The "Tic Tac Toe" C++ game is a simple console application that allows two players to take turns marking their spots on a 3x3 grid until one player wins or the game ends in a draw.
Here's a basic implementation in C++:
#include <iostream>
using namespace std;
char board[3][3] = {{'1', '2', '3'}, {'4', '5', '6'}, {'7', '8', '9'}};
char currentPlayer = 'X';
void drawBoard() {
cout << "Current Board:\n";
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
cout << board[i][j] << " ";
}
cout << endl;
}
}
bool checkWin() {
for (int i = 0; i < 3; i++) {
if (board[i][0] == currentPlayer && board[i][1] == currentPlayer && board[i][2] == currentPlayer) return true;
if (board[0][i] == currentPlayer && board[1][i] == currentPlayer && board[2][i] == currentPlayer) return true;
}
if (board[0][0] == currentPlayer && board[1][1] == currentPlayer && board[2][2] == currentPlayer) return true;
if (board[0][2] == currentPlayer && board[1][1] == currentPlayer && board[2][0] == currentPlayer) return true;
return false;
}
int main() {
int choice, turns = 0;
while (true) {
drawBoard();
cout << "Player " << currentPlayer << ", enter a number to place your marker: ";
cin >> choice;
if (choice < 1 || choice > 9 || (board[(choice - 1) / 3][(choice - 1) % 3] != 'X' && board[(choice - 1) / 3][(choice - 1) % 3] != 'O')) {
cout << "Invalid move! Try again.\n";
continue;
}
board[(choice - 1) / 3][(choice - 1) % 3] = currentPlayer;
turns++;
if (checkWin()) {
drawBoard();
cout << "Player " << currentPlayer << " wins!\n";
break;
}
if (turns == 9) {
drawBoard();
cout << "It's a draw!\n";
break;
}
currentPlayer = (currentPlayer == 'X') ? 'O' : 'X';
}
return 0;
}
What is Tic Tac Toe?
Tic Tac Toe is a classic game enjoyed by people of all ages, originating from ancient times. Its simplicity makes it an ideal gateway for learning programming concepts. The game is played on a 3x3 grid where two players take turns placing their symbols—commonly an 'X' and an 'O'—in an attempt to be the first to get three in a row, either vertically, horizontally, or diagonally.

Why Create a Tic Tac Toe Game in C++?
Creating a Tic Tac Toe C++ game serves multiple educational purposes. It allows beginners to grasp fundamental programming concepts such as:
- Control Structures: Understanding if-else logic for gameplay decisions.
- Loops: Utilizing loops for repeated actions like displaying the board.
- Arrays: Handling arrays to store the game state.
By engaging in game development, learners apply theoretical knowledge in a practical context, especially advantageous in a language like C++ that emphasizes strong foundational skills.

Setting Up Your C++ Development Environment
Choosing the Right IDE for C++
Your choice of Integrated Development Environment (IDE) can greatly affect your learning experience. Popular options for C++ include:
- Code::Blocks: Lightweight and user-friendly, ideal for beginners.
- Visual Studio: A robust solution, especially for Windows users, with extensive features.
- CLion: A powerful IDE with comprehensive code assistance and navigation.
Each of these options provides an environment designed to streamline coding, compiling, and debugging processes.
Compiling and Running Your First C++ Program
Before diving into the Tic Tac Toe C++ game, it's essential to know how to compile and run a basic C++ program. Start with a simple "Hello, World!" example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Compile and run this code in your chosen IDE to ensure everything is set up correctly.

Designing the Tic Tac Toe Game
Understanding Game Structure
To craft an effective game, it’s crucial to break the project into manageable parts. The main components include:
- Game Board: The visual representation of the grid.
- User Input: Mechanism for players to indicate their moves.
- Win Conditions: Logic to check if either player has won.
- Replay Feature: Allowing players to start a new game after finishing.
Creating the Game Board
The board can be represented using a 2D array in C++. Here’s how to initialize it:
char board[3][3] = { {' ', ' ', ' '}, {' ', ' ', ' '}, {' ', ' ', ' '} };
This array holds the state of each cell on the board, which can be empty at the start.

Building the Game Logic
Displaying the Game Board
A function to print the current state of the board is essential for user interaction. Below is an example:
void printBoard() {
for (int i = 0; i < 3; i++) {
std::cout << " " << board[i][0] << " | " << board[i][1] << " | " << board[i][2] << std::endl;
if (i < 2) std::cout << "-----------" << std::endl;
}
}
This function utilizes a loop to display each row and separate them with lines, making the interface more user-friendly.
Handling User Input
It’s important to gather input from players correctly. Here's how you can implement a simple input function:
void getInput(int player) {
int x, y;
std::cout << "Player " << player << ", enter row and column (0-2): ";
std::cin >> x >> y;
}
Note: You must validate input to ensure the cells are unoccupied and in the correct range (0 to 2).
Switching Turns Between Players
Managing player turns is straightforward. This code snippet demonstrates toggling between two players:
int currentPlayer = 1;
currentPlayer = (currentPlayer == 1) ? 2 : 1;
This logic switches the player number with each turn.
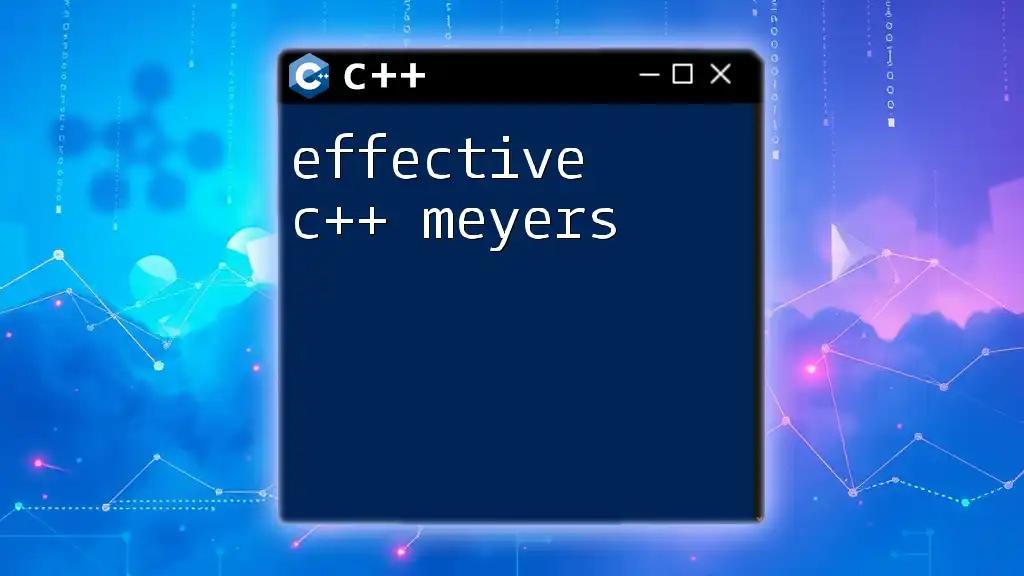
Checking for Winning Conditions
Vertical, Horizontal, and Diagonal Checks
To determine if a player has won, you must define conditions that check for three matching symbols in a row:
bool checkWin() {
// Check rows, columns, and diagonals for a winner
}
In this function, implement logic to iterate through possible winning combinations. This is a critical component that encapsulates the game's logic.
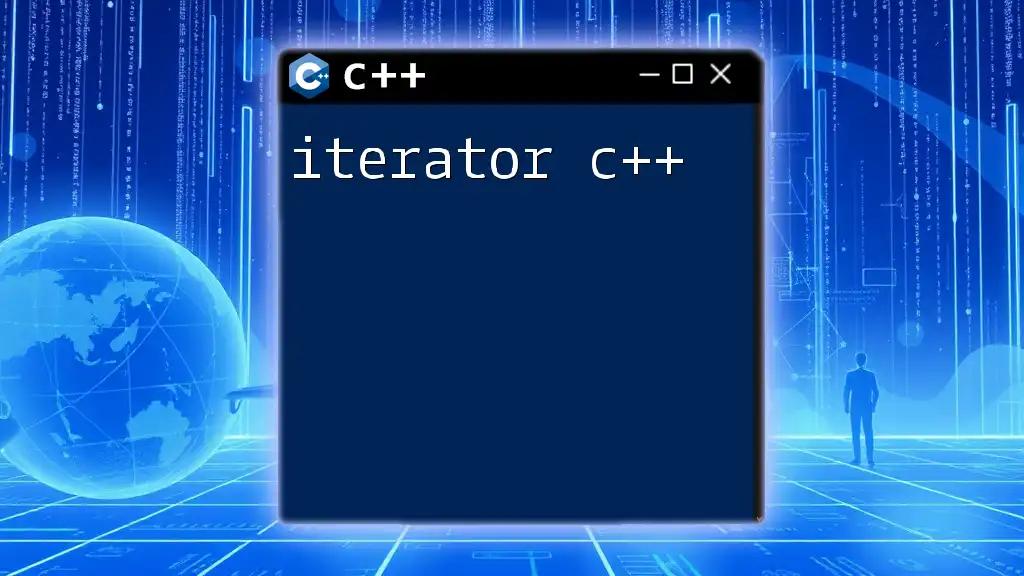
Implementing Game Replay Logic
After a game concludes, give players the option to play again. Consider this simple implementation:
char choice;
std::cout << "Play again? (y/n): ";
std::cin >> choice;
This allows the game to restart easily based on players' preferences.
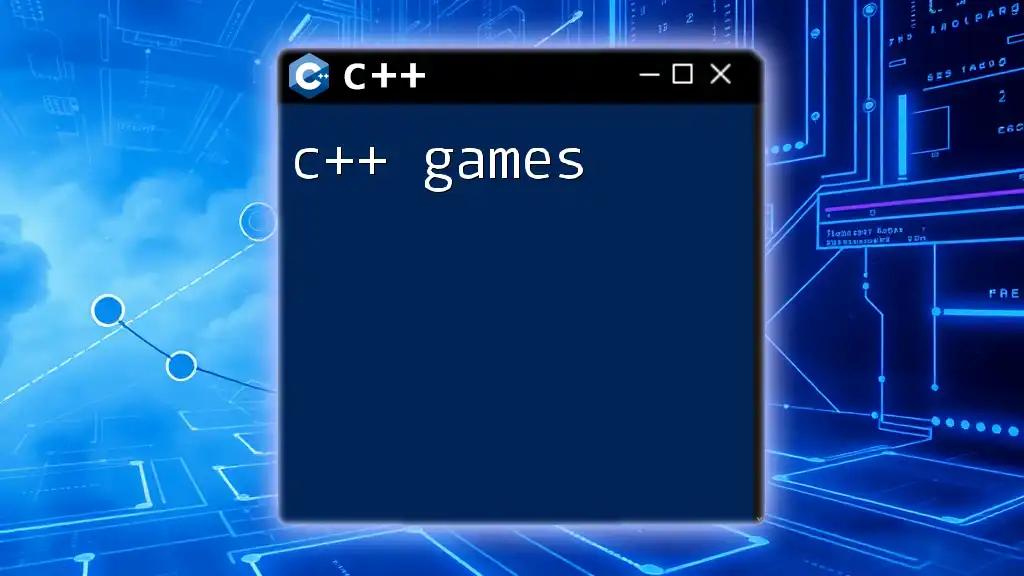
Complete Code for Tic Tac Toe in C++
Here's a complete example of a Tic Tac Toe game in C++. Gather all the previous components into a single functioning program:
#include <iostream>
char board[3][3] = { {' ', ' ', ' '}, {' ', ' ', ' '}, {' ', ' ', ' '} };
void printBoard();
void getInput(int player);
bool checkWin();
void resetBoard();
int main() {
int currentPlayer = 1;
bool gameRunning = true;
while (gameRunning) {
printBoard();
getInput(currentPlayer);
// Logic to update the board and check for win here...
// Assuming a win condition and checking for replay...
char choice;
std::cout << "Play again? (y/n): ";
std::cin >> choice;
if (choice != 'y') gameRunning = false;
}
return 0;
}
This code pulls together the various functions and flow of the game, demonstrating how each piece interacts within the program.

Enhancing Your Tic Tac Toe Game
Adding AI Opponent
To make the game more engaging, consider implementing a simple AI opponent. The AI could decide moves randomly or follow basic strategies. Here’s a basic structure for the AI move:
void aiMove() {
// Logic for AI to make a move
}
This function gives you the freedom to explore more complex algorithms, such as implementing the Minimax strategy for an unbeatable AI.
User Interface Improvements
Although a console application is functional, you can enhance the user interface using libraries such as ncurses or SFML for a more graphical presentation. This allows for a wider array of interactive capabilities.
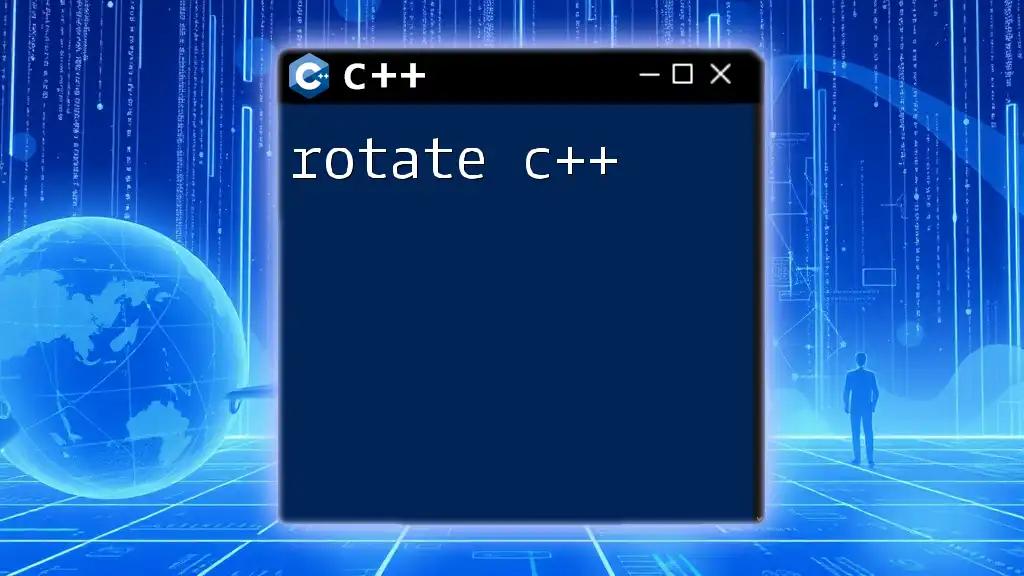
Conclusion
Creating a Tic Tac Toe C++ game is a fantastic way to practice programming, develop problem-solving skills, and understand game logic deeply. This engaging project encourages experimentation and customization, allowing you to add your personal flair. As you become more comfortable with the concepts, you can expand the game further, perhaps by introducing new features or sophisticated AI logic.
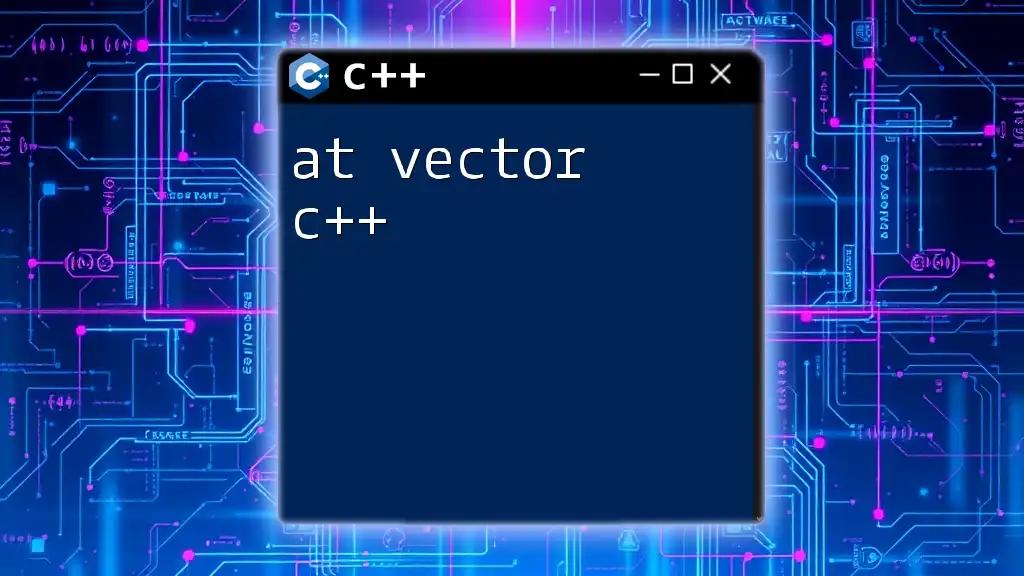
Additional Resources
To continue learning, consider exploring C++ documentation, tutorials, and programming forums like Stack Overflow, where you can ask questions and share your projects.
Call to Action
Now it's your turn! Try building your own version of Tic Tac Toe in C++. Experiment with different features, styles, and add your unique twists. Happy coding!