`size_t` is an unsigned integer type defined in the C++ standard library, used primarily for representing the size of objects in bytes and as the return type for the `sizeof` operator.
Here's a code snippet demonstrating its use:
#include <iostream>
int main() {
int arr[10];
size_t size = sizeof(arr) / sizeof(arr[0]); // Calculate the number of elements in the array
std::cout << "The array has " << size << " elements." << std::endl;
return 0;
}
Understanding size_t
What is size_t?
`size_t` is a fundamental data type in C++ used primarily for representing the sizes of objects and for array indexing. It is defined in the `<cstddef>` header file. The primary reason for using `size_t` is that it provides a portable, efficient, and safe way to handle memory sizes across different architectures.
Unlike many integer types, `size_t` is an unsigned integer type. This means it can only represent non-negative values, which makes it inherently suitable for sizes and lengths, as these values cannot logically be negative.
The Nature of size_t
The value of `size_t` can vary depending on the system architecture:
- On a 32-bit system, `size_t` is typically a 4-byte value, allowing for a range of 0 to 4,294,967,295.
- On a 64-bit system, it grows to an 8-byte value, which allows for a substantially larger range of 0 to 18,446,744,073,709,551,615.
This flexibility ensures that `size_t` can accommodate the requirements of larger datasets on modern hardware, enabling developers to write portable code that can run across various platforms.
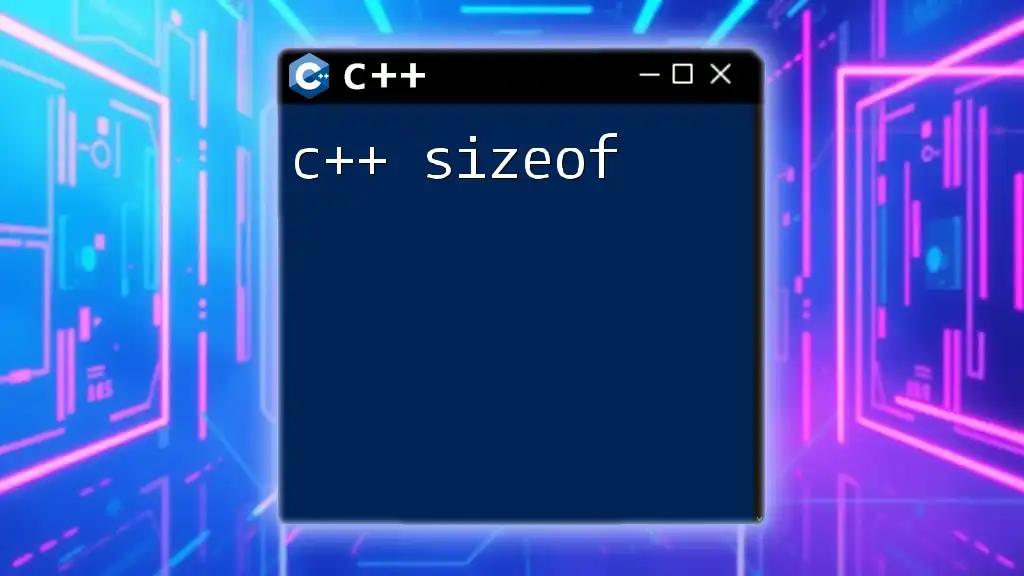
When to Use size_t
Common Use Cases for size_t
Array Indexing: Using `size_t` for array indices and sizes helps prevent potential negative indexing errors. For example, consider a situation where you are iterating over an array. By using `size_t`, you safeguard your code from inadvertently assigning negative values to index variables.
Loop Counters: In loops, especially when iterating through containers such as `std::vector`, it’s advisable to use `size_t`. This helps optimize performance and ensures that you're working with positive integer values, improving the robustness of your code.
Storage Sizes: When working with data structures like arrays or vectors, using `size_t` to define their sizes ensures compatibility with various standard library functions and methods.
When to Avoid size_t
While `size_t` has its advantages, there are scenarios where it might not be suitable. For instance, when dealing with signed integer operations, you may want to use `int` or `long` instead. Consequently, be cautious when converting `size_t` to signed types, as this may lead to unexpected behavior if a negative value is assigned.
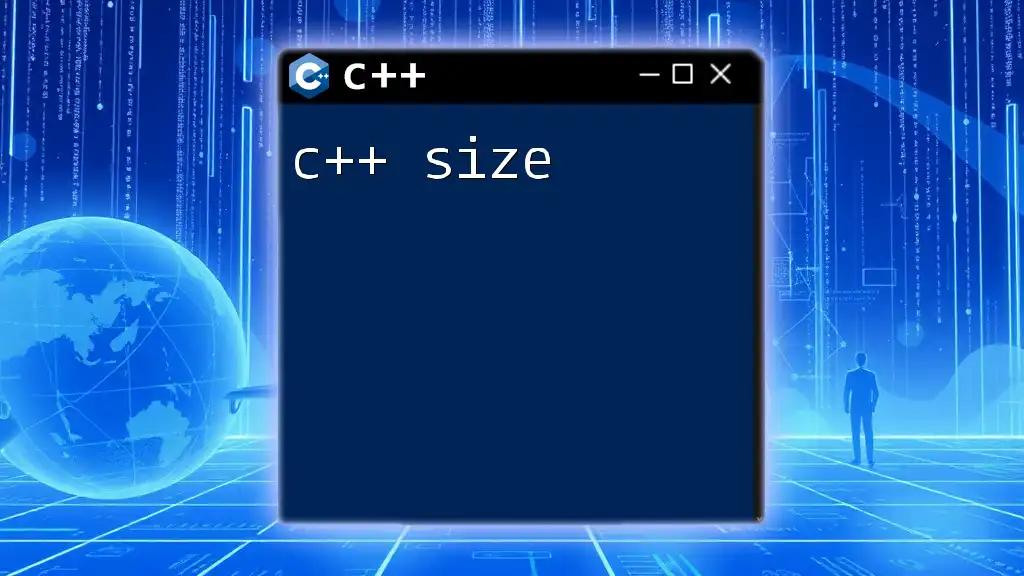
Characteristics of size_t
Memory Allocation
When allocating memory in C++, the size of the memory block is often specified using `size_t`. Functions like `malloc` and operators like `new` use `size_t` to specify the block size. This makes your memory allocations safer and ensures that your code behaves predictably.
#include <cstdlib>
int main() {
size_t num_elements = 10;
int* array = (int*)malloc(num_elements * sizeof(int));
// Always check if the allocation was successful
if (array == nullptr) {
// Handle allocation failure
}
free(array); // Don't forget to free allocated memory
return 0;
}
This snippet demonstrates how to allocate an array using `malloc`. The `size_t` variable `num_elements` clearly expresses the number of elements you want to allocate memory for, enhancing code readability.
Compatibility with STL (Standard Template Library)
Many of the STL containers leverage `size_t` for their size and index methods. For example, in a `std::vector`, the `size()` method returns a `size_t` type.
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
size_t size = numbers.size();
for (size_t i = 0; i < size; ++i) {
std::cout << numbers[i] << std::endl;
}
return 0;
}
In the code above, you can see how `size_t` serves efficiently both for maintaining the count of elements with `size()` and while iterating through the vector, ensuring that the index is always a positive integer.

Using size_t in Your Code
Declaring size_t Variables
Declaring a `size_t` variable is straightforward. You can define it to store the size of data types or iterate through collections in your code.
For instance:
size_t count = 5;
size_t index;
When naming your `size_t` variables, choose descriptive names that clearly indicate their purpose, such as `element_count` or `array_index`, to enhance code readability.
Example: Basic Usage of size_t
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
size_t size = numbers.size();
for (size_t i = 0; i < size; ++i) {
std::cout << numbers[i] << std::endl;
}
return 0;
}
This snippet demonstrates how `size_t` is used for both the container's size and the loop index, ensuring that the operations remain within safe bounds.
Interoperability with Other Data Types
Since `size_t` is an unsigned type, mixing it with signed types can lead to unpredictable results, especially in arithmetic operations.
If you need to perform calculations with `size_t`, be wary of implicit type conversions. For instance:
size_t a = 5;
int b = -3;
size_t result = a + b; // This could lead to unexpected behavior
In this scenario, `b` is converted to an unsigned type, leading to a large positive number being added instead of `2`. Always validate the origin of the variables you're mixing and perform explicit type conversions when necessary.

Performance and Efficiency Considerations
Why size_t is a Good Choice for Size Calculation
Using `size_t` can lead to performance optimizations by allowing the compiler to efficiently handle unsigned integers. This is particularly true in loop iterations, which can be a frequent operation in many algorithms.
Limitations of Using size_t
`size_t`, being an unsigned type, can cause overflow if not monitored, particularly when performing arithmetic operations. If you exceed the maximum value of `size_t`, it wraps around to 0, which can be problematic.
For example, observe how the following code leads to wraparound when `result` exceeds `size_t` limits:
#include <iostream>
#include <limits>
int main() {
size_t maxSize_t = std::numeric_limits<size_t>::max();
size_t result = maxSize_t + 1; // Wraps around to 0
std::cout << "Result after overflow: " << result << std::endl; // Outputs 0
return 0;
}

Comparing size_t with Other Data Types
size_t vs int
While both `size_t` and `int` can be used for counting, `size_t` is optimally used for sizes and counts, given its unsigned nature. If you mix these types in your code, you might face issues where negative values are assigned to variables intended for lengths or sizes.
A quick demonstration:
int arr[5];
for (int i = 0; i < 5; ++i) {
arr[i] = i;
}
// This is safe, as we are using int for indices.
// If you attempt to use a signed variable for indexing,
// you might inadvertently create bugs.
size_t vs long
`long` can store larger values than a typical `int`, but being signed presents inherent risks when dealing with sizes. If your code manipulates array sizes or dimensions, sticking to `size_t` ensures you're working with logical constraints.
As a comparison, consider this scenario:
long size = -1; // This poses a logical issue if used for array sizing
Whereas using `size_t` for sizing avoids such pitfalls entirely.

Conclusion
The `size_t` type is essential for safe and effective C++ coding, particularly when dealing with sizes, counts, and array indices. Its unsigned nature, flexibility based on platform architecture, and seamless integration with STL make it a go-to choice for developers. By adopting best practices around the use of `size_t`, developers can enhance the reliability and efficiency of their C++ applications.
Ensuring that you incorporate `size_t` appropriately in your code will not just make it safer but also more efficient. As you continue to expand your skills in C++, taking full advantage of `size_t` will contribute significantly to producing robust code.

Additional Resources
For further learning about `size_t`, check official C++ documentation or explore additional articles and tutorials that delve deeper into data types and memory management. Also, consider subscribing to platforms that offer C++ tips and tricks to refine your understanding and practices in this powerful language.