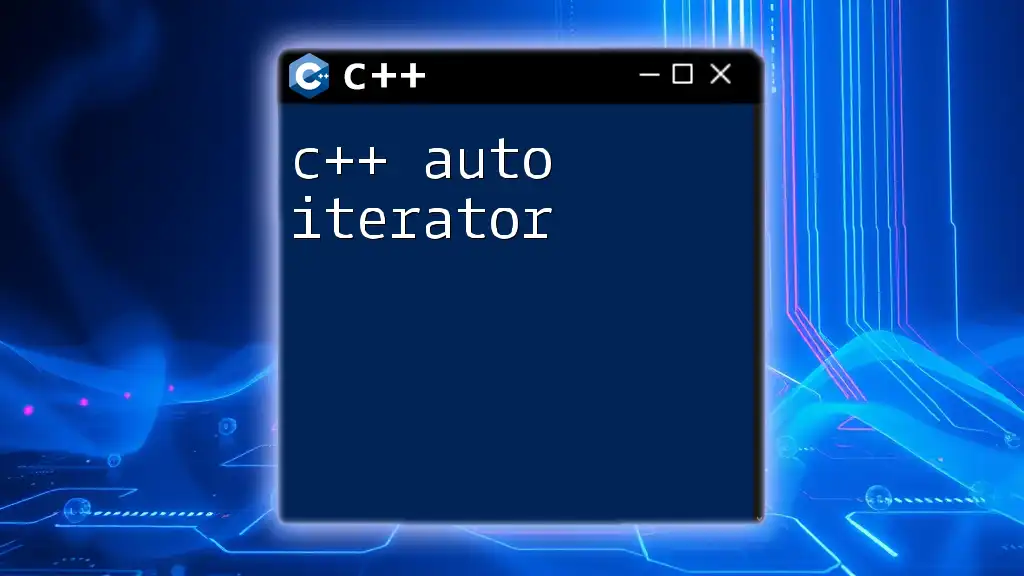
Call to Action
Now that you have a comprehensive understanding of the C++ arrow operator, it’s time to put your knowledge into practice. Consider engaging in coding challenges or exercises focusing on pointers to deepen your mastery of this essential C++ feature. Happy coding!