When you encounter the error "cannot open source file" in C++, it typically indicates that the compiler cannot find the specified header or source file, which may be due to incorrect file paths or missing files.
Here's an example of how to include a header file correctly in C++:
#include "myHeader.h" // Ensure 'myHeader.h' is located in the same directory or provide the correct path.
Understanding the "Cannot Open Source File" Error
What Does This Error Mean?
The "cannot open source file" error in C++ typically arises during the compilation phase. It indicates that the compiler cannot locate a specified source file necessary for building the program. This can be frustrating, particularly for beginners, as it disrupts the workflow and hinders progress.
Common Causes of the Error
File Does Not Exist
One of the primary causes for this error is that the file simply does not exist in the given location. This often happens due to typos in file names or incorrect file paths specified in the code or project settings. For instance, referencing a file named `myFile.cpp` as `myfile.cpp` (note the lowercase "f") will lead to the error.
Example:
#include "myfile.cpp" // Incorrect reference
Incorrect Project Configuration
Another common cause is related to the development environment settings. Integrated Development Environments (IDEs) like Visual Studio or Code::Blocks require proper configuration to locate source files correctly. If the project settings are misconfigured, you will likely face this error.
For example, missing source files in the project explorer or incorrect project properties could lead to an inability to find files during compilation.
Path Issues
Path issues can also be a significant source of confusion. C++ projects often utilize include paths to reference external files. If the include paths are not set correctly, the compiler won’t be able to find those files.
Here’s how you can identify incorrect paths in your code:
#include "myIncludes/myHeader.h" // Ensure correct path
If `myHeader.h` is not in the folder `myIncludes`, you'll trigger this error.
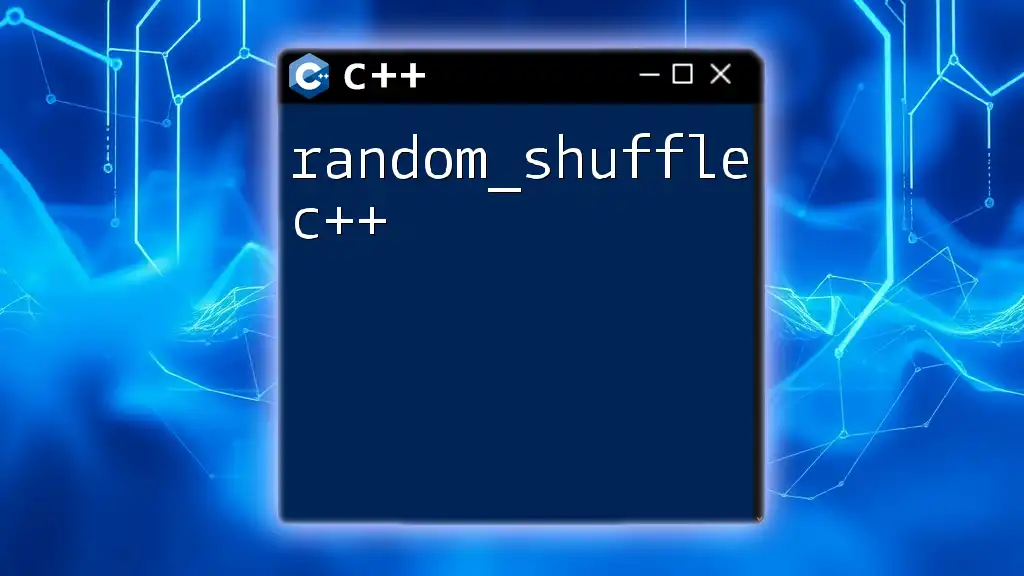
Troubleshooting the "Cannot Open Source File" Error
Step-by-Step Diagnostic Approaches
Verify File Location
Start by verifying whether the source file exists in the expected directory. Using terminal commands can be helpful for this process:
Linux/Unix:
ls /path/to/your/project/
Windows:
dir C:\path\to\your\project\
Ensure that the file is indeed present.
Check File and Directory Permissions
Sometimes, file permissions can prevent access to C++ source files. Ensure that the necessary permissions are set for reading files. In Linux/Unix systems, you can change file permissions with:
chmod 644 myFile.cpp
This command allows the owner to read and write, while others can only read the file, ensuring sufficient access rights.
IDE-Specific Solutions
Visual Studio
In Visual Studio, follow these steps to resolve the issue:
- Open your project in Visual Studio.
- Navigate to Solution Explorer and find your file. Ensure it is included in the project.
- Right-click on your project in Solution Explorer and select Properties to check if the include directories are set correctly.
- Under Configuration Properties > C/C++ > General, ensure the Additional Include Directories field contains paths to any required headers.
Code::Blocks
For users of Code::Blocks, here’s a simple fix:
- Open your project and right-click on the project name in the Projects tab, selecting Build Options.
- Go to the Search Directories tab to ensure the correct paths are listed under Compiler and Linker.
- Adjust any incorrect paths to point to the right locations.
Eclipse
Eclipse users should inspect their build path settings:
- Right-click on your project and select Properties.
- Navigate to C/C++ Build > Settings.
- Check the Includes section to make sure that paths to header files are correctly specified.
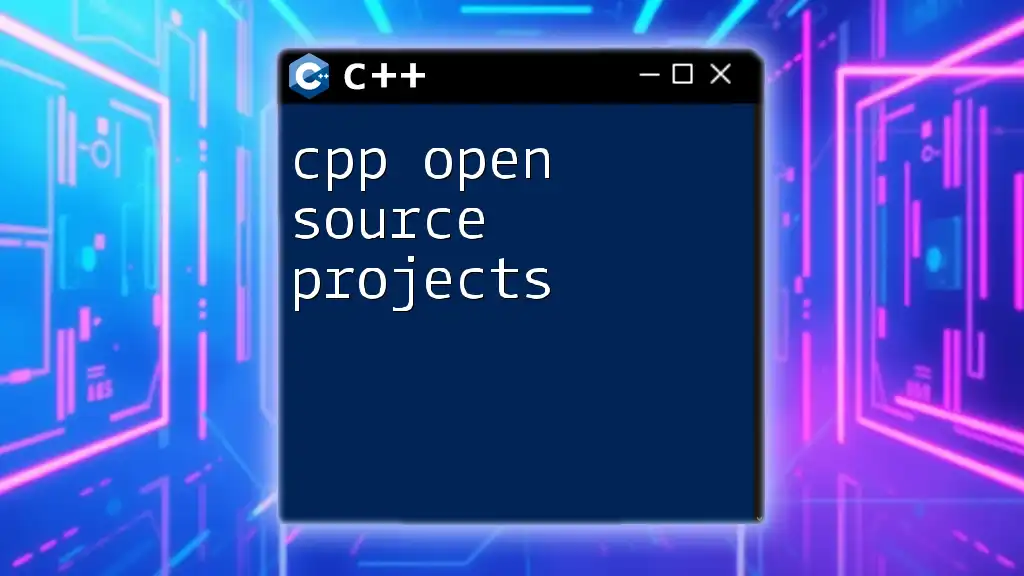
Best Practices to Avoid Future Errors
Consistent Naming Conventions
Implementing a consistent naming convention for files is crucial to avoid confusion. Using camelCase or snake_case can help establish clarity. For example, name your files in a predictable manner:
- `myFile.cpp`
- `myHeader.h`
By maintaining consistent names, you significantly reduce the chance of encountering errors due to typos.
Keeping Project Structure Organized
A well-organized project structure minimizes the potential for errors. Group related files into folders based on functionality, ensuring that all files are easy to locate. For example:
/ProjectRoot
/src
main.cpp
utils.cpp
/include
utils.h
This layout keeps everything tidy and simplifies the debugging process.
Regular Backups and Version Control
Utilizing version control systems like Git is vital. Frequent commits and pushing code updates to a remote repository not only keeps track of changes but also provides a safe fallback if a problem arises. For instance, basic Git commands include:
git add .
git commit -m "Fixed path issues"
git push origin main
Implementing version control not only helps prevent loss but also provides clarity on changes made over time.
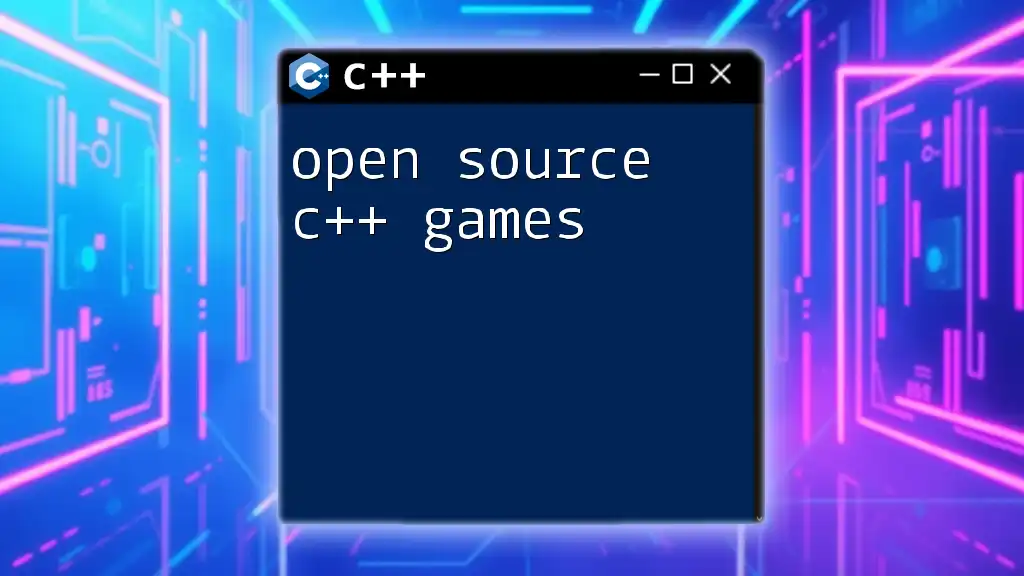
Conclusion
The "cannot open source file c++" error can stem from several issues including missing files, incorrect paths, and misconfigured project settings. By understanding the causes and applying diagnostic approaches, developers can effectively troubleshoot and prevent this error in the future. Adopting best practices such as naming conventions, project organization, and version control will enhance the reliability of your C++ development process.
Further exploration of resources, communities, and tools available for C++ programming can empower developers with the necessary support to succeed in their coding endeavors.