In C++, the `sort` function from the `<algorithm>` library is used to arrange the elements of a container in ascending order by default.
#include <iostream>
#include <algorithm>
#include <vector>
int main() {
std::vector<int> numbers = {4, 2, 5, 1, 3};
std::sort(numbers.begin(), numbers.end());
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
Understanding Sorting in C++
What is Sorting?
Sorting in a programming context refers to arranging data in a specific order, which can be either ascending or descending. The ability to sort data is crucial because it enhances usability, improves search efficiency, and enables data analysis. A well-structured dataset can significantly improve performance in various applications, from simple list displays to complex algorithms.
Types of Sorting Algorithms
There are many sorting algorithms, each with unique characteristics. Common algorithms include:
- Bubble Sort: A simple algorithm that repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order. Its performance is not optimal for large datasets.
- Selection Sort: This algorithm divides the input list into a sorted and an unsorted region, continuously selecting the smallest (or largest) element from the unsorted region to move it to the sorted region.
- Quick Sort: A more efficient algorithm that uses a divide-and-conquer strategy. It selects a 'pivot' element and partitions the other elements into two sub-arrays according to whether they are less than or greater than the pivot.
- Merge Sort: Another divide-and-conquer algorithm that splits the array into halves, sorts them, and then merges the sorted halves.
In C++, developers primarily utilize the built-in `sort` function from the Standard Template Library (STL), which often provides optimal performance.
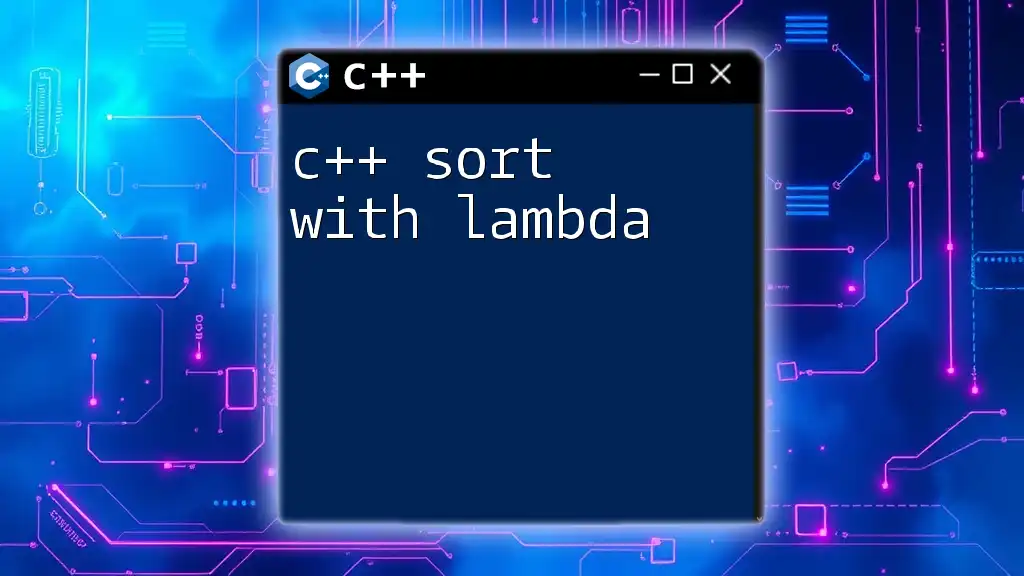
The C++ `sort` Function
Introduction to the C++ STL
The Standard Template Library (STL) is a powerful toolkit in C++ that includes data structures, algorithms, and iterator functionality. It enables developers to write efficient and general-purpose code. One of the most powerful features of the STL is its set of sorting algorithms, which allow for efficient and easy data ordering.
Syntax of `sort`
The basic syntax for using the `sort` function is as follows:
std::sort(begin, end);
Here, `begin` and `end` are iterators that define the range of the elements to be sorted. Importantly, to utilize the `sort` function, developers must include the appropriate header file:
#include <algorithm>
Default Behavior of `sort`
By default, the `sort` function arranges elements in ascending order. It works with various data types, including arrays, vectors, and lists, optimizing the organization of data. The `sort` function is efficient and adapts well to different data structures due to its use of templates.
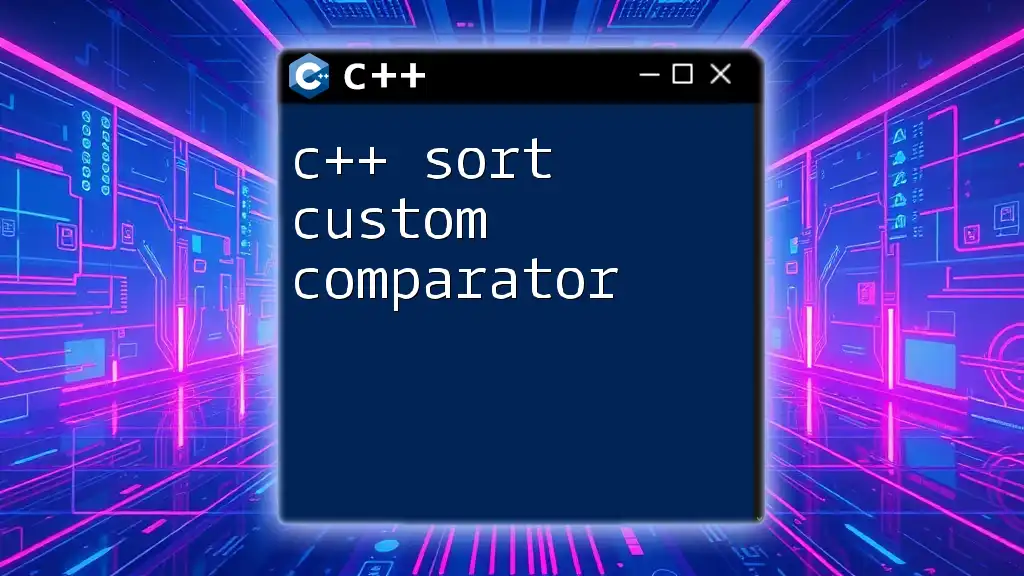
Custom Sorting with C++
Sorting in Descending Order
While the default behavior of `sort` is to order elements in ascending order, developers might want to sort elements in descending order. This can be achieved by providing a custom comparison function:
std::sort(begin, end, std::greater<T>());
Here is an example of sorting a vector of integers in descending order:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {5, 2, 8, 1, 3};
std::sort(vec.begin(), vec.end(), std::greater<int>());
for (int n : vec) {
std::cout << n << " ";
}
return 0;
}
In this example, the output will be `8 5 3 2 1`, demonstrating the capability of `std::sort` to arrange the elements in a descending order.
Custom Sorting Criteria
The `sort` function can also accept a custom comparator, allowing sorting based on specific criteria. This feature is beneficial when dealing with user-defined data types. Consider the following example using a custom object:
struct Point {
int x, y;
bool operator<(const Point& other) const {
return (x < other.x) || (x == other.x && y < other.y);
}
};
// Sorting vector of Points
std::vector<Point> points = {{1, 2}, {3, 1}, {2, 2}};
std::sort(points.begin(), points.end());
In this code snippet, the `Point` struct defines a custom comparison; it sorts points based on their `x` and `y` coordinates. Developers can customize the comparison logic as desired to suit varying scenarios and requirements.
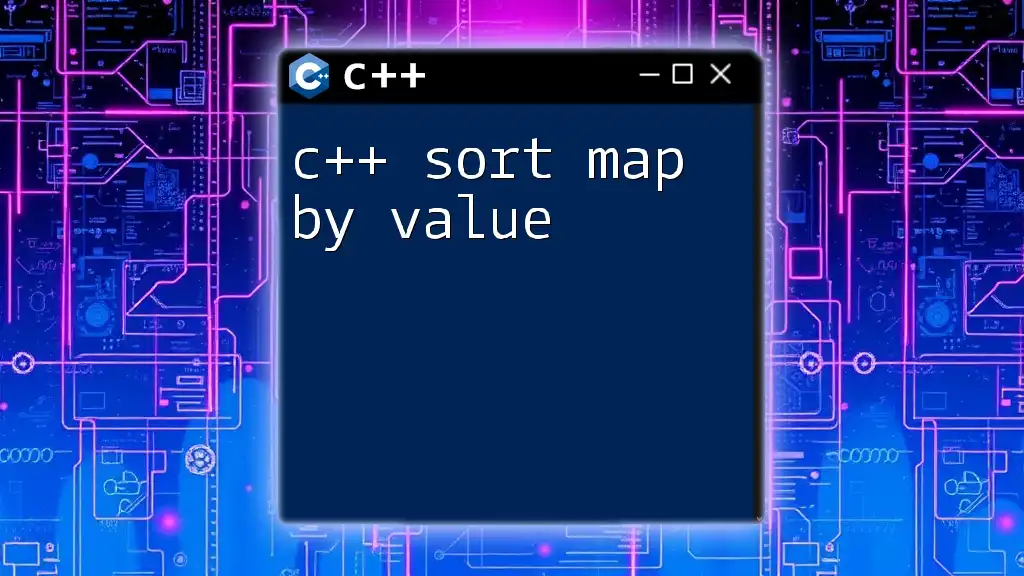
Performance Considerations
Time Complexity of `sort`
The time complexity of `std::sort()` is generally O(n log n), making it highly efficient for sorting large datasets. The underlying algorithm that is commonly implemented in `std::sort` is IntroSort, which combines quicksort, heapsort, and insertion sort. This approach offers a good balance between performance and efficiency, dynamically adapting to the characteristics of the data being sorted.
Space Complexity
In terms of space complexity, `std::sort()` is considered efficient as it typically sorts in-place, requiring O(log n) additional space for the call stack when using a recursive approach. This constraint means minimal extra memory usage, making it suitable for large datasets.
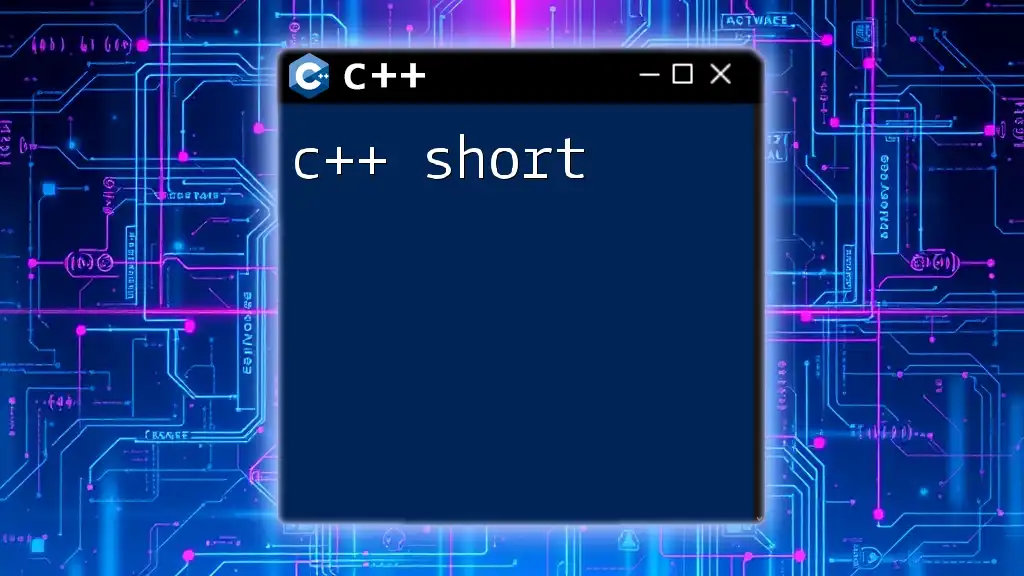
Real-World Applications of Sorting
Sorting in Data Analysis
Sorting is a foundational operation in data preprocessing for analytical tasks. In a data analysis context, datasets often need to be sorted based on various attributes. For example, sorting user records by name or age prior to generating reports simplifies access and enhances report readability. Sorting enables analysts and developers to automate processes that rely on orderly data presentation.
Sorting for Search Operations
Sorting enhances the efficiency of search algorithms. For example, when dealing with a sorted array, developers can implement binary search, a significantly faster algorithm compared to simple linear search. The performance improvement is substantial; binary search operates in O(log n) time when applied to sorted data, showcasing the critical role of sorting in search efficiency.
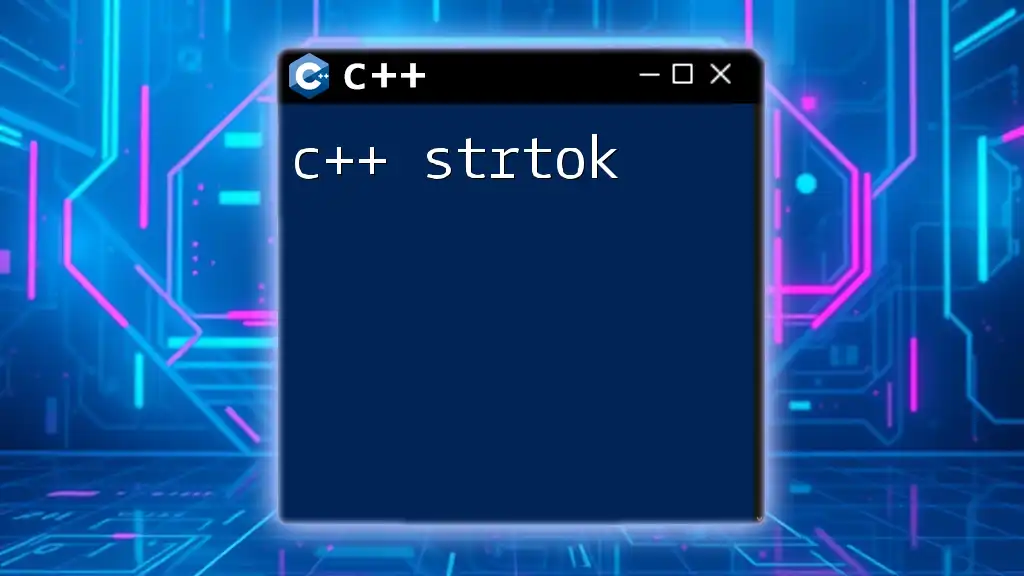
Conclusion
The C++ `sort` function is a powerful tool for organizing data effectively. Understanding its capabilities, from basic usage to custom sorting criteria, equips developers with the skills necessary to manage data efficiently in their applications. Whether for optimizing search operations, facilitating data analysis, or handling complex datasets, mastering `std::sort` will enhance both coding ability and program performance. Experiment with various data types and sorting conditions to further your proficiency in utilizing C++ for efficient data management.
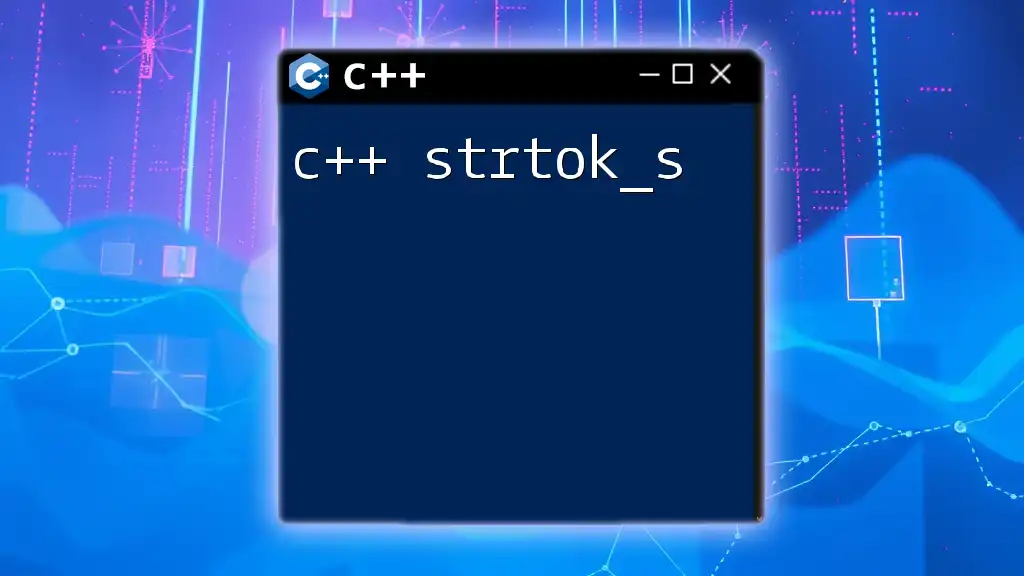
Additional Resources
For further learning, refer to the official C++ documentation concerning the Standard Template Library (STL) and its various algorithms. Explore online coding platforms for hands-on practice with `std::sort()` and delve into advanced topics of sorting algorithms to enhance your understanding and application skills.