A C++ optimizer is a tool or compiler feature that improves the efficiency and performance of C++ code by analyzing and transforming it to reduce resource consumption and execution time.
#include <iostream>
int main() {
int sum = 0;
for (int i = 1; i <= 100; ++i) {
sum += i;
}
std::cout << "Sum of first 100 integers: " << sum << std::endl;
return 0;
}
In this example, a C++ optimizer could unroll the loop or apply other transformations to enhance performance.
Understanding C++ Optimization
What is C++ Optimization?
C++ optimization is the process of making a C++ program faster and more efficient, which often involves modifying the code, choosing the right algorithms, and using proper data structures. The goal is to enhance performance while maintaining code readability and functionality. Optimization can lead to reduced run times, better memory management, and an overall improved user experience.
Why Optimize C++ Code?
Optimizing C++ code is crucial for several reasons:
- Reduction in Execution Time: Efficient code leads to faster computations, making applications more responsive, particularly in performance-critical applications such as games, simulations, and real-time systems.
- Minimization of Memory Usage: By using resources judiciously, optimized code can handle larger datasets and complex processes without crashing or slowing down a system.
- Improved Responsiveness: In interactive applications, optimization helps ensure that users experience minimal lag and delays.

Types of C++ Optimization
Compile-Time Optimization
Compile-time optimization involves improvements made by the compiler before code execution. The compiler analyzes the code and applies several strategies, including constant folding and dead code elimination. When you compile a C++ program with optimization flags such as `-O2` or `-O3`, the compiler performs various analyses and transformations to speed up the generated binary.
Example of using optimization flags during compilation:
g++ -O2 -o my_program my_program.cpp
Run-Time Optimization
Run-time optimization occurs during the execution of the program. For instance, the C++ Standard Library provides containers with algorithms optimized for run-time performance. Dynamic memory management techniques, such as memory pooling and object reuse, can significantly enhance run-time efficiency.
Consider this example of dynamic memory handling:
#include <iostream>
#include <vector>
int main() {
std::vector<int*> pool;
for (int i = 0; i < 100; i++) {
int* ptr = new int(i);
pool.push_back(ptr);
}
// Use the allocated memory...
for (auto ptr : pool) {
delete ptr; // Important to free the memory!
}
return 0;
}
Algorithmic Optimization
Choosing the right algorithms can be a game-changer in performance. For example, selecting a more efficient sorting or searching algorithm can drastically affect the time complexity of your program.
- Sorting Algorithms: For instance, using quicksort (`O(n log n)`) instead of bubble sort (`O(n^2)`) for larger datasets can yield significant speed improvements.
- Searching Algorithms: Implementing binary search on a sorted array is preferable to linear search, especially as the dataset size increases.
Example illustrating a simple binary search:
#include <iostream>
#include <vector>
int binarySearch(const std::vector<int>& arr, int target) {
int left = 0;
int right = arr.size() - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == target) {
return mid; // Target found
}
if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1; // Target not found
}
Code-Level Optimization
Writing optimal code often requires making small but impactful changes. Here are a few strategies:
- Minimizing the Use of Unnecessary Variables: Unused variables consume memory and may lead to confusion. Declaring them only when necessary is key.
- Avoiding Deep Nesting of Loops: Nested loops can exponentially increase execution time. Optimizing your logic may allow for fewer nested structures, leading to cleaner and faster iterations.
Below is a poor vs. optimized code example:
// Poorly optimized code
for (int i = 0; i < 1000; i++) {
for (int j = 0; j < 1000; j++) {
std::cout << i * j; // Heavy processing in nested loops
}
}
// Optimized code
for (int i = 0; i < 1000; i++) {
int result = i * j; // Move computation outside
std::cout << result;
}

C++ Code Optimization Techniques
Using Efficient Data Structures
Choosing the appropriate data structures is foundational to optimization. C++ provides various containers, each with its advantages and trade-offs:
- Arrays: Use when the size is known and fixed.
- Vectors: Ideal when size needs to be dynamic with the benefit of array-like access.
- Lists: Use for frequent insertions and deletions.
For example, using `std::vector` can simplify and optimize memory management compared to raw arrays.
Compiler Optimizations
Compiler optimizations through specific flags can greatly enhance performance. These flags instruct the compiler to use various strategies during the translation of code, including loop unrolling and function inlining, which can significantly reduce function call overhead.
Example:
g++ -O3 -o my_program my_program.cpp
Inlining Functions
Function inlining is a technique where the compiler replaces a function call with the actual function code. This is effective for small functions that are called frequently, thereby reducing function call overhead.
Example:
inline int square(int x) {
return x * x; // This function will be inlined at the call site
}
Memory Management Optimization
In C++, manual memory management can lead to issues like memory leaks and fragmentation. Using smart pointers (`std::unique_ptr`, `std::shared_ptr`) helps manage memory automatically and efficiently.
Example of using smart pointers:
#include <memory>
void useSmartPointer() {
std::unique_ptr<int> ptr = std::make_unique<int>(10);
std::cout << *ptr; // Automatically managed memory
}

Tools for C++ Optimization
Profilers
Profilers are invaluable for optimization by providing insights into where your program spends the most time. Tools like `gprof` or `Valgrind` can help you understand performance bottlenecks and memory usage.
To use gprof:
- Compile your program with the `-pg` option.
- Execute your program.
- Run `gprof` on the produced profile data.
Static Analysis Tools
Static analysis tools help identify inefficiencies during development, allowing early detection of performance issues. Tools like Clang-Tidy and CPPCheck can catch potential pitfalls and enforce best practices.
Debugging Tools
Debugging tools are crucial for identifying logical errors that can affect performance. GDB and the Visual Studio Debugger offer powerful capabilities to step through code, inspect variable states, and catch inefficient algorithms before they cause trouble.

Best Practices for C++ Optimization
Code Readability vs. Optimization
While optimization is essential, it shouldn't come at the cost of code readability. A balanced approach is crucial—maintain clear, understandable code while implementing optimization techniques. Guides such as single-responsibility functions and clear variable names help achieve this balance.
Continuous Optimization
Optimization should not be a one-time event but an ongoing process. Engaging your team in a culture of optimization can enhance project quality. Regular code reviews and performance evaluations can contribute significantly to maintaining robustness and efficiency in software development.
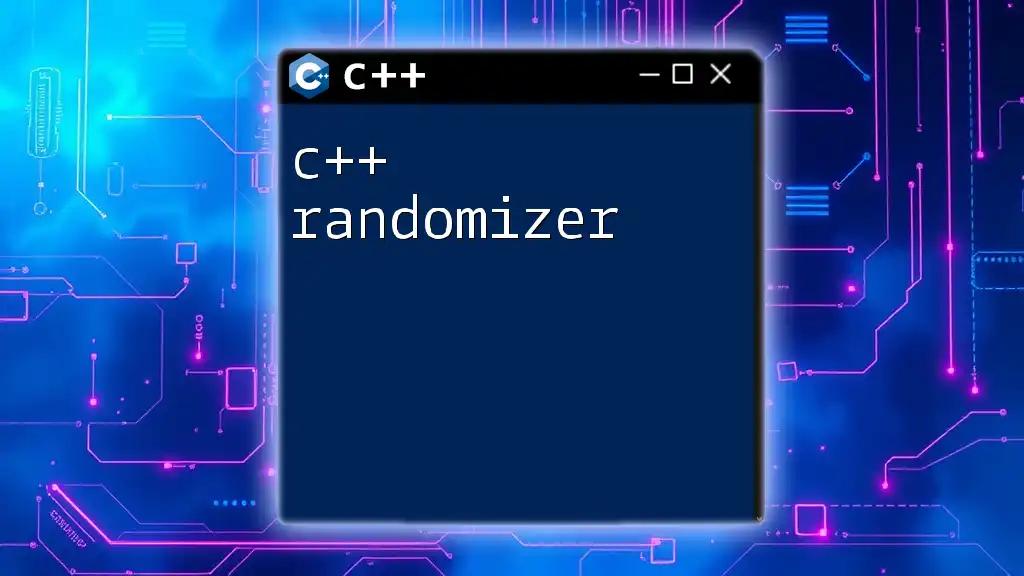
Conclusion
C++ optimization is an essential part of software development, directly impacting performance, memory usage, and user experience. By understanding and applying various optimization techniques—ranging from compile-time and run-time optimizations to effective use of data structures and algorithms—you can significantly improve your C++ applications. Embrace ongoing optimization practices to ensure continued efficiency and performance in your projects.
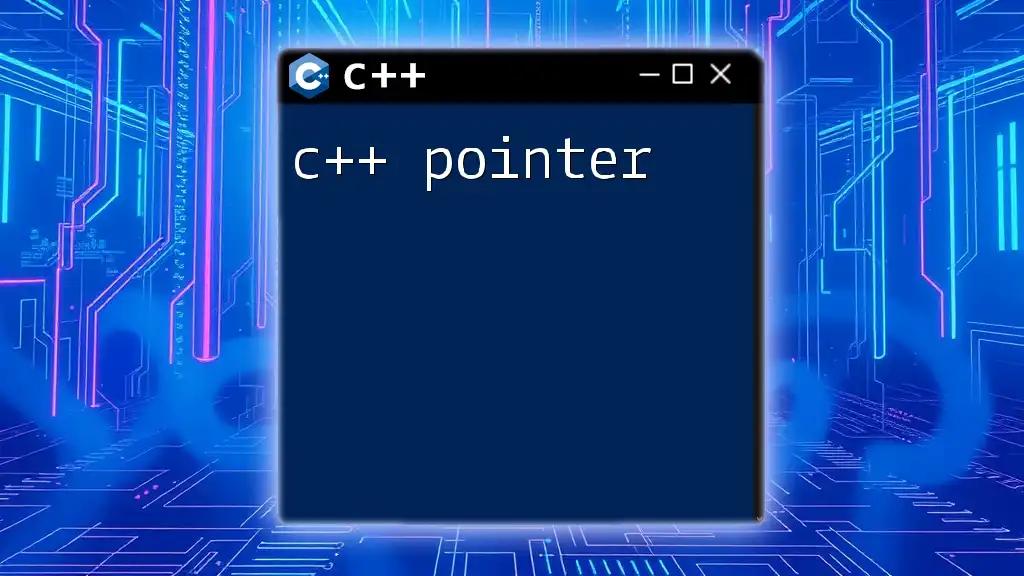
Call to Action
Have you implemented any of these optimization techniques in your C++ projects? Share your experiences and insights with us! Also, consider subscribing for more tips and guides on effective C++ programming.

References
For further reading on C++ optimization techniques and best practices, consider exploring established resources, online tutorials, and forums dedicated to C++ programming and optimization strategies.