The "Learning Resource Center CPP" is a valuable hub for streamlined, efficient tutorials and resources that empower users to master C++ commands quickly and effectively.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
C++ Learning Resource Center Essentials
What is C++?
C++ is a powerful programming language that evolved from the C programming language, introducing object-oriented concepts that enable developers to create robust and efficient software applications. Developed in the early 1980s by Bjarne Stroustrup at Bell Labs, C++ has gained widespread recognition for its versatility and performance.
Key Features of C++ include:
- Object-Oriented Programming (OOP): Facilitates code reusability and organization through the use of classes and objects.
- Strong Type Checking: Ensures data type integrity, reducing runtime errors.
- Low-Level Manipulation: Provides direct access to hardware and memory, allowing developers to create high-performance applications.
How to Start Learning C++
To embark on your C++ learning journey, the first step is setting up your development environment. Choosing the right Integrated Development Environment (IDE) is crucial for a streamlined coding experience. Recommended IDEs include:
- Visual Studio: Designed primarily for Windows, it offers a comprehensive set of tools.
- Code::Blocks: A flexible and cross-platform IDE that is easy to set up.
- CLion: A powerful IDE by JetBrains that engages advanced C++ developers.
Once you have installed your preferred IDE, you can verify setup with a simple C++ code snippet:
#include <iostream>
int main() {
std::cout << "Hello, C++!" << std::endl;
return 0;
}
After confirming the installation, you can dive into the basic concepts of C++. Understanding data types, variables, and operators is foundational. Here’s a quick look:
- Data Types: C++ supports several built-in data types, including `int`, `float`, `double`, and `char`.
- Variables: To store data values, variables must be declared with a specific type.
- Operators: Understanding how to use arithmetic, relational, and logical operators is essential for building expressions and handling computations.
C++ Learning Resources
When it comes to learning C++, several resources can significantly enhance your knowledge and skills.
Online Courses and Tutorials: Platforms like Coursera, Udemy, and edX offer a variety of courses ranging from beginner to advanced. Each course often provides practical projects that help solidify your learning.
Books for C++ Learning: Some renowned titles include:
- "C++ Primer" by Stanley B. Lippman: Ideal for beginners, covering the basics comprehensively.
- "Effective C++" by Scott Meyers: Focuses on best practices and advanced features for intermediate learners.
Community and Forums: Engaging with online communities such as Stack Overflow and Reddit can be beneficial. These platforms allow you to ask questions, share knowledge, and learn from experienced developers.
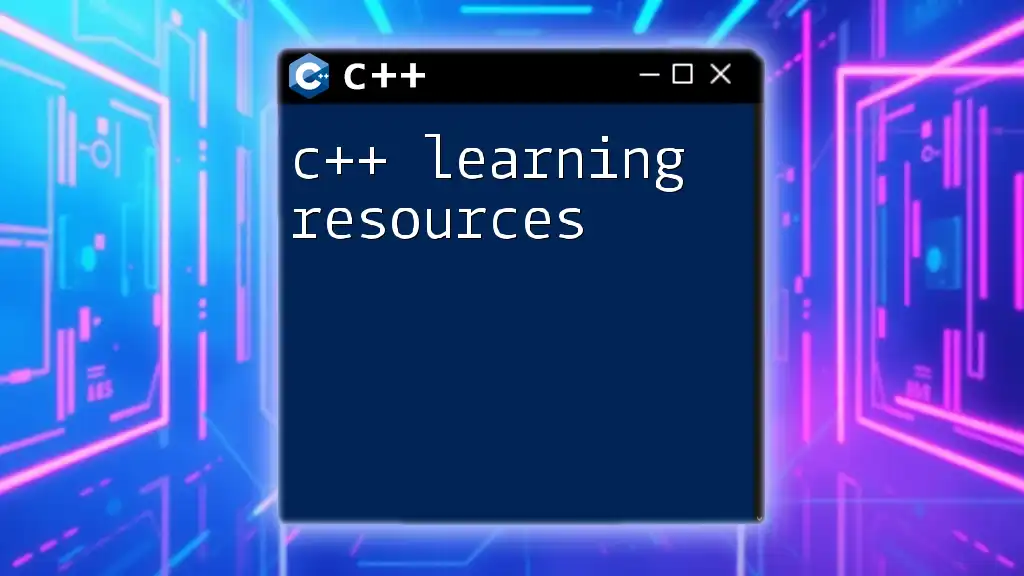
Building Blocks of C++
Understanding Syntax and Structure
Grasping the syntax and structure of C++ is crucial for writing effective code. Control structures such as `if-else` statements and loops (e.g., `for` and `while`) are fundamental. Below are the key control structures:
- If-Else Statements: Used for conditional execution.
int number = 10;
if (number > 0) {
std::cout << "Positive Number" << std::endl;
} else {
std::cout << "Non-Positive Number" << std::endl;
}
- Loops: Useful for executing code repeatedly until a condition is met. For example, the `for` loop:
for(int i = 0; i < 5; i++) {
std::cout << "Iteration: " << i << std::endl;
}
Object-Oriented Programming in C++
C++ is fundamentally structured around Object-Oriented Programming (OOP). Key concepts include:
- Classes and Objects: Classes serve as blueprints for creating objects, encapsulating data and behaviors.
Here's a simple example of a class definition:
class Car {
public:
std::string brand;
int year;
void display() {
std::cout << "Brand: " << brand << ", Year: " << year << std::endl;
}
};
-
Inheritance: This mechanism allows a new class to inherit properties and behaviors from an existing class.
-
Polymorphism: Enables objects to be treated as instances of their parent class, showcasing flexibility in code.
Advanced C++ Concepts
As you become proficient, exploring advanced concepts like pointers and memory management is essential.
- Pointers: Pointers hold memory addresses, allowing for dynamic memory allocation. Using the `new` and `delete` operators, you can manage memory efficiently. For example:
int *ptr = new int(5);
std::cout << *ptr << std::endl;
delete ptr;
- Templates and STL: Templates allow developers to write generic and reusable code. The Standard Template Library (STL) is an essential collection of template classes and functions. A simple use of a vector from STL looks like this:
#include <vector>
std::vector<int> myVector = {1, 2, 3, 4};
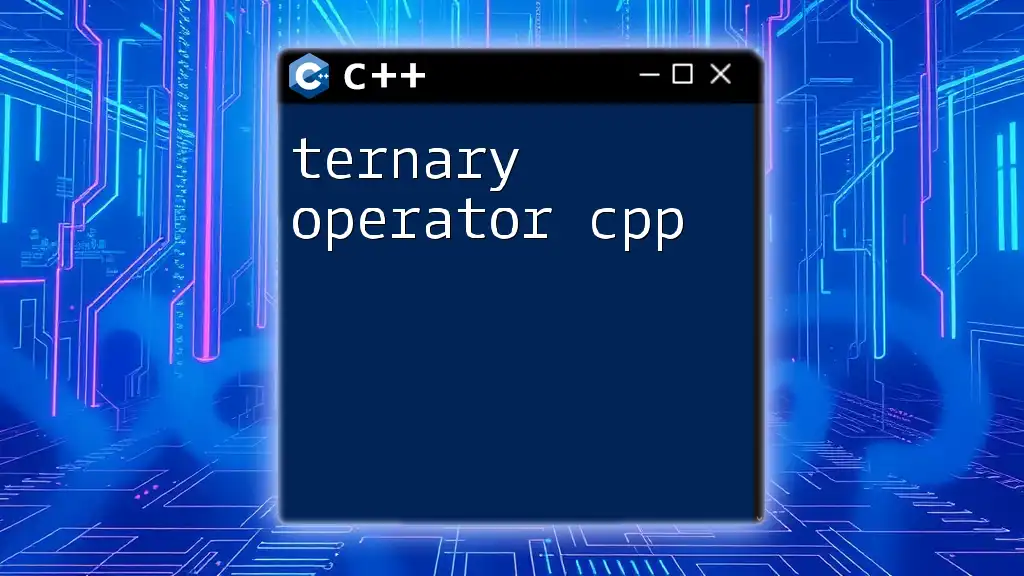
Practical Applications of C++
Developing Real-World Projects
As you gain confidence, the next step involves practically applying your skills through real-world projects.
Project Ideas for Beginners:
- A Calculator Application: A simple project to implement arithmetic functions.
- A To-Do List Application: Organizing tasks with features for adding and deleting items.
Advanced Project Examples:
- Game Development: Creating simple games using libraries like SDL or SFML.
- GUI Applications: Developing interfaces using frameworks such as Qt or GTK.
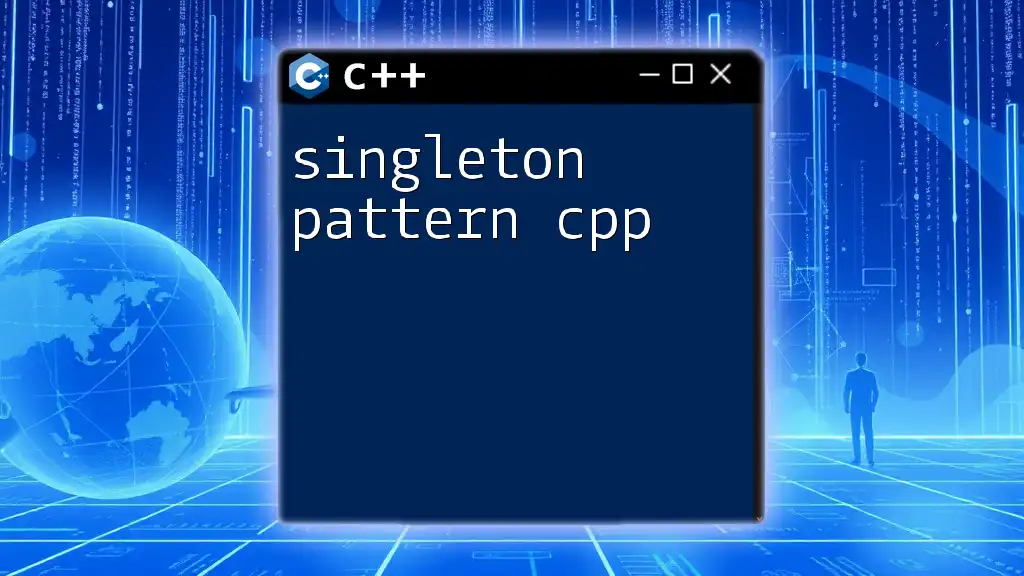
Tips for Mastering C++
To truly master C++, consider the following tips:
-
Regular Practice: Programming is a skill honed over time. Regular practice through coding challenges can strengthen your problem-solving abilities. Platforms such as LeetCode and Codeforces offer numerous challenges tailored to C++.
-
Participate in Open Source: Engaging in open-source projects provides hands-on experience and allows you to collaborate with seasoned programmers.
-
Join C++ Coding Meetups: Networking with fellow C++ enthusiasts can offer inspiration, guidance, and collaboration opportunities.
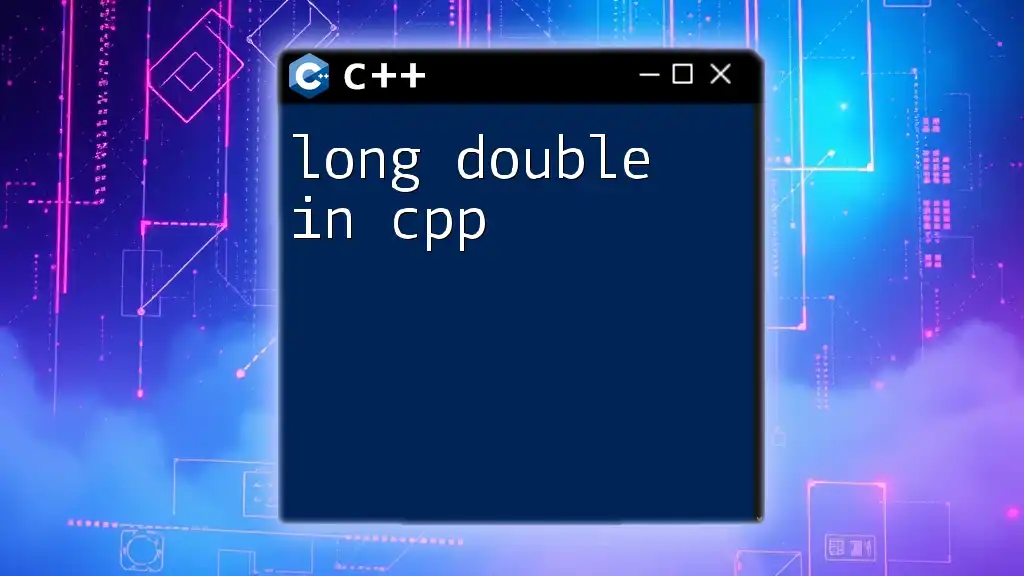
Conclusion
In summary, the learning resource center for C++ provides invaluable guidance and resources to help you on your coding journey. From foundational concepts to advanced practices, the key is to stay curious and engaged in continuous learning. Remember, each line of code you write brings you closer to becoming a proficient C++ developer. Embrace the journey and don’t hesitate to explore beyond the basics to uncover the vast potential of C++.
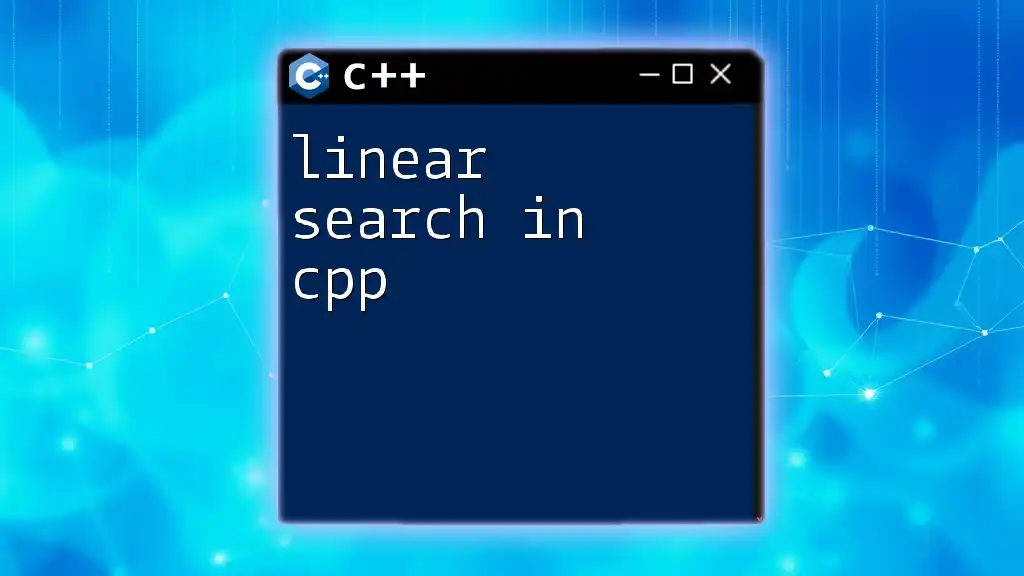
Additional Resources
A comprehensive list of useful websites and tools can further enhance your learning experience. Look for official documentation, tutorials, and interactive platforms that can offer both depth and breadth in your understanding of C++.
Maintain an enthusiastic and inquisitive approach as you navigate your C++ learning path. The world of programming is vast, and every learning opportunity can lead to new heights in your coding expertise.