Short-circuit evaluation in C++ occurs when the evaluation of a logical expression is halted as soon as the result is determined, typically seen in the use of the logical AND (`&&`) and OR (`||`) operators.
Here’s a code snippet demonstrating this concept:
#include <iostream>
bool check(bool a, bool b) {
return a && (b = false); // b will not be evaluated if a is false, preserving its original value
}
int main() {
bool x = true;
bool y = true;
bool result = check(x, y);
std::cout << "Result: " << result << ", y: " << y << std::endl; // y remains true because the second condition is not evaluated
return 0;
}
Understanding Short Circuit Evaluation
What is Short Circuit Evaluation?
C++ short circuit evaluation refers to the behavior of logical operators, specifically the logical AND (`&&`) and logical OR (`||`), when evaluating expressions. In short circuit evaluation, an expression's evaluation stops as soon as the overall outcome is determined. This behavior can lead to more efficient code as unnecessary evaluations are avoided.
How Short Circuit Evaluation Works
When evaluating an expression with logical operators:
- For `&&`: If the first operand is `false`, the second operand isn't evaluated because the entire expression can only be `false`.
- For `||`: If the first operand is `true`, the second operand is not evaluated because the entire expression can only be `true`.
Example:
bool a = true;
bool b = false;
if (a && (b = true)) {
// This block will not execute; b remains false
}
In this example, even though `b` is manipulated inside the conditional statement, it remains `false` because the first operand evaluates to `true`.
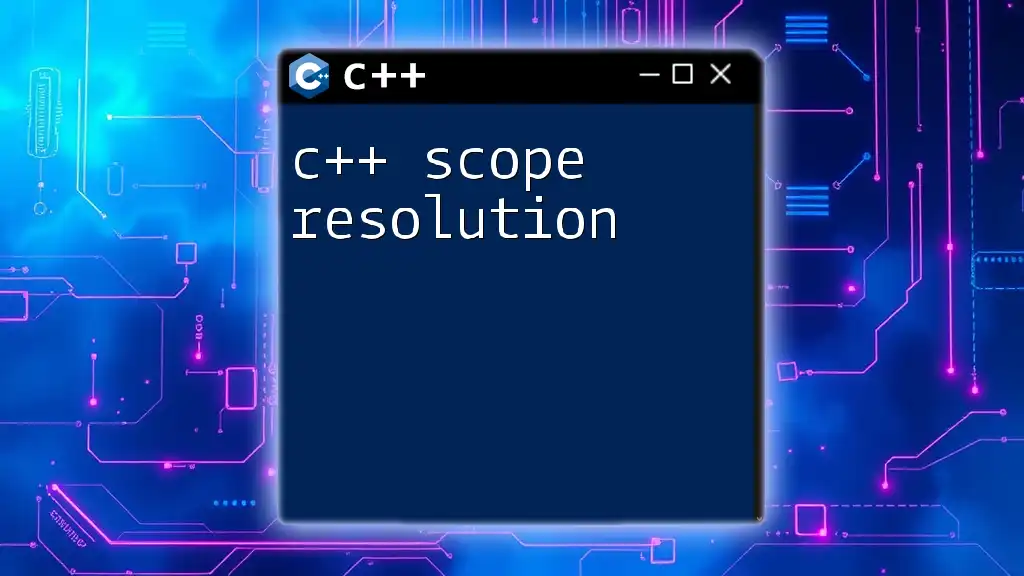
The Mechanics of Logical Operators
The Logical AND (`&&`) Operator
The logical AND operator evaluates operands in a way that if any operand is `false`, the result becomes `false`. Hence, with short circuit evaluation, if the first operand is `false`, the entire expression won't be evaluated further.
Consider this code snippet:
bool a = true;
bool b = false;
if (a && (b = true)) {
// This block will not execute
}
In this scenario, since `b` is assigned `true` within the conditional, it never happens because of short circuit evaluation. Here, `b` remains `false`.
The Logical OR (`||`) Operator
The logical OR operator behaves similarly but in the opposite manner. If any operand evaluates to `true`, the result is `true`, and subsequent expressions are ignored.
Code Snippet:
bool x = false;
bool y = true;
if (x || (y = false)) {
// This block will execute
}
In this code, since `x` is `false`, the expression evaluates `y = false`, which is also ignored within the condition since `y` is already `true` at the time of evaluation.
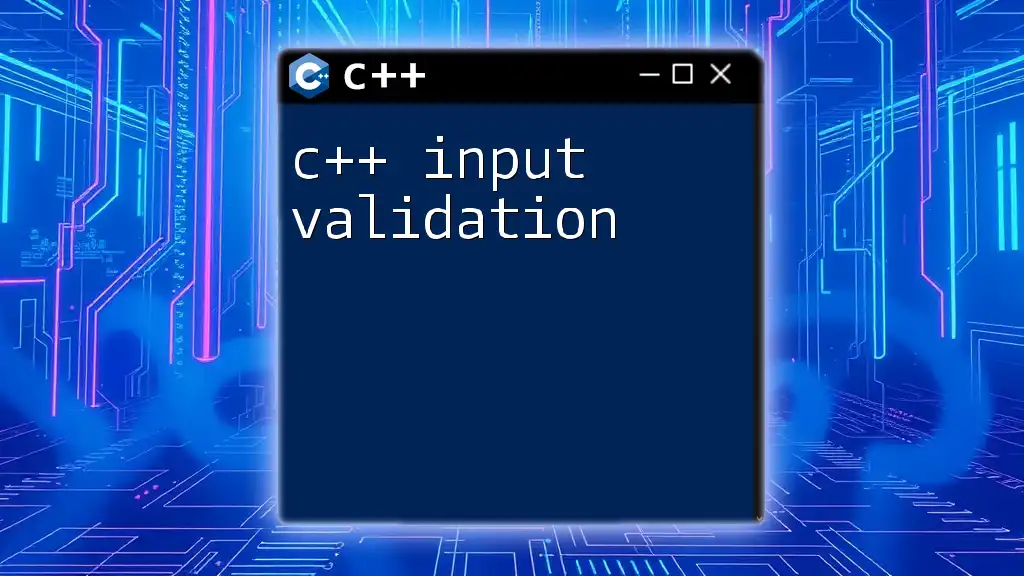
Advantages of Short Circuit Evaluation
Performance Benefits
By avoiding unnecessary evaluations, C++ short circuit evaluation significantly improves performance. In scenarios where the second operand may involve heavy computation or expensive function calls, this behavior becomes especially useful.
Prevention of Side Effects
In cases where function calls are made within logical expressions, short circuit evaluation can prevent unintended side effects. For example:
bool shouldExecute() {
return false; // An expensive function
}
if (false && shouldExecute()) {
// shouldExecute() is never called
}
In this code, `shouldExecute` is never executed because the first condition is `false`, showcasing how short circuit evaluation can optimize performance by avoiding unnecessary function calls.
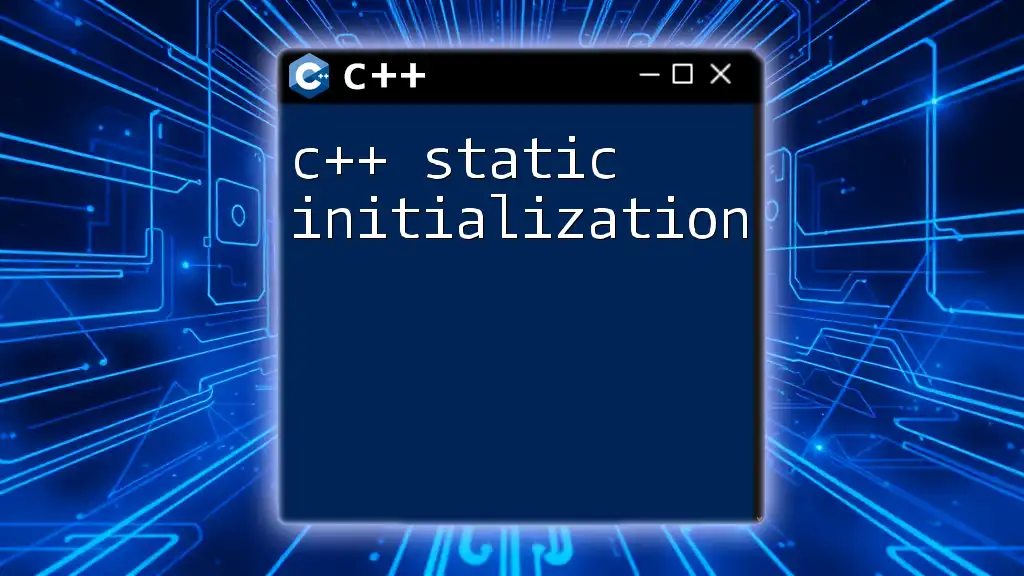
Practical Applications
Use Cases in Real Applications
Understanding C++ short circuit evaluation is critical in various practical applications, such as:
- Conditional validations: Where multiple conditions need to be checked, using short circuit evaluation can optimize checks that only need to consider the first condition's result to determine the path of execution.
- Resource management: If resources are allocated conditionally based on previous checks, short circuit evaluation prevents unnecessary resource allocation calls.
Common Mistakes to Avoid
Developers often misunderstand how short circuit evaluation works, leading to potential bugs in their code. For example:
bool a = false;
bool b = true;
if (a || (b = false)) {
// This block will execute
}
// This can lead to confusion if one thought b would always end up false.
Here, developers might mistakenly assume both conditions will always execute, but due to the behavior of the || operator, the assignment to `b` takes place only if `a` is `false`, which might diverge from developer expectations.
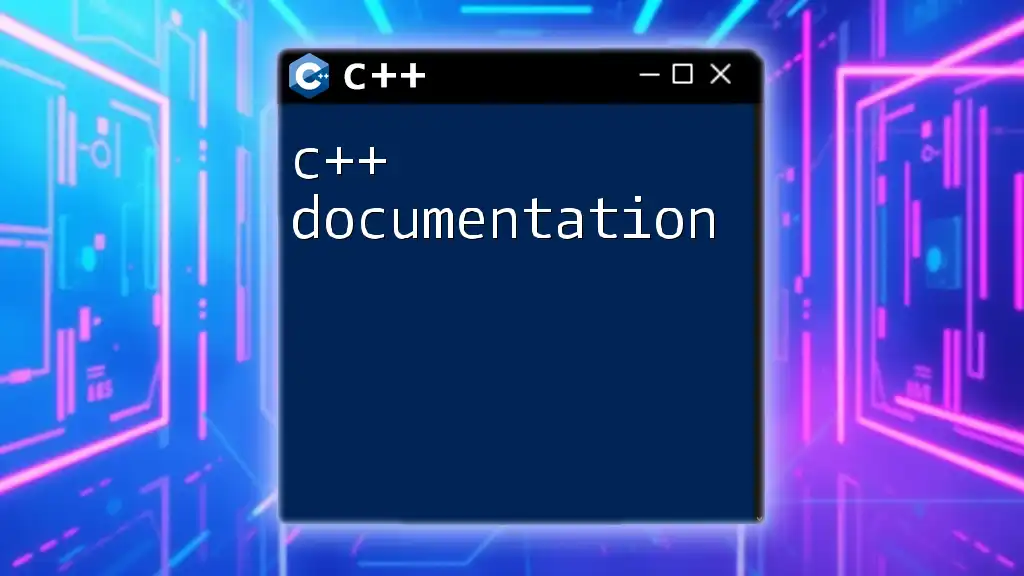
Best Practices for Using Short Circuit Evaluation
Writing Clear and Understandable Code
Clarity in writing code is essential. By using parentheses to clearly define groups of expressions, you ensure that your intentions match what the code executes. For example:
if ((condition1 || condition2) && condition3) {
// More readable logic
}
This clarity helps other developers (and yourself in the future) to better understand the logic behind conditions, leading to fewer mistakes.
Testing and Debugging Tips
- Test cases defining the behavior of each logical operation can help reveal pitfalls in your usage of short circuit evaluation.
- Debugging becomes more straightforward when you precisely understand how each logical operator behaves. Using debugging tools to step through logical blocks that utilize short circuit expressions can clarify expected results and actual outcomes.
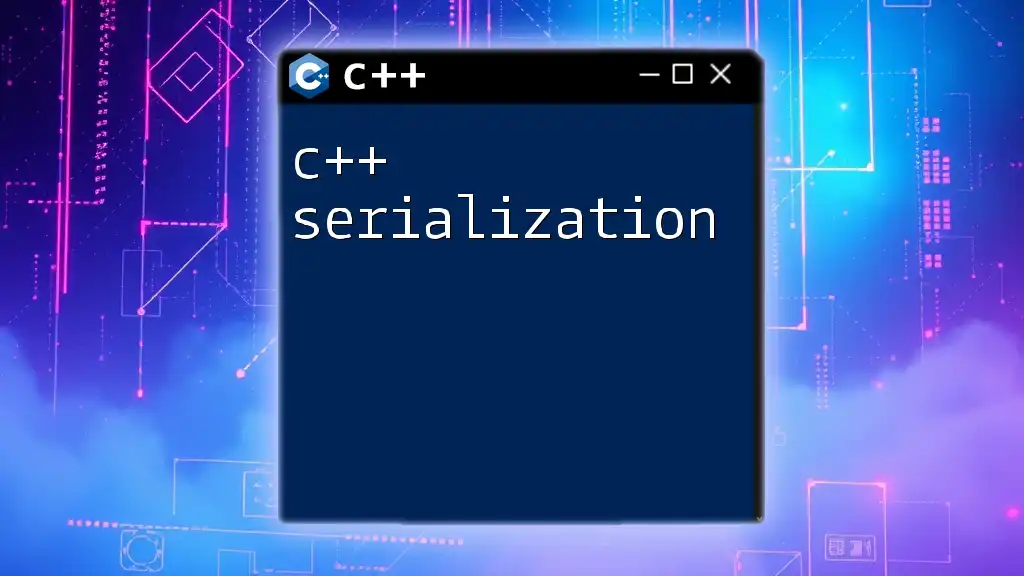
Conclusion
In summary, understanding C++ short circuit evaluation is instrumental in writing efficient, clear, and effective code. The performance benefits and the prevention of side effects make it an essential concept for any developer working with logical expressions. Experimenting with your own code will deepen your understanding while encouraging you to look out for potential pitfalls that can arise from misassumptions about logical operators.