A C++ key-value pair is commonly represented using the `std::map` or `std::unordered_map` data structures, which store keys and their associated values for efficient retrieval.
Here’s a simple code snippet demonstrating how to use a `std::map` in C++:
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> ageMap;
ageMap["Alice"] = 30;
ageMap["Bob"] = 25;
for (const auto& pair : ageMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
What are Key-Value Pairs?
Key-value pairs are fundamental data structures that consist of two linked data items—keys and values. The key is a unique identifier, while the value is the data associated with that key. This association allows for efficient data retrieval, as you can directly access values using their corresponding keys.
Using key-value pairs is crucial in programming because they provide a way to organize and manage data meaningfully. They are instrumental in multiple scenarios, such as caching, databases, configuration settings, and even data processing.
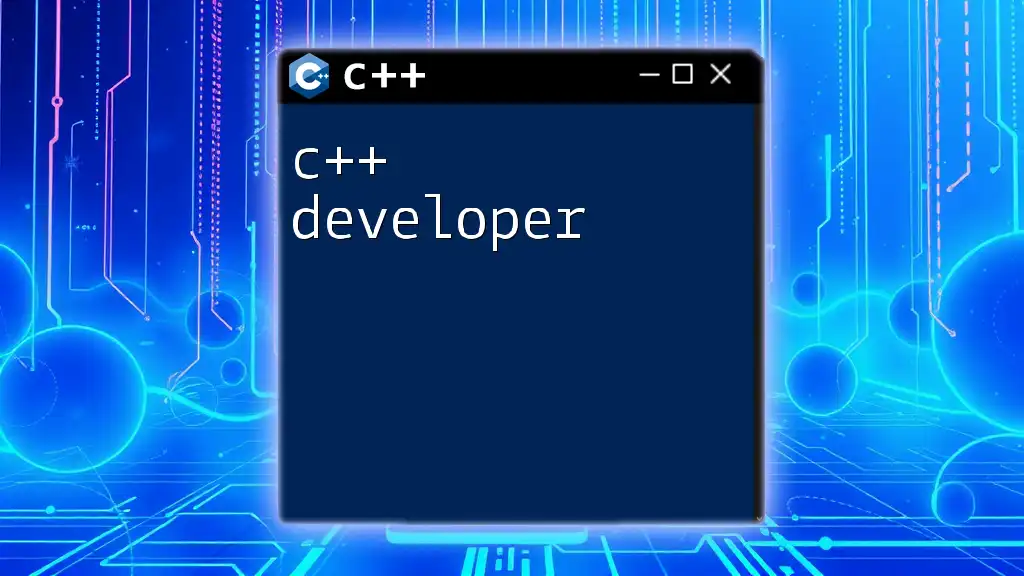
C++ STL Containers for Key-Value Pairs
C++ Standard Template Library (STL) provides two primary containers that enable the use of key-value pairs: `std::map` and `std::unordered_map`.
Using std::map
Overview of std::map
`std::map` is an associative container that maintains its elements in a specific order based on the keys. Each key must be unique, as duplicate keys are not allowed. The underlying structure is typically implemented as a red-black tree, allowing for efficient sorted access to elements.
Basic Usages
To create a simple mapping of student names to their grades, you might use `std::map` like this:
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> studentGrades;
studentGrades["Alice"] = 90;
studentGrades["Bob"] = 85;
std::cout << "Alice's grade: " << studentGrades["Alice"] << std::endl;
return 0;
}
This example demonstrates how to declare a map, insert key-value pairs, and retrieve values using their corresponding keys. The `operator[]` acts as a convenient syntax for accessing values through their keys directly.
Using std::unordered_map
Overview of std::unordered_map
In contrast, `std::unordered_map` is an associative container that uses a hash table for storage, allowing for average-time complexity of O(1) for lookups. However, this comes with the trade-off of unordered access, meaning the elements are not sorted based on their keys.
Basic Usages
You can leverage `std::unordered_map` similarly:
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<std::string, int> ageMap;
ageMap["John"] = 25;
ageMap["Doe"] = 30;
std::cout << "John's age: " << ageMap["John"] << std::endl;
return 0;
}
Key differences between `std::map` and `std::unordered_map` include their performance profiles. Choosing the appropriate type depends on your specific needs—whether you require ordered data or faster access.
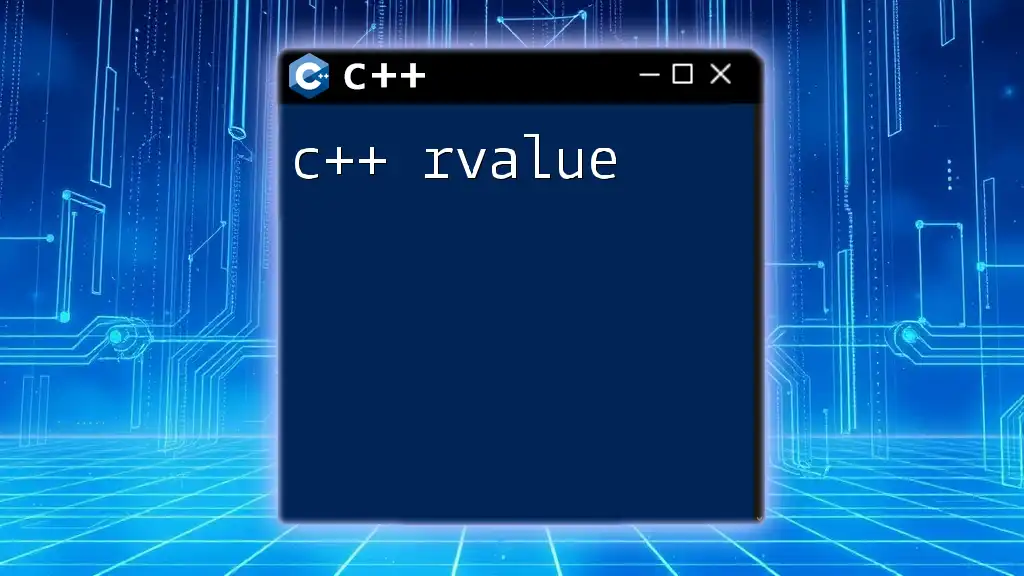
Operations on Key-Value Pairs
Insertion
Inserting elements into a map is straightforward. You can assign a value to a key directly. Both `std::map` and `std::unordered_map` support this operation seamlessly.
Accessing Values
Retrieving values associated with a specific key is done using the `operator[]`. To prevent potential issues when accessing non-existent keys in an unordered map, consider using the `.find()` method:
auto it = ageMap.find("Undefined");
if (it != ageMap.end()) {
std::cout << it->first << ": " << it->second << std::endl;
} else {
std::cout << "Key not found!" << std::endl;
}
Erasing Key-Value Pairs
You can remove key-value pairs using the `.erase()` method. For instance, to remove Bob's grade:
studentGrades.erase("Bob");
This command will efficiently remove the entry with the key "Bob" from the `studentGrades` map.
Iterating Over Key-Value Pairs
Iteration through all key-value pairs can be performed using a range-based for loop:
for (const auto& pair : studentGrades) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
This structure allows for clean and efficient access to both keys and values.
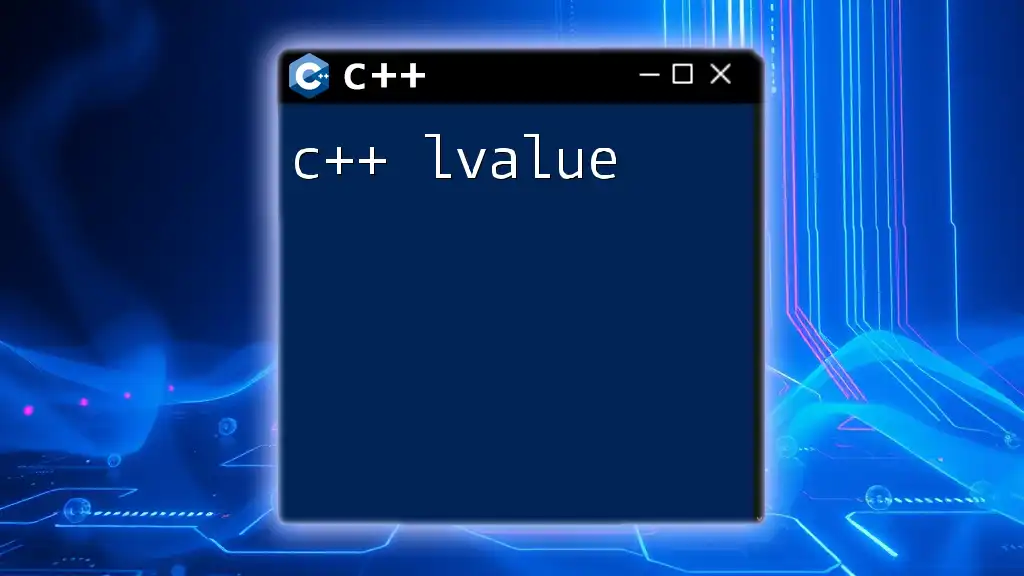
Advanced Usage of Key-Value Pairs
Custom Objects as Values
You can also use custom structures or classes as values. For example:
struct Student {
std::string name;
int grade;
};
std::map<int, Student> studentRecords;
studentRecords[1] = {"Alice", 90};
In this instance, each student is associated with an ID, allowing for more complex data types to be stored alongside their unique identifiers.
Nested Key-Value Pairs
C++ maps can contain other maps, accommodating nested key-value pair scenarios. For instance, if you want to map grades to subjects, you could structure it as follows:
std::map<std::string, std::map<std::string, int>> school;
school["Grade1"]["Math"] = 90;
This hierarchy allows you to organize data in an even more granular fashion.
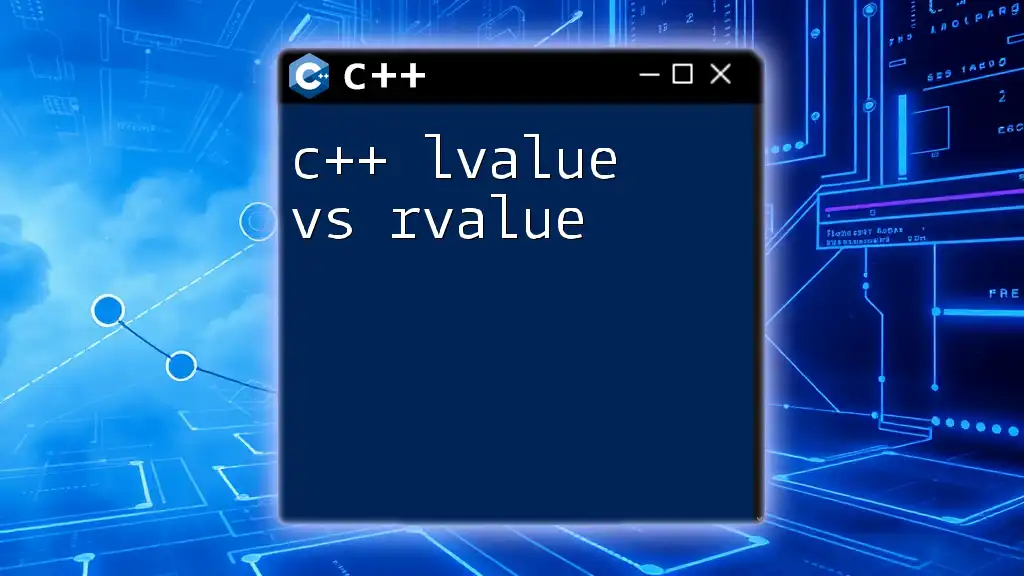
Performance Considerations
Complexity of Operations
When opting between `std::map` and `std::unordered_map`, understanding the complexity of operations becomes essential. While `std::map` offers logarithmic time complexity—O(log n)—for insertion, deletion, and search operations, `std::unordered_map` typically guarantees constant time complexity—O(1)—for these operations.
Memory Usage
Memory consumption varies between the two containers as well. `std::map` requires more memory overhead due to its tree structure, while `std::unordered_map` may require rehashing, which can lead to dynamic memory allocation.
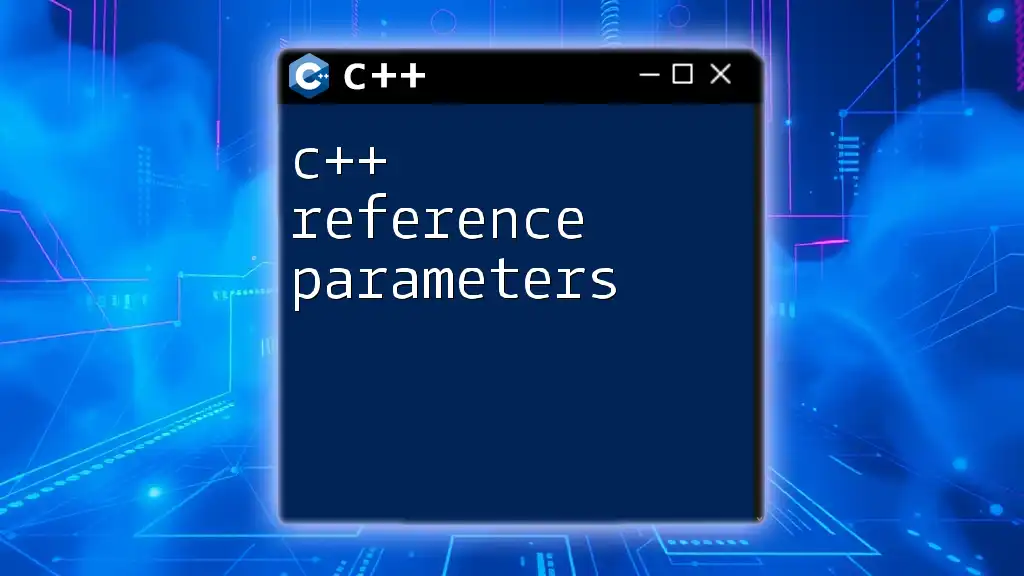
Common Mistakes to Avoid
Using the Wrong Container
Using the wrong container type can significantly impact your program's performance. For instance, if your application requires sorted access to keys, using `std::unordered_map` may lead to inefficiencies.
Key Collisions in Unordered Maps
In unordered maps, unique keys are essential. If you accidentally insert an entry with a key that already exists, the existing value will be overwritten without warning. Understanding how your keys are generated and ensuring their uniqueness is crucial.
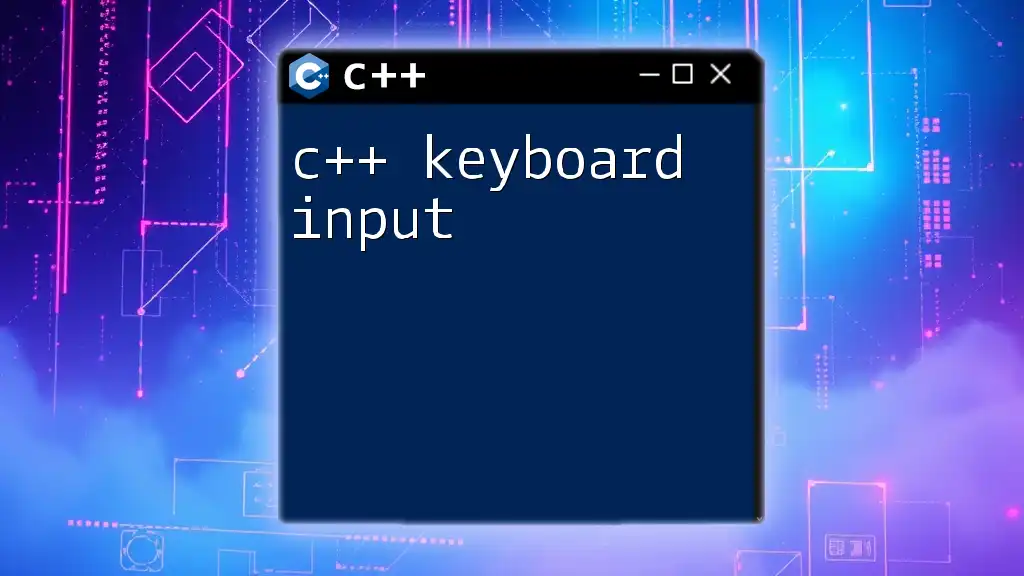
Conclusion
C++ key-value pairs, implemented through `std::map` and `std::unordered_map`, serve as foundational tools in the language's data handling arsenal. Mastering their use opens doors to better-organized code and enhanced performance in your applications. Whether you're building custom data structures or optimizing existing code, leveraging key-value pairs effectively is a skill worth developing.
As you continue your journey into C++, consider exploring further resources to solidify your understanding and capabilities.