In C++, keyboard input can be captured using the `cin` object from the standard input library to read data from the user.
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter a number: ";
cin >> number;
cout << "You entered: " << number << endl;
return 0;
}
Understanding Input in C++
What is Input in C++?
Input is a fundamental concept in programming that allows us to obtain data from users or other sources. In C++, input typically refers to the process of receiving data through keyboard commands or from files. Understanding input in C++ is crucial for creating interactive applications that respond to user interactions.
The Standard Input Stream
The most common mechanism for keyboard input in C++ is through the standard input stream, represented by `cin`. This object is defined in the `<iostream>` header and allows developers to read data entered by the user. The operations performed on the standard input stream allow us to gather information that can be processed in our programs.
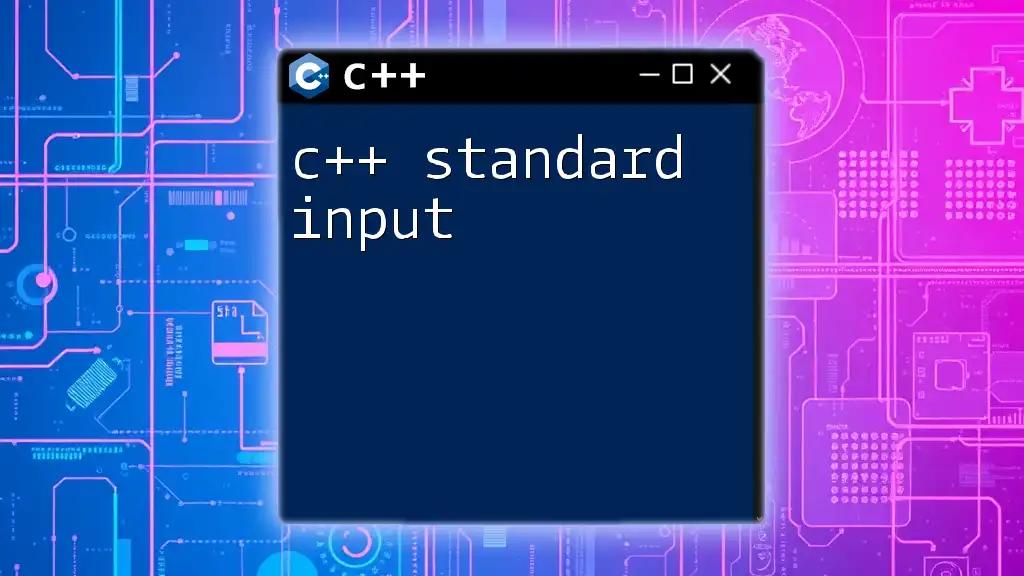
Basic Keyboard Input with `cin`
Using `cin` for Basic Input
The `cin` function is straightforward and allows us to read data of various types. Here’s a simple example to illustrate how to read a single integer value from the keyboard:
#include <iostream>
using namespace std;
int main() {
int num;
cout << "Enter an integer: ";
cin >> num;
cout << "You entered: " << num << endl;
return 0;
}
In this snippet, the user is prompted to enter an integer. The `cin >> num;` line reads the input and stores it in the variable `num`. After that, we output the value, confirming the input received.
Reading Different Data Types
In C++, `cin` can handle various data types. Here’s how to read integers, floating-point numbers, characters, and strings effectively.
Example for Reading Various Data Types:
#include <iostream>
#include <string>
using namespace std;
int main() {
float f;
cout << "Enter a float value: ";
cin >> f;
cout << "You entered: " << f << endl;
char c;
cout << "Enter a character: ";
cin >> c;
cout << "You entered: " << c << endl;
string str;
cout << "Enter a string: ";
cin >> str;
cout << "You entered: " << str << endl;
return 0;
}
In the code above, we demonstrate reading various types: a floating-point number with `float`, a single character with `char`, and a string with `string`. Each input prompt clearly indicates what the user needs to enter.
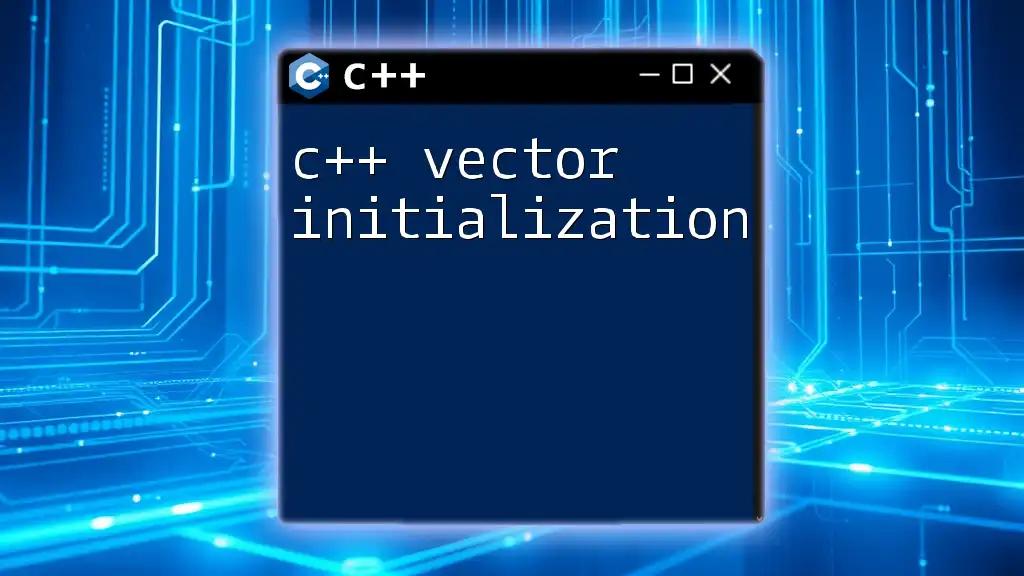
Handling Multiple Inputs
Reading Multiple Values at Once
`cin` allows you to read multiple values in one line, making your code more efficient and user-friendly. For example, if you wanted to read two integers at once, you can do it as follows:
#include <iostream>
using namespace std;
int main() {
int a, b;
cout << "Enter two integers: ";
cin >> a >> b;
cout << "You entered: " << a << " and " << b << endl;
return 0;
}
This approach allows for a more streamlined user interaction, enabling users to input multiple values seamlessly.
Using `cin.get()` for Character Input
For character input, `cin` and `cin.get()` serve different purposes. While `cin` discards whitespace, `cin.get()` reads everything, including spaces. Here’s how to use `cin.get()` effectively:
#include <iostream>
using namespace std;
int main() {
char ch;
cout << "Enter a character: ";
cin.get(ch);
cout << "You entered: " << ch << endl;
return 0;
}
This method is particularly useful when you need to capture input in its entirety without omitting spaces or line breaks.

Advanced Input Techniques
Reading Strings with Spaces
If you want to capture an entire line of input, including spaces, `getline()` is your best friend. Using `getline()` allows for capturing full sentences or phrases effortlessly:
#include <iostream>
#include <string>
using namespace std;
int main() {
string line;
cout << "Enter a full line: ";
getline(cin, line);
cout << "You entered: " << line << endl;
return 0;
}
In the code above, the user can input a full line without any limitation on spaces, making it extremely useful for applications requiring detailed input from users.
Input Validation Techniques
Validating input is essential to ensure that the data received is appropriate and expected. This can prevent your program from crashing or misbehaving due to invalid data types.
For example, checking if a user enters a valid age can be accomplished as follows:
#include <iostream>
#include <limits>
using namespace std;
int main() {
int age;
cout << "Enter your age: ";
while (!(cin >> age) || age < 0) {
cout << "Invalid input. Please enter a positive integer: ";
cin.clear(); // clear error flag
cin.ignore(numeric_limits<streamsize>::max(), '\n'); // ignore invalid input
}
cout << "Your age is: " << age << endl;
return 0;
}
In this example, we prompt the user to enter their age and ensure the input is a positive integer. If the user enters an invalid input, `cin.clear()` resets the state flags, and `cin.ignore()` discards the invalid input, allowing the user to try again.
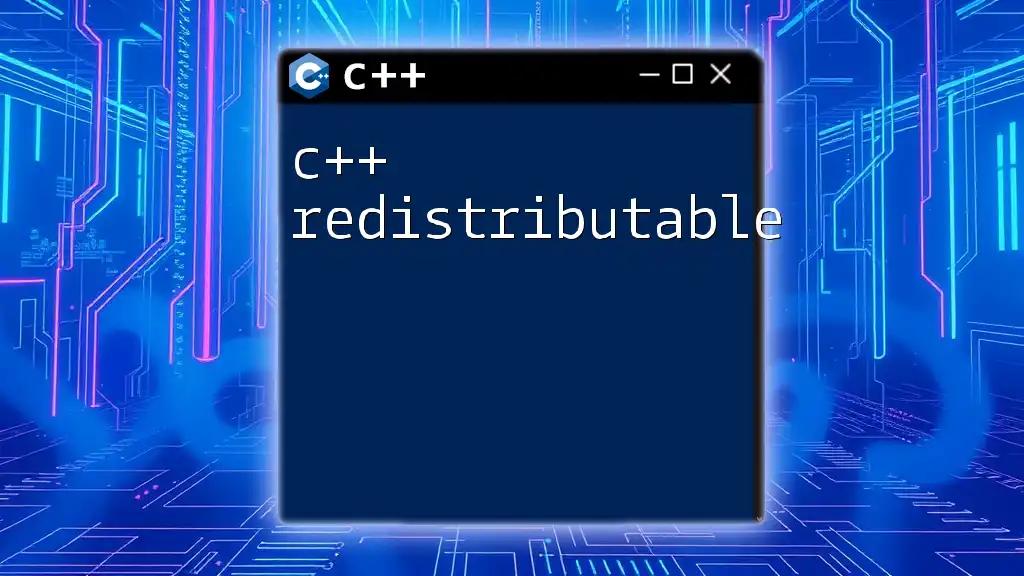
Common Input-Related Issues
Handling Errors with `cin`
Errors are common when dealing with user input. It’s crucial to understand how to handle these to maintain the proper functionality of your program. C++ provides several flags like `failbit` and `badbit` to indicate errors in the input process:
- `failbit`: This flag is set if input operation fails to extract a valid value.
- `badbit`: This flag is set if the input stream encounters a serious problem.
You can check for these flags using `cin.fail()` or `cin.bad()`, which will allow you to handle errors gracefully.
Clearing Input Buffer
Another pivotal aspect of handling user input is ensuring the input buffer is handled correctly to avoid unexpected behavior during input operations. Clearing the input buffer can be accomplished using:
cin.ignore(numeric_limits<streamsize>::max(), '\n');
This line ignores any characters remaining in the input stream until the newline character is reached, ensuring the next input operation is clean and accurate.
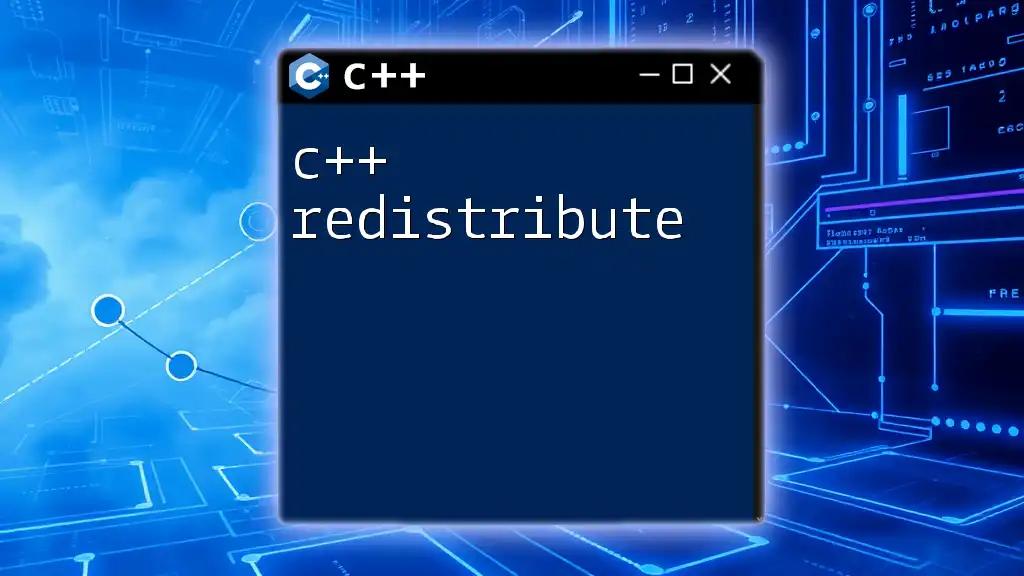
Conclusion
Understanding C++ keyboard input is essential for developing robust and interactive applications. By mastering various input methods and implementing proper validation techniques, you can enhance user experience significantly. Practicing these concepts will empower you to handle a wide array of input scenarios effectively, paving the way for more complex and capable C++ applications.

Additional Resources
To further enhance your knowledge of C++, consider exploring the official C++ documentation or enrolling in online courses dedicated to C++ programming. Engaging with community forums can also provide invaluable insights and support as you continue your programming journey.