To sort a C++ map by its values, you can use a vector of pairs and the `std::sort` function, like this:
#include <iostream>
#include <map>
#include <vector>
#include <algorithm>
int main() {
std::map<std::string, int> myMap = {{"apple", 3}, {"banana", 1}, {"cherry", 2}};
std::vector<std::pair<std::string, int>> sortedVec(myMap.begin(), myMap.end());
std::sort(sortedVec.begin(), sortedVec.end(), [](const auto& a, const auto& b) { return a.second < b.second; });
for (const auto& pair : sortedVec) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
Understanding C++ Maps
What is a C++ Map?
A C++ map is a part of the C++ Standard Template Library (STL) that stores data in key-value pairs. This associative container allows for efficient data retrieval organized based on keys. Maps are particularly useful when you need to maintain unique keys while accessing their associated values quickly.
Key Characteristics of Maps
- Associative Nature: In a map, every key is linked to a specific value. This means you can look up a value by its key efficiently.
- Unique Keys: Each key in a C++ map must be unique. If you try to insert a duplicate key, the existing value will be overwritten.
- Order of Elements: By default, elements are sorted based on the keys, which provides an ordered view of the data.
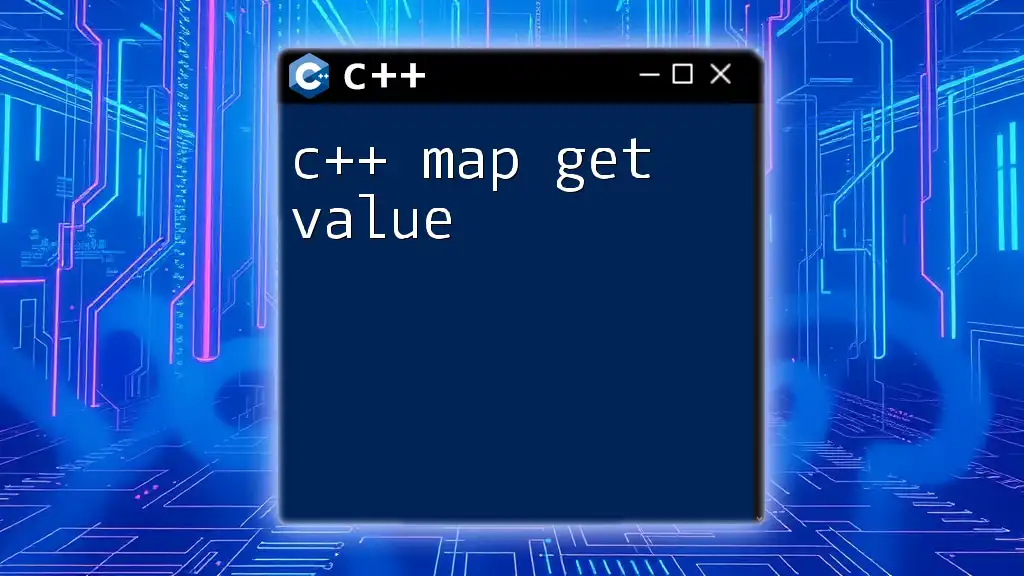
Sorting a Map in C++
Why Sort by Value?
Sorting a C++ map by value becomes essential in scenarios where the relationship between keys and their values matters. For instance:
- You might want to rank items based on sales figures represented by values.
- In data analysis, you may want to organize frequencies of occurrences for better insights.
- For leaderboards, you ideally display player names (key) sorted by their scores (value).
To effectively implement a sorting algorithm in C++, understanding the default behaviors of maps is crucial.
The Default Behavior
It's important to remember that a standard C++ map sorts its elements by keys. For example, consider the following code snippet demonstrating this behavior:
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap = {{2, "two"}, {1, "one"}, {3, "three"}};
for (const auto &pair : myMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
In this example, the output will display the items sorted by keys rather than values.
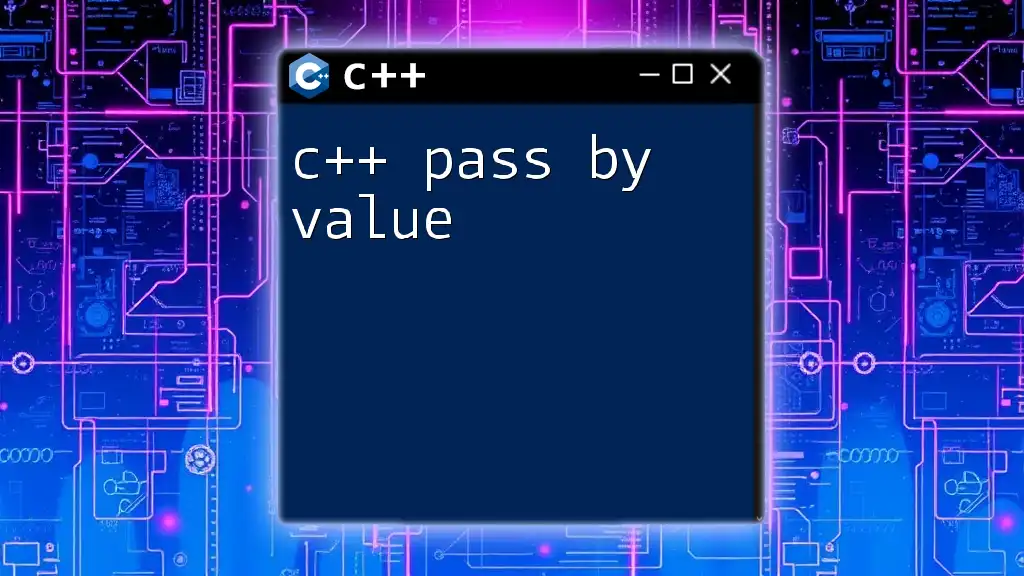
Method to Sort Map by Value in C++
Sorting with Vectors
To sort a map by value, one effective method is to extract the key-value pairs into a vector.
Extracting Key-Value Pairs
First, you can create a vector that copies these pairs from the map. This allows you to perform operations that C++ maps do not support, such as sorting based on values.
Here's how to extract the pairs:
#include <iostream>
#include <map>
#include <vector>
int main() {
std::map<int, std::string> myMap = {{2, "two"}, {1, "one"}, {3, "three"}};
std::vector<std::pair<int, std::string>> vec(myMap.begin(), myMap.end());
// Continue with sorting...
return 0;
}
Custom Sorting Function
Using the extracted vector, you can now implement a custom sorting function. This allows you to define how the elements should be compared and organized.
Here’s how to sort the vector by values:
#include <algorithm>
// ... (previous code)
std::sort(vec.begin(), vec.end(), [](const auto &a, const auto &b) {
return a.second < b.second; // Sort by value
});
// Display sorted results
for (const auto &pair : vec) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
Final Sorted Output
After sorting, the output will now reflect the values in ascending order, illustrating a practical approach to sorting a map by value.
Using std::multimap for Sorting
Advantages of std::multimap
The `std::multimap` is another useful STL container that allows for multiple entries with the same key. This property can be particularly beneficial when dealing with duplicate values.
Example Implementation
You can use a `std::multimap` to store values that are identical while maintaining ordering:
#include <iostream>
#include <map>
int main() {
std::multimap<std::string, int> sortedMap;
std::map<int, std::string> myMap = {{2, "two"}, {3, "three"}, {1, "one"}, {2, "deux"}};
for (const auto& pair : myMap) {
sortedMap.insert(std::make_pair(pair.second, pair.first)); // swap key and value
}
// Display the sorted map by value
for (const auto& pair : sortedMap) {
std::cout << pair.second << ": " << pair.first << std::endl;
}
return 0;
}
This snippet demonstrates leveraging `std::multimap` to produce a sorted view based on values.
Using STL Algorithms with Custom Sort
Utilizing std::unordered_map and std::vector
For additional flexibility, consider using the `std::unordered_map`, which does not maintain order but allows quicker look-ups.
The process remains similar:
#include <iostream>
#include <unordered_map>
#include <vector>
#include <algorithm>
int main() {
std::unordered_map<int, std::string> unorderedMap = {{2, "two"}, {3, "three"}, {1, "one"}};
std::vector<std::pair<int, std::string>> vec(unorderedMap.begin(), unorderedMap.end());
std::sort(vec.begin(), vec.end(), [](const auto &a, const auto &b) {
return a.second < b.second; // Sort by value
});
// Display sorted results
for (const auto &pair : vec) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
This approach allows greater flexibility and speed when handling large datasets.
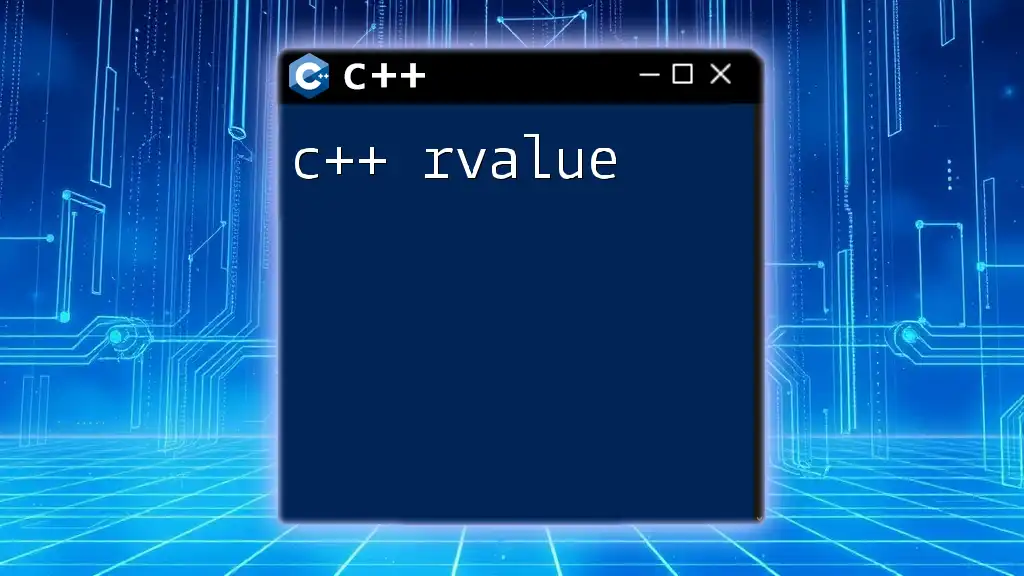
Handling Edge Cases
Sorting Maps with Duplicate Values
When working with maps that may contain duplicate values, consider how you want to handle these scenarios. Preserving original key-value pairs in your results can be important in some applications; hence, using a `std::multimap` can be a great choice to handle such duplicates more effectively.
Considering Performance
It's essential to consider the time complexity of sorting and the impact it might have on performance, especially with larger datasets. The sorting operation typically has a complexity of O(n log n), meaning it may be necessary to optimize your map's size or explore efficient data structures if performance is a key concern.
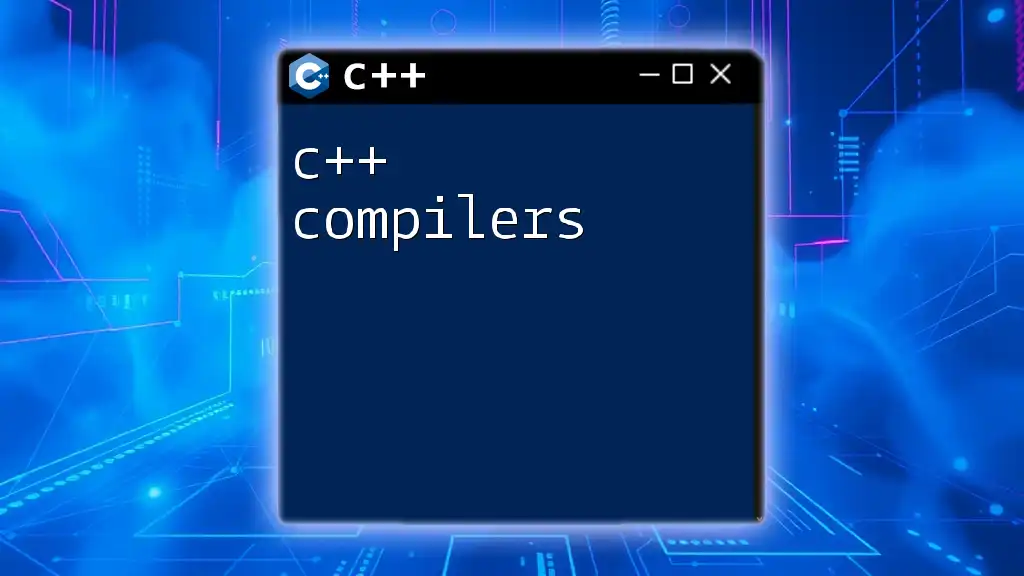
Practical Application
Real-World Use Cases
Sorting a map by value is useful across various domains, including:
- Data Analysis: Evaluating and ranking data inputs linked to performance metrics.
- User Scores and Leaderboards: Displaying participant scores in a competitive scenario allows users to see who's on top quickly.
- Frequency Counting: In fields like natural language processing, sorting words by their occurrence makes sense to analyze text data.
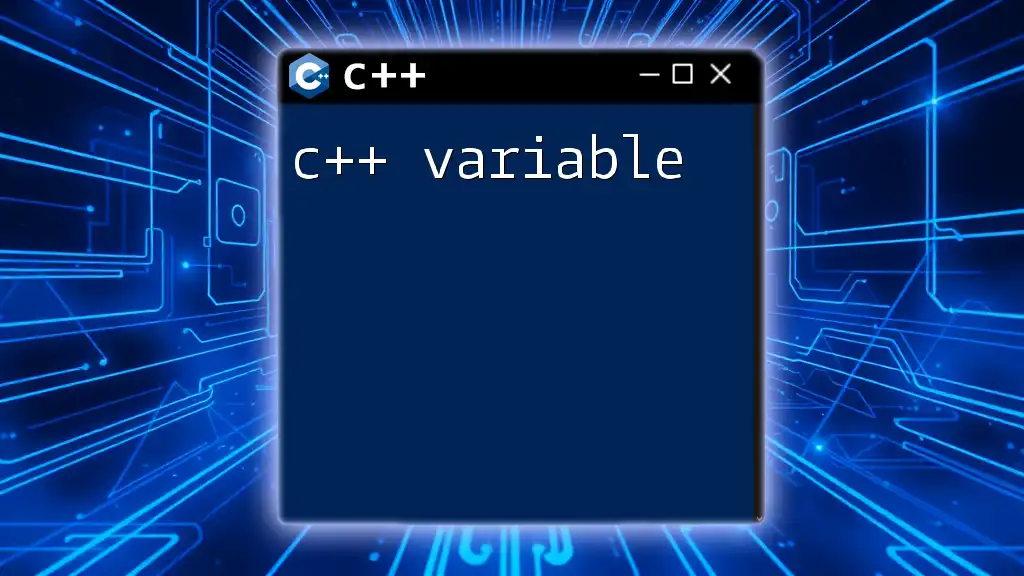
Conclusion
In conclusion, understanding how to sort a C++ map by value is a valuable skill for programmers. Whether it involves extracting to vectors, using multimaps or unordered maps, C++ provides multiple solutions to structure data based on requirements. The methods discussed allow for quick implementation in real-world scenarios while ensuring optimal performance and usability. Engaging in practice and experimenting with these techniques will lead to greater mastery of C++.