In this post, we'll create a simple command-line Tic Tac Toe game in C++ that allows two players to take turns placing their marks on a 3x3 grid.
#include <iostream>
using namespace std;
char board[3][3] = {{'1','2','3'},{'4','5','6'},{'7','8','9'}};
char player = 'X'; // Current player
void printBoard() {
cout << "Current board layout:\n";
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
cout << board[i][j] << " ";
}
cout << endl;
}
}
bool checkWin() { /* Implementation of win check logic */ }
void switchPlayer() { player = (player == 'X') ? 'O' : 'X'; }
int main() {
int choice;
for (int turn = 0; turn < 9; turn++) {
printBoard();
cout << "Player " << player << ", enter a number (1-9): ";
cin >> choice;
/* Update board logic and check for a win */
switchPlayer();
}
cout << "It's a draw!";
return 0;
}
This code sets up the game board and the turns for players, while you can implement additional logic to check for wins and updates to the board.
Setting Up Your C++ Environment
To start developing your C++ Tic Tac Toe game, you need a suitable Integrated Development Environment (IDE) and compiler. Here are some popular choices:
- Visual Studio: A powerful IDE perfect for C++ development.
- Code::Blocks: Lightweight and easy to use for beginners.
- CLion: A more advanced option with smart code completion features.
To install your chosen IDE, visit its official website and follow the installation instructions. After installation, create a simple "Hello, World!" program to ensure your environment is set up correctly. This will also help you get accustomed to compiling and running basic C++ code.
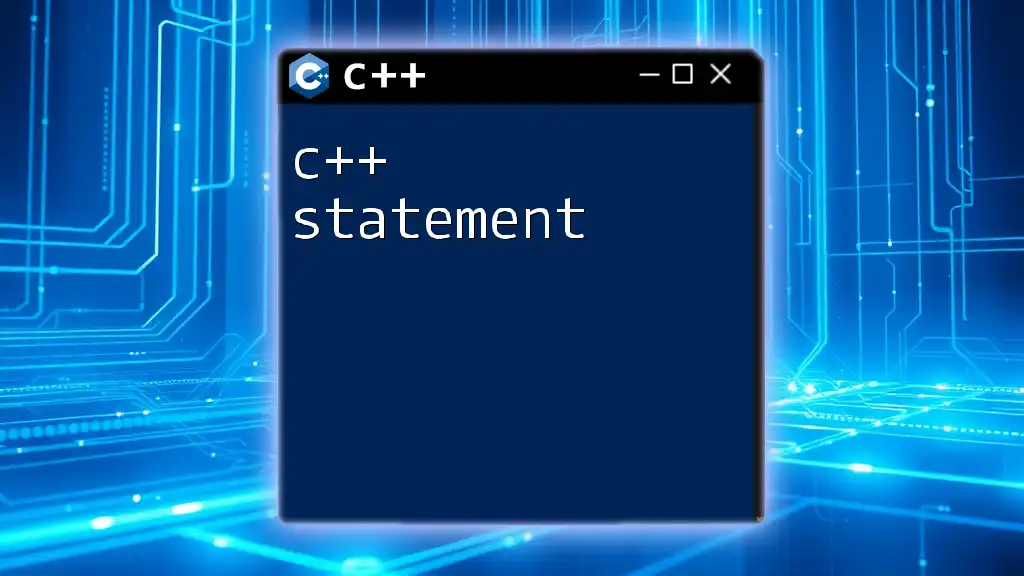
Understanding the Rules of Tic Tac Toe
Before jumping into coding, it's essential to understand the rules of Tic Tac Toe. The game is played on a 3x3 grid where two players take turns marking either an 'X' or an 'O' in the empty cells. The objective is to get three of your marks in a row—vertically, horizontally, or diagonally.
This understanding will inform your programming logic as you translate the game's mechanics into code.
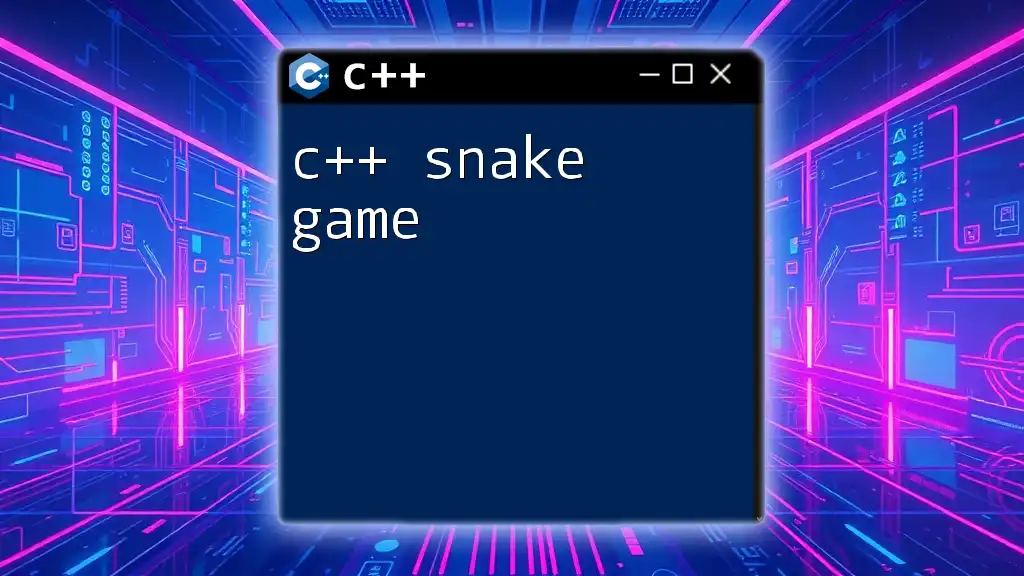
Designing the Tic Tac Toe Game
Game Structure
Your C++ Tic Tac Toe game will need a few components:
- Board Representation: How the board state is stored.
- Player Representation: How the players are identified and their respective marks.
A solid choice for the board representation is a 2D array, which allows you to easily track the state of each cell.
Choosing the Right Data Structures
Using arrays is beneficial for fixed-size structures like a tic tac toe board, while vectors are more flexible if you ever want to resize or modify the structure dynamically.
Game Flow
Define the core counter states of the game:
- Start State: Initialize the game by setting up the board.
- Player Turns: Alternate player input until the game ends.
- End State: Check for win/lose/tie conditions.
Understanding these states will help you create a loop that drives the game until a conclusion is reached.
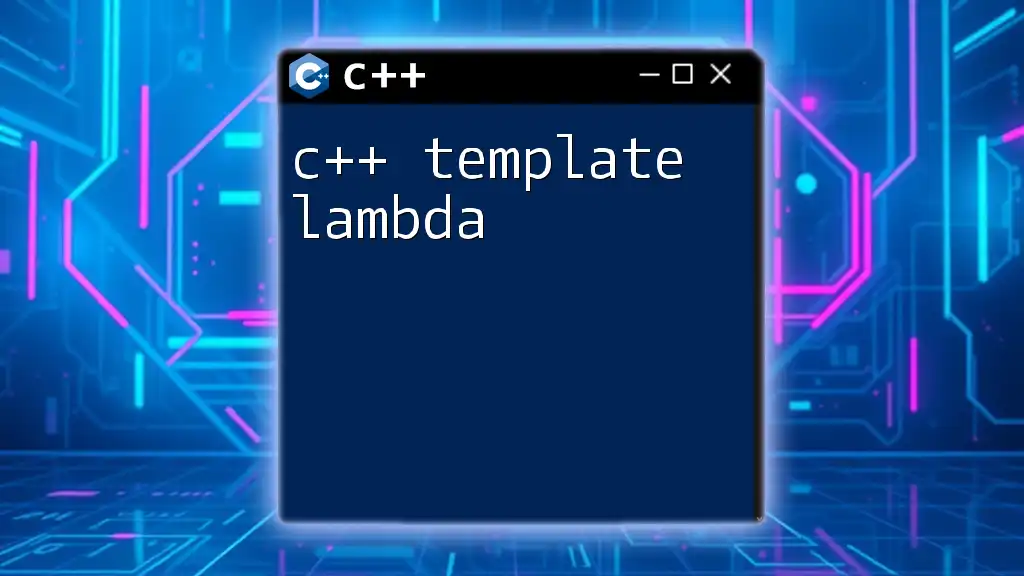
Implementing the Tic Tac Toe Game
Board Representation
For representing the board, you can initialize a simple 2D array:
char board[3][3] = { {' ', ' ', ' '}, {' ', ' ', ' '}, {' ', ' ', ' '} };
This code snippet creates a 3x3 board filled with spaces, indicating that no moves have been made yet.
Player Input
You will need to take input from players regarding their moves. The code snippet below illustrates how to capture this input:
int row, col;
cout << "Enter row and column (0, 1, or 2): ";
cin >> row >> col;
To ensure that players do not make invalid moves, implement input validation that checks if the chosen cell is within the board's boundaries and if it is already occupied.
Game Logic
Checking for Win Conditions
The game logic involves checking whether a player has won after each turn. For this, you'll implement a function to inspect rows, columns, and diagonals for a winning condition:
bool checkWin(char player) {
// Check rows and columns
for (int i = 0; i < 3; i++) {
if ((board[i][0] == player && board[i][1] == player && board[i][2] == player) ||
(board[0][i] == player && board[1][i] == player && board[2][i] == player)) {
return true;
}
}
// Check diagonals
if ((board[0][0] == player && board[1][1] == player && board[2][2] == player) ||
(board[0][2] == player && board[1][1] == player && board[2][0] == player)) {
return true;
}
return false;
}
This function checks for three consecutive marks on the board and returns `true` if a player has won.
Displaying the Board
To improve user experience, it’s important to visually display the board after each turn. Here’s a simple function to print the board state:
void printBoard() {
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
cout << board[i][j];
if (j < 2) cout << " | ";
}
cout << endl;
if (i < 2) cout << "---------" << endl;
}
}
This function formats the board neatly, helping players see their moves clearly.
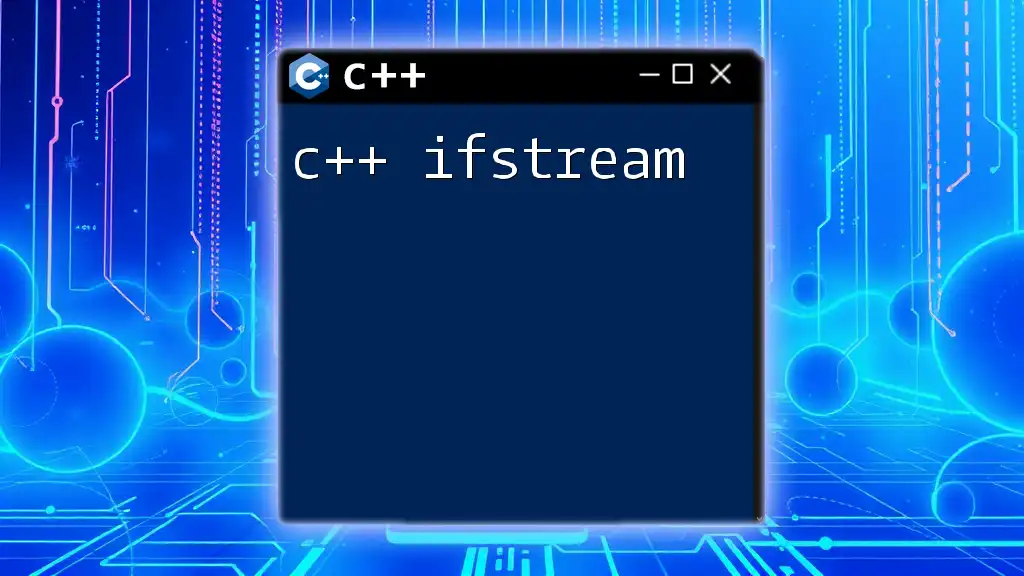
Adding Features to Enhance Gameplay
AI Opponent
As an advanced feature, you can introduce an AI opponent using the concept of randomness. To implement a simple AI that makes random moves, you would generate random row and column values until an empty cell is found. While this simple approach is a good start, you could later integrate more complex strategies like the Minimax algorithm for a challenging AI experience.
Game Restart Feature
To enable a seamless transition between games, provide an option for players to restart. You can implement this using a simple prompt after the game ends:
char playAgain;
cout << "Play again? (y/n): ";
cin >> playAgain;
Based on user input, you can reset the game state and allow for new gameplay.
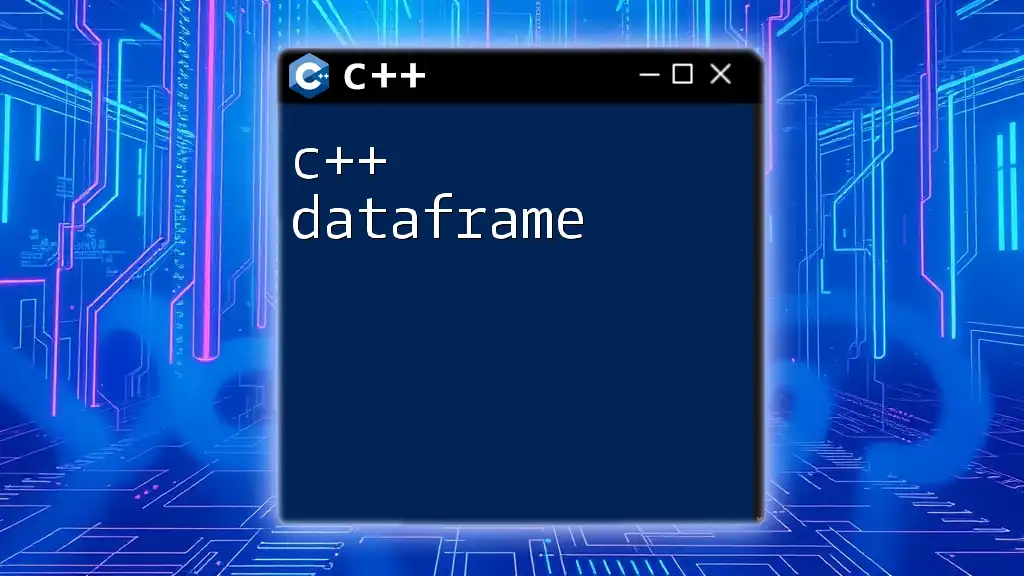
Testing Your Tic Tac Toe Game
Testing is a crucial part of software development. Manually test your game by playing it several times to identify bugs, such as invalid move scenarios or win condition checks that may not work as expected. Pay attention to common bugs, such as boundary errors or mismatched player inputs. Debugging is an essential skill to develop as you refine your C++ Tic Tac Toe game.
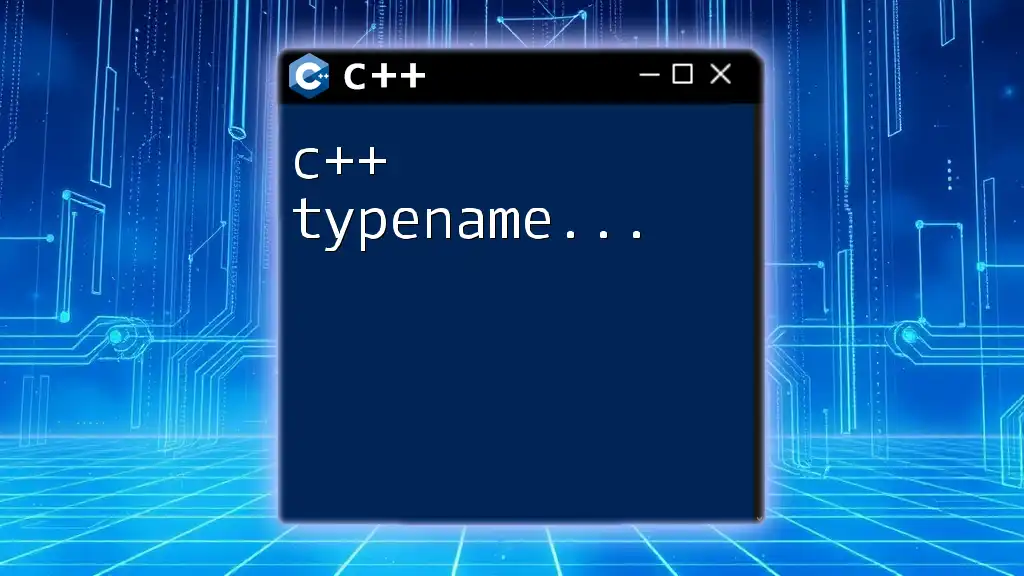
Conclusion
In this guide, you've learned how to build a C++ Tic Tac Toe game from the ground up. From understanding the rules of the game and designing the structure to implementing the actual game logic, you're now equipped with the knowledge to create your own version. Exploring additional features like AI opponents and game restarts can significantly enhance the player's experience. Dive deeper into C++ game development and don’t hesitate to share your own improvements and new features!