Open source C++ games are community-driven projects that allow developers and enthusiasts to collaborate, modify, and share their code, fostering learning and innovation in game design.
Here's a simple example of a C++ code snippet for a basic game loop:
#include <iostream>
int main() {
bool isRunning = true;
while (isRunning) {
// Game logic goes here
std::cout << "Game is running..." << std::endl;
// Example condition to exit the loop
char userInput;
std::cout << "Type 'q' to quit: ";
std::cin >> userInput;
if (userInput == 'q') {
isRunning = false;
}
}
return 0;
}
Understanding Open Source C++ Games
Open source software plays a pivotal role in modern game development, particularly in the world of C++ games. By allowing developers to access and modify source code, open source projects encourage collaboration, creativity, and rapid learning. With open source C++ games, the code is openly available for anyone to study, modify, and enhance, providing a foundation for both aspiring and seasoned developers.
Why C++ for Game Development?
C++ remains a popular choice among game developers due to its exceptional performance and fine control over system resources. This language allows for more complex calculations and better memory management, essential attributes in game development. Its long-standing association with major game engines solidifies C++ as a reliable and efficient choice.
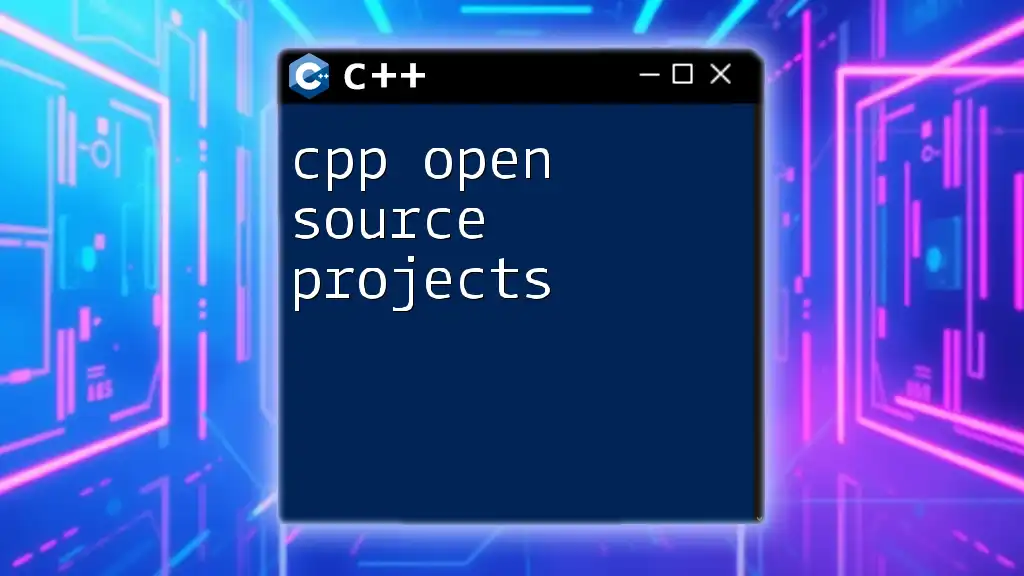
Benefits of Using Open Source C++ Games
Learning from open source C++ games can significantly accelerate one’s programming skills and game design understanding.
Learning Opportunities
- Access to Examples: Studying existing code exposes learners to various programming techniques, design patterns, and best practices.
- Community Support: Open source projects typically foster active communities, offering support through forums, chats, and collaborative contributions. This creates an environment where learners can ask questions, share ideas, and receive feedback.
Customization and Flexibility
One of the main advantages of open-source code is the ability to modify existing projects. Developers can experiment with gameplay mechanics, add new features, or even completely overhaul a game. This flexibility allows for creative expression and unique customization—a significant advantage over proprietary games.
Cost-Effective Solutions
Almost all open source C++ games are available for free. This eliminates any licensing fees, making it accessible for students, hobbyists, and indie developers. Additionally, open source games provide a diverse range of experiences, from casual games to complex simulations.
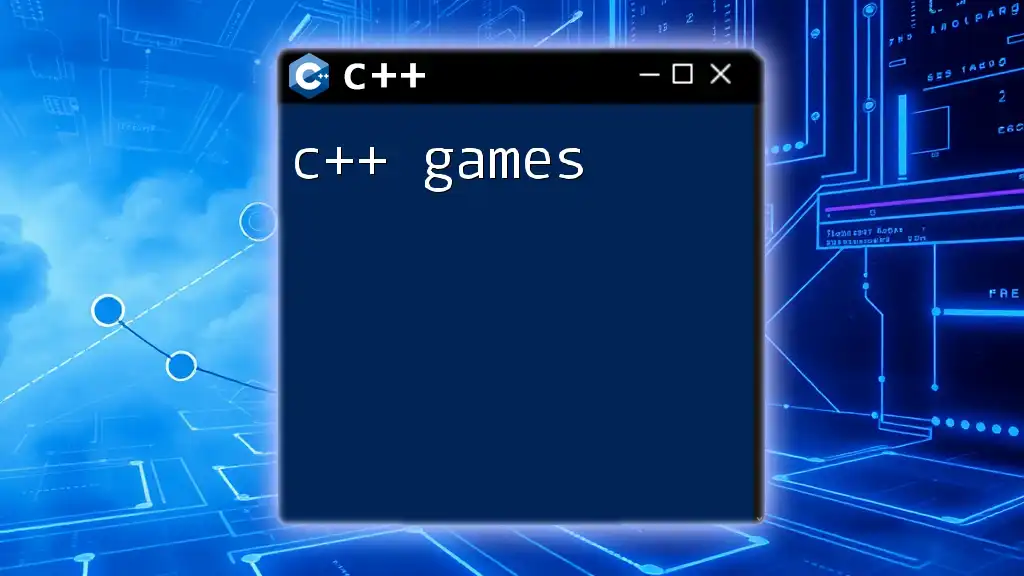
Popular Open Source C++ Game Engines
Understanding C++ game engines is vital for anyone looking to develop games. These engines facilitate game creation, offering tools for rendering, physics, sound management, and more.
Overview of Game Engines
Game engines handle complex processes such as rendering graphics, managing resources, and processing inputs, allowing developers to focus more on creativity than on technical constraints.
Godot Engine
The Godot Engine is an empowering open-source game engine known for its flexibility and user-friendly interface. It supports 2D and 3D game development, leveraging C++ for efficiency.
- Community-Driven Contributions: The Godot community actively contributes to its ecosystem. This ensures that the engine is continuously updated with new features and optimizations.
- Example Game: The Godot Wild Jam Projects showcase the diverse creative output possible within the engine.
SDL (Simple DirectMedia Layer)
SDL is a powerful library that provides low-level access to audio, keyboard, mouse, joystick, and graphics hardware via OpenGL and Direct3D.
- Overview of SDL in Game Development: SDL is often used as a foundation for developing games due to its simplicity and effectiveness. Developers can build complex games from a solid base while using C++ for game logic.
- Example Game: Doom, SDL version is a** prime example** of a classic game revitalized through an open-source approach.
SFML (Simple and Fast Multimedia Library)
SFML provides simple APIs to manage graphics, audio, and network operations, making it an excellent choice for both beginners and advanced developers.
- Advantages of Using SFML: It features a straightforward and intuitive interface, which allows developers to focus more on game design rather than on technical implementation.
- Example Game: Invasion - The Game showcases the capabilities of SFML and serves as a learning resource for new developers.
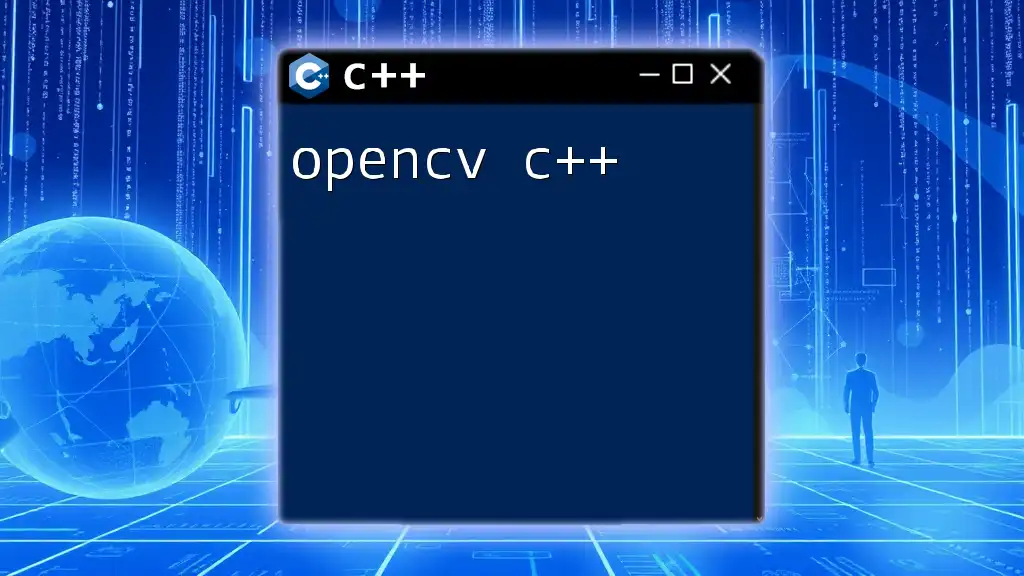
Notable Open Source C++ Games
Numerous notable games demonstrate the potential of open source C++ games and the vibrant communities surrounding them.
Freeciv
Freeciv is an empire-building strategy game inspired by the classic Civilization series. It encourages users to take part in development or improve the gameplay through community contributions.
- Overview of the Game Mechanics: Players build and develop a civilization while competing with others. The game implements various strategies and tactics.
- Code Structure and Repository Link: The Freeciv repository is a treasure trove for budding developers wishing to learn about game architecture.
- How to Contribute: New contributors can start with a Getting Started Guide provided in the repository.
Battle for Wesnoth
Battle for Wesnoth is a fantasy-themed turn-based strategy game featuring rich lore and deep gameplay.
- Explanation of the Gameplay: Players engage in tactical battles, managing units while exploring a vast world filled with quests.
- Key Features and Community Assets: The game boasts extensive user-created content, allowing for a rich gaming experience.
- Source Code Analysis: Analyzing the architecture provides unique insights into game mechanics and programming practices.
OpenRA
OpenRA is a reimagining of classic real-time strategy games like Command & Conquer. It showcases modern improvements while retaining the spirit of the originals.
- Overview and Gameplay Mechanics: Players control units and resources, leading their factions to victory using strategy and tactics.
- Contribution Opportunities: OpenRA welcomes contributions; developers can easily join the project.
- Example Code Snippets for Modification: This project allows developers to experiment with code snippets, enhancing their understanding of game development.
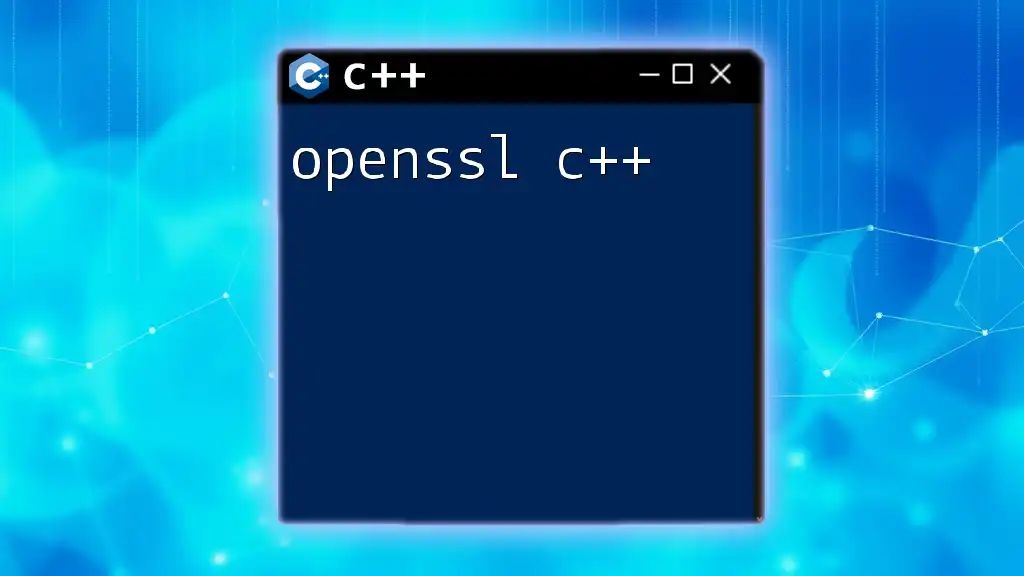
Getting Started with Open Source C++ Games
Setting Up Your Environment
To begin working on open source C++ games, developers must set up their environment properly.
- Required Tools and Software: Developers will need compilers, libraries, and perhaps an IDE like Visual Studio or Code::Blocks.
- Example Setup for Godot and SDL: Follow the installation instructions specific to each engine, ensuring all dependencies are met.
Cloning the Repository
To engage with open source projects, you will first need to clone the game repository.
- Step-by-Step Instructions: You can clone a repository from GitHub using the following command:
git clone https://github.com/freeciv/freeciv.git
Building the Project
Open source projects usually come with instructions for building. Familiarizing oneself with build systems like CMake and Makefile is essential.
- Example Build Process for a Game: Building Freeciv could involve running a simple command or two to compile the sources into an executable. Follow the guidelines provided in the project documentation.
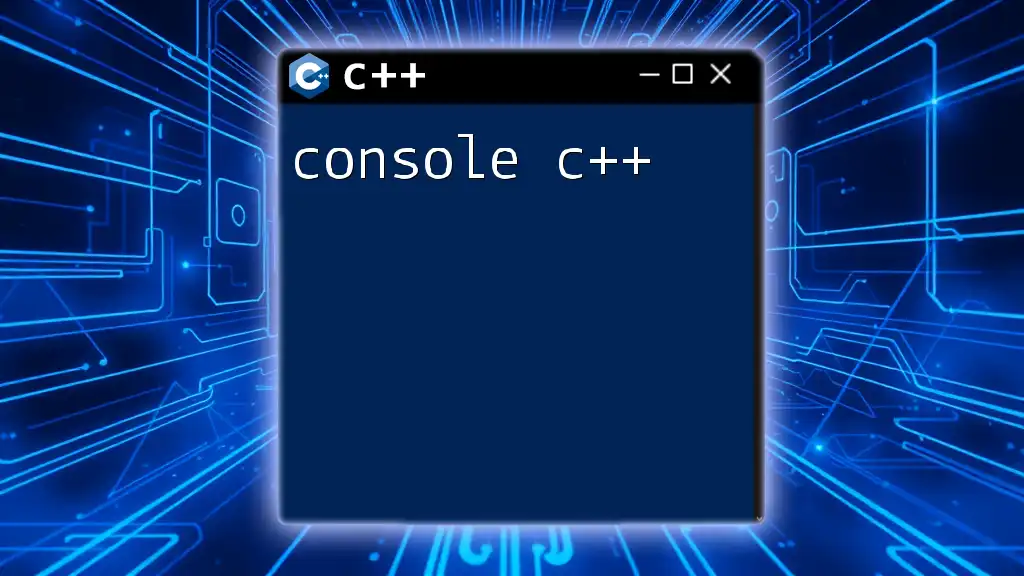
Contributing to Open Source C++ Games
Understanding Version Control
Familiarity with version control systems, particularly Git, is essential for effective collaboration in open-source projects.
- Basic Git Commands: Learn essential Git commands for cloning, committing changes, and pushing updates.
- Importance of Branching and Pull Requests: Understand the branching model to contribute effectively and utilize pull requests to submit changes for review.
Writing and Documenting Code
Code quality is crucial in open-source development.
- Best Practices for Clean Code: Write clear, concise, and organized code, maintaining consistency across the project.
- Importance of Comments and Documentation: Properly comment your code to explain logic, keeping documentation up-to-date to help others understanding your contributions.
Engaging with the Community
Participation in the community enhances the experience and can significantly accelerate learning.
- Participating in Forums and Discussions: Engaging in discussions and communities related to open-source C++ games can provide insights, solutions, and new friendships.
- Encouraging Feedback and Suggestions: Ask for critiques on your contributions. Feedback is invaluable and fosters improvement.
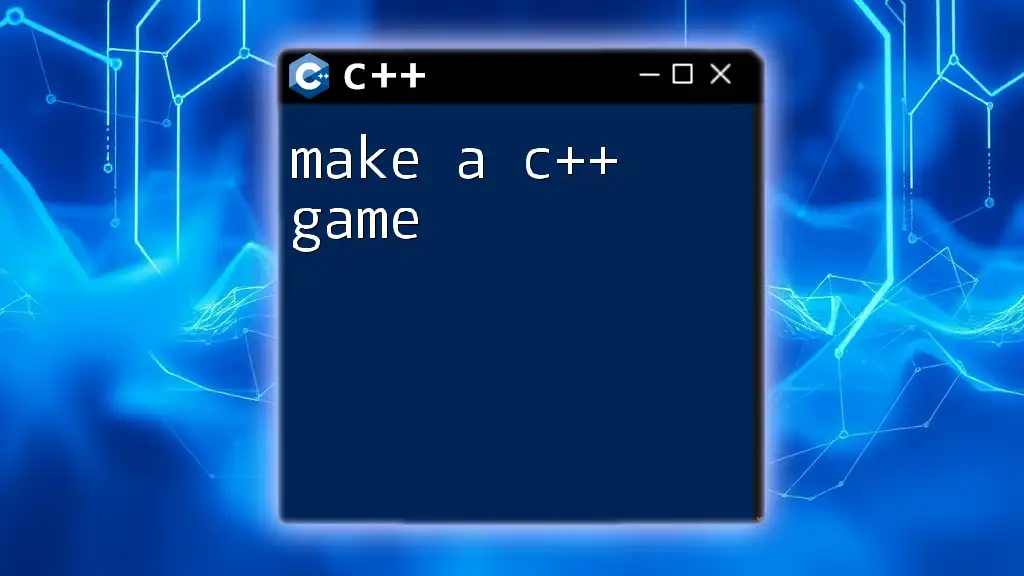
Resources for Learning and Development
Online Platforms for C++ Game Development
Utilize online resources that host tutorials, articles, and forums dedicated to C++ game development.
- Recommended Websites and Forums: Sites like GitHub, Stack Overflow, and dedicated forums are great for finding guides and engaging with peers in the field.
Books on Game Development in C++
Investing in literature can provide more depth in understanding C++ and game design principles.
- Titles Worth Considering: Seek out key books covering foundational game design, C++ programming, and graphics rendering techniques.
YouTube Channels and Tutorials
Visual resources can be immensely helpful for grasping complex concepts.
- Video Resources for Hands-On Learning: Educational channels often provide real-world coding examples, step-by-step guides, or project walkthroughs.
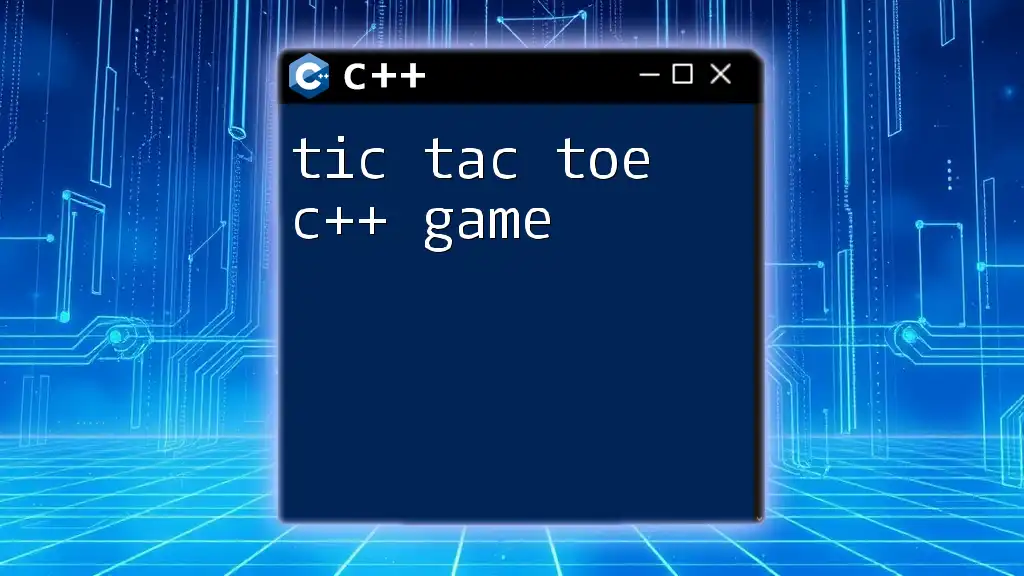
Conclusion
Contributing to open source C++ games offers a unique opportunity for developers to enhance their skills while being part of a community. The wealth of resources, platforms, and support ensures that any aspiring game developer can find their place in this vibrant sector.
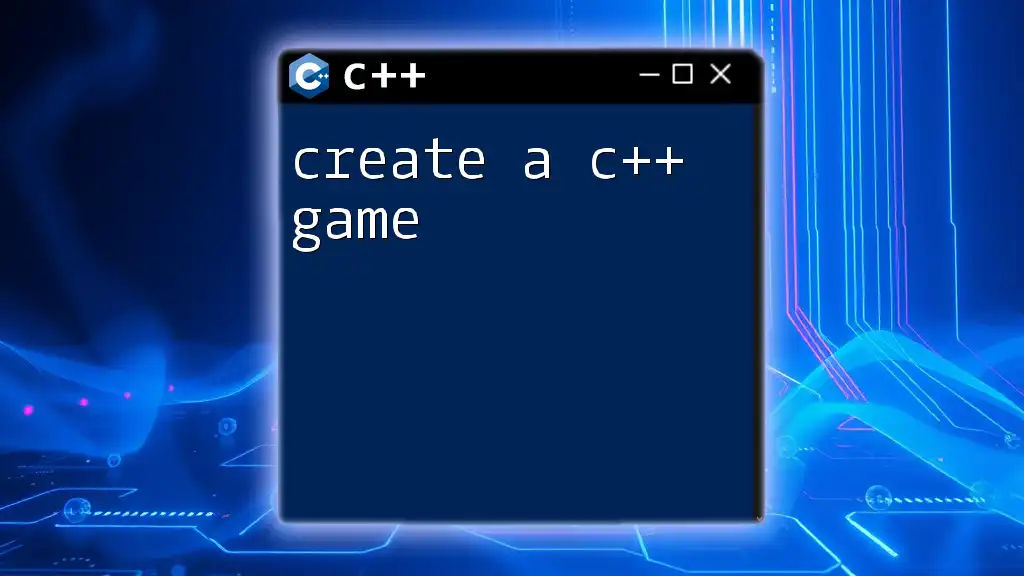
Call to Action
Join the open source movement today! Explore the plethora of open source C++ games available, dive into the code, and contribute your talents to innovative projects. Visit community forums and relevant repositories to expand your network and share your ideas.
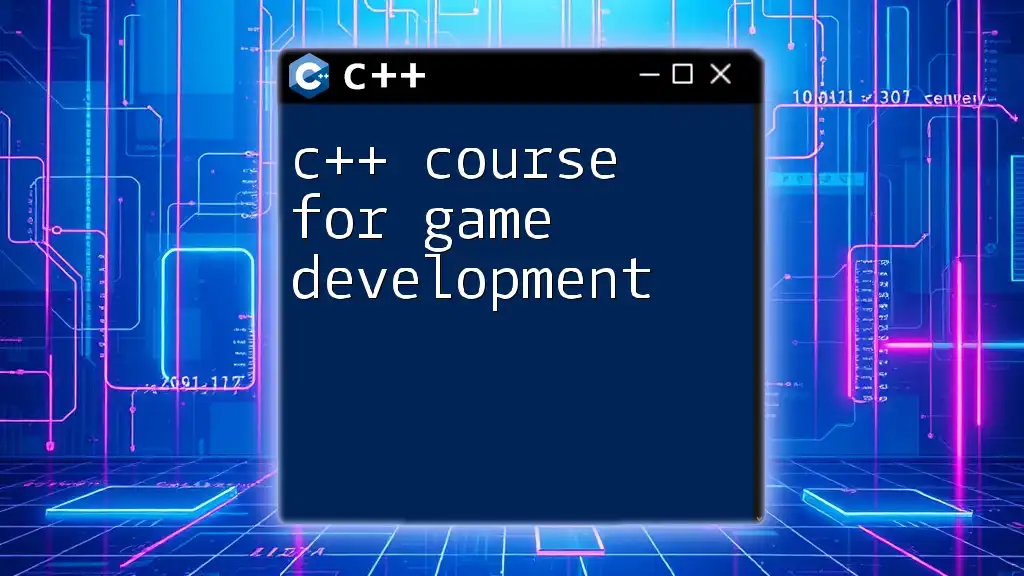
FAQs About Open Source C++ Games
As you start your journey, you may have questions about the best practices, license concerns, or the future of open source gaming. Engage with the community, read the available documentation, and don't hesitate to ask for help!
Appendix
- Glossary of Terms: Familiarize yourself with terminology relevant to open source and game development to ensure you understand the community's language and processes.
Your journey into open-source C++ gaming is just beginning, and with the right resources and community support, you'll be creating and contributing in no time!