In C++, the `<chrono>` library enables precise time measurement and manipulation, allowing developers to track durations and intervals easily.
Here's a simple example demonstrating how to measure the execution time of a code block:
#include <iostream>
#include <chrono>
int main() {
auto start = std::chrono::high_resolution_clock::now();
// Sample code block
for (int i = 0; i < 1000000; ++i);
auto end = std::chrono::high_resolution_clock::now();
std::chrono::duration<double> duration = end - start;
std::cout << "Execution time: " << duration.count() << " seconds" << std::endl;
return 0;
}
Understanding the C++ Time Library
In C++, time handling is crucial for a variety of applications ranging from simple logging to complex scheduling systems. The C++ Time Library provides developers with robust tools to effectively manage and manipulate time.
Key Concepts and Terminology
Epoch Time refers to the number of seconds that have elapsed since a specific date and time, often set at January 1, 1970, at UTC. Understanding epoch time is foundational for time calculations.
Time Units in C++ include seconds, milliseconds, and microseconds. These units allow you to specify time intervals precisely, which is vital when measuring durations.
Time Points are specific moments in time while Durations represent spans of time. A clear distinction between these two is essential for accurate time manipulation.
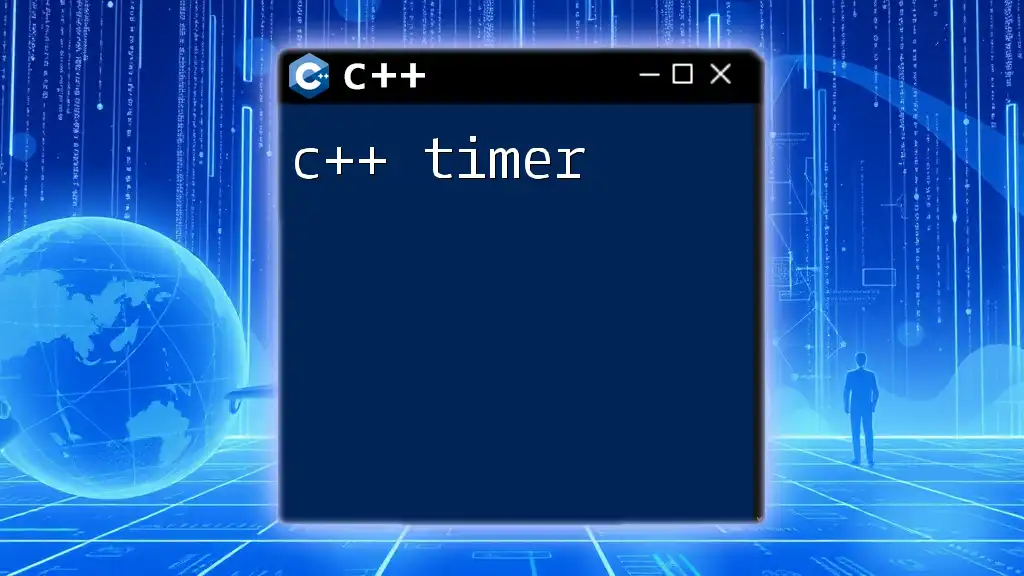
Getting Started with the `<chrono>` Library
Introduction to the `<chrono>` Header
The `<chrono>` header offers a powerful set of tools for time manipulation without the complexities often associated with traditional handling. Using `<chrono>` can lead to cleaner, more readable, and less error-prone code.
Components of `<chrono>`
Time Points
A time point represents a moment in time. You can obtain the current time using the system clock like so:
#include <chrono>
#include <iostream>
int main() {
auto now = std::chrono::system_clock::now();
std::cout << "Current time point: " << now.time_since_epoch().count() << std::endl;
return 0;
}
This snippet effectively retrieves the current system time as a time point, allowing you to manipulate or format it as required.
Durations
A duration is a way to express time intervals and can be defined using various types, such as:
#include <chrono>
std::chrono::seconds s(60); // 60 seconds
std::chrono::milliseconds ms(1000); // 1 second in milliseconds
You can also perform calculations with durations, as shown below:
#include <chrono>
#include <iostream>
int main() {
std::chrono::seconds duration1(5);
std::chrono::seconds duration2(3);
auto total_duration = duration1 + duration2; // Result will be 8 seconds
std::cout << "Total Duration: " << total_duration.count() << " seconds" << std::endl;
return 0;
}
Timers
Timers can be effectively created using `<chrono>` for various applications. Here's a simple usage of `std::chrono::steady_clock` to measure elapsed time:
#include <chrono>
#include <iostream>
#include <thread>
int main() {
auto start = std::chrono::steady_clock::now();
std::this_thread::sleep_for(std::chrono::seconds(2)); // Simulates a task
auto end = std::chrono::steady_clock::now();
auto elapsed = std::chrono::duration_cast<std::chrono::seconds>(end - start);
std::cout << "Elapsed time: " << elapsed.count() << " seconds" << std::endl;
return 0;
}
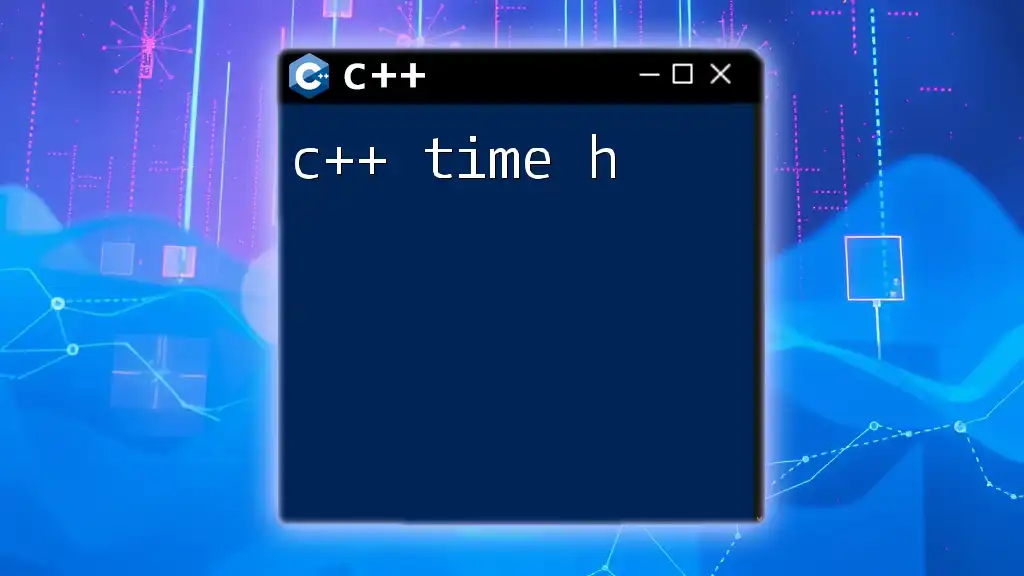
Working with Time Points
Creating and Using Time Points
Creating time points typically involves the system clock:
#include <chrono>
#include <iostream>
void printCurrentTime() {
auto now = std::chrono::system_clock::now();
std::time_t currentTime = std::chrono::system_clock::to_time_t(now);
std::cout << "Current Time: " << std::ctime(¤tTime);
}
This function retrieves and prints the current local time.
Converting Time Points
Conversion between time points and string representations is often required for logging and user interfaces. Use `std::put_time` along with `std::strftime` for formatting:
#include <chrono>
#include <iostream>
#include <iomanip>
#include <ctime>
void formatTimePoint() {
auto now = std::chrono::system_clock::now();
std::time_t currentTime = std::chrono::system_clock::to_time_t(now);
std::cout << "Formatted Current Time: "
<< std::put_time(std::localtime(¤tTime), "%Y-%m-%d %H:%M:%S") << std::endl;
}
Calculating the difference between time points can reveal how long an operation took:
#include <chrono>
#include <iostream>
#include <thread>
void measureFunction() {
auto start = std::chrono::system_clock::now();
// Simulating a task
std::this_thread::sleep_for(std::chrono::seconds(1));
auto end = std::chrono::system_clock::now();
auto duration = std::chrono::duration_cast<std::chrono::milliseconds>(end - start).count();
std::cout << "Function executed in " << duration << " milliseconds." << std::endl;
}
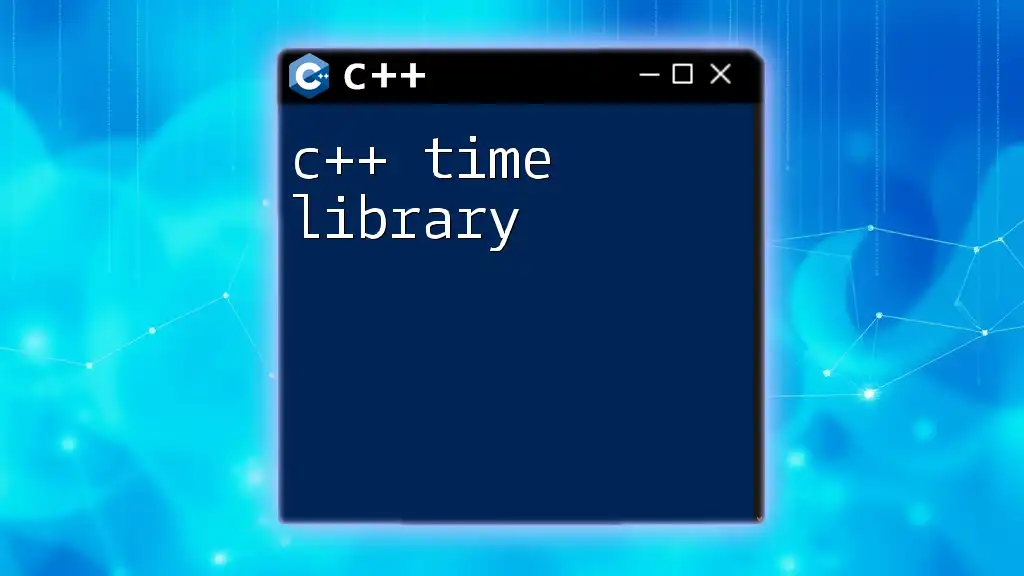
Dealing with Durations
Understanding and Creating Durations
The `<chrono>` library supports various duration types, making it simple to define time intervals depending on the context. For example:
std::chrono::duration<int> days(7); // 7 days
std::chrono::duration<double> hours(24.0); // 24 hours as double
Manipulating Durations
You can perform arithmetic operations on durations effortlessly:
#include <chrono>
#include <iostream>
int main() {
std::chrono::duration<int> durationA(5); // Represents 5 seconds
std::chrono::duration<int> durationB(10); // Represents 10 seconds
auto result = durationA + durationB; // Adds 5 + 10 seconds
std::cout << "Total Duration: " << result.count() << " seconds" << std::endl; // Outputs 15 seconds
return 0;
}
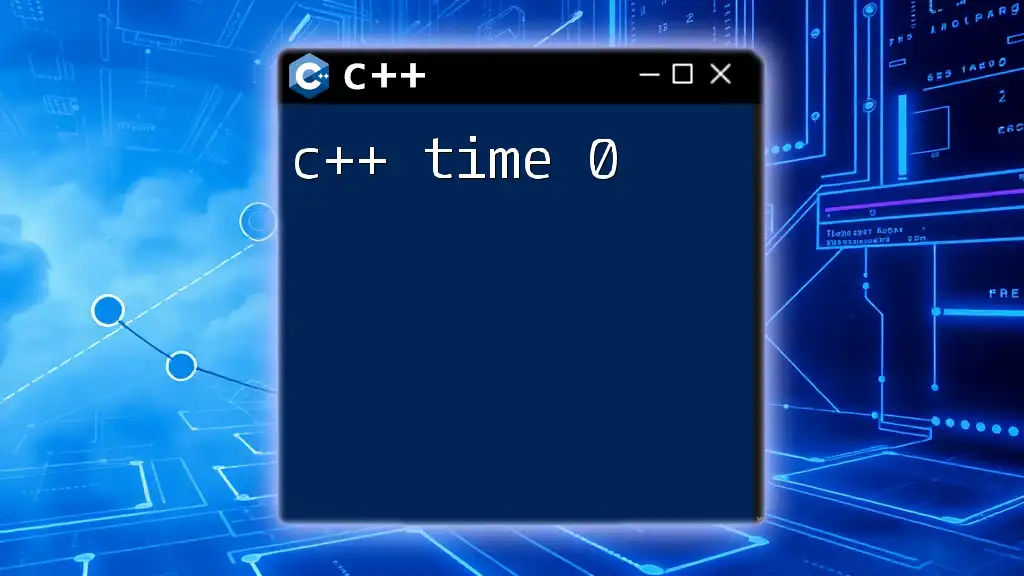
Using Clock Classes Effectively
C++ Clock Types Explained
C++ provides multiple clock types within the `<chrono>` library that serve different purposes:
- High Resolution Clock: This offers the highest precision available, suitable for benchmarking.
- Steady Clock: This clock is guaranteed to be monotonic, making it ideal for measuring intervals.
- System Clock: Represents the system's wall clock time, which can change due to adjustments.
Example: Comparing Different Clock Types
Understanding how different clocks impact timing can be insightful, especially when benchmarking functions. Here's a basic example demonstrating execution time:
#include <chrono>
#include <iostream>
void sampleFunction() {
std::this_thread::sleep_for(std::chrono::seconds(2)); // Simulating work
}
int main() {
auto start = std::chrono::high_resolution_clock::now();
sampleFunction();
auto end = std::chrono::high_resolution_clock::now();
auto duration = std::chrono::duration_cast<std::chrono::milliseconds>(end - start).count();
std::cout << "Function execution time: " << duration << " milliseconds." << std::endl;
return 0;
}
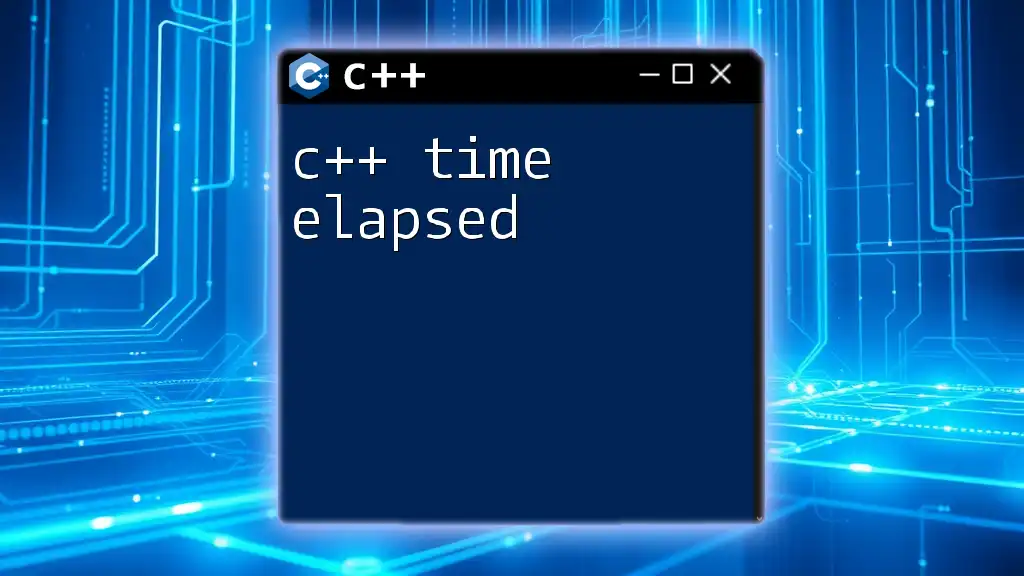
Practical Applications of Time in C++
Building a Timer Application
You can create a simple countdown timer that utilizes both time points and durations.
Here's a basic version:
#include <chrono>
#include <iostream>
#include <thread>
void countdown(int seconds) {
while (seconds > 0) {
std::cout << "Time remaining: " << seconds << " seconds" << '\r';
std::cout.flush();
std::this_thread::sleep_for(std::chrono::seconds(1));
--seconds;
}
std::cout << "Time's up!" << std::endl;
}
int main() {
countdown(10); // 10 seconds countdown
return 0;
}
Scheduling Tasks
Using durations for scheduled tasks can optimize performance. The following snippet demonstrates using a delay for executing a task:
#include <chrono>
#include <iostream>
#include <thread>
void task() {
std::cout << "Task executed after a delay!" << std::endl;
}
int main() {
std::cout << "Task will be executed in 3 seconds..." << std::endl;
std::this_thread::sleep_for(std::chrono::seconds(3));
task();
return 0;
}
Performance Timing and Benchmarking
Measuring the efficiency of your functions is crucial for optimizing performance. Here’s an example of using the `std::chrono` utilities for this purpose:
#include <chrono>
#include <iostream>
void performCompute() {
for (int i = 0; i < 1e6; ++i); // Some computational work
}
int main() {
auto start = std::chrono::steady_clock::now();
performCompute();
auto end = std::chrono::steady_clock::now();
auto elapsed = std::chrono::duration_cast<std::chrono::microseconds>(end - start);
std::cout << "Computation time: " << elapsed.count() << " microseconds." << std::endl;
return 0;
}
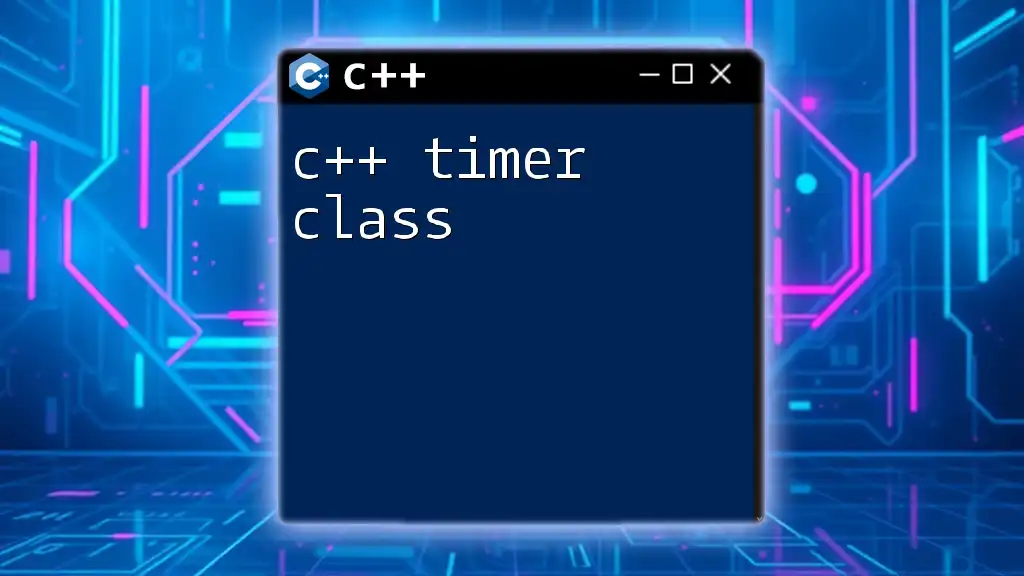
Common Challenges and Best Practices
Time Zone Handling in C++
Time zone handling in C++ can be tricky, as the standard library doesn’t provide intrinsic support. Utilizing external libraries like Howard Hinnant's Date library, or using time conversion functions can facilitate accurate time zone management.
Debugging Time Issues
Debugging time-related issues requires attention to detail: ensure you account for leap seconds and daylight saving changes, as these can introduce subtle bugs in calculations. Verifying that your time points and durations are being manipulated correctly is crucial for reliable code.
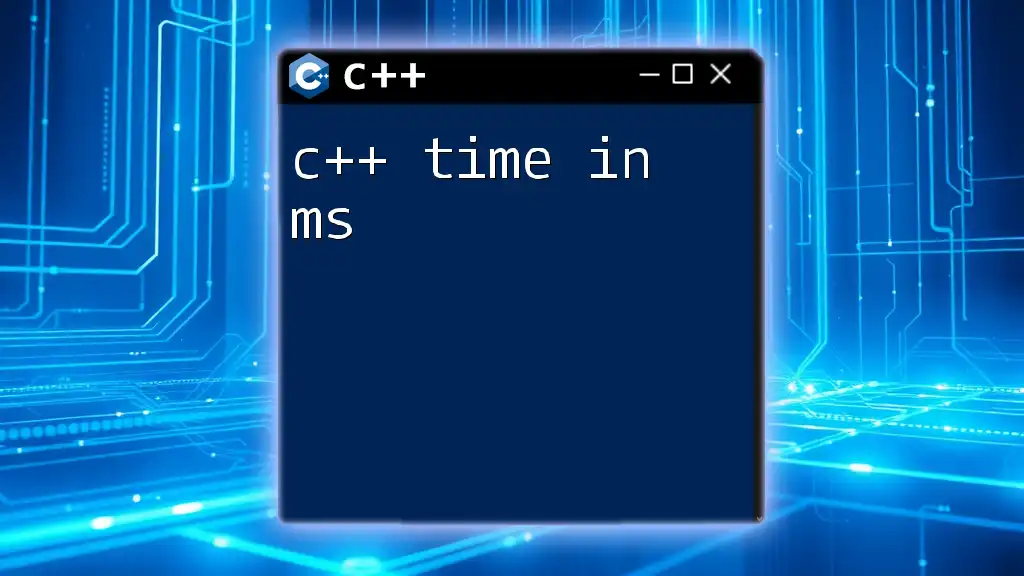
Conclusion
The C++ Time Library provides powerful and flexible utilities for managing time in your applications. Understanding the concepts and practical applications of C++ Time, as well as leveraging the `<chrono>` functionalities, can enhance the efficiency and reliability of your programming projects. As you delve deeper into C++ time handling, consider experimenting with these libraries to develop your own practical applications and tools.