In C++, you can retrieve the local time using the `<ctime>` library and the `std::localtime` function, as shown in the following code snippet:
#include <iostream>
#include <ctime>
int main() {
std::time_t now = std::time(nullptr);
std::cout << "Local time: " << std::asctime(std::localtime(&now));
return 0;
}
Understanding Local Time in C++
In programming, local time refers to time calculated based on the current timezone settings of the operating system. This is crucial when developing applications that log events, timestamps, or when working with user interfaces that rely on current locale settings. As a developer, understanding how to manipulate and use local time in C++ can significantly enhance your application's usability and functionality.
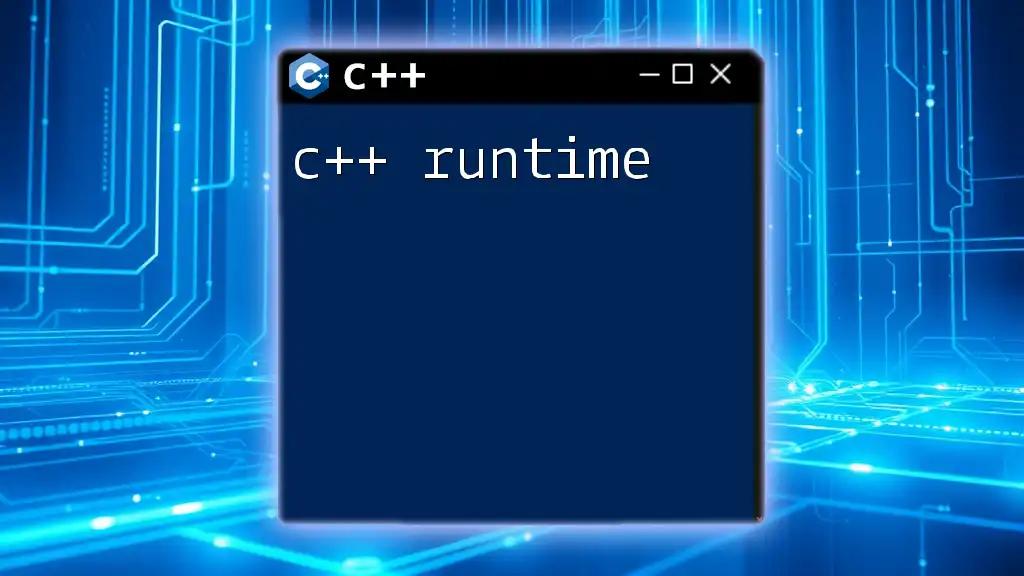
The C++ Time Library
The `<ctime>` header in C++ is your go-to for handling date and time. It provides functions for accessing and manipulating time values, based on the methods provided by the C Standard Library. Some of the key functions you’ll come across include `std::time()`, `std::localtime()`, and `std::strftime()`, which play a vital role in getting current time, converting it to local time, and formatting it respectively.
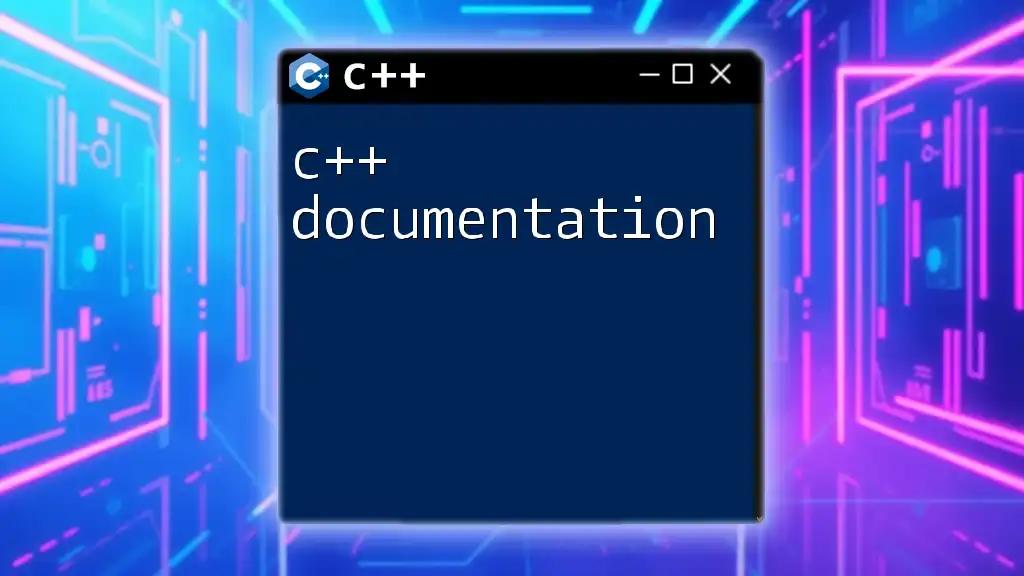
Working with Local Time
Getting Current Local Time
To fetch the current local time, you can use `std::time()` along with `std::ctime()`. Below is an example demonstrating how to obtain and display the current local time:
#include <iostream>
#include <ctime>
int main() {
std::time_t currentTime = std::time(nullptr); // Get current time
std::cout << "Current time: " << std::ctime(¤tTime);
return 0;
}
In this code snippet, `std::time(nullptr)` returns the current time as a `time_t` object, which is then converted to a human-readable format using `std::ctime()`. It's important to understand that `std::ctime()` formats the time in the local timezone of the system running the code.
Converting Time to Local Time
Using `std::localtime()` allows you to convert `time_t` objects directly to local time, providing a `tm` structure that contains components such as year, month, day, hour, minute, and second. Here’s a simple example:
#include <iostream>
#include <ctime>
int main() {
std::time_t currentTime = std::time(nullptr);
std::tm* localTime = std::localtime(¤tTime);
std::cout << "Local time: " << std::asctime(localTime);
return 0;
}
The `std::localtime()` function converts the `currentTime` into a `tm` structure containing the local time, which is then printed using `std::asctime()`. This representation is highly readable and showcases the exact local time tailored to the system settings.
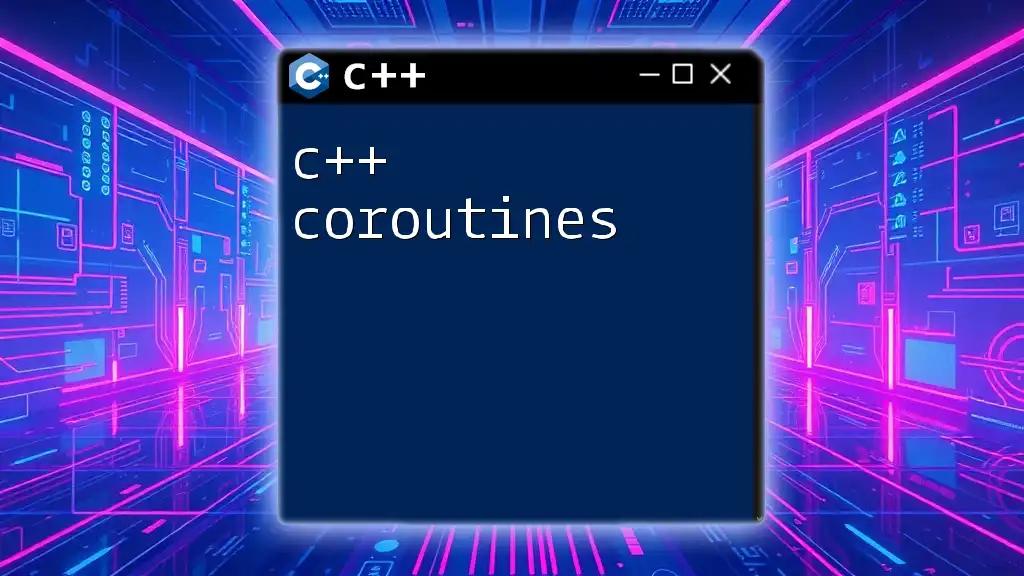
Formatting Local Time
Using `strftime` for Custom Formatting
For cases requiring specific formats, `strftime()` is the function you’ll utilize. It allows you to create a formatted string representation of time. Here’s an example demonstrating how to format local time:
#include <iostream>
#include <ctime>
int main() {
std::tm* localTime;
std::time_t currentTime = std::time(nullptr);
localTime = std::localtime(¤tTime);
char buffer[80];
std::strftime(buffer, sizeof(buffer), "%Y-%m-%d %H:%M:%S", localTime);
std::cout << "Formatted local time: " << buffer << std::endl;
return 0;
}
In this code, `strftime` uses format specifiers like `%Y` for the year, `%m` for the month, and `%d` for the day. This flexibility allows you to tailor the output to meet your application requirements or user preferences.

Practical Use Cases
Logging Events with Local Time
In many applications, particularly those involving auditing or debugging, it is essential to log events with timestamps. Here’s an example that demonstrates how to effectively include local time in your logs:
#include <iostream>
#include <ctime>
void logEvent(const std::string& event) {
std::time_t currentTime = std::time(nullptr);
std::tm* localTime = std::localtime(¤tTime);
char buffer[80];
std::strftime(buffer, sizeof(buffer), "%Y-%m-%d %H:%M:%S", localTime);
std::cout << "[" << buffer << "] Event: " << event << std::endl;
}
int main() {
logEvent("Application started");
// ... other events
return 0;
}
This function captures the current time, formats it, and outputs it alongside the event description, ensuring that each logged item is timestamped accurately.
Handling Time Zones
Dealing with various time zones can be a challenge in software applications. While C++ itself does not handle time zones directly with the standard library, you might set the `TZ` environment variable using `setenv()` on POSIX systems to adjust your program’s timezone before calling time functions. This ensures that the local time returned by `std::localtime()` reflects the desired zone.

Tips and Best Practices
When working with C++ local time, keep in mind:
- Be mindful of your system's timezone settings, as they impact how local time is computed.
- Consistently use formatting functions to keep your logs uniform and easily readable.
- Avoid mixing UTC and local time within your application to prevent confusion.
- Practice safe programming by checking return values where applicable to ensure valid operations, especially with time functions.

Conclusion
In summary, understanding how to manipulate C++ local time effectively can enhance your applications' functionality and user experience greatly. From logging events to formatting timestamps, mastering local time manipulation provides significant advantages.
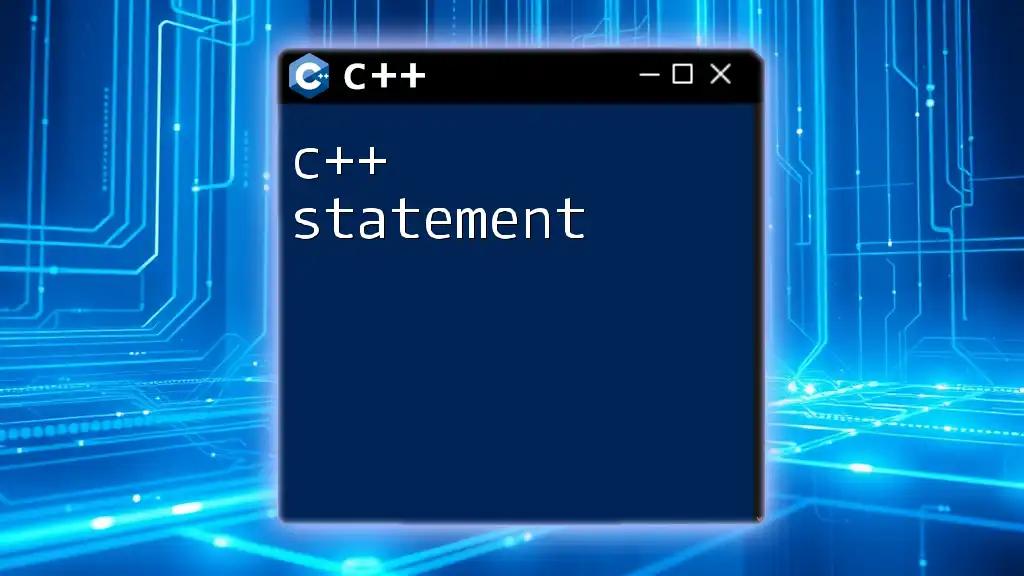
Further Reading
For those keen on diving deeper into C++, explore resources such as the official C++ documentation for `<ctime>`, `std::localtime`, and `strftime`. They offer further insights and examples for more advanced time and date handling scenarios.
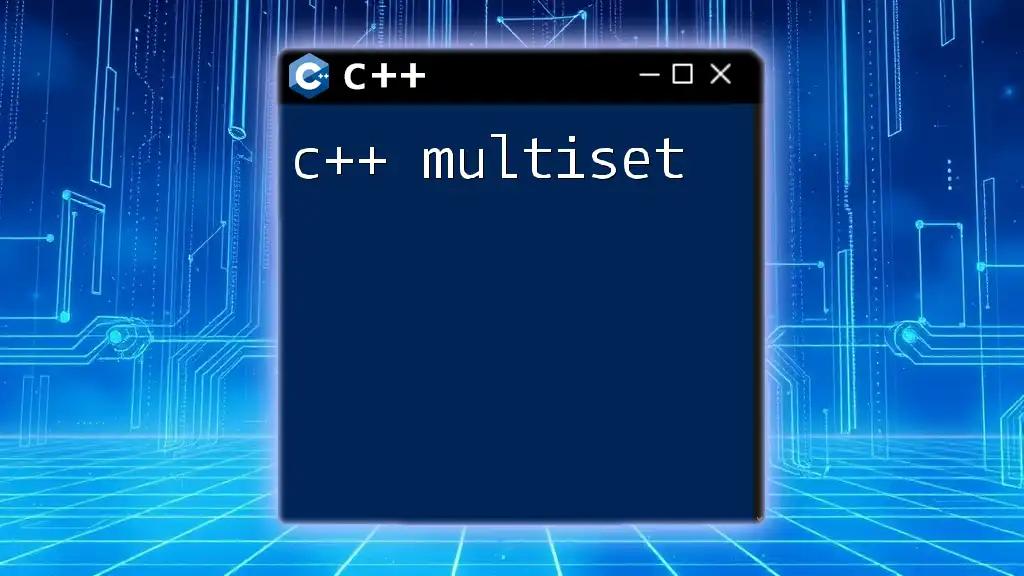
FAQs
What is the difference between UTC and local time?
UTC (Coordinated Universal Time) is a time standard that is not subject to time zones or daylight saving time adjustments. Local time, on the other hand, is based on the geographical location's time zone settings.
How can I calculate differences between two local times?
You can retrieve two `time_t` values and subtract them to determine the difference in seconds. You can then format this difference into a more user-friendly representation if needed.
Can C++ handle milliseconds in local time?
While the basic C++ standard library does not provide millisecond precision directly, you can work with time using additional libraries such as `<chrono>` in C++11 and later, which offer high-resolution clock functionalities.