In C++, the `std::vector` does not have a `push_front` member function, but you can mimic this functionality by inserting elements at the beginning using the `insert` method or by utilizing a `std::deque` instead.
Here’s how you can insert an element at the front of a `std::vector`:
#include <vector>
#include <iostream>
int main() {
std::vector<int> vec = {2, 3, 4}; // Initial vector
vec.insert(vec.begin(), 1); // Insert 1 at the front
for (int val : vec) {
std::cout << val << " "; // Output: 1 2 3 4
}
return 0;
}
Understanding C++ Vectors
What is a C++ Vector?
A C++ vector is an important component of the Standard Template Library (STL) that provides a flexible array-like structure. Unlike arrays, vectors can dynamically resize when elements are added or removed, making them an ideal choice for handling collections of data whose size may change during execution.
Importance of Vectors in C++
Vectors are particularly essential in modern C++ programming for several reasons:
- Dynamic Sizing: Unlike static arrays that have a fixed size, vectors grow and shrink dynamically, allowing for efficient memory usage.
- Ease of Use: Vectors provide a rich set of member functions for managing their contents, making them easier to work with than raw arrays.
- Performance: Vectors typically exhibit faster random access times compared to linked lists and are well-optimized for various tasks.
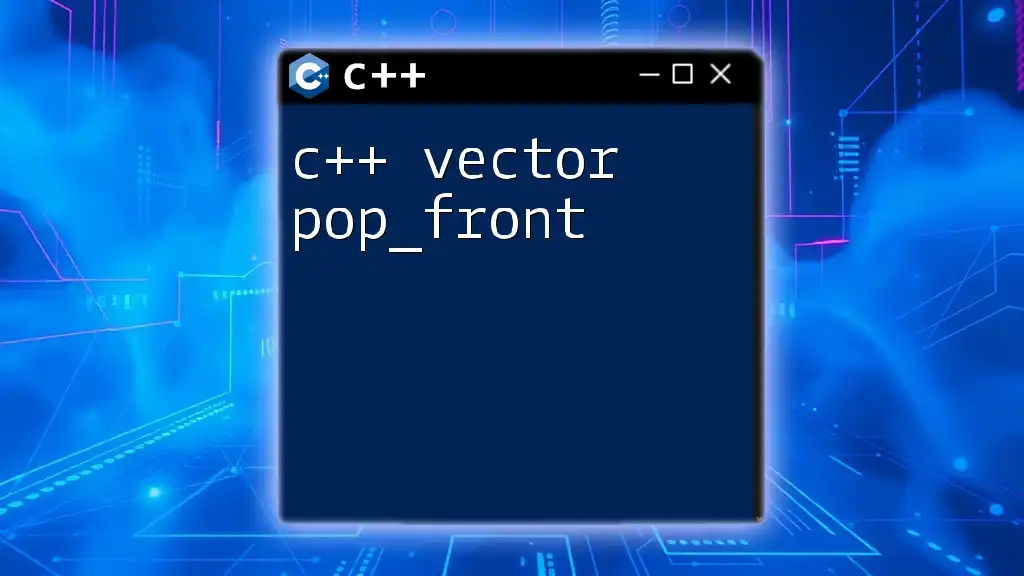
Understanding the push_front Concept
Introduction to push_front
The `push_front` operation is commonly found in data structures like deques but is not directly supported by `std::vector`. Its purpose is to insert an element at the front of the container, shifting existing elements to the right.
Why Use push_front?
Utilizing `push_front` can be advantageous in scenarios where the most recently added elements must be accessed first. For instance, implementing a queue system where new arrivals push older entries back. While vectors excel at random access and append operations, inserting elements at the front repeatedly can lead to inefficiencies due to the need for shifting existing elements.
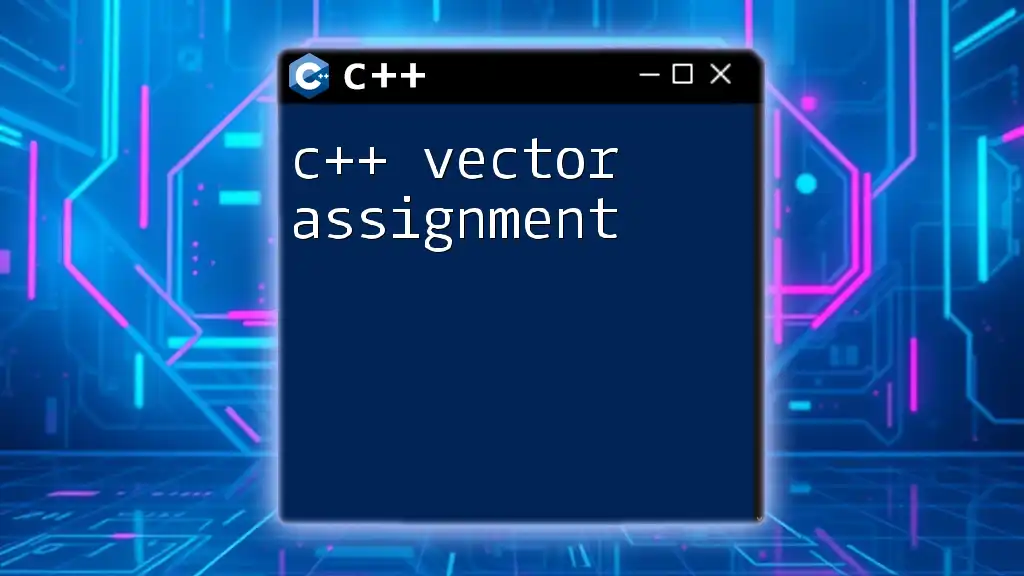
Implementing push_front in C++
C++ Vectors Do Not Directly Support push_front
The `std::vector` class does not have a built-in `push_front` method due to its nature of dynamic resizing. The insertion at the beginning of a vector is computationally expensive, as it involves moving all the existing elements one index forward. This results in a time complexity of O(n) for this operation.
Recommended Alternatives
Using std::deque
The `std::deque` (double-ended queue) is a robust alternative that provides the `push_front` functionality efficiently. This data structure allows you to add and remove elements from both ends with constant time complexity. Here’s how you can implement `push_front` using `std::deque`:
#include <iostream>
#include <deque>
int main() {
std::deque<int> myDeque;
myDeque.push_front(10);
myDeque.push_front(20);
for (int n : myDeque) {
std::cout << n << " "; // Output: 20 10
}
return 0;
}
In this snippet, we create a deque, add elements using `push_front`, and print the contents, successfully demonstrating how to have the most recently pushed item at the front.
Manual Implementation of push_front in std::vector
If you still prefer using `std::vector`, you can manually implement the `push_front` functionality through the `insert` function. While this method incurs the overhead of moving elements, it is still useful when vectors are your only option:
#include <iostream>
#include <vector>
void push_front(std::vector<int>& vec, int value) {
vec.insert(vec.begin(), value); // Inserting at the beginning
}
int main() {
std::vector<int> myVector = {30, 40, 50};
push_front(myVector, 20);
for (int n : myVector) {
std::cout << n << " "; // Output: 20 30 40 50
}
return 0;
}
Here, the `insert` method facilitates adding a new integer at the front of a vector, allowing for an example of the desired functionality.
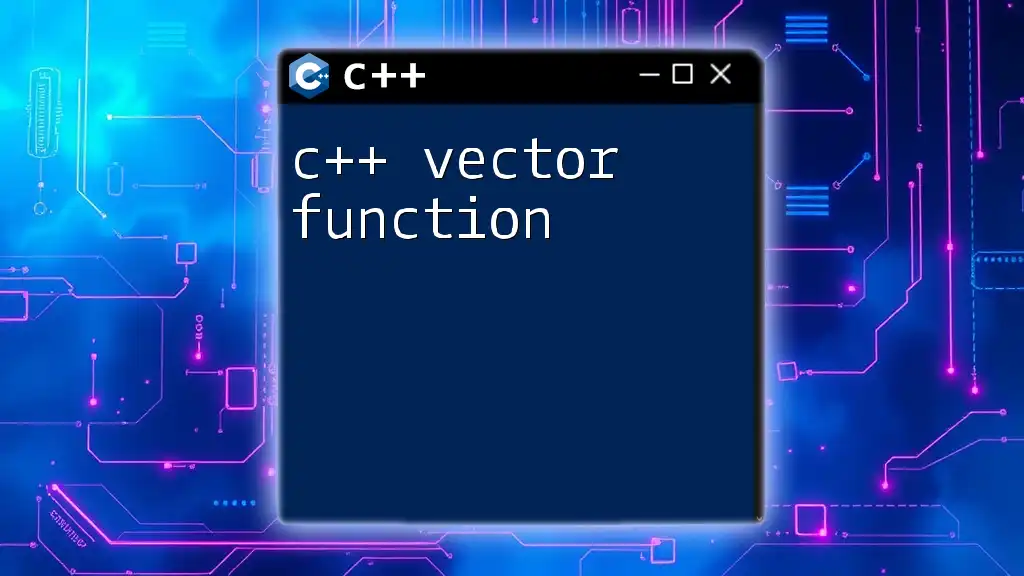
When to Choose Vector vs Deque
Performance Comparison
When deciding between `std::vector` and `std::deque`, performance is a crucial factor.
- For `std::vector`, both `push_back` and `pop_back` come with an average time complexity of O(1). However, `push_front` is O(n), as shown in previous examples.
- Conversely, `std::deque` offers O(1) performance for both `push_front` and `push_back`, making it a better choice for applications that require frequent additions or removals from both ends of the container.
Use Cases
- Vectors: Use vectors when you have a collection where most additions happen toward the end and when accessing elements randomly is essential.
- Deques: If you frequently need to add or remove items from both ends or if the data structure's size may vary rapidly, a deque is more appropriate.
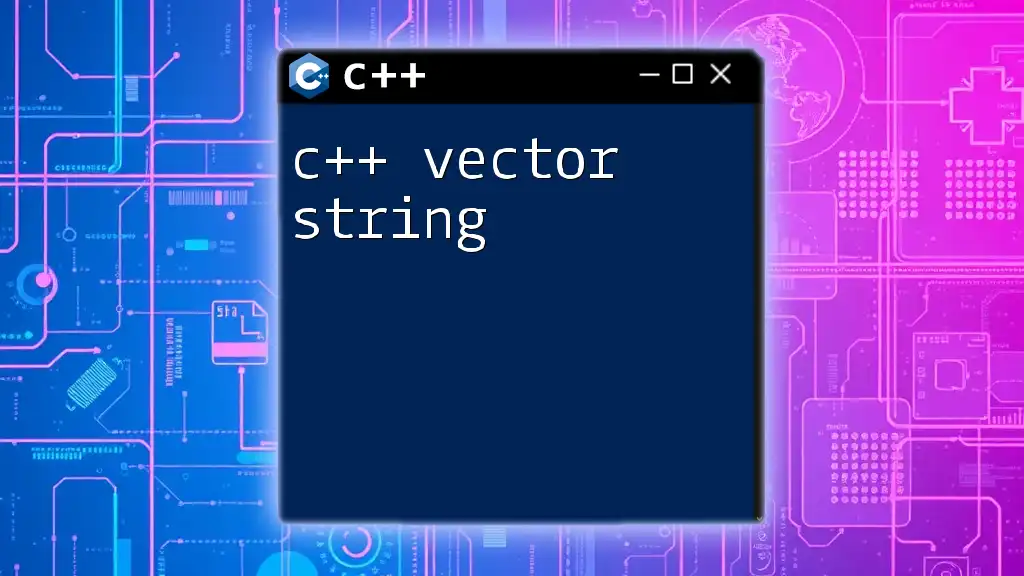
Best Practices for Handling C++ Vectors
Memory Management
When using dynamic structures like vectors, memory management is paramount. Since every insertion at the front may cause a reallocation and copying of elements (if the initial capacity is exceeded), it is advisable to reserve capacity during initialization if you anticipate growth. This strategy minimizes reallocations:
std::vector<int> vec;
vec.reserve(expected_size); // Reserve memory upfront
Common Pitfalls
While vectors are user-friendly, there are certain pitfalls to be aware of:
- Frequent push_front Operations: Overusing `push_front` in a vector can lead to inefficient memory use and performance slowdowns. If your application logic often requires adding elements at the beginning, consider switching to a more suitable data structure like `std::deque`.
- Out-of-Bounds Access: As with all containers, always ensure that indices are valid when accessing elements to prevent runtime errors.
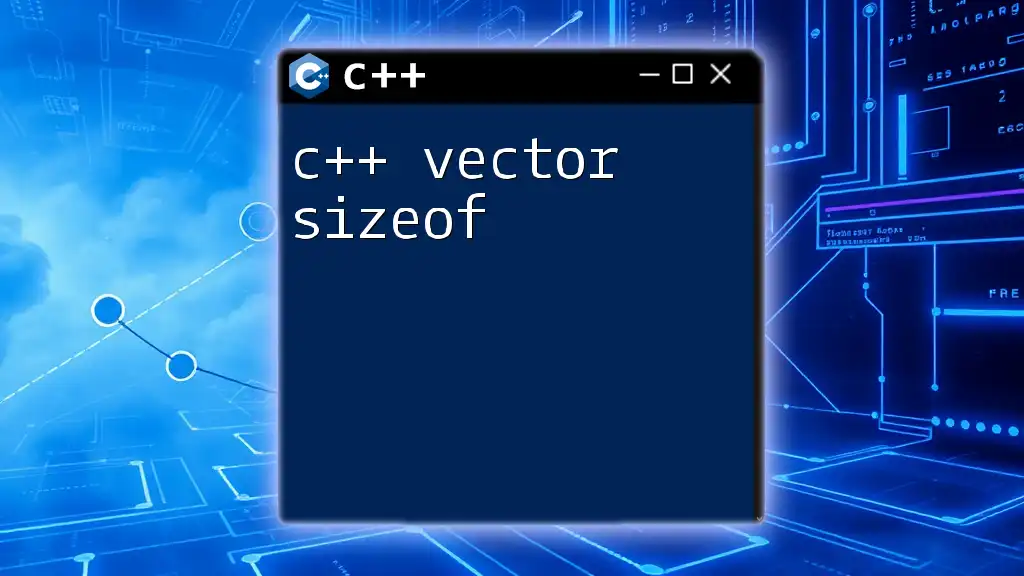
Conclusion
Summary of Key Points
In summary, while the C++ vector push_front operation is not natively supported, there are ways to achieve desired behavior through either `std::deque` or custom implementations using `std::vector`. Understanding the constraints and strengths of these data structures allows developers to select the most efficient and appropriate option based on their specific needs.
Encouragement to Experiment
We encourage you to try the code examples provided in this guide. Experiment with both vectors and deques to see how they fit into your programming style. Understanding when and how to use these data structures effectively is key to mastering C++ programming.
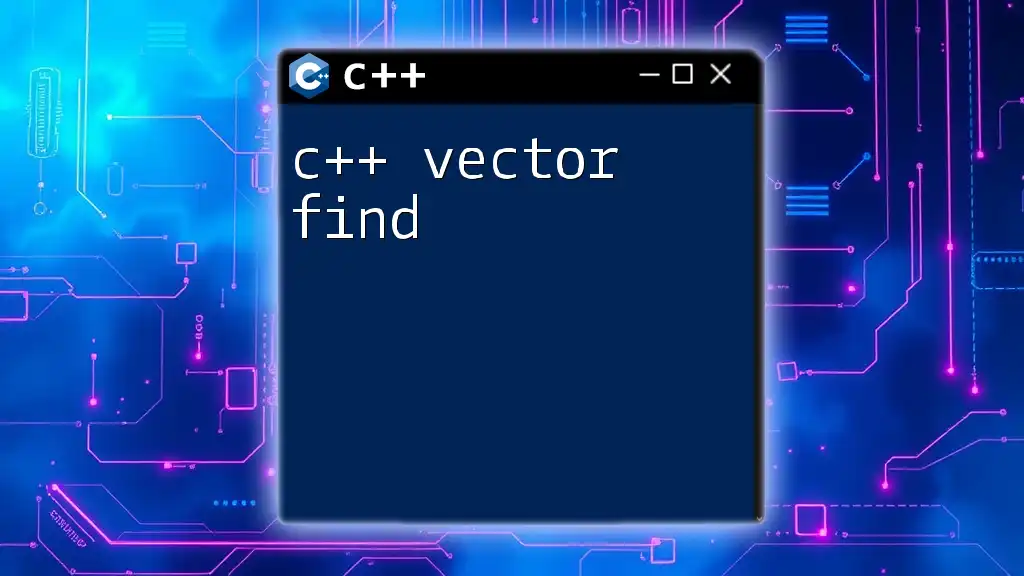
Additional Resources
Recommended Reading
Explore additional C++ books and online resources to deepen your understanding and keep your skills sharp.
Community Engagement
We invite you to ask questions and share your experiences with implementing push_front in your projects. Engaging with the community is a great way to learn and grow in your C++ journey.