C++ open source projects provide valuable opportunities for learning and collaboration, allowing developers to contribute to real-world applications while honing their programming skills.
Here’s a basic example of how to read a file using C++:
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream inputFile("example.txt");
std::string line;
if (inputFile.is_open()) {
while (getline(inputFile, line)) {
std::cout << line << std::endl;
}
inputFile.close();
} else {
std::cerr << "Unable to open file";
}
return 0;
}
Understanding C++ and Open Source
C++ is a versatile programming language that has stood the test of time. It plays a significant role in numerous domains, including system software, game development, and high-performance applications. The ability to use C++ effectively is crucial for developers aiming to create robust applications.
The Open Source Model
Open source refers to software that is freely available to anyone to use, modify, and distribute. The principles of open source emphasize collaboration, allowing a community to collectively improve a project. This model has become increasingly vital in software development, as it fosters innovation and reduces development costs.
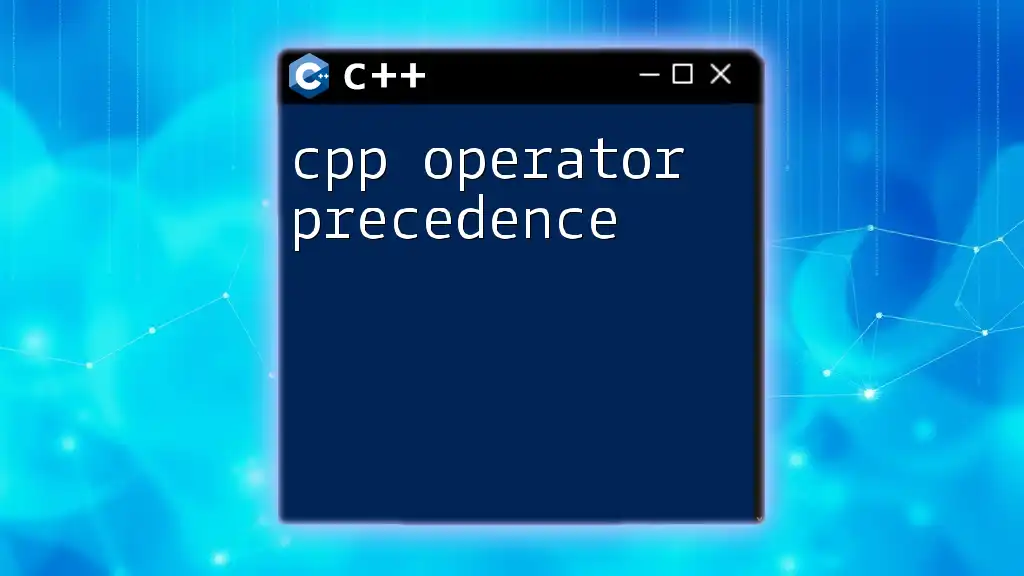
Why Contribute to C++ Open Source Projects?
Contributing to C++ open source projects is beneficial on multiple levels. For beginners and experienced developers alike, engaging in open source can enhance programming skills, as contributors encounter real-world challenges that require thoughtful solutions.
Additionally, by actively participating in these projects, developers can build a professional portfolio and establish a network within the tech community. Many successful programmers began their careers with open source contributions.
One personal experience emphasizes this point: while working on a small C++ project, I learned how to read and write code collaboratively, ultimately improving my coding standards and practices.
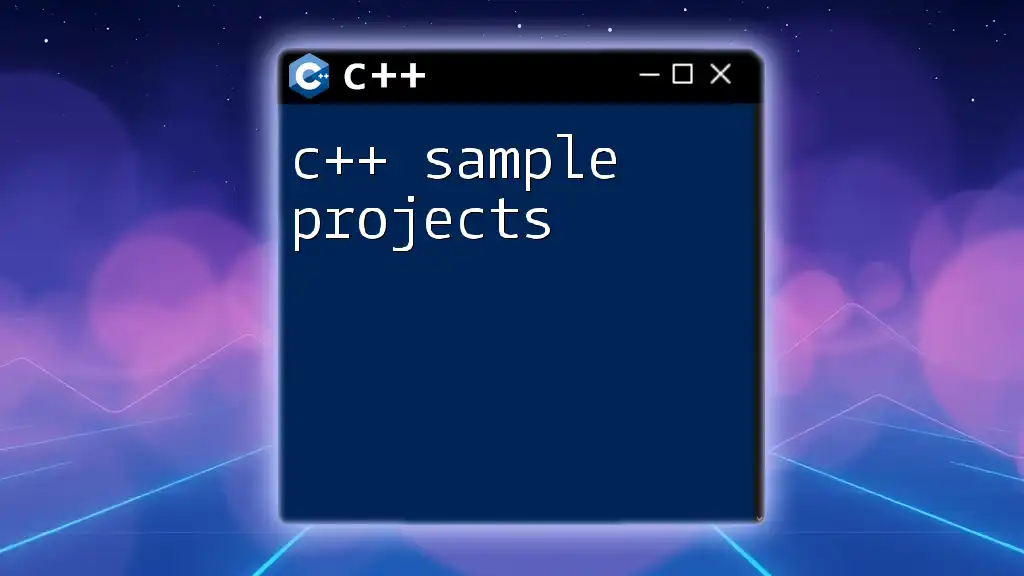
Popular C++ Open Source Projects to Consider
Overview of Notable C++ Projects
There are numerous C++ open source projects available, each with its niche and community. Below are some noteworthy projects that you may find interesting.
Game Development
- Godot Engine
Godot is a feature-rich game engine that supports 2D and 3D game development. It is well-known for its intuitive interface and a large library of built-in features.
To contribute, consider helping with documentation or submitting code improvements that enhance engine performance or add new functionalities.
// Example of creating a simple scene in Godot
extends Node
func _ready():
print("Hello, Godot!")
- OpenRA
OpenRA is a project that reimagines classic real-time strategy games. The community is regularly looking for contributors for both coding and artwork.
As a contributor, you might focus on bug fixes, implementing new mechanics, or enhancing user experience.
Networking and Communication
- POCO C++ Libraries
POCO provides essential libraries to develop networked applications easily. Its comprehensive set of features targets different aspects of modern application development, including networking, threading, and database connectivity.
To contribute, you can help by writing unit tests or adding documentation to improve user understanding.
#include <Poco/Net/HTTPClientSession.h>
#include <Poco/Net/HTTPResponse.h>
#include <Poco/StreamCopier.h>
// Code snippet for a simple HTTP GET request
void doGetRequest(const std::string& url) {
Poco::Net::HTTPClientSession session(url);
Poco::Net::HTTPResponse response;
Poco::Net::HTTPRequest request(Poco::Net::HTTPRequest::HTTP_GET, "/");
session.sendRequest(request);
std::istream& rs = session.receiveResponse(response);
Poco::StreamCopier::copyStream(rs, std::cout);
}
- libcurl
Libcurl is a powerful library used for transferring data with URLs. This is particularly useful in network programming, handling file transfers, and more.
To get started with contributions, check the issue tracker for bugs or outdated documentation.
Graphics and Visualization
- OpenCV
OpenCV is a highly-acclaimed library focused on computer vision and machine learning. It is widely used in various fields, including robotics and real-time image processing.
By contributing to OpenCV, you can address bugs or add new features. For instance, you might work on a module that enhances image processing algorithms.
#include <opencv2/opencv.hpp>
// Simple usage of OpenCV to read and display an image
int main() {
cv::Mat img = cv::imread("image.jpg");
cv::imshow("Display Image", img);
cv::waitKey(0);
return 0;
}
- Blender
Blender is not just a rendering tool; it is also an extensive 3D animation suite. As a contributor, you can participate in various aspects, including scripting, user interface enhancements, and bug fixes.
Machine Learning
- dlib
Dlib is a modern C++ toolkit for machine learning, image processing, and computer vision. Its rich set of algorithms makes it suitable for many applications.
By joining the community, you can work on implementing new algorithms or optimizing existing ones. Start with small tasks to familiarize yourself with the codebase.
#include <dlib/dnn.h>
// Example structure of a simple neural network
template <typename SUBNET>
using my_layer = dlib::add_layer<dlib::relu, dlib::con_<16,3,3,SUBNET>>;
using net_type = dlib::loss_mean_square<my_layer<dlib::input_rgb_image>>;
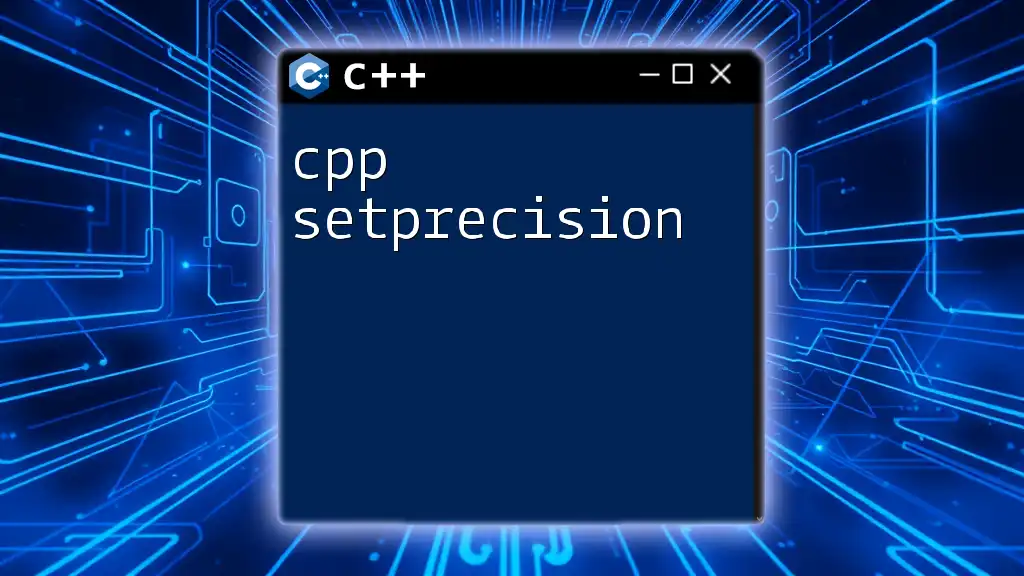
Getting Started with C++ Open Source Contributions
Setting Up Your Environment
Before diving into contributions, you’ll need to set up your development environment properly. Recommended Integrated Development Environments (IDEs) for C++ include Visual Studio Code, Eclipse, and CLion. Each of these IDEs offers tools for code navigation, debugging, and version control.
Understanding how to manage libraries and dependencies is also critical. Tools like CMake can help you automate this process effectively.
Finding Projects to Contribute To
Finding the right C++ open source projects to contribute to can be exciting. Platforms like GitHub and GitLab host a wealth of projects. You can search using terms like "C++" or "open source" to find projects that align with your interests.
Assessing project documentation is essential to gauge how welcoming a community is towards new contributors. Look for projects with clear guidelines and active discussions.
Understanding Version Control with Git
Familiarity with Git is paramount for modern development. Basic commands such as `git clone`, `git commit`, `git push`, and `git pull` form the foundation of collaborative coding.
Example of a basic Git command sequence:
# Cloning a repository
git clone https://github.com/example/repo.git
# Making changes
git add .
git commit -m "Initial commit with changes"
git push origin main
Reading and Understanding Codebases
Diving into a large codebase can be daunting. Start by identifying key components of the project and understanding their interactions. Utilize documentation, README files, and community forums to clarify concepts.
Issues and Pull Requests
Issues can range from bugs to feature requests. Begin by addressing smaller issues to build confidence. When you’ve made your changes, submitting a pull request is your way of suggesting improvements to the project.
A well-structured pull request should include:
- A clear description of the changes
- Reference to the issue it addresses
- Explanations for more complex changes
Staying Engaged with the Community
Engagement is vital. Follow project maintainers on social media platforms and participate in discussions. Engaging with the community can lead to further opportunities and enhanced learning.
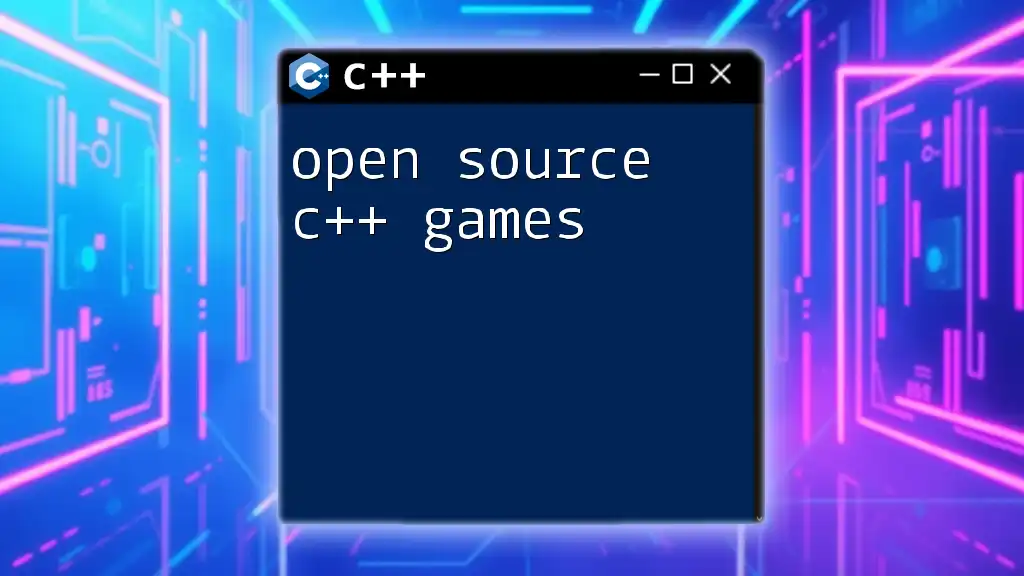
Challenges and Considerations in C++ Open Source
Contributing to C++ open source projects can present challenges, such as navigating complex codebases or managing time effectively. Balancing personal projects with contributions takes practice and planning.
Encountering criticism is part of the process. Use feedback constructively to learn and grow as a developer, refining your skills through practice.
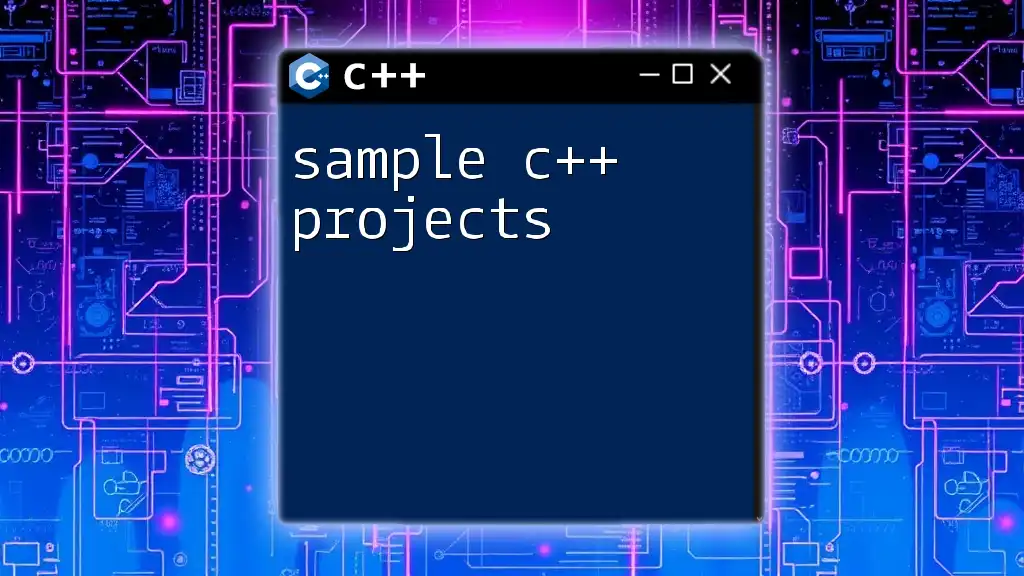
Future Trends in C++ and Open Source
C++ continues to evolve, introducing innovative features tailored for modern programming paradigms. Technologies such as Artificial Intelligence and IoT are driving a surge in demand for proficient C++ developers in open source communities.
By aligning your skills with these trends, you can position yourself advantageously in the dynamic tech landscape.
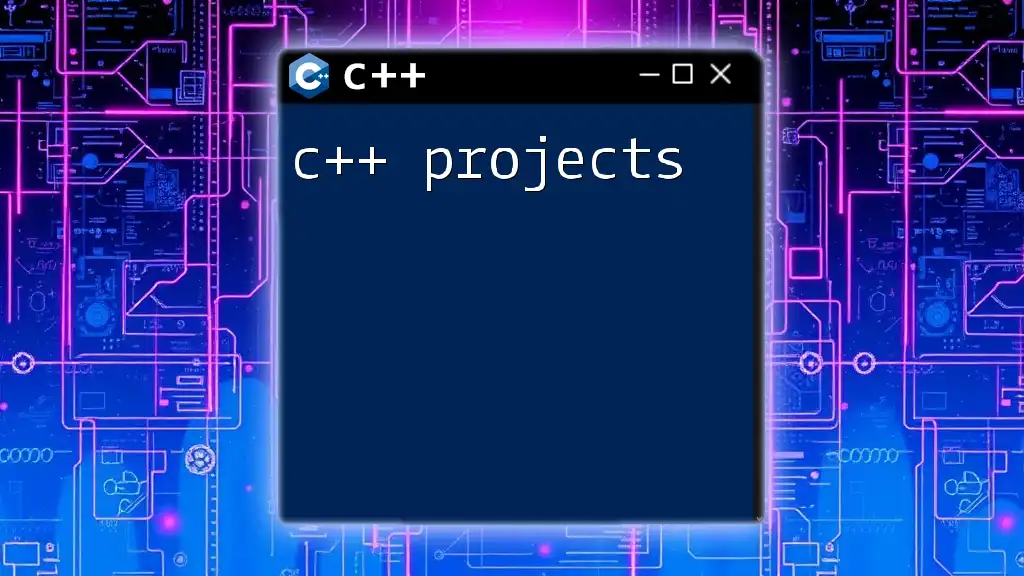
Conclusion
Participating in C++ open source projects offers invaluable experiences that enhance both technical and collaborative skills. Whether you’re looking to deepen your knowledge or build a professional network, these contributions can significantly shape your career.
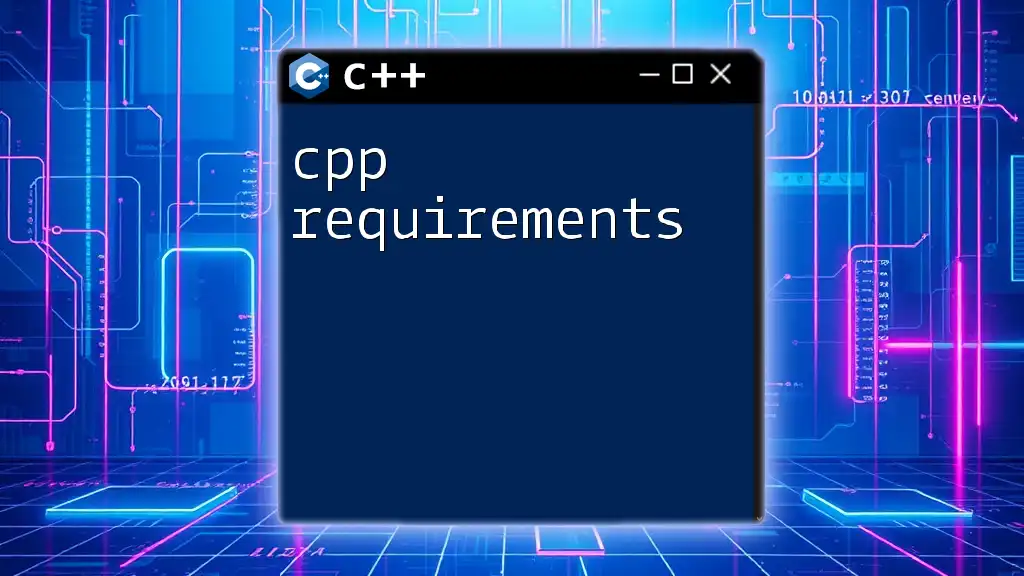
Resources for Further Learning
To continue your learning journey, explore books, online courses, and forums dedicated to C++. Engage with communities on platforms like Stack Overflow or Reddit to discover more about open source contributions and best practices in C++ development.