In C++, "pass by value" means that a copy of the variable is passed to a function, so changes made to the parameter within the function do not affect the original variable.
#include <iostream>
void modifyValue(int num) {
num = num + 10; // This change does not affect the original variable
}
int main() {
int original = 5;
modifyValue(original);
std::cout << "Original value: " << original << std::endl; // Output: Original value: 5
return 0;
}
Understanding Pass by Value
What is Pass by Value?
Pass by value in C++ is a parameter passing mechanism where a copy of the argument's value is passed to the function. In simpler terms, when a parameter is passed by value, the function works with a duplicate of the variable rather than the variable itself. This ensures that any modification within the function does not affect the original variable.
When we compare this to other parameter passing mechanisms, such as pass by reference or pass by pointer, we can see that they have different behaviors. Pass by reference allows a function to access the original variable directly, while pass by pointer involves passing the memory address, enabling modification at that location. Understanding the nuances of pass by value, especially in contrast to these alternatives, is crucial for proficient C++ programming.
How Passing by Value Works
When a function takes parameters by value, it creates local copies of the values passed in. Each time a function is called, this copying occurs.
For example, consider the following scenario. Imagine taking a snapshot of a photo. If you pass the snapshot to a friend, they can play around with it (e.g., edit, resize, etc.), but the original photo remains untouched. This analogy reflects the way pass by value operates in C++. The original variable remains intact, and functions can manipulate their own copies without repercussions.
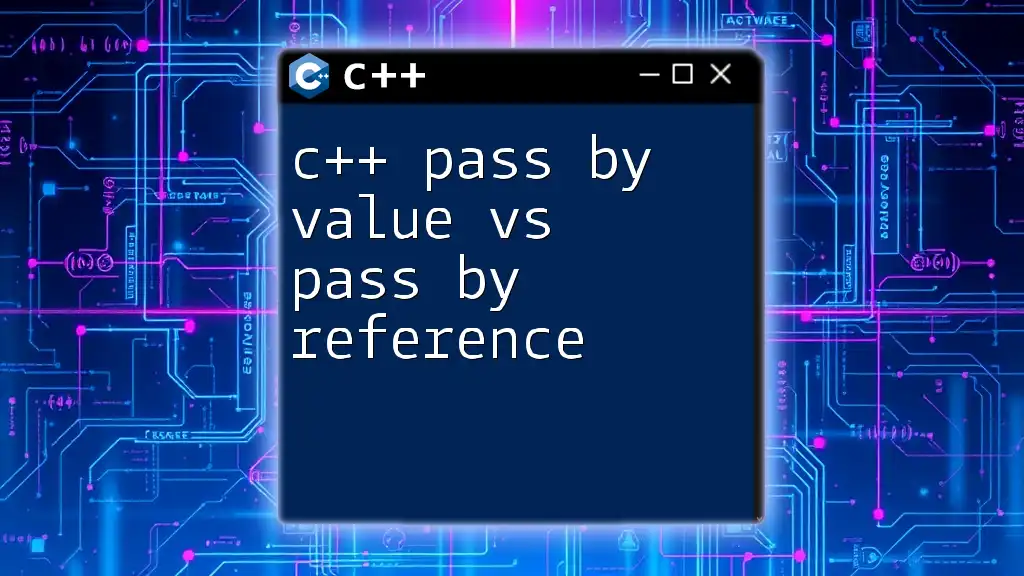
Advantages of Passing by Value
Simplicity and Safety
One of the primary advantages of using pass by value is its simplicity. For beginner programmers, understanding how values are passed—without the added complexity of memory address management—makes it easier to grasp function behavior.
Moreover, pass by value helps prevent unintended side effects. Since function parameters are local copies, any modifications do not influence the original data, leading to safer code. For instance, consider this code snippet:
#include <iostream>
using namespace std;
void modifyValue(int value) {
value += 5; // Local copy modified
cout << "Inside function: " << value << endl;
}
int main() {
int num = 10;
modifyValue(num);
cout << "Outside function: " << num << endl; // Outputs 10
return 0;
}
In this example, the output from `main()` remains unchanged despite the modification within `modifyValue()`—demonstrating the safety of the pass by value approach.
Function Isolation
Passing by value also enhances function isolation, meaning that each function can operate on its own independent copy of data. This isolation not only leads to fewer dependencies between functions but also aids in debugging since one function's state cannot inadvertently influence another's.
Consider the following example of how pass by value allows manipulation without affecting the original variable:
#include <iostream>
using namespace std;
void updateScore(int score) {
score += 10; // This only changes the copy.
cout << "Updated Score in function: " << score << endl;
}
int main() {
int playerScore = 100;
updateScore(playerScore);
cout << "Player Score after function call: " << playerScore << endl; // Outputs 100
return 0;
}
In this code, `updateScore()` modifies its local copy of `score`, leaving `playerScore` unaltered.
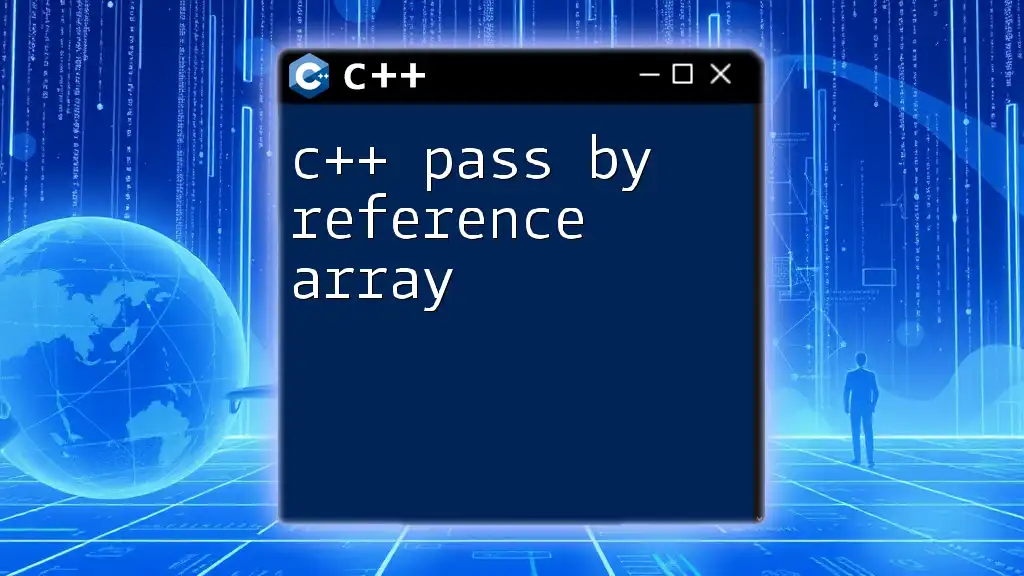
Disadvantages of Passing by Value
Performance Considerations
While pass by value is straightforward, it does come with potential performance drawbacks. When dealing with large data structures or objects, the overhead associated with copying values can lead to inefficiencies.
For example, passing large structs or classes could slow down your program, as each call involves duplicating extensive data. In such cases, alternatives like pass by reference or pass by pointer may be more efficient.
Limited Capability
An inherent limitation of passing by value is the inability to modify the original variable. If you need to change the value of an argument within a function and have that change persist outside of it, passing by value will not serve your needs.
For instance, consider this example contrasting pass by value with pass by reference:
#include <iostream>
using namespace std;
void increase(int& refValue) { // Pass by reference
refValue += 10;
}
int main() {
int myNumber = 50;
increase(myNumber);
cout << "After increasing: " << myNumber << endl; // Outputs 60
return 0;
}
In contrast, if using pass by value:
#include <iostream>
using namespace std;
void increase(int value) { // Pass by value
value += 10;
}
int main() {
int myNumber = 50;
increase(myNumber);
cout << "After increasing: " << myNumber << endl; // Outputs 50
return 0;
}
Here, you can see that in the pass by value example, the original number remains unchanged.
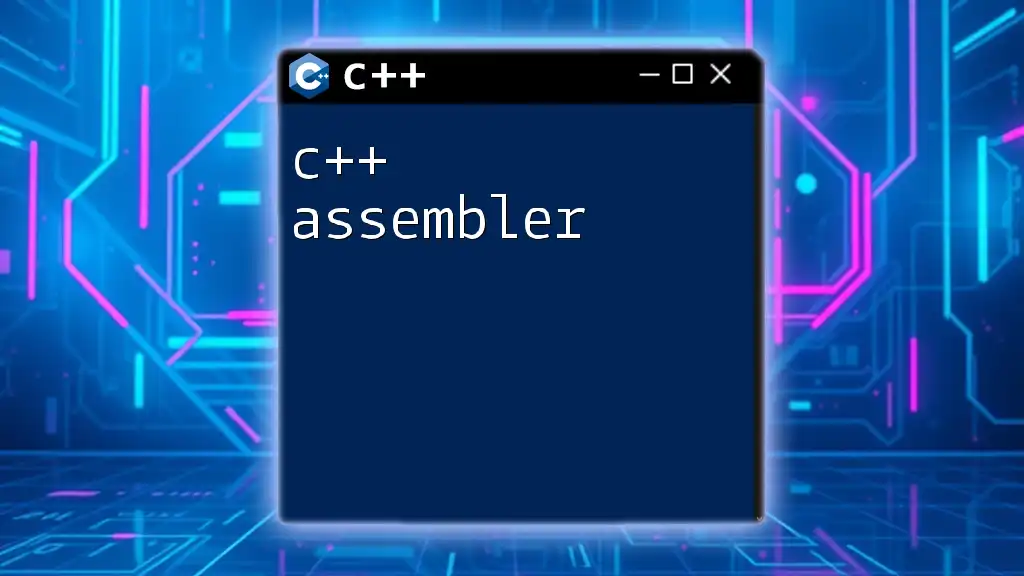
When to Use Pass by Value
Appropriate Scenarios
Using pass by value is most beneficial with primitive data types such as `int`, `char`, and `float`. These types are small and manage memory efficiently, making the copying process negligible.
Best Practice: Use pass by value in cases where you want to maintain the immutability of the original variable, such as in mathematical functions where calculations do not require modification of inputs.
Best Practices
When deciding between pass by value and other methods, consider:
- Pass by value for small, simple data types.
- Choose pass by reference or pointers for larger objects to enhance performance.
- Evaluate if the function needs to modify the original variable; if so, prefer passing by reference.
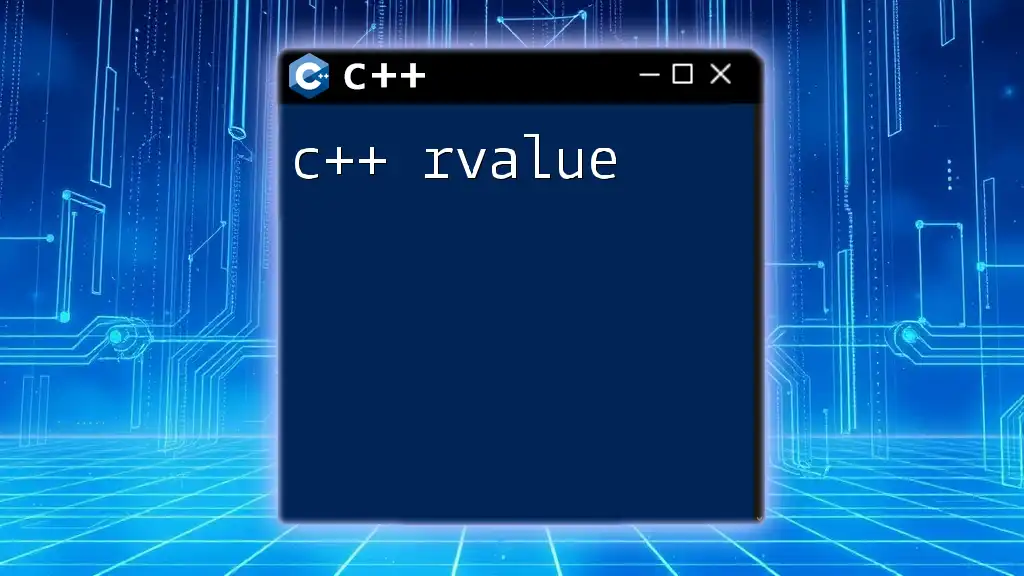
Code Examples
Basic Example of Pass by Value
Here’s a straightforward example demonstrating how pass by value works with integers:
#include <iostream>
using namespace std;
void increment(int value) {
value++; // This modifies the local copy only
cout << "Inside function: " << value << endl;
}
int main() {
int num = 10;
increment(num);
cout << "Outside function: " << num << endl; // Will output 10
return 0;
}
This snippet illustrates that the variable `num` in the `main()` function remains unchanged despite being passed to `increment()`.
Detailed Example with Structs
Now, let’s explore a more complex structure with a custom data type and see the implications of passing it by value:
#include <iostream>
using namespace std;
struct Point {
int x;
int y;
};
void movePoint(Point p) {
p.x += 1; // Modifying a copy
p.y += 1;
cout << "Moved Point: (" << p.x << ", " << p.y << ")" << endl;
}
int main() {
Point myPoint = {2, 3};
movePoint(myPoint);
cout << "Original Point: (" << myPoint.x << ", " << myPoint.y << ")" << endl; // Will output original values
return 0;
}
In this case, even though `movePoint()` alters `p`, the original `myPoint` remains unchanged when accessed outside the function.
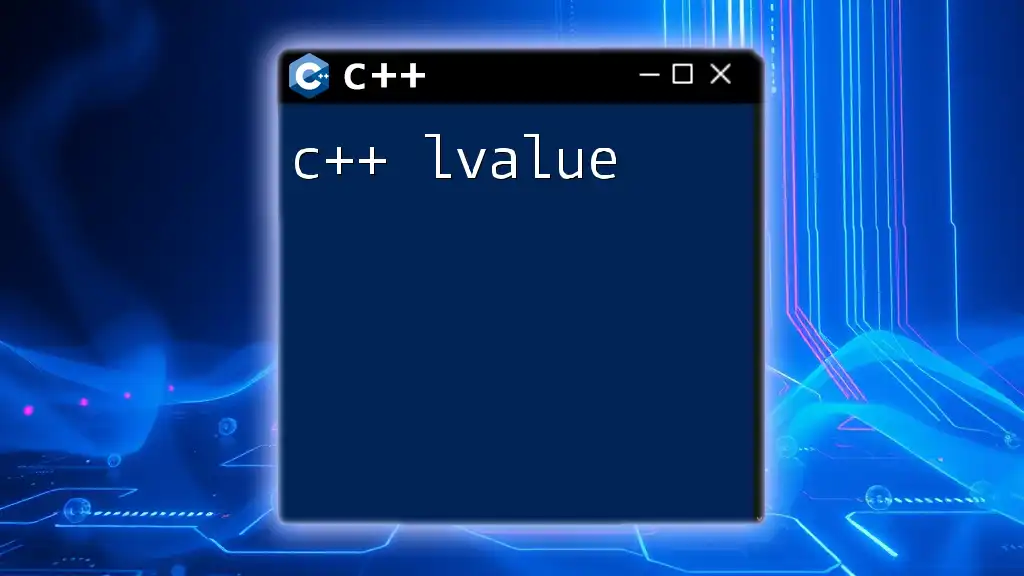
Common Mistakes in Pass by Value
Forgetting the Impact of Large Objects
A common mistake among developers, particularly novices, is using pass by value for large structures or classes. This misstep can lead to performance bottlenecks. Instead, opting for pass by reference or pointers should be considered when handling complex objects.
Misunderstanding Behavior
Another frequent error is confusing pass by value with pass by reference. Understanding the differences between these two methods is critical to mastering C++ programming. Always verify how parameters are passed in function definitions to avoid unintended behavior.
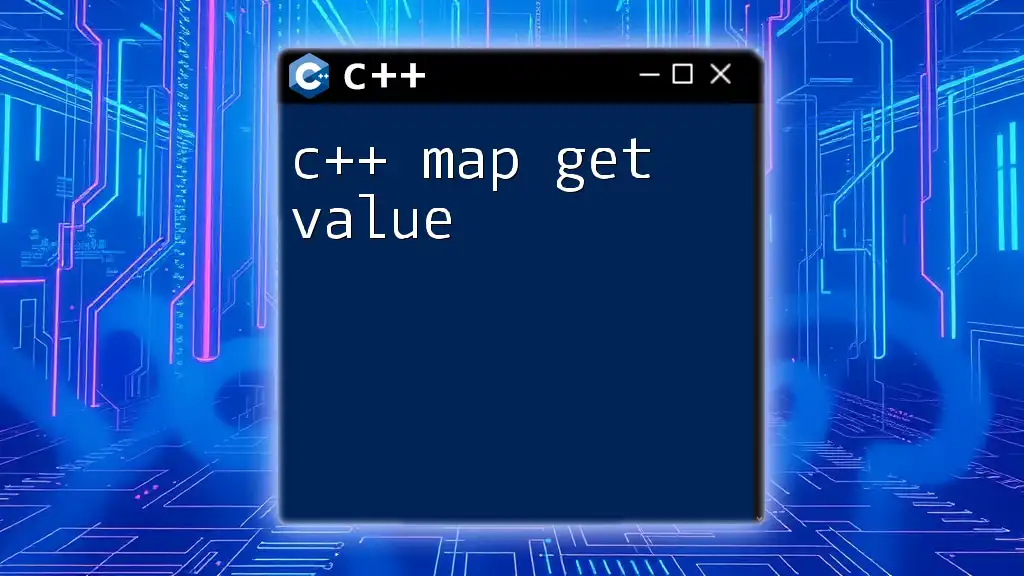
Conclusion
In summary, C++ pass by value is a fundamental concept that provides clarity and safety in function arguments. It allows for straightforward data manipulation while protecting original variables from modification. Recognizing the scenarios where it shines and the limitations it carries is essential for effective coding in C++. Practice implementing this concept alongside other parameter passing methods, and delve deeper into C++ to enhance your programming skill set.
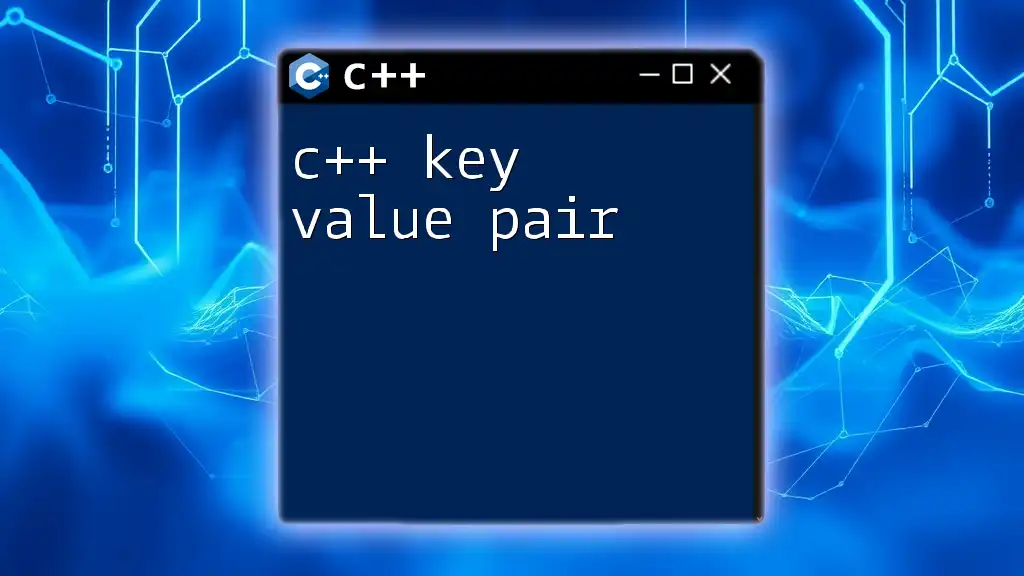
Further Reading and Resources
Recommended Books and Online Courses
- Explore resources that focus specifically on C++ and parameter passing techniques.
- Online platforms like Coursera, Udemy, and Codecademy provide comprehensive courses.
Community and Discussion Forums
Joining forums such as Stack Overflow can further your understanding and provide platforms for queries and discussions. Engaging with the C++ community can reinforce learning and offer insights from experienced programmers.