C++ comparison operator overloading allows you to define custom behaviors for comparison operators (such as `>`, `<`, `==`, etc.) so that objects of your user-defined classes can be compared directly.
Here's a code snippet demonstrating how to overload the `==` operator for a simple `Point` class:
class Point {
public:
int x, y;
Point(int x, int y) : x(x), y(y) {}
bool operator==(const Point& other) const {
return (x == other.x) && (y == other.y);
}
};
Understanding Comparison Operators
What are Comparison Operators?
In C++, comparison operators enable you to evaluate the relationship between two objects or values. These operators return a boolean value—either `true` or `false`—determining how the elements compare to one another. The most common comparison operators include:
- `==` for equality
- `!=` for inequality
- `<` for less than
- `<=` for less than or equal to
- `>` for greater than
- `>=` for greater than or equal to
By overloading these operators, you allow custom classes to be compared in a manner that aligns with the intended logic of the objects’ properties.
Use Cases for Comparison Operators
The primary use cases for overloaded comparison operators include:
-
Comparing Objects: By defining how two instances of a class compare, you can create intuitively understandable logic, essential for types that possess meaningful comparisons.
-
Sorting Collections: In data structures like arrays or vectors, overloaded comparison operators facilitate the sorting and ordering of complex objects.
-
Implementing Business Logic: Specific applications in business scenarios may require complex types to be compared easily. For instance, comparing financial transactions or product specifications can lead to more readable and maintainable code.
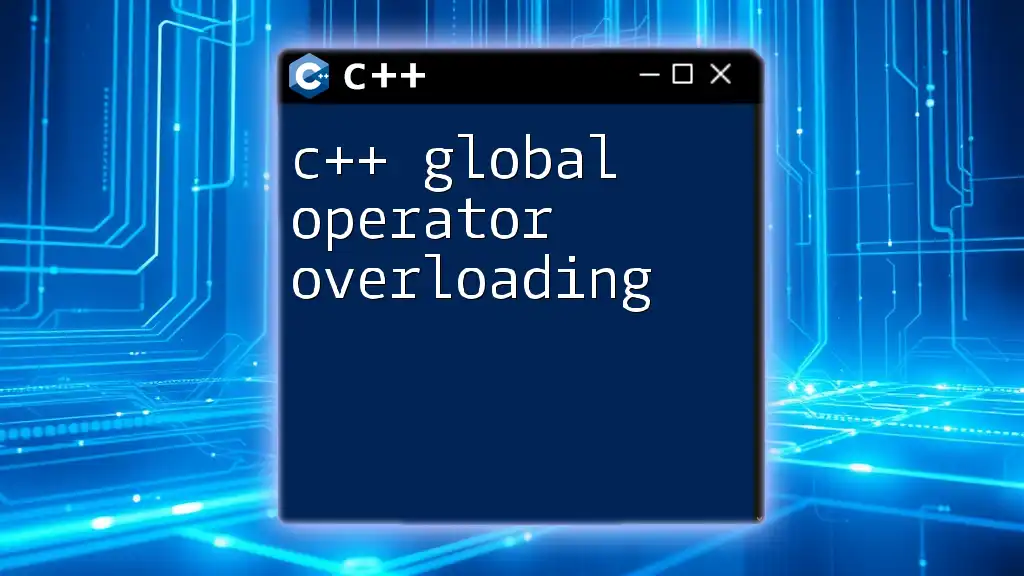
Basics of Operator Overloading
What is Operator Overloading?
Operator overloading allows developers to redefine the way operators work for user-defined types. This means you can implement custom behavior for operators such as arithmetic, relational, and bitwise operators. By leveraging operator overloading, you create a more expressive and modular codebase.
Syntax of Operator Overloading
The syntax for overloading an operator involves defining a function whose name is the operator you wish to overload. The function usually appears in the class definition or can be defined outside the class. Here’s an example of basic operator overloading:
class Example {
public:
int value;
Example(int v) : value(v) {}
// Overloading the '+' operator
Example operator+(const Example& other) {
return Example(this->value + other.value);
}
};
In this instance, when you use the `+` operator with instances of `Example`, it sums their values in a manner that is both intuitive and readable.
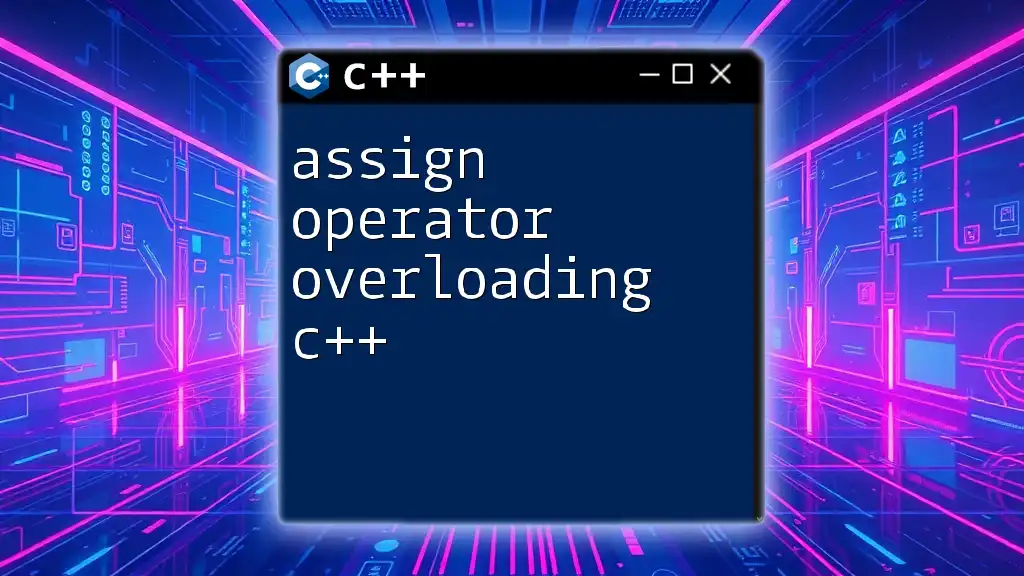
Overloading Comparison Operators
Why Overload Comparison Operators?
Overloading comparison operators significantly enhances the functionality of custom types. It allows objects to be compared directly using conventional operators, which improves the readability of the code. Consequently, this helps maintain logical integrity within your applications.
Steps to Overload Comparison Operators
Step 1: Define Your Class
The first step in operator overloading is to define the class that will use these operators. For example, let's create a simple `Point` class that has two integer coordinates:
class Point {
public:
int x, y;
Point(int x, int y) : x(x), y(y) {}
};
Step 2: Implement Comparison Operators
Next, implement the comparison operators that you wish to support for your class. Below are implementations for `==`, `!=`, `<`, `<=`, `>`, and `>=` operators for the `Point` class:
bool operator==(const Point& p1, const Point& p2) {
return (p1.x == p2.x) && (p1.y == p2.y);
}
bool operator!=(const Point& p1, const Point& p2) {
return !(p1 == p2);
}
bool operator<(const Point& p1, const Point& p2) {
return (p1.x < p2.x) || (p1.x == p2.x && p1.y < p2.y);
}
bool operator<=(const Point& p1, const Point& p2) {
return (p1 < p2) || (p1 == p2);
}
bool operator>(const Point& p1, const Point& p2) {
return !(p1 <= p2);
}
bool operator>=(const Point& p1, const Point& p2) {
return !(p1 < p2);
}
Each operator is designed to return a boolean value based on the logical comparison of the `Point` objects.
Step 3: Use the Overloaded Operators
Now that you have overloaded the comparison operators, you can use them within your code just like built-in types.
Point p1(1, 2);
Point p2(2, 3);
if (p1 < p2) {
// The comparison logic reads clearly: p1 is less than p2
// Implement additional logic here
}
This code demonstrates how you can directly compare two instances of the `Point` class, improving code clarity.
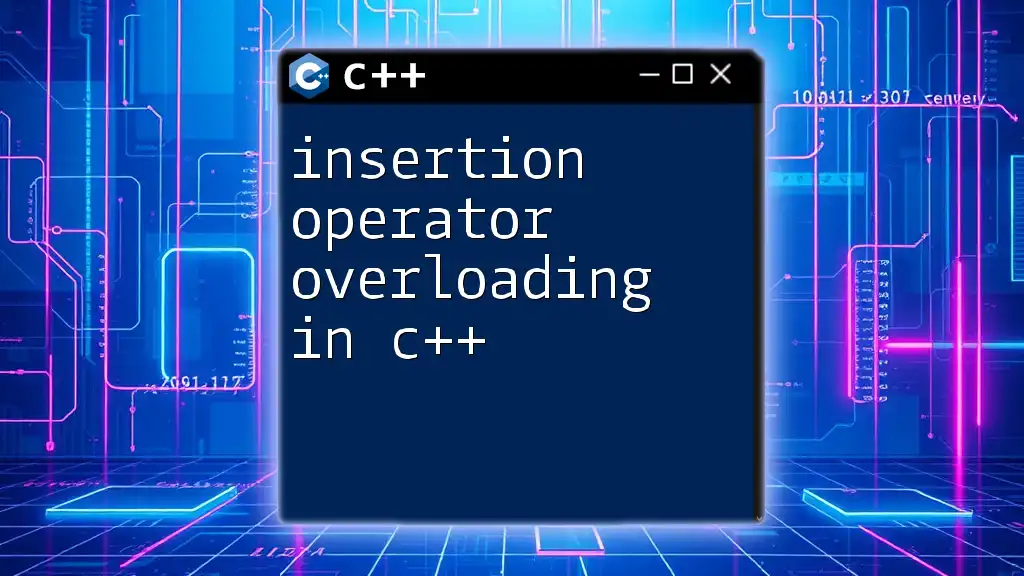
Best Practices for Comparison Operator Overloading
Keep it Consistent
When overloading operators, consistency is key. Your comparisons must behave logically so that they meet user expectations. For example, if `a < b` returns true, then `!(a >= b)` should also be true. Mismatched behavior can lead to confusion and bugs in your programs.
Optimize Performance
When designing your overloaded comparison operators, performance should not be overlooked. Consider returning by const reference or using pointer-based comparisons when applicable. For complex types, you want to avoid unnecessary object copies.
Maintain Readability and Clarity
Using descriptive names for your classes and ensuring clean code contributes to the overall clarity of your program. Overloaded operators should feel intuitive to users familiar with the type, allowing for self-documenting code.
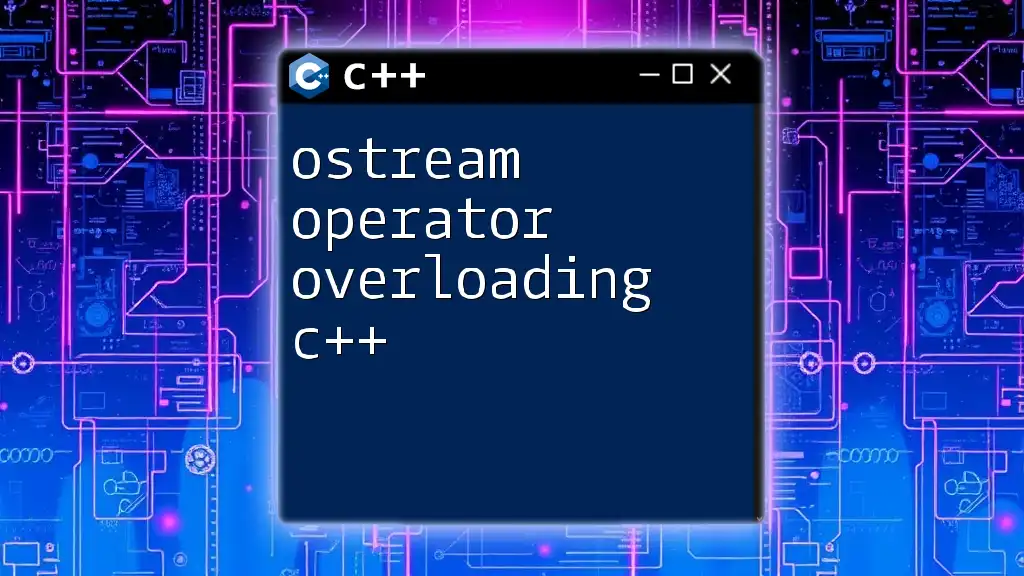
Common Pitfalls in Comparison Operator Overloading
Misleading Operators
One common pitfall is creating overloaded operators that function unintuitively. If an overloaded operator does not behave as expected, this can lead to significant misunderstandings. For instance, two objects that are equal should always return `true` for `==` and `false` for `!=`.
Complexity and Overhead
Overloading operators can introduce complexity and possible performance issues. If your comparison logic begins to involve multiple class members or involves heavy computations, it may lead to efficiency problems. In such cases, consider separating the logic or simplifying your approach.
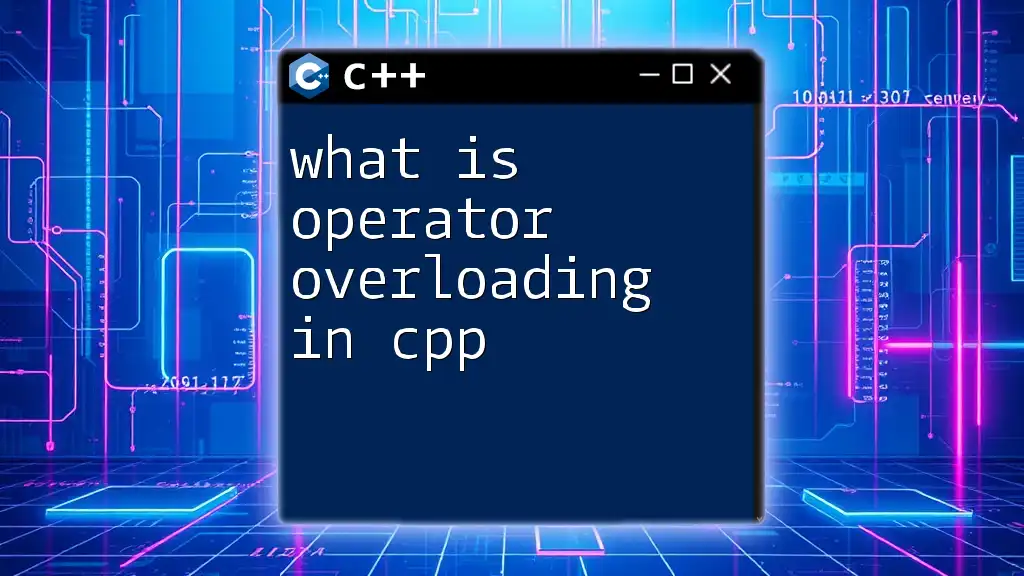
Conclusion
Understanding and implementing C++ comparison operator overloading is a powerful way to enhance the functionality and clarity of your C++ code. By defining how objects of your custom types interact with standard comparison operators, you create a more maintainable and readable codebase.
Remember to focus on consistency, performance optimization, and clarity in your operator overloads and engage with the C++ community to further sharpen your skills. Happy coding!